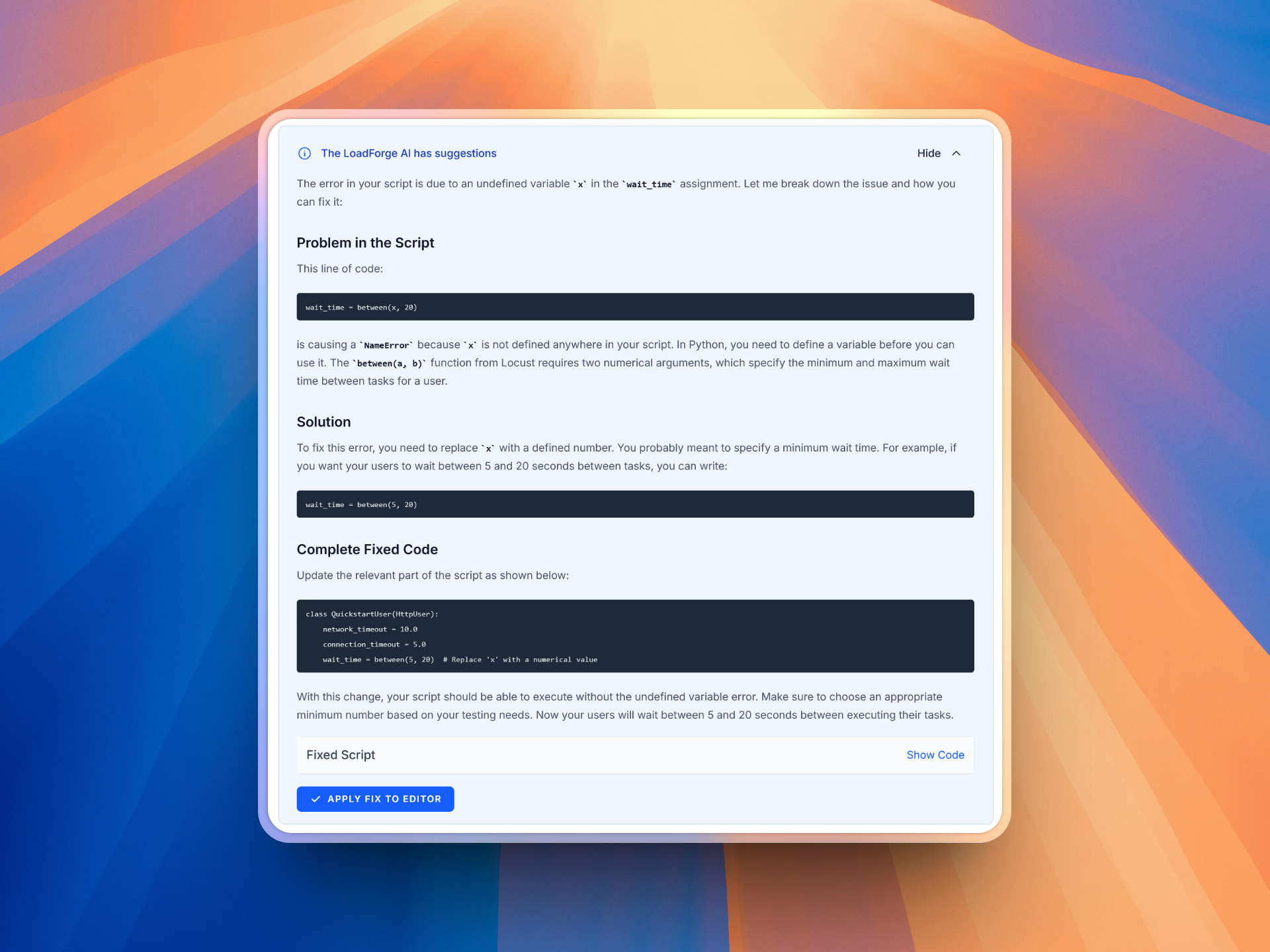
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
In the realm of modern web applications, optimizing static assets is essential for achieving superior performance, an enhanced user experience, and robust scalability. Phoenix, a powerful web framework built with Elixir, provides a solid foundation for developing high-performance web applications....
In the realm of modern web applications, optimizing static assets is essential for achieving superior performance, an enhanced user experience, and robust scalability. Phoenix, a powerful web framework built with Elixir, provides a solid foundation for developing high-performance web applications. However, the way static assets—such as CSS, JavaScript, images, and fonts—are handled can significantly impact the overall efficiency and responsiveness of your app.
Optimizing these assets is not just about reducing load times; it also plays a pivotal role in improving your application's scalability. Efficient management of static assets ensures that your server resources are used judiciously, allowing your Phoenix application to handle more concurrent users without degrading performance. Furthermore, a finely-tuned asset pipeline can enhance user satisfaction by delivering faster load times, thereby reducing bounce rates and increasing engagement.
In this guide, we will explore a comprehensive set of strategies and best practices to optimize static assets in Phoenix applications. We'll delve into various techniques and tools to ensure that your static resources are served efficiently and effectively. Here’s an overview of what you can expect:
Understanding Static Assets in Phoenix:
Compiling and Minifying Assets:
Leveraging Browser Caching:
Using a Content Delivery Network (CDN):
Image Optimization Techniques:
Serving Compressed Files:
Tips for Efficient Asset Management:
Monitoring Performance with Load Testing:
By the end of this guide, you'll have a comprehensive understanding of how to optimize static assets in your Phoenix applications, resulting in faster load times, better user experiences, and scalable architectures. Let's embark on this journey to perfect your Phoenix application's performance!
In the context of Phoenix applications, static assets refer to the files that are served directly to the client without requiring any server-side processing. These assets typically include CSS, JavaScript, images, and fonts. Properly managing these static assets is crucial for delivering a swift and seamless user experience.
Here's a brief breakdown of common static assets in a Phoenix application:
Phoenix uses a straightforward approach to manage static assets. By default, these assets are stored in the priv/static
directory and served directly to the client. The Plug.Static
plug in Phoenix serves files from the priv/static
directory efficiently.
priv
└── static
├── css
│ └── app.css
├── js
│ └── app.js
├── images
│ └── logo.png
└── fonts
└── custom-font.woff
In a newly generated Phoenix project, you will find the most basic static assets setup. But for production, you'll likely need a more complex setup to handle compiling, minification, and other optimizations.
Plug.Static
PlugThe Plug.Static
plug serves as the middleware responsible for serving static files. By default, it's typically included in the endpoint configuration of a Phoenix app.
Here's a snippet from the Endpoint
module:
defmodule MyAppWeb.Endpoint do
use Phoenix.Endpoint, otp_app: :my_app
# Serve at "/" the static files from "priv/static" directory.
plug Plug.Static,
at: "/",
from: :my_app,
gzip: false,
only: ~w(css fonts images js favicon.ico robots.txt)
# Other plugs...
end
In this section, we've defined what static assets entail within Phoenix applications—covering CSS, JavaScript, images, and fonts. We've also touched upon how Phoenix handles these assets out of the box using the priv/static
directory and the Plug.Static
plug. In subsequent sections, we’ll delve into various techniques for optimizing these static assets, including compilation, minification, caching strategies, and more. This foundation will help you better understand the subsequent optimizations we’ll discuss to enhance the performance, scalability, and user experience of your Phoenix applications.
Optimizing your static assets by compiling and minifying them is a crucial step in improving the performance of your Phoenix application. This not only reduces the size of your CSS and JavaScript files, leading to faster load times, but also helps in organizing and managing your assets more efficiently. In this section, we'll guide you through the process using tools like Brunch and Webpack.
By default, Phoenix projects come configured with Brunch, a lightweight build tool. Here’s how you can leverage Brunch to compile and minify your CSS and JavaScript files:
node -v
npm -v
brunch-config.js
, is located in the root of your Phoenix project. To enable minification, you need to set up production-specific settings:
exports.config = {
files: {
javascripts: {
joinTo: "js/app.js"
},
stylesheets: {
joinTo: "css/app.css"
}
},
plugins: {
babel: {
presets: ["@babel/preset-env"]
},
terser: {
ecma: 6,
compress: {
global_defs: {
DEBUG: false
}
}
},
cssnano: {
preset: 'default'
}
}
};
The terser
plugin is used for JavaScript minification, and cssnano
is used for CSS minification.
npm run deploy
This will generate minified versions of your CSS and JavaScript files in the priv/static
directory.While Brunch is the default, using Webpack can provide more flexibility and features. Here's how you can set up Webpack in a Phoenix project:
Install Webpack and Dependencies: Install Webpack along with necessary plugins:
npm install --save-dev webpack webpack-cli babel-loader @babel/core @babel/preset-env css-loader style-loader terser-webpack-plugin css-minimizer-webpack-plugin
Configure Webpack:
Create a webpack.config.js
file in your project root:
const TerserPlugin = require('terser-webpack-plugin');
const CssMinimizerPlugin = require('css-minimizer-webpack-plugin');
module.exports = {
mode: 'production',
entry: './assets/js/app.js',
output: {
filename: 'js/app.js',
path: __dirname + '/priv/static'
},
module: {
rules: [
{
test: /.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env']
}
}
},
{
test: /.css$/,
use: [
'style-loader',
'css-loader'
]
}
]
},
optimization: {
minimize: true,
minimizer: [
new TerserPlugin(),
new CssMinimizerPlugin()
]
}
};
This configuration sets up Webpack for handling JavaScript and CSS files, enabling minification through TerserPlugin
and CssMinimizerPlugin
.
npx webpack --config webpack.config.js
Your minified assets will be generated and stored in the priv/static
directory.Minification is the process of removing unnecessary characters (like whitespace, comments, and line breaks) from the code without altering its functionality. Here are some key benefits:
By compiling and minifying your static assets, you can ensure that your Phoenix application runs more efficiently, providing a smoother experience for your users. In the next sections, we'll explore additional techniques to optimize your static assets further.
Browser caching is a powerful technique to enhance the performance of your Phoenix application by storing static assets locally on users' devices. By configuring your app to utilize browser caching efficiently, you can significantly reduce load times, decrease server load, and improve the overall user experience. This section explores the concept of browser caching and how to configure caching headers in Phoenix to ensure static assets are cached effectively.
When a user visits your application, the browser fetches all necessary resources such as CSS, JavaScript, images, and fonts. Each time the user navigates to a new page, the browser may need to download these assets again, leading to unnecessary delays and increased server load. Browser caching allows the browser to store these resources locally after the first download, so subsequent visits are much faster as the assets are retrieved from the local cache rather than the server.
To make use of browser caching, you need to instruct browsers on how long they should cache a particular resource. This is done by setting caching headers in your Phoenix application. Phoenix relies on plug.Static
to serve static assets, and you can configure cache headers through this module.
Here’s how you can configure plug.Static
for efficient browser caching:
Open your Phoenix application's endpoint file, usually found at lib/my_app_web/endpoint.ex
.
Locate the plug.Static
configuration.
Modify the cache_control_for_etags
and cache_control_for_vsn_requests
attributes to specify the caching duration.
Here is an example configuration to cache static assets for one year:
defmodule MyAppWeb.Endpoint do
use Phoenix.Endpoint, otp_app: :my_app
plug Plug.Static,
at: "/",
from: :my_app,
gzip: false,
cache_control_for_etags: "public, max-age=31536000",
cache_control_for_vsn_requests: "public, max-age=31536000"
# Other plugs and endpoint configurations
end
cache_control_for_etags
: Applies cache control headers to files that have an ETag, which helps in validating if a cached resource has changed.cache_control_for_vsn_requests
: Applies to versioned requests (usually with ?vsn=XYZ
parameter) and helps in controlling the cache lifetime.After configuring the cache headers, it’s essential to ensure they work as expected. You can use browser developer tools to inspect the HTTP headers returned with your static assets. Here’s how you can do it in popular browsers:
Open Developer Tools: Right-click on your application page and select "Inspect" or press Ctrl+Shift+I
/Cmd+Opt+I
.
Go to Network Tab: Navigate to the "Network" tab.
Reload the Page: Refresh the page and look for static asset requests.
Inspect Headers: Click on individual assets and look for the Cache-Control
header under the "Headers" section.
The Cache-Control
header should reflect your configuration, indicating that the resources will be cached for the specified duration.
By leveraging browser caching, you can:
In conclusion, effective use of browser caching can dramatically improve the performance and scalability of your Phoenix application. With the proper configuration, your static assets will load quickly, providing a better user experience and reducing the strain on your servers.
Optimizing the performance of static assets in your Phoenix application can significantly enhance user experience and scalability. One of the most effective strategies for achieving this is using a Content Delivery Network (CDN). A CDN is a distributed network of servers that delivers content to users based on their geographic location, effectively reducing latency and improving load times. In this section, we will demonstrate how to set up and configure a CDN for serving static assets in Phoenix applications and discuss the various advantages of using a CDN.
Before diving into the setup, it's important to understand the benefits of integrating a CDN with your Phoenix application:
Setting up a CDN requires modifying your application's configuration to ensure that static assets are served through the CDN. Below are the steps to achieve this:
There are several CDN providers available, such as Cloudflare, Amazon CloudFront, and Fastly. For this guide, we'll use Amazon CloudFront as an example. The steps will be similar regardless of the provider you choose.
Sign Up and Create a Distribution:
Configure Caching and Behavior Settings:
Cache-Control
headers in your Phoenix application to manage this.Get the CDN URL:
https://<distribution_id>.cloudfront.net
.You need to update your Phoenix application's configuration to use the CDN URL for serving static assets. Here's how you can do it:
Modify Endpoint Configuration:
config/prod.exs
.static_url
configuration to point to your CDN URL.config :my_app, MyAppWeb.Endpoint,
url: [host: "example.com", port: 443],
cache_static_manifest: "priv/static/cache_manifest.json",
static_url: [scheme: "https", host: "<your_cdn_domain>", port: 443]
Asset Building:
After configuring your Phoenix application to use the CDN, it’s crucial to verify that everything is working correctly:
Clear Browser Cache:
Inspect Network Requests:
Monitor Performance:
By integrating a CDN with your Phoenix application, you can leverage a powerful tool to reduce latency, improve performance, and ensure better scalability. This setup not only enhances the user experience but also takes a significant load off your primary servers, allowing them to handle more critical tasks efficiently.
In the following sections, we will explore additional techniques such as image optimization and serving compressed files to further optimize your Phoenix application.
Optimizing images is crucial for enhancing load times, reducing bandwidth consumption, and improving the overall user experience in Phoenix applications. Here we explore various techniques and tools to optimize images, ensuring they are efficiently delivered to users.
Choosing the right image format is the first step in optimizing images. Different formats are better suited for different types of images:
By utilizing appropriate formats, you can significantly reduce file sizes without compromising quality.
Image compression tools help reduce file sizes even further. There are two types of compression: lossy and lossless. Lossy compression reduces file size by eliminating some data, which might affect image quality, while lossless compression reduces size without any quality loss.
Here are some popular tools for both:
Imagemin: A powerful tool for compressing images via a range of plugins.
To use Imagemin with Phoenix, add Imagemin to your build process:
npm install imagemin imagemin-mozjpeg imagemin-pngquant imagemin-svgo --save-dev
Then, configure it in your asset build script:
const imagemin = require('imagemin');
const imageminMozjpeg = require('imagemin-mozjpeg'); const imageminPngquant = require('imagemin-pngquant'); const imageminSvgo = require('imagemin-svgo');
(async () => { await imagemin(['static/images/*.{jpg,png,svg}'], { destination: 'priv/static/images', plugins: [ imageminMozjpeg({quality: 75}), imageminPngquant({quality: [0.6, 0.8]}), imageminSvgo() ] }); })();
Delivering appropriately sized images based on the user's device can also lead to significant performance improvements. HTML5 introduced the srcset
attribute, which allows you to define different image sources for various screen sizes and resolutions.
<img srcset="images/hero-small.jpg 500w,
images/hero-medium.jpg 1000w,
images/hero-large.jpg 2000w"
sizes="(max-width: 600px) 500px,
(max-width: 1200px) 1000px,
2000px"
src="images/hero-large.jpg"
alt="Hero Image">
By default, browsers load all images on a page as soon as the page loads, which can slow down performance. Lazy loading defers the loading of off-screen images until the user scrolls near them.
With native lazy loading in HTML:
<img src="images/large-image.jpg" loading="lazy" alt="Lazy Loaded Image">
This simple attribute addition can boost your page load speeds significantly.
Integrating image optimization into your build process ensures that your images are always efficiently compressed and optimized. Using tools like webpack, you can automate the entire process.
For example, using the image-webpack-loader
for Webpack:
npm install image-webpack-loader --save-dev
Add the loader to your Webpack configuration:
module.exports = {
module: {
rules: [
{
test: /\.(png|jpe?g|gif|svg)$/i,
use: [
{
loader: 'file-loader',
},
{
loader: 'image-webpack-loader',
options: {
mozjpeg: {
progressive: true,
quality: 75
},
optipng: {
enabled: true,
},
pngquant: {
quality: [0.65, 0.90],
speed: 4
},
svgo: {
plugins: [
{ removeViewBox: false }
]
}
}
},
],
},
],
},
};
By incorporating these practices and tools into your Phoenix application, you can ensure that images are optimized for both performance and quality, contributing to a faster, more responsive user experience.
When it comes to optimizing static assets for your Phoenix application, serving compressed files such as gzip or Brotli can significantly reduce the size of your assets during transfer, leading to quicker load times and a better overall user experience. In this section, we will explore how to enable and configure gzip and Brotli compression in your Phoenix application.
Compressed files provide several benefits:
Phoenix comes with built-in support for gzip compression. To enable gzip compression for your static assets, you need to update your endpoint.ex
configuration file.
Update Endpoint Configuration:
Open your lib/my_app_web/endpoint.ex
file and add :gzip
to the Plug.Static
configuration:
plug Plug.Static,
at: "/",
from: :my_app,
gzip: true,
only: ~w(css fonts images js favicon.ico robots.txt)
HTTP Server Configuration:
Ensure that your HTTP server is configured to serve compressed files. For example, if you are using Cowboy as your server, update your config/prod.exs
:
config :my_app, MyAppWeb.Endpoint,
http: [compress: true, port: 4000],
...
To serve Brotli-compressed files, you will need to use an external tool to pre-compress your static assets and then configure Phoenix to serve these files.
Install Brotli:
Depending on your environment, you can install Brotli using package managers such as Homebrew for macOS:
brew install brotli
Pre-compress Assets:
After installing Brotli, you can create a script to pre-compress your static assets. You can add this script as part of your build process:
find priv/static -type f -iname "*.js" -exec brotli --best {} \;
find priv/static -type f -iname "*.css" -exec brotli --best {} \;
find priv/static -type f -iname "*.html" -exec brotli --best {} \;
Update Endpoint Configuration:
Modify your endpoint configuration to serve Brotli-compressed files. You'll need to add a custom Plug to check for Brotli files and serve them if they exist:
defmodule MyAppWeb.BrotliPlug do
import Plug.Conn
def init(default), do: default
def call(conn, _opts) do
path = conn.request_path <> ".br"
if File.exists?(path) do
conn
|> put_resp_header("content-encoding", "br")
|> send_resp(200, File.read!(path))
|> halt()
else
conn
end
end
end
plug MyAppWeb.BrotliPlug
By enabling gzip and Brotli compression, you can achieve significant performance improvements for your Phoenix applications. Gzip is straightforward to enable out-of-the-box with Phoenix while Brotli, which offers superior compression rates, may require additional steps for pre-compressing files. Both methods can substantially enhance user experience by reducing asset load times and conserving bandwidth usage.
Review these configurations periodically and monitor your application's performance using LoadForge to ensure that your optimizations remain effective under varying traffic conditions.
Efficient asset management is crucial for maintaining the performance and scalability of your Phoenix application. Below are some tips and best practices for managing static assets effectively to ensure your application remains optimized and maintainable.
Properly organizing your static files helps keep your codebase clean and manageable. Here are some strategies:
css
, js
, images
, fonts
). This makes it easier to locate and manage specific assets.Your directory structure might look something like this:
assets/
css/
main.css
admin.css
js/
app.js
utils.js
images/
logo.png
favicon.ico
fonts/
Regularly auditing your static assets can help identify unnecessary files, outdated resources, and opportunities for optimization:
Automated build tools streamline the process of managing, compiling, and optimizing static assets. Here are some popular tools and how they can assist:
// webpack.config.js
module.exports = {
entry: './assets/js/app.js',
output: {
filename: 'bundle.js',
path: __dirname + '/priv/static/js'
},
module: {
rules: [
{
test: /\.css$/,
use: ['style-loader', 'css-loader']
},
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader'
}
}
]
}
};
// brunch-config.js
exports.files = {
javascripts: {
joinTo: 'js/app.js'
},
stylesheets: {
joinTo: 'css/app.css'
}
};
exports.plugins = {
babel: {presets: ['@babel/preset-env']}
};
// gulpfile.js
const gulp = require('gulp');
const cssmin = require('gulp-cssmin');
const rename = require('gulp-rename');
gulp.task('minify-css', function() {
return gulp.src('./assets/css/*.css')
.pipe(cssmin())
.pipe(rename({suffix: '.min'}))
.pipe(gulp.dest('./priv/static/css'));
});
Efficiently managing static assets in Phoenix applications involves a combination of good organization, regular auditing, and leveraging powerful build tools. By implementing these tips, you can ensure your assets are optimized, your application performs efficiently, and your codebase remains maintainable.
After implementing various optimizations for static assets in your Phoenix application, it's crucial to ensure these changes effectively improve performance under real-world traffic conditions. Load testing offers valuable insights into how your application behaves under stress, identifies potential bottlenecks, and verifies the efficacy of your optimizations. In this section, we'll guide you through using LoadForge to perform comprehensive load testing on your Phoenix application.
Load testing simulates user traffic on your application to measure performance metrics such as response time, throughput, and resource utilization. By stress-testing your application, you can:
LoadForge is a powerful load testing tool that makes it easy to simulate user load and collect performance data for your Phoenix application. Follow these steps to set up and execute load tests using LoadForge:
First, sign up for a LoadForge account if you haven't already. Visit LoadForge and complete the registration process.
A well-defined test plan is crucial for effective load testing. Consider the following parameters:
Once your test plan is ready, configure your test in LoadForge:
After configuring your test, start the load test by clicking the "Run Test" button. LoadForge will begin simulating traffic to your Phoenix application, and you can monitor the progress in real-time.
Upon completion, LoadForge provides detailed reports and metrics. Focus on the following key performance indicators (KPIs):
Inspect these metrics to determine the effectiveness of your static asset optimizations. Look for:
You might want to customize your load test scenarios using LoadForge’s scripting capabilities. Here’s an example of a simple load testing script targeting your Phoenix application's static assets:
// LoadForge scripting example to test static assets
let request = new Request("GET", "https://yourdomain.com/assets/app.js");
let response = http.send(request);
check(response, {
"Status is 200": (r) => r.status === 200,
"Response time is below 200ms": (r) => r.time < 200
});
// Add additional requests for other static assets as needed
Load testing should not be a one-time activity. Regularly schedule load tests, especially after making significant changes or optimizations to your application. Continuous monitoring helps identify and address performance regressions early, ensuring that your Phoenix application remains performant and scalable.
By systematically load testing your Phoenix application using LoadForge, you can ensure that your static asset optimizations lead to tangible performance improvements. This ongoing process of testing, analyzing, and iterating will help maintain a high-performance, scalable application that delivers an excellent user experience even under heavy traffic conditions.
Optimizing static assets in Phoenix applications is crucial for achieving superior performance, enhanced user experience, and improved scalability. Throughout this guide, we've delved into various strategies and best practices to help you streamline and optimize your static assets effectively.
Here’s a quick recap of the key points discussed:
Understanding Static Assets in Phoenix:
Compiling and Minifying Assets:
Leveraging Browser Caching:
Using a Content Delivery Network (CDN):
Image Optimization Techniques:
Serving Compressed Files:
Tips for Efficient Asset Management:
Monitoring Performance with Load Testing:
In summary, optimizing static assets involves multiple facets, from compilation and minification to effective caching, using CDNs, and continuous monitoring. Each step builds upon the last to create a robust, performant application.
By consistently applying these techniques and regularly revisiting your optimization strategies, you can ensure that your Phoenix application remains fast, efficient, and scalable. The landscape of web performance is always evolving, so staying informed on the latest practices and tools is essential.
Remember, the journey of optimization is continuous. Keep monitoring your application's performance, stay up-to-date with new optimization techniques, and utilize tools like LoadForge to ensure your application can handle the demands of your users.
Thank you for following this guide, and happy optimizing!