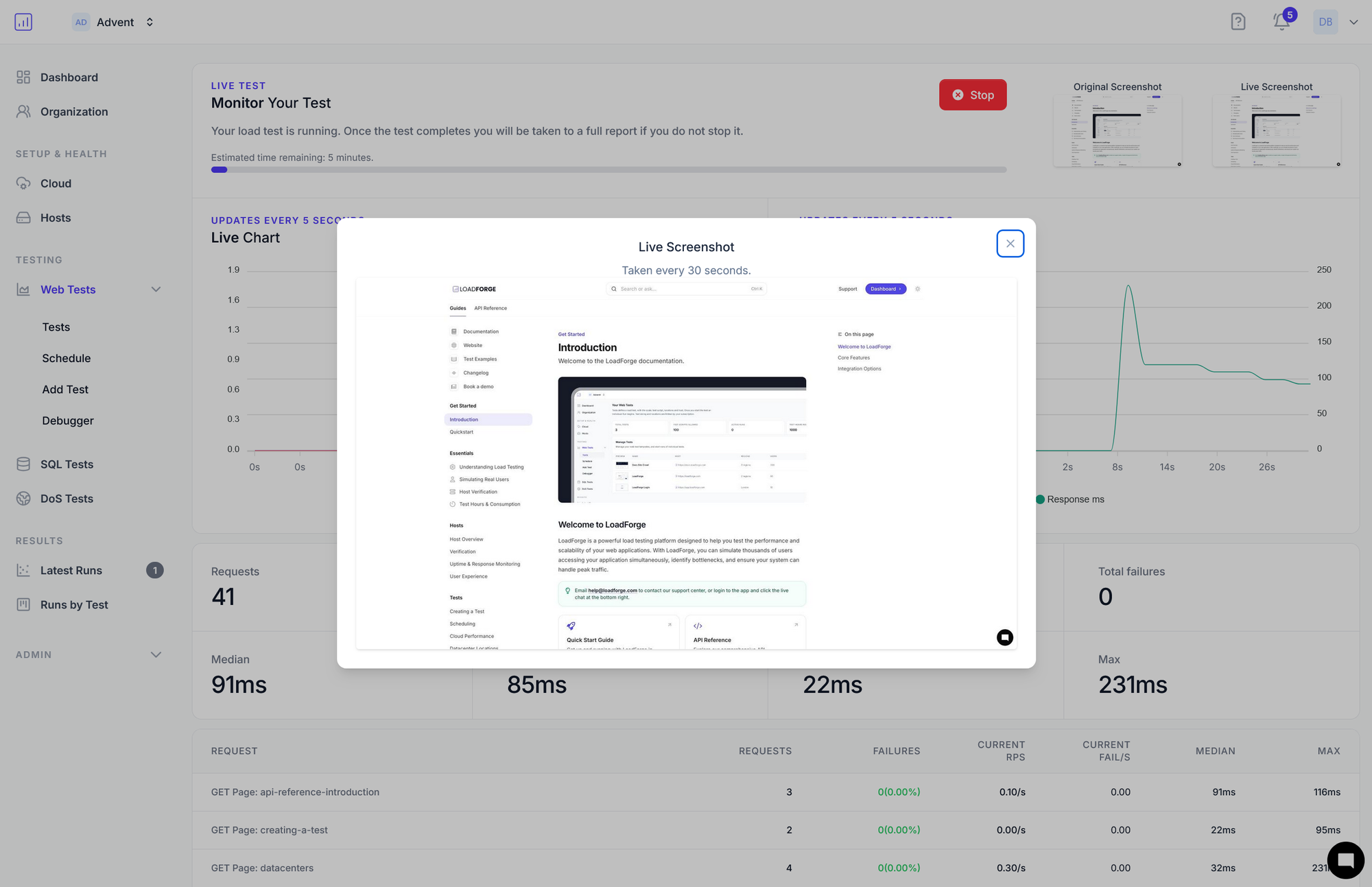
Faster Launches, Better Screenshots
Sometimes it's the small things that make the load testing experience better! We've rolled out an update that lets you...
In today's data-driven world, the efficiency of your database system directly influences your application's scalability and user experience. MongoDB, a widely adopted NoSQL database, offers unparalleled flexibility and scalability features, but optimizing its performance is essential to fully leverage these...
In today's data-driven world, the efficiency of your database system directly influences your application's scalability and user experience. MongoDB, a widely adopted NoSQL database, offers unparalleled flexibility and scalability features, but optimizing its performance is essential to fully leverage these advantages. This guide aims to provide a comprehensive roadmap for enhancing MongoDB performance through a series of strategic practices including indexing, caching, query tuning, and more.
MongoDB is designed to handle large volumes of data across a distributed architecture. However, improper configuration or inefficient querying can significantly degrade performance, hampering your application's ability to scale. By optimizing MongoDB, you ensure your database can handle growing volumes of data and user requests without the need for extensive hardware upgrades.
Nothing frustrates users more than slow or unresponsive applications. Latency in database operations can cascade into poor user experience, leading to higher bounce rates and loss of engagement. A finely-tuned MongoDB ensures quick data retrieval and seamless user interactions, directly contributing to user satisfaction and loyalty.
Operating a high-performance database isn't just about speed; it's also about cost. Efficient database operations require fewer resources, minimizing operational costs. By optimizing performance, you can maximize infrastructure utilization, potentially saving thousands of dollars in the long run.
This guide will walk you through various facets of MongoDB optimization, covering:
Performance optimization is not a one-time effort but an ongoing process. As your application evolves, so will the demands on your MongoDB setup. Through careful planning and systematic implementation of the techniques detailed in this guide, you'll be equipped to maintain a robust, high-performance database that scales effortlessly and delivers a stellar user experience.
Let's dive deeper into each optimization area, starting with an understanding of MongoDB's architecture.
MongoDB's architecture is designed to provide high scalability, flexibility, and performance. To fully utilize MongoDB’s potential, it’s crucial to grasp the core components that constitute its architecture. This section will break down the primary components: collections, documents, and replica sets.
In MongoDB, a collection is analogous to a table in traditional relational databases. It’s a grouping of MongoDB documents, stored within a single database, and serves as the basic unit of storage.
database.collection
, providing a clear namespace for organizing data.Documents are the fundamental units of data in MongoDB, similar to rows in relational databases but more dynamic and rich in structure.
{
"_id": ObjectId("507f191e810c19729de860ea"),
"name": "Alice",
"email": "[email protected]",
"age": 30,
"address": {
"street": "123 Elm St",
"city": "Metropolis",
"state": "NY"
},
"interests": ["reading", "hiking", "coding"]
}
Replica sets ensure data redundancy and high availability in MongoDB, which is essential for maintaining performance and reliability.
rs.initiate(
{
_id : "myReplicaSet",
members: [
{ _id: 0, host: "mongo1:27017" },
{ _id: 1, host: "mongo2:27017" },
{ _id: 2, host: "mongo3:27017" }
]
}
)
Understanding the architecture of MongoDB, particularly collections, documents, and replica sets, is key to optimizing its performance and ensuring scalability. These core components work together to provide a flexible, robust, and high-performing database solution. In the following sections, we will dive deeper into strategies and techniques that leverage this architecture for optimal performance, scalability, and reliability.
Effective indexing is crucial for optimizing MongoDB performance. Indexes support efficient query execution, which is essential for scalable applications. This section delves into how indexes work in MongoDB, the types of indexes available, and best practices for implementing effective indexing strategies.
Indexes in MongoDB function like the index in a book, allowing the database engine to locate and access data quickly without scanning every document. An index stores a portion of the collection data in an easy-to-traverse form, thus expediting query performance. Whenever a query references a field indexed by MongoDB, the database can leverage the index to swiftly pinpoint the corresponding documents.
MongoDB offers several types of indexes, each targeting specific use cases:
Single Field Indexes
db.collection.createIndex({ field: 1 }) // Ascending index
db.collection.createIndex({ field: -1 }) // Descending index
Compound Indexes
db.collection.createIndex({ firstField: 1, secondField: -1 })
Multikey Indexes
db.collection.createIndex({ arrayField: 1 })
Text Indexes
db.collection.createIndex({ field: "text" })
Geospatial Indexes
db.collection.createIndex({ location: "2dsphere" })
Hashed Indexes
db.collection.createIndex({ field: "hashed" })
To ensure your MongoDB indexes are effective, consider the following best practices:
Identify Critical Queries
Use Explain Plan
explain()
method to understand query plans and metrics.
db.collection.find({ field: value }).explain("executionStats")
Index Fields Used in Filters and Sorts
find
, sort
, group
, and aggregate
stages.
db.collection.createIndex({ searchField: 1, sortField: -1 })
Limit the Number of Indexes
Monitor Index Efficiency
Update Indexes as Data Evolves
Consider Compound and Multikey Indexes
Leverage Partial Indexes
db.collection.createIndex({ field: 1 }, { partialFilterExpression: { status: { $eq: "active" } } })
By leveraging the appropriate indexing strategies, you can drastically improve query performance and ensure your MongoDB database scales effectively. Remember that the effectiveness of your indexes should be assessed continuously, fine-tuning them as data evolves and query patterns shift.
Writing optimized MongoDB queries is crucial for minimizing execution time and maximizing efficiency. Poorly written queries can significantly impact performance, leading to slower response times and degraded user experience. In this section, we'll cover guidelines and tips for crafting efficient queries that take full advantage of MongoDB's capabilities.
One of the most effective ways to enhance query performance is by leveraging indexes. Ensure that your queries are supported by appropriate indexes to minimize the amount of data MongoDB needs to scan.
db.users.createIndex({ "email": 1 })
This index on the email
field can tremendously speed up queries searching for users by email.
Fetching only the fields you need can conserve memory and improve performance. This is known as using projections in MongoDB.
db.users.find({ "isActive": true }, { "name": 1, "email": 1 })
This query returns only the name
and email
fields from the documents where isActive
is true
.
Covered queries are those in which all the fields in the query are part of an index, and the fields returned in the results are in the same index. These queries are much faster as they do not require fetching the document itself.
If your query looks like this:
db.users.find({ "email": "[email protected]" }, { "email": 1, "_id": 0 })
And you have an index on the email
field:
db.users.createIndex({ "email": 1 })
MongoDB can fulfill the query entirely using the index, making it very efficient.
Minimize the amount of data your queries need to scan by using selective queries and indexes.
db.orders.find({ "creationDate": { "$gte": ISODate("2022-01-01") } }).sort({ "creationDate": -1 }).limit(10)
Here, an index on creationDate
would help quickly locate the relevant documents.
Aggregation pipelines can be powerful but can also be resource-intensive. Always ensure they are designed for efficiency.
db.orders.aggregate([
{ "$match": { "status": "completed" } },
{ "$group": { "_id": "$customerId", "totalSpent": { "$sum": "$amount" } } },
{ "$sort": { "totalSpent": -1 } }
])
Using match
stages early in the pipeline can limit the data processed by subsequent stages, making the operation more efficient.
While limit
and skip
can help manage query results, they should be used cautiously in large collections.
db.users.find().sort({ "createdAt": -1 }).skip(100).limit(50)
Ensure that the sort
field has an appropriate index to avoid performance bottlenecks.
MongoDB provides the explain()
method to analyze query performance. Use this tool to understand how queries are executed and identify bottlenecks.
db.users.find({ "isActive": true }).explain("executionStats")
Review the execution stats to determine if your query is efficiently using indexes and resources.
Following these guidelines will help you write more efficient MongoDB queries. Proper indexing, limiting fields with projection, using covered queries, cautious aggregation pipeline usage, mindful limit and skip operations, and leveraging tools like explain()
will collectively enhance query performance, ensuring a responsive and scalable MongoDB database.
Effective analysis of query performance is pivotal in optimizing MongoDB operations. MongoDB offers a suite of built-in tools like explain()
and profiling that provide deep insights into how queries are executed and where improvements can be made. In this section, we will explore these tools and learn how to use them to achieve better query performance.
explain()
The explain()
method is a powerful tool in MongoDB that provides details about how a query is executed. It helps identify inefficiencies by breaking down the steps involved in query execution.
Here's how you can use the explain()
method:
db.collection.find({ field: "value" }).explain("executionStats")
The above command provides detailed statistics about the query execution, including:
db.users.find({ age: { $gt: 25 } }).explain("executionStats")
Output snippet:
{
"executionStats": {
"executionTimeMillis": 5,
"totalKeysExamined": 1000,
"totalDocsExamined": 1000,
"executionStages": {
"stage": "COLLSCAN",
"nReturned": 100,
"totalDocsExamined": 1000,
"inputStage": {
"stage": "EOF"
}
}
}
}
In this example, the COLLSCAN
stage indicates a collection scan, which is less efficient than an indexed search. This indicates a need for an appropriate index.
MongoDB’s profiler is a built-in tool that collects data about query performance across the database. It allows you to see slow-performing queries and provides insights into how to optimize them.
To enable profiling, use the following command:
db.setProfilingLevel(1)
This command activates profiling for slow operations. You can set it to level 2
to capture all operations (use this sparingly as it can be resource-intensive).
Once profiling is enabled, you can access collected performance data using:
db.system.profile.find().sort({ ts: -1 }).limit(10).pretty()
This returns the 10 most recent profiled operations, including query execution times and resource utilization.
explain()
and ProfilingRun explain()
on slow-performing queries indicated by the profiler to understand execution details and identify bottlenecks. For instance, if a query is slow due to a collection scan, you might need to create or adjust indexes.
Frequently monitor index usage via explain()
to ensure optimal index performance. Profiling can reveal if new indexes need to be created or existing ones updated to handle query loads effectively.
Both explain()
and profiling data can suggest changes in query structure or conditions to make them more performant. For example, adjusting query filters based on indexed fields can significantly reduce execution times.
Analyzing query performance with MongoDB’s explain()
and profiler tools is crucial for maintaining efficient database operations. Regularly use these tools to identify and resolve performance bottlenecks. Employing these practices along with other optimization strategies like indexing, schema design, and caching will ensure a scalable and high-performing MongoDB environment.
Next, we will delve into caching techniques to further reduce database load and enhance performance.
Caching is an essential strategy for optimizing MongoDB performance. It helps alleviate the load on the database by storing frequent query results or often-used data in faster storage solutions, enabling quick data retrieval. This section will explore various caching techniques, including in-memory data stores and query result caching, to enhance MongoDB performance.
In-memory data stores like Redis or Memcached are widely used to cache data temporarily for high-speed access. These stores can offload read-heavy operations from MongoDB, resulting in faster query performance and reduced database load.
Redis is a popular choice for in-memory data caching due to its efficiency and ease of use. Here's a basic example of how to integrate Redis caching with your MongoDB queries:
Install Dependencies: Ensure you have the necessary Redis dependencies installed in your project.
npm install redis mongodb
Setup Redis and MongoDB: Establish connections to both Redis and MongoDB.
const redis = require('redis');
const { MongoClient } = require('mongodb');
const redisClient = redis.createClient();
const mongoClient = new MongoClient('mongodb://localhost:27017');
Implement Caching Logic: When querying MongoDB, first check Redis for cached results. If no cached result is found, query MongoDB and store the result in Redis for future use.
async function getUserData(userId) {
const cacheKey = `user:${userId}`;
const cachedData = await redisClient.get(cacheKey);
if (cachedData) {
return JSON.parse(cachedData);
}
const db = mongoClient.db('mydatabase');
const user = await db.collection('users').findOne({ _id: userId });
if (user) {
redisClient.set(cacheKey, JSON.stringify(user), 'EX', 3600); // Cache for 1 hour
}
return user;
}
Query result caching involves storing the results of frequent and expensive database queries to quickly serve future requests. This can be particularly beneficial for read-heavy applications.
Identify Frequent Queries: Use MongoDB’s profiling tools to identify queries that are frequently executed and are resource-intensive.
Implement a Cache Layer: Cache the results of these identified queries. Here's an example using Node.js:
async function getCachedQueryResults(query) {
const cacheKey = `query:${JSON.stringify(query)}`;
const cachedResult = await redisClient.get(cacheKey);
if (cachedResult) {
return JSON.parse(cachedResult);
}
const db = mongoClient.db('mydatabase');
const result = await db.collection('collectionName').find(query).toArray();
redisClient.set(cacheKey, JSON.stringify(result), 'EX', 600); // Cache for 10 minutes
return result;
}
Caching is a powerful technique for optimizing MongoDB performance. By strategically using in-memory data stores like Redis and query result caching, you can achieve reduced latency, decreased database load, and cost-effective scalability. Applying these caching techniques will significantly enhance the responsiveness and performance of your MongoDB-backed application.
A well-designed schema is crucial for optimizing MongoDB performance, maintainability, and scalability. Unlike traditional relational databases, MongoDB offers flexible schema design, which can lead to significant performance gains if utilized correctly. Below are best practices and guidelines to help design efficient and scalable MongoDB schemas.
Before diving into the schema design, it’s essential to understand the specific use cases and access patterns of your application. Consider the following questions:
Understanding these factors will help you tailor your schema to optimize performance for your particular workload.
MongoDB supports embedding documents within other documents, which is particularly useful for storing related data together. This approach is advantageous for use cases where:
For example, embedding an address document within a user document:
{
"user_id": "123",
"name": "John Doe",
"address": {
"street": "123 Main St",
"city": "Springfield",
"state": "IL",
"zip": "62701"
}
}
Pros:
Cons:
In some scenarios, embedding can lead to excessively large documents or unnecessary data duplication. Instead, you can reference documents:
{
"user_id": "123",
"name": "John Doe",
"address_id": "abc"
}
Pros:
Cons:
In many cases, a hybrid approach combining both embedding and referencing delivers the best performance. For example, embed frequently accessed data and reference infrequently accessed data.
Adopt consistent naming conventions for fields to ensure clarity and maintainability:
"userId"
, "firstName"
, "createdAt"
.Schema design and indexing go hand-in-hand. Define indexes based on query patterns to improve read performance. Consider compound indexes for queries involving multiple fields.
While MongoDB supports arrays, large arrays can lead to performance issues. Optimize array usage by limiting their size or breaking them into smaller documents if necessary.
For use cases like logging where data is written frequently and read less often, consider using capped collections. These collections maintain insertion order and automatically delete the oldest entries once a size limit is reached.
db.createCollection("log", {
capped: true,
size: 5242880, // 5MB
max: 5000 // optional, limit to 5000 documents
});
Design your schema to accommodate future growth without necessitating significant changes. Factor in potential increases in data volume and adjust your schema design accordingly.
Leverage MongoDB’s support for atomic operations on single documents to maintain data integrity and consistency. For example, use operators like $inc
, $set
, and $push
to update documents atomically.
A practical example illustrating an optimized schema might involve users and their orders:
db.users.insertOne({
"user_id": "123",
"name": "John Doe",
"email": "[email protected]",
"orders": [
{
"order_id": "001",
"amount": 199.99,
"items": [
{ "product_id": "A100", "qty": 2, "price": 50.00 },
{ "product_id": "B200", "qty": 1, "price": 99.99 }
],
"order_date": ISODate("2023-10-01T14:12:00Z")
},
{
"order_id": "002",
"amount": 149.99,
"items": [
{ "product_id": "C300", "qty": 1, "price": 149.99 }
],
"order_date": ISODate("2023-10-10T11:24:00Z")
}
]
});
In this schema:
Effective schema design is a foundational aspect of optimizing MongoDB performance. By understanding your use cases, choosing the appropriate data model, and adhering to best practices, you can create schemas that enhance performance, maintainability, and scalability. Remember, schema design is an iterative process; continually revisit and refine your schema as your application evolves and your understanding of your data grows.
## Sharding for Scalability
One of MongoDB's most powerful features for handling large datasets and scaling horizontally is sharding. Sharding involves partitioning your data across multiple servers, or shards, allowing MongoDB to support deployments with very large data sets, high throughput operations, or both.
### What is Sharding?
Sharding is the process of distributing data across multiple machines to support deployments with large data sets and high throughput operations. MongoDB uses a shard key — a value within documents in a collection — to distribute the documents among shards. By distributing data across various shards, MongoDB can horizontally scale and accommodate the demands of growing applications.
### How Sharding Works in MongoDB
MongoDB’s approach to sharding involves several critical components:
- **Shard:** Each shard is an independent database, holding a subset of the data. Together, these shards make up the entire data set.
- **Config Server:** Config servers store metadata and configuration settings for the cluster. This metadata includes a mapping of which data is held within which shard.
- **Query Router (mongos):** These are the instances that user applications interact with. A query router routes the client requests to the appropriate shard(s) depending on the operation and the distribution of the data.
### Implementing Sharding
Let's dive into how to effectively implement sharding in MongoDB:
#### 1. Choosing a Shard Key
The choice of a shard key is critical for effective distribution of data. A good shard key should offer:
- **Even Distribution:** Ensure data is evenly distributed across shards to avoid hotspots.
- **High Cardinality:** Choose a key with a wide range of possible values to ensure effective distribution.
- **Unchanging:** Avoid shard keys that will change frequently as it can impact distribution consistency.
#### Example
Here is an example of enabling sharding for a collection:
<pre><code>
// Enable sharding on the database
sh.enableSharding("myDatabase")
// Shard the collection on a specified shard key
sh.shardCollection("myDatabase.myCollection", { "userId": 1 })
</code></pre>
#### 2. Shard Key Considerations
- **Compound Shard Keys:** Sometimes single keys may not be sufficient. MongoDB allows compound shard keys, which can be advantageous for distributing data based on multiple fields.
<pre><code>
// Shard the collection using a compound shard key
sh.shardCollection("myDatabase.myCollection", { "userId": 1, "orderId": 1 })
</code></pre>
- **Hashed Shard Keys:** This method hashes the value of the shard key to provide a more even distribution of data if sequential insertion is a concern.
<pre><code>
// Shard the collection using a hashed key
sh.shardCollection("myDatabase.myCollection", { "userId": "hashed" })
</code></pre>
### Strategies for Effective Sharding
To ensure the effectiveness of sharding, consider the following strategies:
- **Monitor Balancer:** The balancer process distributes the data evenly across shards. Ensure it is functioning correctly and adjust its settings if necessary.
- **Pre-Splitting:** For collections anticipated to grow significantly, pre-splitting can help distribute initial load evenly across shards.
- **Index Considerations:** Ensure all queries using the shard key are indexed to avoid scatter-gather queries that hit all shards.
### Best Practices for Sharding
- **Plan Ahead:** Anticipate future growth and sharding requirements from the beginning. Retrofitting sharding can be complex and disruptive.
- **Distribution Uniformity:** Regularly monitor shard distribution and adjust shard key strategies if you notice imbalances.
- **Maintenance Windows:** Execute shard-related migrations and balancing during low-traffic periods to minimize user impact.
### Conclusion
Sharding is a powerhouse for achieving horizontal scalability in MongoDB. By correctly implementing and managing sharding strategies, you can ensure your MongoDB deployment can handle significant growth and large-scale data operations. Monitoring and continuously optimizing your sharding setup is essential for maintaining performance and efficiency as your data grows.
## Monitoring and Performance Tuning
Ensuring optimal MongoDB performance is not a one-time task; it requires continuous monitoring and adjustments as your application evolves. In this section, we will delve into the methods and tools available for monitoring MongoDB performance, along with actionable techniques for performance tuning.
### Monitoring Tools
#### MongoDB Monitoring Service (MMS)
MongoDB provides a dedicated monitoring service called MongoDB Monitoring Service (MMS), which offers comprehensive insights into database performance. MMS collects and visualizes data on various performance metrics such as operation counts, replication statuses, and hardware utilization.
#### Built-in Monitoring Commands
MongoDB also includes built-in monitoring commands that deliver real-time performance data. Some of the essential commands are:
- `db.serverStatus()`: Provides an overview of the database status, including metrics on memory usage, connections, and indexes.
```bash
db.serverStatus()
db.stats()
: Offers statistics on a particular database, such as data size, index size, and collection counts.
db.stats()
db.collection.stats()
: Returns detailed statistics about a collection, including document count, average document size, and storage size.
db.collection.stats()
db.currentOp()
: Displays currently running operations. Helpful for identifying long-running queries.
db.currentOp()
MongoDB’s built-in profiling capabilities allow you to log and analyze operations that take more than a specified amount of time. The two main profiling tools are:
Query Profiler: The query profiler logs all database operations that exceed a given threshold. You can set the profiling level to capture slow queries or all operations.
// Enable profiling at level 1 (low), capturing operations longer than 100ms
db.setProfilingLevel(1, 100)
Explain Plan: The explain()
method provides verbose information about how MongoDB executes a query. This includes details like index usage and execution time.
db.collection.find({ field: value }).explain("executionStats")
When monitoring MongoDB performance, certain key metrics should be examined regularly. These include:
Revisit your indexing strategy to ensure it aligns with the current query patterns. Use the output of explain()
to verify index usage and make necessary adjustments.
Refine your queries by:
Optimize your hardware resources by:
MongoDB connection pooling configurations can have a significant impact on performance. Ensure your connection pool size is tuned according to your application’s requirements.
Review your sharding strategy to ensure data is evenly distributed and queries are effectively routed. When using replica sets, ensure that secondary nodes are capable of handling query loads to offload reads from the primary node.
Persistent monitoring coupled with proactive tuning is indispensable for maintaining MongoDB performance. By routinely analyzing key metrics, leveraging built-in tools, and adapting to changing workloads, you can achieve a robust, scalable MongoDB deployment that meets the demands of your application.
## Load Testing with LoadForge
In order to ensure your MongoDB setup can handle the anticipated load and traffic, load testing is an essential step. LoadForge is a powerful tool designed for this purpose. It simulates heavy load on your database to uncover performance bottlenecks, measure the impact of optimizations, and ensure your system remains robust under stress. This section outlines how to use LoadForge for load testing your MongoDB setup effectively.
### Setting Up LoadForge for MongoDB
1. **Account Setup**: Begin by creating an account on the LoadForge platform if you haven't done so already.
2. **Project Creation**: Navigate to your dashboard and create a new project. You can name it something relevant to your MongoDB load test, such as `MongoDB Performance Test`.
3. **Script Creation**: LoadForge allows you to create load-testing scripts using multiple protocols. For MongoDB, you can use the HTTP protocol if you are testing a web application that interacts with MongoDB, or directly use LoadForge's capabilities to run custom scripts that simulate database operations.
### Writing Load Test Scripts
LoadForge supports various scripting languages and tools. Here’s an example of a simple HTTP-based load test script simulating load on a web application backed by MongoDB:
```javascript
const { scenario, step, check } = require('k6');
export default scenario('Load MongoDB via WebApp')
.exec(http.get, 'https://yourwebapp.example.com/api/data')
.after(response => {
check(response, {
'status is 200': r => r.status === 200,
'response time < 200ms': r => r.timings.duration < 200,
});
});
Test Configuration: Configure your test settings in LoadForge by specifying the number of virtual users (VUs), the duration of the test, and the ramp-up/down times.
Starting the Test: Once configured, start the load test from the LoadForge dashboard. Monitor real-time metrics displayed on the dashboard, such as request rates, average response times, and error rates.
After completing the load test, LoadForge provides detailed reports and visual insights into various performance metrics that are crucial for performance tuning:
Throughput and Latency:
Error Rates:
Resource Utilization:
Based on the insights gathered from LoadForge's load testing, you can take specific actions to improve MongoDB performance:
By routinely running load tests using LoadForge, you can ensure your MongoDB setup remains robust and performs optimally as your application scales. Regular load testing helps identify potential performance issues before they impact your users, ensuring a smooth and responsive experience.
This concludes our section on load testing with LoadForge. Consistent load testing coupled with ongoing performance monitoring and optimization is critical for maintaining an efficient and scalable MongoDB setup.
In the journey towards optimizing MongoDB performance, we have traversed a range of critical strategies and best practices. Implementing these can significantly enhance the scalability, responsiveness, and efficiency of your MongoDB deployments. Here, we encapsulate the key takeaways and underscore the vitality of continuous optimization and monitoring.
Understanding MongoDB Architecture:
Indexing Strategies:
Efficient Query Execution:
Query Performance Analysis:
explain()
and profiling, to analyze and improve query performance.Caching Techniques:
Schema Design:
Sharding for Scalability:
Monitoring and Performance Tuning:
Load Testing with LoadForge:
All these strategies work best when integrated cohesively. Indexing, efficient querying, and schema design are interlinked, with each influencing the others. Likewise, caching and sharding need to be considered within the broader context of your application's architecture and data access patterns.
MongoDB performance optimization is not a one-time effort but an ongoing process. Database workloads, query patterns, and usage scenarios evolve, necessitating regular reassessments and adjustments. Continuous monitoring provides the feedback loop required to identify emerging performance issues and address them proactively.
By embracing a holistic approach to MongoDB optimization and committing to continuous improvement, you can ensure that your database infrastructure scales elegantly, remains responsive, and provides an excellent user experience.
In closing, the principles and practices discussed here are foundational to crafting a resilient, high-performance MongoDB environment. Keep learning, stay vigilant, and leverage the wealth of tools and strategies at your disposal to maintain optimal database performance.