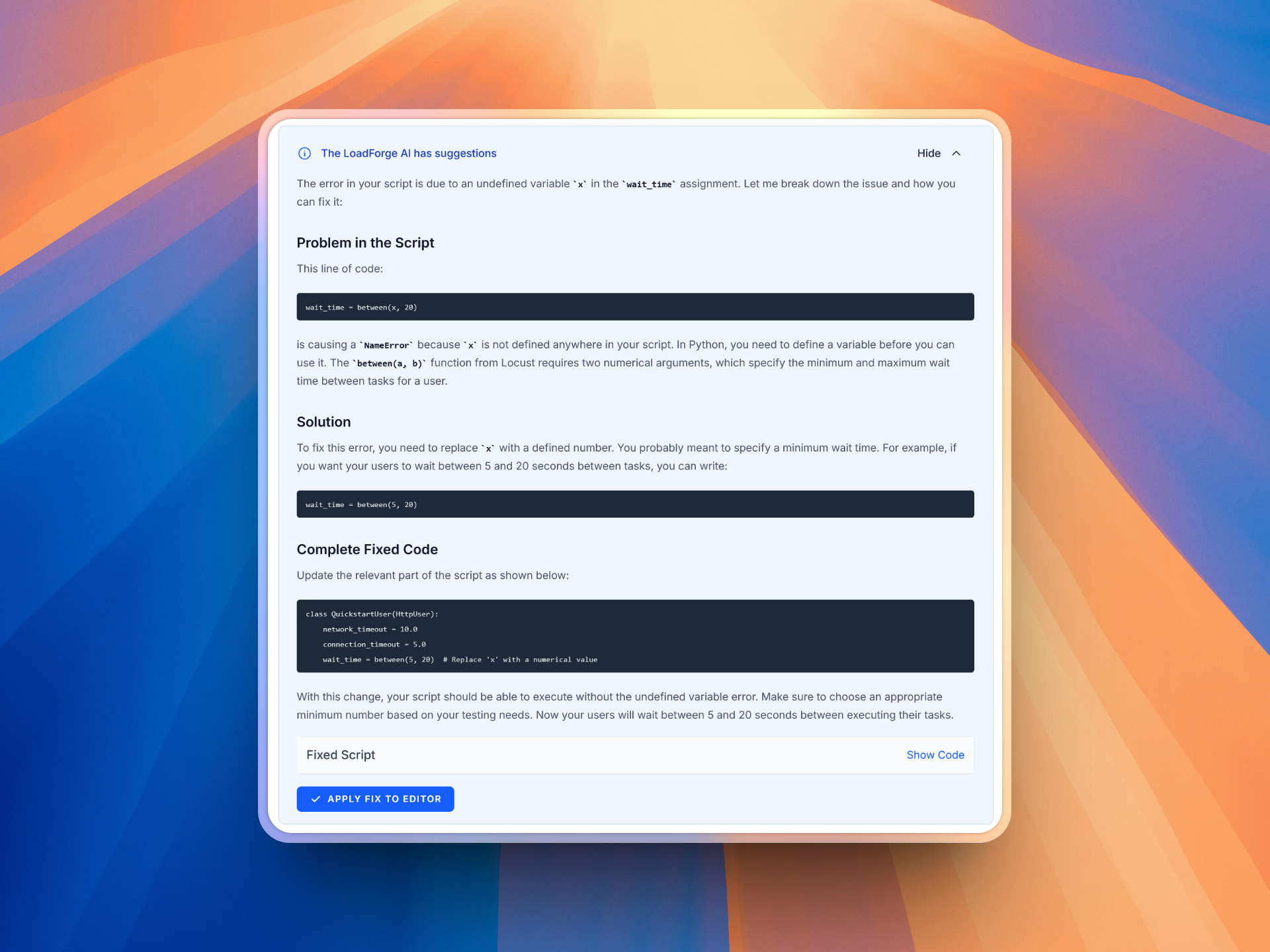
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
In the realm of web development, securing an application is as crucial as its functionality. Laravel, a prominent PHP framework known for its elegant syntax and robust feature set, also brings a strong emphasis on security. This section provides an...
In the realm of web development, securing an application is as crucial as its functionality. Laravel, a prominent PHP framework known for its elegant syntax and robust feature set, also brings a strong emphasis on security. This section provides an overview of common security concerns in web development and discusses how Laravel equips developers to address these challenges effectively.
Before diving into Laravel-specific features, it's essential to understand the common security pitfalls in web development:
Laravel is designed with security in mind, providing several built-in functionalities that help safeguard applications:
CSRF Protection: Laravel automatically generates and verifies CSRF tokens for each active user session, providing a straightforward way to secure forms against CSRF attacks.
<form method="POST" action="/profile">
@csrf
<!-- Form Contents -->
</form>
XSS Protection: Laravel provides easy-to-use Blade templating engine which escapes all output by default. This prevents malicious script injections when displaying user inputted data.
{{ $userInput }}
SQL Injection Protection: Eloquent, Laravel’s ORM, uses PDO parameter binding, thus preventing SQL injection.
User::where('email', $email)->first();
Password Hashing and Reset: Laravel uses bcrypt for password hashing, ensuring that passwords are not stored as plain text. It also offers a simple way to handle password resets securely.
Authentication and Authorization: Laravel has an authentication system built right in, which includes out-of-the-box configurations for user registration, password reset, and more. Policies and Gates provide an elegant way to define and enforce authorization.
Encrypted Storage: Laravel's encrypted storage solutions ensure that sensitive data is kept secure using the AES-256 encryption standard.
use Illuminate\Support\Facades\Crypt;
$encrypted = Crypt::encryptString('Hello world');
$decrypted = Crypt::decryptString($encrypted);
While Laravel provides many security features, adhering to security best practices is imperative:
By leveraging Laravel’s features and adhering to these practices, developers can significantly mitigate the risks associated with web application security. In the following sections, we will further explore specifics such as authentication, data validation, API security, encryption, and more, providing a comprehensive guide on maintaining a secure Laravel application.
Laravel is well-regarded for its comprehensive and flexible security infrastructure, which is crucial for building robust web applications. The framework offers robust mechanisms for user authentication and authorization, providing easy-to-implement options that are both secure and adaptable to various business requirements.
Laravel makes implementing authentication very simple. Out of the box, it provides a streamlined and secure system for handling user authentication with minimal setup. A significant part of Laravel's authentication system is built on top of the guard
and providers
configurations, which are defined in the config/auth.php
file.
The auth.php
configuration file enables you to specify which guard and user provider Laravel should utilize when performing authentication. Laravel supports various types of guards like sessions and tokens, allowing for stateful and stateless authentication in web and API environments respectively. Here is a basic example of setting a guard:
'guards' => [
'web' => [
'driver' => 'session',
'provider' => 'users',
],
'api' => [
'driver' => 'token',
'provider' => 'users',
'hash' => false,
],
],
The guard configuration directs Laravel on how to store and retrieve information regarding authenticated users.
For enhancing security, Laravel facilitates options like two-factor authentication, secure password hashing, and session encryption. Laravel utilizes the bcrypt
hashing algorithm by default to ensure your user passwords are securely stored. Here is how you might hash a password:
use Illuminate\Support\Facades\Hash;
$password = Hash::make('your-plain-password');
To safeguard the application further, you should consider enabling HTTPS in your web server configuration to protect sensitive data transmissions.
Managing user rights and permissions is a critical aspect of securing an application. Laravel's built-in authorization system, known as "Gates" and "Policies," allows developers to define clear logic for what users can and cannot do within an application. Here's a quick example of defining a gate:
use Illuminate\Support\Facades\Gate;
Gate::define('edit-post', function ($user, $post) {
return $user->id === $post->user_id;
});
This gate ensures that a post can only be edited by its creator.
Properly managing sessions is vital for maintaining application security. Laravel provides several features out-of-the-box to help manage sessions securely:
secure
and http_only
help prevent unauthorized access to cookies.You configure session settings in the config/session.php
file. Here is an example to ensure sessions are only carried over HTTPS:
'secure' => true,
'http_only' => true,
By leveraging Laravel’s robust authentication and authorization capabilities, developers can ensure that their applications are secure out of the box. Moreover, fine-tuning these capabilities with advanced security features and proper session management helps in building a secure and trustworthy application environment. Given these tools, maintaining vigilance through regular updates and employing robust tools for load testing, like LoadForge, are critical practices to ensure your application remains secure against evolving threats.
In web development, securing the integrity of your data involves robust input validation and data sanitization strategies. Laravel provides powerful and flexible tools to help you prevent common security risks like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). This section explores practical techniques for implementing these protections in your Laravel applications.
Laravel’s validation features are integral in ensuring that only properly formed data makes its way into your application. By using Laravel's validation rules, you can easily enforce formats, types, and specific values. Here’s how you can use these features in your projects:
One of the most powerful features of Laravel is the form request class, which encapsulates validation logic. To create a custom validation request, you can use the Artisan CLI:
php artisan make:request StorePostRequest
In the generated StorePostRequest
class, you can define your validation rules like so:
public function rules()
{
return [
'title' => 'required|max:255',
'body' => 'required',
'publish_at' => 'nullable|date',
];
}
By type-hinting this request in your controller method, Laravel automatically applies these rules before your controller logic runs, ensuring that only valid data is processed:
public function store(StorePostRequest $request)
{
// Your data is valid...
}
While validation ensures data adheres to expected rules, sanitization cleanses the data, removing potentially harmful elements like scripts or SQL fragments. Laravel does not provide explicit sanitization functions because it handles many aspects of sanitization internally, especially with regard to XSS prevention. For example, Blade templating engine escapes all output by default:
{{ $input }} // Automatically escaped
For manual data cleansing, especially for inputs that might be used in URLs or JavaScript contexts, you can utilize PHP's native functions like htmlspecialchars
or strip_tags
.
Laravel's Eloquent ORM uses prepared statements which inherently protect against SQL injection. However, if you must write raw SQL queries, ensure they are properly parameterized:
$results = DB::select('SELECT * FROM users WHERE email = ?', [$email]);
To further mitigate XSS risks in Laravel:
{{ }}
syntax in Blade files to ensure data is escaped.{!! !!}
syntax with explicit sanitation.Laravel automatically generates and verifies CSRF tokens for all POST forms and AJAX requests when using the web middleware group. Ensure all forms include the CSRF token:
<form method="POST" action="/profile">
@csrf
<!-- form body -->
</form>
For AJAX requests, Laravel's Javascript scaffold will automatically add the CSRF token to all outgoing requests. Alternatively, you can retrieve the token and add it manually:
headers: {
'X-CSRF-TOKEN': document.querySelector('meta[name="csrf-token"]').getAttribute('content')
}
Implementing thorough input validation and data sanitization protocols is essential for maintaining the security and integrity of your applications. By leveraging Laravel’s built-in functionalities, developers can significantly reduce the risk of common web vulnerabilities and safeguard their applications against potential attacks.
Securing API endpoints is crucial in safeguarding your Laravel applications against unauthorized access and potential threats. Laravel provides several built-in features to enhance the security of your APIs, including rate limiting, authentication tokens, Cross-Origin Resource Sharing (CORS) configurations, and the use of Laravel Sanctum for API token management. This section will guide you through implementing these tools to secure your Laravel APIs effectively.
One of the foundational steps in securing an API is to ensure that all requests are authenticated. Laravel Sanctum provides a simple and robust way to handle API authentication using token-based systems. Here's how you can set up Sanctum to manage API tokens:
Install Sanctum: Start by installing Laravel Sanctum via Composer:
composer require laravel/sanctum
Publish the Sanctum configuration file:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
Migration: Run the Sanctum migrations to create the necessary tables:
php artisan migrate
Set Up Middleware:
Ensure that your api
middleware group within app/Http/Kernel.php
includes Sanctum's middleware:
'api' => [
\Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class,
'throttle:api',
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
Issuing API Tokens: You can issue API tokens to users, which will be used to make authenticated requests to your API. Here's a basic example:
use App\Models\User;
Route::get('/api/token', function (Request $request) {
$user = User::find($request->user_id);
return $user->createToken('YourAppName')->plainTextToken;
});
Rate limiting is essential to protect your API from overuse and abuse, such as denial-of-service (DoS) attacks. Laravel makes it easy to implement rate limiting using its built-in functionality. You can configure rate limits in app/Http/Kernel.php
:
'api' => [
'throttle:60,1',
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
This configuration will limit requests to 60 per minute per route from a single IP.
Handling Cross-Origin Resource Sharing (CORS) is vital for API security, especially if you're serving your API to different domains. Laravel includes support for CORS, which you can configure by updating the CORS configuration file located in config/cors.php
. Here you can specify which domains, HTTP methods, and headers are allowed:
return [
'paths' => ['api/*'],
'allowed_methods' => ['*'],
'allowed_origins' => ['https://example.com'],
'allowed_origins_patterns' => [],
'allowed_headers' => ['*'],
'exposed_headers' => [],
'max_age' => 0,
'supports_credentials' => false,
];
After securing your APIs with the methods described above, it's critical to test their resilience against high traffic and malicious attacks. LoadForge offers powerful load testing and security stress testing tools designed for Laravel applications. Using LoadForge, you can simulate a variety of scenarios to ensure your API remains reliable and secure under any condition.
Implementing these security measures will significantly enhance the protection of your Laravel API endpoints. Regular updates and audits, along with rigorous testing using tools like LoadForge, will help maintain a robust security posture for your applications.
In the realm of web application security, the proper use of encryption and hashing cannot be overstated. Laravel, being a framework that emphasizes security, provides robust features for both encryption and hashing, enabling developers to protect sensitive data effectively. This section will guide you through the essential techniques of configuring and utilizing Laravel’s encryption and hashing capabilities to fortify your application's security.
Laravel uses OpenSSL to provide a robust, secure encryption of data. The framework offers an easy-to-use, yet powerful interface for encrypting and decrypting data via its Crypt
facade. Before diving into encrypting data, it's pivotal to set the application key, which Laravel uses as the encryption key. This key is stored in your .env
file as APP_KEY
and should be set using the php artisan key:generate
command, which ensures that your key is adequately secure.
To utilize Laravel's encryption, no further configuration is needed beyond setting the APP_KEY
. Laravel automatically handles the configuration, choosing the appropriate cipher. For most applications, the default AES-256-CBC cipher is suitable and secure. Ensure that you never store sensitive data in your application without encryption.
use Illuminate\Support\Facades\Crypt;
$encrypted = Crypt::encryptString('Sensitive Data');
$decrypted = Crypt::decryptString($encrypted);
echo $decrypted; // Outputs 'Sensitive Data'
Hashing is used extensively for storing data that should not be reverse-engineered, such as passwords. Laravel utilizes the Hash
facade, which provides secure Bcrypt and Argon2 hashing for storing user passwords securely. The framework’s built-in user authentication model automatically uses these secure hashing techniques.
While Bcrypt is used by default, Laravel also supports Argon2, which is considered more memory-resistant. You can configure the hashing driver in your config/hashing.php
configuration file.
use Illuminate\Support\Facades\Hash;
$password = 'secret';
$hashedPassword = Hash::make($password);
if (Hash::check('secret', $hashedPassword)) {
echo 'Password is valid!';
}
APP_KEY
.By properly configuring and employing Laravel's built-in encryption and hashing features, developers can significantly enhance the security level of their applications. Always keep your application’s dependencies updated and be vigilant of new security practices to protect data effectively.
When building web applications with Laravel, ensuring the security of file uploads and the server environment is crucial. This section explores best practices for handling file permissions and securing file uploads. Additionally, it touches on essential server-side security considerations to prevent unauthorized access and data breaches.
File uploads are a common feature in many web applications but can introduce significant security risks if not handled properly. Below are key strategies to secure file uploads in Laravel:
Validate File Types: Always validate the MIME types of uploaded files to ensure only allowed types are processed. You can use Laravel’s validation rules to restrict file types.
$request->validate([
'document' => 'required|file|mimes:pdf,docx,txt'
]);
Limit File Sizes: Restrict the size of the files that users can upload to avoid performance degradation and potential denial-of-service (DoS) attacks.
$request->validate([
'image' => 'required|file|max:2048'
]);
Storage Path Separation: Never store uploaded files in a directory accessible directly from the web. Instead, use Laravel’s built-in storage system configured outside the web root.
$path = $request->file('avatar')->store('avatars');
Rename Uploaded Files: Automatically rename files upon upload to avoid any potentially malicious file names and mitigate the risk of executing harmful scripts.
$filename = md5(time()) . '.' . $file->getClientOriginalExtension();
$path = $file->storeAs('uploads', $filename);
Use Antivirus Scanning: Implement server-side antivirus scanning of uploaded files, if possible, especially if users can upload executable or binary data.
Proper file permissions are fundamental to securing your Laravel application. Here’s what you need to consider:
Securing the server environment is as important as application-level security. Below are some Laravel-specific and general server security practices:
Use Environment Variables: Store sensitive configuration options like database credentials and API keys in environment files (.env).
DB_PASSWORD=supersecretpassword
HTTPS Everywhere: Ensure that all traffic is transmitted over HTTPS, using SSL/TLS, to protect data in transit. Configure HSTS (HTTP Strict Transport Security) to force HTTPS connections.
Limit Database Access: The database should only be accessible from specific, required services and not exposed directly to the internet.
Update Regularly: Keep your Laravel application and server dependencies up-to-date to ensure all known vulnerabilities are patched.
Use Security Headers: Implement security headers like X-Frame-Options, X-Content-Type-Options, and Content-Security-Policy to enhance security.
Monitor & Audit: Regularly monitor the server and application logs. Implement auditing and alerting for any suspicious activity.
Finally, ensure to regularly test the security of your Laravel application and the server:
By implementing these file, server security practices, and regular testing, you enhance the overall security posture of your Laravel application, aligning with industry standards and best practices.
Ongoing vigilance is vital in the maintenance of any web application to ensure it adapts to the evolving landscape of cybersecurity threats. For developers using Laravel, integrating proactive security testing and regular maintenance protocols into the development lifecycle is not just beneficial; it's necessary. This section outlines effective strategies and tools for security testing and maintenance, focusing on load testing, security stress testing, and regular audits.
Load testing is essential to determine how your Laravel application behaves under significant stress, which can mimic either standard or peak usage scenarios. LoadForge is a powerful tool for simulating a large number of users interacting with your application to test the resilience and scalability of your Laravel environment.
Define Test Scenarios: Identify the parts of your application that will likely face the highest traffic. It generally includes user login, data retrieval, and data submission endpoints.
Configure Load Tests: Using LoadForge, configure the test scenarios by specifying the number of users, duration, and the nature of requests (GET, POST, etc.).
LoadForge allows customization of scripts to simulate real-world user behavior accurately.
Run Tests: Execute the tests and collect the data on response times, failure rates, and server resource utilization.
Analyze Results: Analyze the test outcomes to pinpoint bottlenecks and potential points of failure in your application.
While load testing focuses on volume, security stress testing emphasizes the application's resilience against malicious attacks. Laravel applications should be regularly checked for vulnerabilities such as SQL injection, XSS, CSRF, and more.
Use Automated Tools: Tools like OWASP ZAP can help automate the process of finding security vulnerabilities in your application.
Manual Testing: Engage security experts to perform manual testing, especially for complex security workflows in the application.
Regular Updates: Keep Laravel and its dependencies up-to-date to mitigate vulnerabilities from outdated packages.
Maintaining security is not a one-time task but involves continuous evaluation and updating.
Regular testing and maintenance are crucial for safeguarding Laravel applications against potential threats. By utilizing tools like LoadForge for load testing and incorporating a routine of regular audits and continuous updates, developers can ensure that their applications remain secure, performance-optimized, and resistant to evolving cyber threats. Combining these practices will foster a robust security posture in the high-paced, dynamic landscape of web application development.