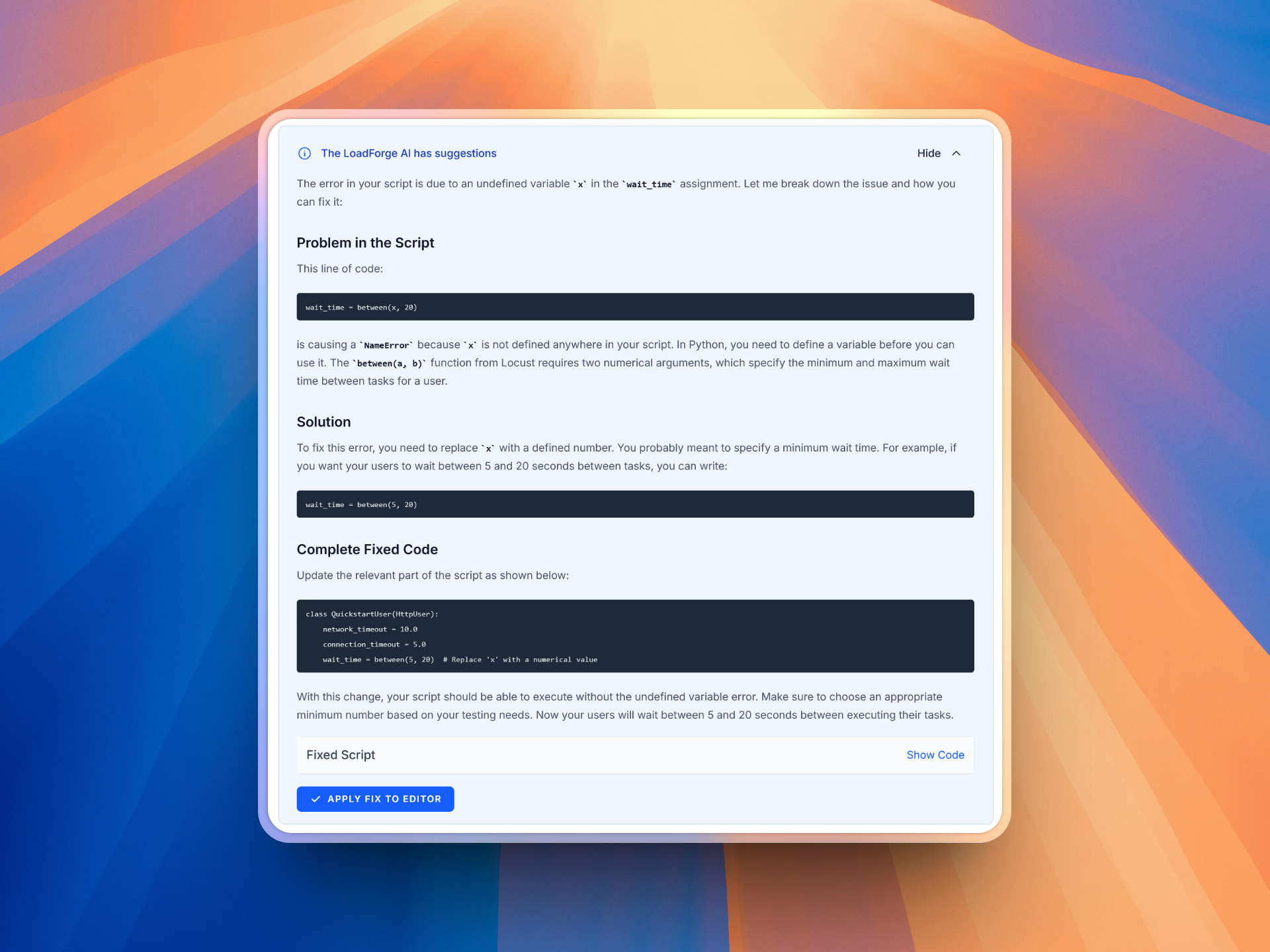
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
In the digital age, web applications handle a tremendous amount of traffic, ranging from legitimate user requests to potentially harmful automated scripts. Without proper control mechanisms, this influx can overwhelm your server, degrade performance, and even render your application unusable....
In the digital age, web applications handle a tremendous amount of traffic, ranging from legitimate user requests to potentially harmful automated scripts. Without proper control mechanisms, this influx can overwhelm your server, degrade performance, and even render your application unusable. This is where rate limiting comes into play.
Rate limiting is a crucial technique used to control the number of requests a user can make to an application within a specified timeframe. By defining these boundaries, you can ensure the stability, security, and reliability of your Laravel application.
Rate limiting serves several pivotal purposes, which make it an indispensable part of modern web development:
Implementing rate limiting in Laravel brings numerous benefits which can vastly improve the overall health and performance of your application. Some of the core advantages include:
Imagine a scenario where your Laravel-based API is being accessed by multiple clients. Some clients might be well-behaved, making periodic requests, while others might be making rapid-fire requests either intentionally (maliciously) or unintentionally (misconfigured client). Without rate limiting, these high-traffic clients might consume all your application's resources, slowing down or even halting service for others.
With rate limiting, you can define rules — for instance, allowing a maximum of 100 requests per minute from any given IP address. This guardrail helps ensure that all users have equitable access to your services:
Route::middleware('throttle:100,1')->group(function () {
Route::get('/user', function () {
// Protected routes go here
});
});
In this example, the throttle:100,1
middleware ensures that the routes in this group only allow 100 requests per minute per IP address — an effective way to prevent abuse and manage resource load.
In the subsequent sections, we will dive deeper into Laravel’s built-in rate limiting features and how to configure and customize these to suit your application’s unique needs. From setting up basic limits to creating sophisticated custom rate limiters and testing their effectiveness using LoadForge, you'll gain comprehensive knowledge to leverage rate limiting for securing and optimizing your Laravel application.
By the end of this guide, you will be well-equipped to implement and manage rate limits in Laravel, ensuring your application remains robust and performs optimally under varying loads.
Laravel comes equipped with powerful and flexible rate limiting capabilities right out of the box. These features enable developers to easily define and enforce limits on the number of requests that can be made to an application within a given timeframe, helping to mitigate abuse, prevent server overload, and ensure fair usage of resources.
Laravel's rate limiting mechanism revolves around the RateLimiter
class, which is part of the broader HTTP middleware stack. This class allows you to define rate limiting rules that can be applied to routes or groups of routes. Here are some key built-in features of Laravel's rate limiting:
Basic Rate Limiting
Dynamic Rate Limiting
Cache-Based Limit Tracking
IP Address Limiting
HTTP Headers for Rate Limit Status
Laravel uses middleware to handle rate limiting. Middleware acts as a filter that executes code before or after a request hits your application. The ThrottleRequests
middleware is central to Laravel's rate limiting implementation. When a request is processed:
X-RateLimit-Limit
, X-RateLimit-Remaining
, and Retry-After
to the HTTP response to manage rate limit state.Out of the box, Laravel provides an easy way to apply rate limiting to your routes. Here's how you might apply a simple rate limit to a route in your routes/web.php
or routes/api.php
file:
// Limits the route to 60 requests per minute
Route::middleware('throttle:60,1')->group(function () {
Route::get('/user', function () {
return 'User Data';
});
});
In this example:
'throttle:60,1'
applies the rate limiter, allowing up to 60 requests per minute.You can define global rate limits for your application using the app's RouteServiceProvider
. For example, you can set default rate limits in the boot
method of RouteServiceProvider
:
use Illuminate\Cache\RateLimiting\Limit;
use Illuminate\Support\Facades\RateLimiter;
class RouteServiceProvider extends ServiceProvider
{
public function boot()
{
$this->configureRateLimiting();
// Other boot logic
}
protected function configureRateLimiting()
{
RateLimiter::for('global', function ($request) {
return Limit::perMinute(100);
});
}
}
In this case, all routes within your application will be restricted to 100 requests per minute unless otherwise specified.
Laravel also allows for dynamic and more complex rate limits based on various request properties. For example, you could implement different limits depending on the authenticated user's role:
RateLimiter::for('api', function ($request) {
if ($request->user()->isPremium()) {
return Limit::perMinute(200);
}
return Limit::perMinute(100);
});
Here, premium users are allowed 200 requests per minute, while regular users are limited to 100 requests per minute.
These built-in features make Laravel a powerful tool for implementing effective rate limiting strategies, ensuring that your application remains performant and secure against abuse. With these foundational capabilities, you can move on to configuring and customizing your rate limiting to suit specific needs and use cases, as will be detailed in subsequent sections.
In this section, we'll guide you through the process of configuring rate limiting in your Laravel application. This includes setting up the rate limiters within the RateLimiter
service provider and configuring the appropriate middleware to enforce these limits. Follow these steps to protect your application from being overwhelmed by too many requests in a short period.
RateLimiter
Service ProviderLaravel provides a convenient way to define rate limiters in the RateLimiter
service provider. These limiters dictate how many requests a particular route or group of routes can handle within a given time frame.
Open the App\Providers\RouteServiceProvider.php
file. You'll define your rate limiters inside the configureRateLimiting
method.
namespace App\Providers;
use Illuminate\Support\Facades\RateLimiter;
use Illuminate\Cache\RateLimiting\Limit;
use Illuminate\Support\ServiceProvider;
class RouteServiceProvider extends ServiceProvider
{
protected function configureRateLimiting()
{
RateLimiter::for('global', function (Request $request) {
return Limit::perMinute(60);
});
RateLimiter::for('api', function (Request $request) {
return Limit::perMinute(30)->by($request->user()?->id ?: $request->ip());
});
}
}
In this example, we've defined two different rate limiters:
global
: This limiter restricts the application to 60 requests per minute for any incoming request.api
: This limiter allows each user or IP to make up to 30 requests per minute.Feel free to adjust the limits as per your application's requirements.
Next, you'll need to apply the rate limiters to routes using middleware. Laravel's throttle
middleware is designed for this purpose.
Open the routes/web.php
or routes/api.php
file and apply the middleware to your routes:
use Illuminate\Support\Facades\Route;
Route::middleware('throttle:global')->group(function () {
Route::get('/home', [HomeController::class, 'index']);
Route::get('/dashboard', [DashboardController::class, 'index']);
});
Route::middleware('throttle:api')->group(function () {
Route::get('/api/users', [ApiUserController::class, 'index']);
Route::get('/api/posts', [ApiPostController::class, 'index']);
});
In the example above:
global
rate limiter is applied to the /home
and /dashboard
endpoints.api
rate limiter is applied to the /api/users
and /api/posts
endpoints.You might need to adjust rate limits dynamically based on certain conditions. For example, premium users might have higher limits.
RateLimiter::for('dynamic', function (Request $request) {
return $request->user()?->is_premium
? Limit::perMinute(100)->by($request->user()->id)
: Limit::perMinute(30)->by($request->user()->id);
});
In this example, premium users receive a higher rate limit of 100 requests per minute compared to the standard 30 requests for regular users.
After configuring your rate limiters and middleware, it's crucial to test these settings in a controlled environment. You can utilize LoadForge to simulate different load scenarios and ensure your application handles rate limiting effectively.
Once your configurations are in place, execute your LoadForge tests, analyze the resulting data, and make any necessary adjustments.
By following these steps, you've successfully configured rate limiting in your Laravel application. This not only helps in maintaining the performance and reliability of your application but also secures it against potential abuse. Continue to the next sections to learn about creating custom rate limiters and other advanced configurations for a more tailored solution.
Creating custom rate limiters in Laravel allows you to tailor rate limiting rules to fit the unique requirements of your application. While Laravel provides robust out-of-the-box rate limiting features, there may be scenarios where default settings are insufficient or too generic. This section will guide you through the process of creating custom rate limiters, complete with examples and best practices to ensure your implementation is effective and efficient.
To create a custom rate limiter, you will primarily interact with the RateLimiter
facade within your App\Providers\RouteServiceProvider
. Below is a step-by-step guide to help you create custom rate limiters in Laravel.
Begin by registering your custom rate limiter in the RouteServiceProvider
. Open the App\Providers\RouteServiceProvider
file and locate the boot
method. Inside, you will use the RateLimiter::for
method to define your custom rate limiter.
// In App\Providers\RouteServiceProvider.php
use Illuminate\Support\Facades\RateLimiter;
use Illuminate\Cache\RateLimiting\Limit;
use Illuminate\Http\Request;
public function boot()
{
$this->configureRateLimiting();
$this->routes(function () {
// Register your routes
});
}
protected function configureRateLimiting()
{
RateLimiter::for('custom-limiter', function (Request $request) {
return Limit::perMinute(10)->by($request->ip());
});
}
Next, apply your newly defined rate limiter to specific routes or route groups using middleware. This can be done in your routes/web.php
or routes/api.php
files.
// In routes/web.php or routes/api.php
Route::middleware('throttle:custom-limiter')->group(function () {
Route::get('/user/profile', 'UserProfileController@show');
Route::post('/user/profile', 'UserProfileController@update');
});
In this example, routes within the group will be subject to the custom rate limit of 10 requests per minute, per IP address.
You may need to create more complex rate limiting rules based on various conditions, such as user roles, API keys, or other criteria. Here’s an example that includes user-based rate limiting:
// In App\Providers\RouteServiceProvider.php
RateLimiter::for('user-based-limiter', function (Request $request) {
$user = $request->user();
if ($user && $user->isAdmin()) {
// Higher rate limit for admins
return Limit::perMinute(60)->by($user->id);
} else {
// Standard rate limit for regular users
return Limit::perMinute(20)->by($user ? $user->id : $request->ip());
}
});
By following these steps and best practices, you'll be able to implement custom rate limiters in your Laravel application that cater to your specific needs. Custom rate limiters not only provide better control over your resources but also enhance the security and performance of your application.
Rate limiting on a per-user basis is crucial for ensuring fair usage and preventing abuse within your application. By tailoring rate limits to individual users, you can maintain equitable access to resources while protecting your system from being overwhelmed by any single user. This section delves into configuring per-user rate limiting in Laravel, complete with practical examples and code snippets.
Laravel makes it relatively straightforward to set up rate limiting for individual users. The RateLimiter
service provider allows you to define custom rate limiters based on different criteria. For per-user rate limiting, you can take advantage of the user's unique identifier (typically the user ID).
Define a Per-User Rate Limiter
First, you need to define a rate limiter within the RateLimiter
service provider located in the App\Providers
namespace. Open or create the file App\Providers\RouteServiceProvider.php
and add your custom per-user rate limiter.
use Illuminate\Support\Facades\RateLimiter;
use Illuminate\Cache\RateLimiting\Limit;
use Illuminate\Support\Str;
public function boot()
{
$this->configureRateLimiting();
$this->routes(function () {
// Define your routes here
});
}
protected function configureRateLimiting()
{
RateLimiter::for('api', function ($request) {
// Assuming you use the user ID to differentiate users
return Limit::perMinute(60)->by(optional($request->user())->id ?: $request->ip());
});
}
In this example, each authenticated user is allowed up to 60 requests per minute. If the user is not authenticated, rate limiting is applied based on the user's IP address as a fallback.
Apply the Rate Limiter to Routes
Next, you need to apply the newly defined rate limiter to your routes. You can do this using the throttle
middleware. Open your routes/api.php
file and apply the middleware to the routes you wish to protect.
Route::middleware(['auth:sanctum', 'throttle:api'])->group(function () {
Route::get('/user', function (Request $request) {
return $request->user();
});
Route::get('/posts', [PostController::class, 'index']);
});
Let's consider a scenario where you have an API endpoint that returns user posts. You want each user to be able to request their posts up to 100 times per hour.
Define the Custom Rate Limiter
Add the following code to the RouteServiceProvider.php
:
protected function configureRateLimiting()
{
RateLimiter::for('user-posts', function ($request) {
return Limit::perHour(100)->by(optional($request->user())->id ?: $request->ip());
});
}
Apply the Rate Limiter to the Specific Route
In your routes/api.php
file:
Route::middleware(['auth:sanctum', 'throttle:user-posts'])->get('/user/posts', [PostController::class, 'index']);
In this setup, each authenticated user can fetch their posts up to 100 times per hour. Unauthenticated users are rate-limited based on their IP addresses.
By correctly implementing per-user rate limiting, you can effectively prevent resource abuse while ensuring a positive user experience. The next section will address testing and verifying your rate limits to ensure they are functioning as intended using LoadForge.
Once your rate limiting measures are configured in your Laravel application, it's crucial to test and verify that they function as intended. Using LoadForge, you can simulate different traffic scenarios to ensure your limits effectively manage incoming requests and protect your resources. This section will walk you through the steps necessary to test and verify your rate limits using LoadForge.
Sign Up and Login:
Create a New Test:
When setting up your load tests, the following scenarios should be considered to comprehensively test your rate limiting configurations:
Normal Traffic Scenario:
Example:
{
"scenarios": [
{
"name": "Normal Traffic",
"requestsPerSecond": 10,
"duration": 60
}
]
}
Burst Traffic Scenario:
Example:
{
"scenarios": [
{
"name": "Burst Traffic",
"requestsPerSecond": 100,
"duration": 10
}
]
}
Sustained High Traffic Scenario:
Example:
{
"scenarios": [
{
"name": "Sustained High Traffic",
"requestsPerSecond": 50,
"duration": 300
}
]
}
Review HTTP Status Codes:
429 Too Many Requests
status codes as these indicate that rate limits are being applied.Example Response:
HTTP/1.1 429 Too Many Requests
Content-Length: 45
Content-Type: application/json
{
"message": "Too many requests, slow down!"
}
Analyze Response Times:
Evaluate Logs:
Based on the findings from your tests, you might need to adjust your rate limits to strike the right balance between user access and resource protection. Here’s how you can adjust rate limits in your RateLimiter
service provider:
use Illuminate\Support\Facades\RateLimiter;
public function boot()
{
RateLimiter::for('global', function (Request $request) {
return Limit::perMinute(100)->by($request->user() ? $request->user()->id : $request->ip());
});
}
Always retest your Laravel application after adjusting rate limit configurations to ensure the changes have the desired effect. Use LoadForge to run your defined scenarios again and compare the results with your previous tests.
Testing and verifying your rate limit configurations is an essential step to ensure they effectively manage traffic and protect your Laravel application. By using LoadForge, you can simulate various traffic scenarios and gain insights into the behavior of your rate limits, allowing you to adjust and optimize your configurations as necessary. This process ensures your application remains reliable and performs well under different conditions.
Properly handling responses when rate limits are exceeded is crucial for maintaining a good user experience while protecting your application. Users need to understand why their request was denied and how they might be able to avoid hitting limits in the future. In this section, we will discuss best practices for handling these scenarios in a way that is both developer-friendly and user-friendly.
The first step in handling rate limit exceeded responses is to use the right HTTP status codes. The most commonly used status code for rate limiting is 429 Too Many Requests. This informs the client that the user has sent too many requests in a given amount of time.
Laravel provides a simple way to send this status code using the built-in ThrottleRequests
middleware, but it’s important to ensure your custom limiters also use it.
if ($tooManyAttempts) {
return response()->json([
'message' => 'Too many requests, please try again later.'
], 429);
}
In addition to the status code, you should also provide a clear and informative message explaining the issue. This helps users understand what went wrong and offers guidance on what to do next.
Include a meaningful message in the response body. For instance, rather than just saying "Too Many Requests," you could be more specific:
if ($tooManyAttempts) {
return response()->json([
'message' => 'You have exceeded the rate limit. Please wait 15 minutes before making a new request.'
], 429);
}
Make it as easy as possible for your users to know when they can retry their request by including the Retry-After
header in the response.
if ($tooManyAttempts) {
return response()->json([
'message' => 'Too many requests, please try again later.'
], 429)->header('Retry-After', $retryAfterSeconds);
}
For a more user-friendly experience, consider notifying users through other channels (like email or in-app notifications) before they hit their rate limits. This proactive approach can prevent frustration and reduce the number of questions your support team needs to handle.
A well-structured error response typically includes:
if ($tooManyAttempts) {
return response()->json([
'status' => 429,
'error' => 'Too many requests',
'message' => 'You have exceeded the rate limit. Please wait 15 minutes before making a new request.',
'timestamp' => now(),
'retry_after' => $retryAfterSeconds
], 429);
}
For better monitoring and debugging, log events when users exceed rate limits. This helps you identify potential abuse patterns and adjust rate limits if needed.
if ($tooManyAttempts) {
Log::warning('Rate limit exceeded', [
'user_id' => $userId,
'timestamp' => now(),
'ip_address' => $request->ip()
]);
return response()->json([
'message' => 'Too many requests, please try again later.'
], 429);
}
Handling rate limit exceeded responses effectively ensures that your application remains user-friendly even under constraint conditions. By using appropriate status codes, informative messages, and structured responses, you help maintain a good user experience while protecting your resources. Additionally, logging and proactive user notifications can provide further insights and reduce the likelihood of users hitting these limits unexpectedly.
Rate limiting is not a set-it-and-forget-it feature; ongoing monitoring and adjustment are crucial to maintaining a balance between performance and security. In this section, we will explore strategies for monitoring the effectiveness of your rate limits, analyzing traffic patterns, and adjusting limits as necessary.
Effective monitoring involves keeping track of rate limit performance and violations. Here are some strategies to consider:
Logs and Metrics: Ensure that your application logs every rate limit hit and exceedance. Laravel's ThrottleRequests
middleware automatically logs such events. Use these logs to gain insight into traffic patterns and identify potential abuse.
// Example middleware for logging rate limit hits
if ($rateLimiter->tooManyAttempts($key, $maxAttempts)) {
Log::warning("Rate limit exceeded for key: {$key}");
return response('Too Many Requests', 429);
}
Dashboard Monitoring: Use monitoring dashboards (such as Grafana or Kibana) to visualize your rate limiting data. Monitoring dashboards help you detect trends and unusual patterns in API usage.
Alerts and Notifications: Configure alerts to notify you when rate limits are frequently exceeded. Alerts can be integrated with various tools such as Slack, email, or PagerDuty for instant notifications.
Analyzing traffic patterns is essential to identify when and where adjustments to rate limits are necessary:
Peak Traffic Times: Identify when your application experiences peak traffic. During these times, you may need to temporarily adjust rate limits to ensure performance while maintaining security.
User Behavior: Examine how users interact with your API. Different endpoints may require different rate limits based on their usage patterns. For example, an endpoint for submitting user comments might need stricter limits compared to a read-only endpoint.
Resource Utilization: Track server resource utilization (CPU, memory, etc.) in conjunction with rate limiting metrics. This can help you understand the impact of rate limits on server performance and adjust them accordingly.
Based on your monitoring data and traffic analysis, you may need to adjust your rate limits. Here’s how you can do it effectively:
Fine-Tuning Limits:
// Adjusting a rate limiter in the RateLimiter service provider
RateLimiter::for('api', function (Request $request) {
return Limit::perMinute(100)->by($request->user()->id ?: $request->ip());
});
Use the RateLimiter
service provider to fine-tune your rate limits per endpoint or per user.
Conditional Rate Limiting:
// Applying different limits based on user roles or other conditions
RateLimiter::for('api', function (Request $request) {
if ($request->user()->isPremium()) {
return Limit::perMinute(200);
}
return Limit::perMinute(100);
});
Apply different rate limits based on user roles, subscription plans, or other conditions to cater to varied user needs.
Testing Adjustments: Validate any changes by simulating traffic with LoadForge. LoadForge can help you understand the impact of your adjustments under different load scenarios.
// Example LoadForge script snippet for testing rate limits
loadtest({
// Your LoadForge configuration goes here
});
Regularly monitoring and adjusting rate limits ensures that your Laravel application remains secure, efficient, and user-friendly. By leveraging logs, metrics, dashboards, and LoadForge testing, you can continuously optimize your rate limiting strategy to meet evolving demands.
To provide a comprehensive understanding of how rate limiting can protect valuable resources in a Laravel application, let’s delve into some real-world scenarios where rate limiting has been effectively implemented. These examples will highlight practical applications, challenges solved, and the strategies used to ensure a balanced and secure environment.
An online e-commerce platform faced significant issues with bots scraping their product prices and availability, leading to server overloads and unfair advantages for competitors. By implementing rate limiting, they were able to mitigate these issues effectively.
The platform leveraged Laravel's built-in rate limiting features to restrict the number of requests per minute per IP address for their product endpoints.
use Illuminate\Cache\RateLimiting\Limit;
use Illuminate\Support\Facades\RateLimiter;
RateLimiter::for('products', function (Request $request) {
return Limit::perMinute(30)->by($request->ip());
});
The rate-limiting configuration significantly reduced server load by preventing automated bots from making excessive requests, ensuring fairer access for genuine users.
A Software as a Service (SaaS) provider offering an API-driven solution for marketing analytics faced frequent spikes in API requests that led to performance bottlenecks and degraded user experience. The company adopted a per-user rate-limiting strategy to ensure equitable resource distribution and maintain service availability.
Using a custom rate limiter, the API was configured to allow a maximum number of requests per user based on their subscription plan tiers.
use Illuminate\Cache\RateLimiting\Limit;
use Illuminate\Support\Facades\RateLimiter;
RateLimiter::for('api', function (Request $request) {
$user = $request->user();
return $user && $user->isPremium()
? Limit::perMinute(120)->by($user->id)
: Limit::perMinute(60)->by($user->id);
});
By tailoring rate limits based on user plans, the SaaS provider maintained a high quality of service while upselling premium plans, aligning resource usage with business goals.
A large-scale CMS catering to thousands of content creators experienced issues with distributed denial-of-service (DDoS) attacks against their login endpoints. This threatened the availability of the service for genuine users. Implementing rate limiting helped to detect and deter these malicious attempts.
To protect their authentication routes, the CMS applied stricter rate limits to the login endpoint while allowing higher thresholds for other less critical routes.
use Illuminate\Cache\RateLimiting\Limit;
use Illuminate\Support\Facades\RateLimiter;
RateLimiter::for('login', function (Request $request) {
return Limit::perMinute(5)->by($request->ip());
});
RateLimiter::for('default', function (Request $request) {
return Limit::perMinute(60)->by($request->ip());
});
The CMS drastically reduced the impact of DDoS attacks on their login system, enhancing overall security while maintaining accessibility for legitimate users.
A social media startup encountered issues with aggressive API usage that led to delays and database overloads. By implementing an adaptive rate limiting strategy, they managed to provide a smoother user experience and avoid potential downtime.
The application set up dynamic rate limiters for different API routes based on historical usage patterns and current server load.
use Illuminate\Cache\RateLimiting\Limit;
use Illuminate\Support\Facades\RateLimiter;
RateLimiter::for('posts', function (Request $request) {
return Limit::perMinute(50)
->response(function() {
return response('Too many requests', 429);
});
});
RateLimiter::for('comments', function (Request $request) {
return Limit::perMinute(100)
->response(function() {
return response('Slow down', 429);
});
});
By tailoring rate limits to specific routes and user behaviors, the social media app balanced the load effectively, resulting in improved performance and user satisfaction.
These examples illustrate the versatility and importance of rate limiting in handling a variety of real-world challenges faced by applications. Implementing rate limiting in Laravel not only enhances security and performance but also ensures a fair and accessible service for all users. By analyzing these case studies, you can better strategize and tailor rate-limiting solutions that meet the specific needs of your own application.
In this guide, we've navigated through the intricacies of configuring rate limiting in Laravel to ensure your application remains robust and efficient. Let's recap the key points and outline best practices to solidify your understanding and implementation of rate limiting.
Understanding Rate Limiting:
Built-in Rate Limiting Features:
RateLimiter
service provider and middleware.Configuring Rate Limiting:
RateLimiter
service provider and apply them using middleware.Creating Custom Rate Limiters:
Per-User Rate Limiting:
Testing and Verifying:
Handling Rate Limit Exceeded Responses:
Monitoring and Adjusting:
To ensure your rate limiting implementation in Laravel is both effective and efficient, consider the following best practices:
Be Conservative Initially:
Differentiate Based on Roles and Endpoints:
Use Token Bucket Algorithm:
Leverage Cache for State Management:
Provide Clear Error Messages:
Regularly Review and Adjust:
Test Extensively with LoadForge:
Document Your Limits:
By following these best practices, you'll ensure that your Laravel application's rate limiting mechanism is both efficient and effective, providing optimal server performance while protecting against abuse. Remember, rate limiting is not a set-and-forget feature; it requires continuous monitoring and adjustments based on real-world usage and insights.
Implementing these strategies will help you maintain a robust, user-friendly, and secure application environment.