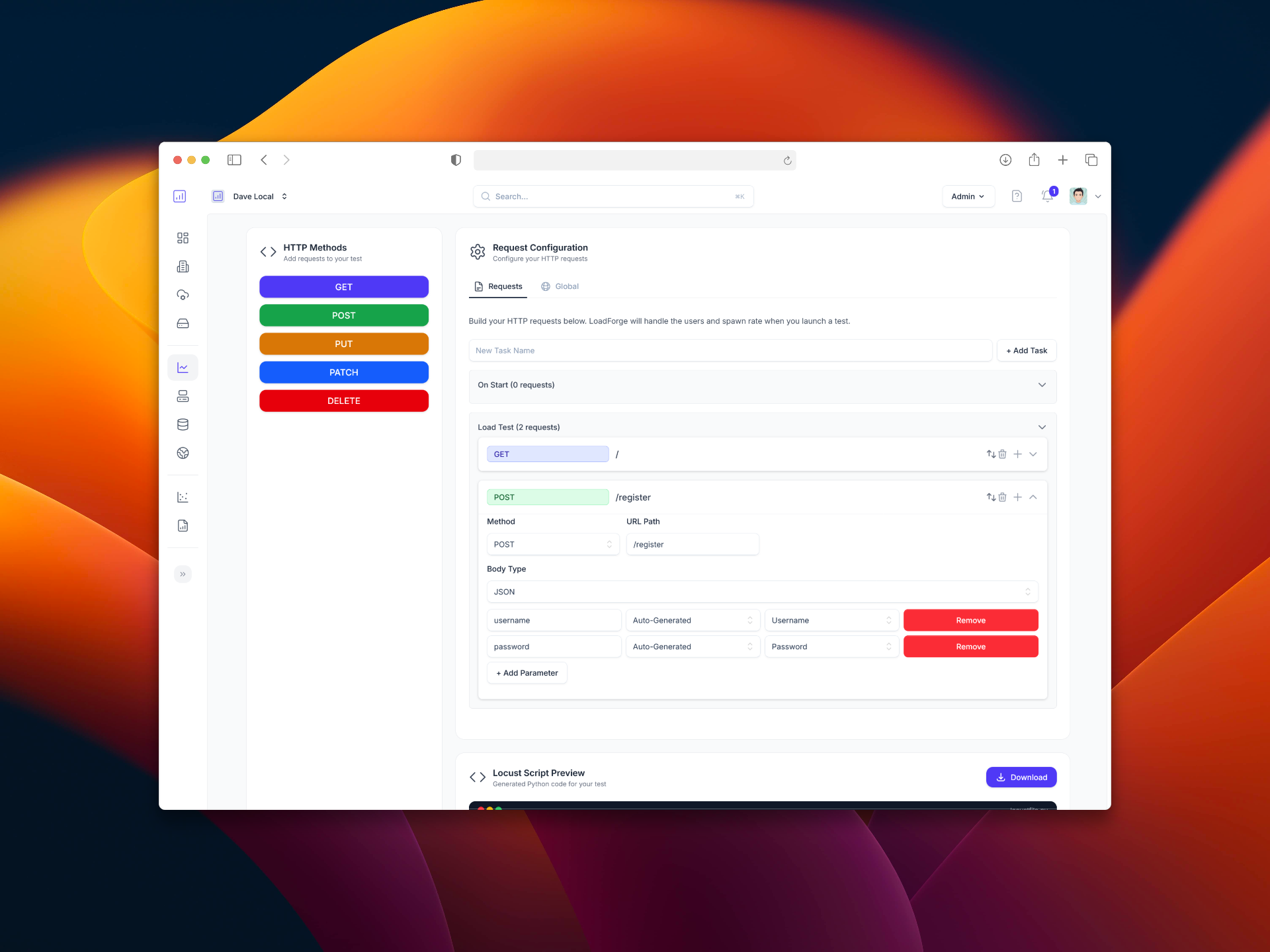
Jira Integration & Graphical Test Builder
We are proud to announce the release of two new features, available immediately. 🚀 Jira Integration: Automate Issue Tracking for...
In today's fast-paced digital landscape, maximizing the performance of your applications is crucial to ensuring a seamless user experience and optimal resource utilization. As organizations increasingly rely on containerization to deploy and manage their applications, Docker has emerged as a...
In today's fast-paced digital landscape, maximizing the performance of your applications is crucial to ensuring a seamless user experience and optimal resource utilization. As organizations increasingly rely on containerization to deploy and manage their applications, Docker has emerged as a leading platform due to its portability, scalability, and ease of use. However, achieving optimal performance in Docker environments can be challenging due to factors such as resource contention, inefficient configurations, and suboptimal resource allocation. This guide aims to provide you with best practices for Docker container resource allocation to help you maximize the performance of your Dockerized applications.
In this guide, we'll cover the following topics:
Understanding Docker Container Resource Allocation: We'll begin by exploring how Docker containers allocate and make use of system resources such as CPU, memory, disk I/O, and network. Understanding these fundamentals is crucial to optimizing container performance effectively.
Setting Resource Limits: Next, we'll provide guidelines on setting resource limits for CPU, memory, and other critical resources. Properly configured resource limits can ensure fair usage among containers and prevent resource contention that could degrade performance.
Using Docker Compose for Resource Management: Docker Compose facilitates the efficient management of multi-container applications. We'll demonstrate how to leverage Docker Compose to manage and limit resources across services, enhancing overall performance.
Optimizing Docker Images: Creating smaller and more efficient Docker images can significantly improve container startup times and reduce resource usage. We’ll share tips and techniques for building lean Docker images.
Leveraging Docker Swarm and Kubernetes: Container orchestration platforms like Docker Swarm and Kubernetes offer powerful tools for managing and scaling your containerized applications. We'll discuss best practices for utilizing these platforms to ensure efficient and scalable container management.
Monitoring and Profiling Container Performance: Ongoing monitoring and profiling are essential to identifying performance bottlenecks and understanding resource usage patterns. We’ll introduce tools and techniques that can help you keep track of your container performance metrics.
Load Testing Docker Containers with LoadForge: Load testing is critical to ensuring your containers can handle expected traffic and load. We’ll explain how to use LoadForge for comprehensive load testing of your Docker containers.
Optimizing Network Performance: Network performance can have a significant impact on the overall performance of your applications. We'll explore strategies to fine-tune network settings and enhance network throughput for Docker containers.
Managing Persistent Storage: Handling persistent storage effectively is key to maintaining performance and reliability. We'll share best practices for managing persistent storage in Docker containers.
Security Considerations: Performance optimization should never come at the cost of security. We'll discuss how to ensure that your performance enhancements do not compromise the security of your Docker containers.
Conclusion: Finally, we'll summarize the key points covered in the guide and provide additional resources for further reading.
By following the best practices outlined in this guide, you can optimize the performance of your Docker containers, ensuring they run efficiently and reliably in production environments. Let’s embark on this journey to mastering Docker container resource allocation and unlock the full potential of your containerized applications.
To effectively optimize and manage Docker containers, it is crucial to understand how they allocate and use system resources. Docker provides mechanisms to manage resources by wrapping processes in containers, which allows for the limitation and isolation of CPU, memory, disk I/O, and network resources. This section breaks down how Docker containers interact with each of these resources:
Docker containers share the host system's CPU by default. However, you can control the CPU usage of containers using the following options:
CPU Shares (--cpu-shares
): This sets the relative weight of CPU time allocation. For example, if one container has 1024 shares and another has 512, the first container gets twice the CPU time of the second in contention scenarios.
docker run --cpu-shares=1024 your_image
CPU Quota (--cpu-quota
) and CPU Period (--cpu-period
): These parameters control the absolute limit of CPU time. For example, a quota of 50000 and a period of 100000 microseconds means the container can use 50% of a single CPU.
docker run --cpu-quota=50000 --cpu-period=100000 your_image
By default, containers use as much memory as the host kernel allows. You can, however, set limits using the following options:
Memory Limit (--memory
): Defines the maximum amount of memory a container can use.
docker run --memory="256m" your_image
Memory Reservation (--memory-reservation
): Specifies a minimum reserve of memory. If the system runs low on memory, Docker tries to keep the available memory above this value.
docker run --memory-reservation="128m" your_image
Swap Limit (--memory-swap
): Controls the swap space usage. Setting it to a value doesn't allow for any swap usage beyond the memory limit set.
docker run --memory="256m" --memory-swap="256m" your_image
Disk I/O performance can significantly impact container performance, especially for I/O-intensive applications. Docker uses the I/O scheduler of the host to control I/O operations:
Block I/O Weight (--blkio-weight
): Sets relative weight for disk I/O, similar to CPU shares.
docker run --blkio-weight=500 your_image
Block I/O Device Limitations (--device-read-bps
, --device-write-bps
): Limits read/write rates (bytes per second) to specific devices.
docker run --device-read-bps /dev/sda:1mb --device-write-bps /dev/sda:1mb your_image
Network performance for Docker containers can be managed via the following:
Network Mode: Determines how containers interact with the host network stack. Common modes include bridge
(default), host
, none
, and container:<name|id>
.
docker run --network bridge your_image
Network Bandwidth Control: Use Linux Traffic Control (tc) to set constraints on network bandwidth.
To set these controls effectively, it's essential to monitor and understand each container's resource requirements and operating profile. Properly configured resource allocation ensures that containers run efficiently without starving others or the host system, leading to improved performance and stability.
In the following sections, we will cover setting these resource limits in further detail, strategies to optimize Docker images, and methods to monitor and profile container performance.
This section provides a comprehensive overview of how Docker handles resource allocation and sets the stage for implementing best practices in subsequent sections.
## Setting Resource Limits
Efficient resource allocation is crucial for maintaining optimal performance and stability in a Docker environment. Without proper control, some containers may monopolize system resources, leading to degraded performance for others. Setting resource limits for CPU, memory, and other resources ensures fair usage and helps prevent resource contention. This section provides guidelines for configuring these limits effectively.
### Understanding Resource Limits
Docker allows you to limit how much of the host's CPU, memory, and other resources a container can use. Here’s a breakdown of the primary resources you can limit:
1. **CPU**: Define how much CPU a container can use.
2. **Memory**: Set the maximum memory usage for a container.
3. **Block I/O**: Control the read and write throughput to block devices.
### Setting CPU Limits
Docker provides two primary options for setting CPU limits:
- **CPU shares**: Prioritizes CPU allocation when the system is under load. The default value is 1024.
- **CPU quota and period**: Limits the total amount of time a container can use the CPU in each scheduling period.
#### Example: Setting CPU Shares
<pre><code>
docker run --cpus-shares 512 my-container
</code></pre>
This command starts `my-container` with a CPU share value of 512, giving it half the default priority of 1024.
#### Example: Setting CPU Quota and Period
<pre><code>
docker run --cpus=2 my-container
</code></pre>
Here, `my-container` is limited to using no more than 200% of a single CPU or effectively using two CPU cores.
### Setting Memory Limits
Memory limits ensure a single container does not consume all available memory, leading to system instability. Docker provides the following parameters to manage memory:
- **`-m` or `--memory`**: Limits the maximum amount of memory a container can use.
- **`--memory-swap`**: Limits the total amount of memory plus swap space a container can use.
#### Example: Setting Memory Limits
<pre><code>
docker run -m 512m --memory-swap 1g my-container
</code></pre>
This example restricts `my-container` to a maximum of 512MB of memory and 1GB of combined memory and swap.
### Limiting Block I/O
You can control a container’s block I/O access using parameters:
- **`--device-read-bps`**: Limits the read rate from a device.
- **`--device-write-bps`**: Limits the write rate to a device.
- **`--device-read-iops`** and **`--device-write-iops`**: Limit the I/O operations per second.
#### Example: Limiting Block I/O
<pre><code>
docker run --device-read-bps /dev/sda:1mb --device-write-bps /dev/sda:1mb my-container
</code></pre>
In this example, `my-container` will be limited to 1MB per second read and write rate on the `/dev/sda` device.
### Practical Guidelines for Setting Resource Limits
To effectively manage resource limits, follow these best practices:
1. **Assess Workload Requirements**: Understand the resource needs of your applications. Profile and monitor resource usage before setting hard limits.
2. **Prioritize Critical Services**: Ensure critical applications and services receive higher priority and resources.
3. **Avoid Over-committing Resources**: Over-committing can lead to resource contention and degraded performance. Be conservative with limits.
4. **Regularly Review and Adjust**: Continuously monitor and adjust resource limits based on usage patterns and performance data.
By adhering to these practices, you can ensure fair resource usage across your containers, optimizing the overall performance and stability of your Docker environment.
## Using Docker Compose for Resource Management
Managing the resource allocation of individual containers is crucial, but when dealing with multi-container applications, resource management becomes even more complex. Docker Compose is a powerful tool for defining and running multi-container Docker applications, and it offers a convenient way to manage resources across these containers. This section provides an overview of how to use Docker Compose for effective resource management.
### Overview of Docker Compose
Docker Compose allows you to define a multi-container application using a single YAML file, simplifying the orchestration and management of resources for your entire application stack. With Docker Compose, you can set resource limits and reservations for CPU, memory, and other resources across all your services, ensuring that each container gets its fair share and doesn't starve others of required resources.
### Resource Limits in Docker Compose
Docker Compose uses the `deploy` section in the `docker-compose.yml` file to specify resource limits and reservations. Below are the primary resource parameters you can set:
- **CPUs**: Limit and reserve CPU usage.
- **Memory**: Limit and reserve memory usage.
- **Disk I/O**: Control the read and write rate to disk.
- **Network**: Manage network bandwidth and latency (using a third-party plugin).
Here's a basic example showcasing how to set resource limits for CPU and memory in a `docker-compose.yml` file:
<pre><code>version: '3.8'
services:
web:
image: nginx
deploy:
resources:
limits:
cpus: '0.50' # Max 50% of available CPUs
memory: '256M' # Max 256MB of memory
reservations:
cpus: '0.25' # Reserve 25% of available CPUs
memory: '128M' # Reserve 128MB of memory
db:
image: postgres
deploy:
resources:
limits:
cpus: '1.0' # Max 100% of available CPUs
memory: '512M' # Max 512MB of memory
reservations:
cpus: '0.5' # Reserve 50% of available CPUs
memory: '256M' # Reserve 256MB of memory
</code></pre>
In this example, the `web` service uses the NGINX image and has its CPU limited to 50% and memory limited to 256MB, with reservations of 25% CPU and 128MB memory. The `db` service uses the PostgreSQL image with corresponding resource limits and reservations. These settings help ensure that the `web` and `db` services don't consume more than their fair share of system resources, preventing potential resource contention and performance degradation.
### Advantages of Using Docker Compose for Resource Management
1. **Centralized Configuration**: Define and manage resource limits for all services in a single YAML file.
2. **Consistency**: Ensure consistent resource allocation across development, testing, and production environments.
3. **Scalability**: Easily manage resources for scalable applications using the same configuration file across all environments.
4. **Simplicity**: Simplified syntax and structure for specifying complex resource allocations.
### Conclusion
Docker Compose offers a streamlined and straightforward way to manage resources for multi-container applications. By defining resource limits and reservations within the `docker-compose.yml` file, you can ensure that your services run efficiently and reliably, without stepping over each other for system resources. This approach not only improves the performance of individual containers but also enhances the stability and scalability of your entire application stack.
In the following sections, we'll explore additional strategies and tools to optimize Docker container performance, including how to load test Docker containers with LoadForge and best practices for managing persistent storage.
## Optimizing Docker Images
In the journey towards achieving high performance in Docker environments, one pivotal step is optimizing Docker images. Creating smaller and more efficient Docker images not only enhances container startup times but also reduces the overall resource usage, leading to a more streamlined and scalable application deployment. Below are some strategic tips and techniques for optimizing your Docker images.
### 1. **Minimize Base Image Size**
The foundation of an optimized Docker image is choosing a minimal base image. Lightweight base images like `alpine` and `busybox` can significantly reduce the image size.
```Dockerfile
# Use Alpine as the base image
FROM alpine:3.12
Multi-stage builds allow you to use multiple FROM
statements in your Dockerfile, enabling you to copy only the necessary artifacts from one stage to the next, reducing the final image size.
# First Stage: Build
FROM golang:1.16-alpine as build
WORKDIR /app
COPY . .
RUN go build -o main .
# Second Stage: Final
FROM alpine:3.12
WORKDIR /root/
COPY --from=build /app/main .
CMD ["./main"]
Each instruction in a Dockerfile creates a new image layer. Combining multiple commands into a single RUN
directive can minimize the number of layers.
# Bad Practice
RUN apt-get update
RUN apt-get install -y curl
RUN apt-get clean
# Better Practice
RUN apt-get update && apt-get install -y curl && apt-get clean
Removing build dependencies and intermediate files after they are no longer needed cuts down on image bloat.
RUN apt-get update && apt-get install -y \
build-essential \
&& apt-get clean && rm -rf /var/lib/apt/lists/*
.dockerignore
FilesSimilar to .gitignore
, the .dockerignore
file helps you exclude unnecessary files and directories from being added to your Docker image context, reducing the size and improving the build time.
node_modules
.git
*.log
Always use specific version tags instead of latest
to ensure reproducibility and limit the risk of unintentionally including unwanted updates and bloat.
FROM node:14.16.0-alpine
Include only the essential dependencies required for your application, removing or not installing any unnecessary packages and files to maintain a lean and efficient image.
For Node.js applications:
COPY package.json package-lock.json ./
RUN npm install --production
Running containers as non-root users mitigates security risks and can also prevent unauthorized access and modifications.
# Create a non-root user and switch to it
RUN addgroup -S appgroup && adduser -S appuser -G appgroup
USER appuser
Using Docker's --squash
option can help flatten the image layers during the build process, though it requires enabling experimental features.
docker build --squash -t myapp:latest .
Regularly rebuild your images to apply the latest base image updates and security patches, ensuring optimal performance and security.
Employing these best practices for optimizing Docker images will help in creating smaller, more efficient images that not only improve startup times but also reduce the resource footprint of your containers. By refining your Docker images, you set the foundation for a highly performant and scalable containerized application environment. Continue reading the guide to explore more on managing and optimizing Docker container performance with other advanced techniques and tools.
In the realm of container orchestration, Docker Swarm and Kubernetes stand out as two powerful tools for managing and scaling containers. Both provide mechanisms for distributing workloads across clusters, ensuring high availability, and automating container deployment processes. This section will delve into the best practices for leveraging Docker Swarm and Kubernetes to manage and scale your Docker containers effectively.
Docker Swarm is Docker's native clustering and orchestration tool. It is simple to set up and use, making it a good starting point for container orchestration. Here are some best practices:
Cluster Configuration:
# Assigning a label to a node
docker node update --label-add region=us-east node-1
Overlay Networks:
docker network create --driver overlay my-overlay-network
Service Replication:
docker service create --name my-service --replicas 3 --network my-overlay-network my-image:latest
Resource Limits:
docker service create --name my-limited-service --limit-cpu 0.5 --limit-memory 512m my-image:latest
Kubernetes offers a more comprehensive and scalable solution for container orchestration with an extensive API and powerful features. Here are some best practices to follow:
Use Namespaces:
kubectl create namespace my-namespace
Deployments and StatefulSets:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-deployment
namespace: my-namespace
spec:
replicas: 3
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-container
image: my-image:latest
Horizontal Pod Autoscaling (HPA):
apiVersion: autoscaling/v1
kind: HorizontalPodAutoscaler
metadata:
name: my-hpa
namespace: my-namespace
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: my-deployment
minReplicas: 1
maxReplicas: 10
targetCPUUtilizationPercentage: 50
Resource Requests and Limits:
apiVersion: v1
kind: Pod
metadata:
name: my-pod
namespace: my-namespace
spec:
containers:
- name: my-container
image: my-image:latest
resources:
requests:
memory: "512Mi"
cpu: "500m"
limits:
memory: "1Gi"
cpu: "1"
Security Contexts and Network Policies:
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: allow-only-specific
namespace: my-namespace
spec:
podSelector:
matchLabels:
app: my-app
policyTypes:
- Ingress
ingress:
- from:
- podSelector:
matchLabels:
access: allowed
By adhering to these best practices, you can leverage Docker Swarm and Kubernetes to effectively manage and scale your Docker containers, ensuring optimal performance and reliability in your containerized applications. Ensuring the proper orchestration and resource allocation strategies not only enhances application performance but also helps in maintaining a stable and resilient system.
Effective monitoring and profiling of Docker containers are essential to ensure optimal performance, identify bottlenecks, and efficiently manage resource usage. By employing the right techniques and tools, you can gain valuable insights into your containerized environment and make informed decisions to enhance performance. In this section, we provide an overview of the top approaches and tools for monitoring Docker container performance.
Docker provides a built-in command called docker stats
that displays a live stream of container resource usage statistics. This command is useful for a quick and straightforward overview of CPU, memory, and network I/O usage.
To monitor a specific container:
docker stats [CONTAINER_NAME_OR_ID]
To monitor all running containers:
docker stats
Setting resource limits helps control the maximum resources a container can use. This practice not only prevents one container from hogging the host's resources but also aids in monitoring usage trends.
Set resource limits in a docker run
command:
docker run -d --name my_container --memory="256m" --cpus="0.5" my_image
cAdvisor (Container Advisor) is an open-source tool from Google that provides persistent resource usage and performance characteristics data for running containers. It supports Docker containers natively and can be used to collect, process, and export container metrics.
Run cAdvisor as a Docker container:
docker run -d \
--name=cadvisor \
--volume=/:/rootfs:ro \
--volume=/var/run:/var/run:ro \
--volume=/sys:/sys:ro \
--volume=/var/lib/docker/:/var/lib/docker:ro \
--publish=8080:8080 \
--detach=true \
gcr.io/cadvisor/cadvisor:latest
Access the cAdvisor UI at http://localhost:8080
.
Prometheus is a powerful time-series database and monitoring tool. Combined with Grafana for visualization, it provides a robust solution for collecting and displaying Docker container metrics.
Set up Prometheus with Docker:
docker run -d \
--name=prometheus \
-p 9090:9090 \
-v $PWD/prometheus.yml:/etc/prometheus/prometheus.yml \
prom/prometheus
Grafana can be used to visualize the metrics collected by Prometheus:
docker run -d \
--name=grafana \
-p 3000:3000 \
grafana/grafana
Use the Grafana dashboard to create visualizations and get deeper insights into resource usage patterns.
To effectively identify bottlenecks, it is crucial to:
Docker includes profiling capabilities that allow developers to capture detailed information on container resource consumption. Utilize docker inspect
for fetching container-specific configuration and state information.
docker inspect [CONTAINER_NAME_OR_ID]
Tools like Weave Scope
provide detailed insights into container environments by mapping out interactions and resource usage in real-time. This helps in better understanding resource distribution and identifying inefficient patterns.
Run Weave Scope:
curl -L https://scope.weave.works | sudo bash
Access the Weave Scope UI at http://localhost:4040
.
Monitoring and profiling are crucial for maintaining efficient, high-performing Docker containers. By employing tools like Docker Stats, cAdvisor, Prometheus, Grafana, and Weave Scope, you can gain profound visibility into your container’s performance metrics and identify potential bottlenecks under different workloads. Additionally, integrating profiling practices ensures your containers are running with the optimal resource configuration, paving the way for a high-performing containerized environment.
Load testing is critical to ensure your Docker containers can handle the expected traffic and load without degradation in performance. With LoadForge, you can simulate realistic load scenarios and identify potential bottlenecks before they become critical issues. This section will guide you through the process of using LoadForge to perform effective load testing on your Docker containers.
To begin load testing your Docker containers with LoadForge, follow these steps:
Create a LoadForge Account: First, ensure you have an active LoadForge account. You can sign up at LoadForge.
Install the LoadForge CLI: Install the LoadForge CLI on your local machine where you will run the load tests.
npm install -g loadforge-cli
Configure Authentication: Authenticate the CLI using your LoadForge API key.
loadforge auth [YOUR_API_KEY]
To effectively simulate the expected traffic and scenarios your Docker containers will face, you need to create a load test configuration. This configuration is usually a YAML or JSON file that describes the load patterns, HTTP methods, endpoints, and other parameters.
Here's an example configuration file in YAML format:
scenarios:
- name: "Basic Load Test"
weight: 1
flow:
- get:
url: "/api/v1/resource"
- post:
url: "/api/v1/resource"
json:
key: "value"
engine:
type: "constant"
stages:
- duration: 300
users: 50
With your configuration file ready, you can execute the load test against your Docker container. Ensure your Docker environment is up and running, and then execute the following command:
loadforge run -c [path_to_configuration_file] -t [target_url]
After the load test completes, LoadForge will provide detailed reports and analytics. Key performance metrics to examine include:
LoadForge's graphical reports make it easy to visualize these metrics and identify areas requiring optimization.
LoadForge offers advanced features such as geolocation testing, where you can simulate traffic from different parts of the world, and automated testing pipelines integrating with CI/CD tools for continuous performance validation.
For continuous performance testing and monitoring, integrate LoadForge into your CI/CD pipeline. This ensures every new build of your Docker container is subjected to load tests, catching performance regressions early. Here's an example using a simple shell script in a CI/CD pipeline:
stages:
- name: load_test
script:
- npm install -g loadforge-cli
- loadforge auth $LOADFORGE_API_KEY
- loadforge run -c load_test_config.yaml -t $TARGET_URL
By integrating LoadForge with your CI/CD processes, you maintain a robust and scalable Docker environment capable of handling real-world traffic.
Load testing with LoadForge is an essential step in ensuring your Docker containers can withstand the demands of production traffic. By following the steps outlined in this section, you can effectively simulate load conditions, identify performance bottlenecks, and ensure your containers operate efficiently under pressure.
This section provides a comprehensive guide to using LoadForge for load testing Docker containers, ensuring that the containers can handle the expected traffic and load efficiently.
## Optimizing Network Performance
Optimizing network performance is crucial for ensuring that your Docker containers communicate efficiently and swiftly, especially in a microservices architecture where numerous containers interact frequently. This section covers strategies and best practices for enhancing the network performance of your Docker containers, including network mode settings and network interfaces.
### Choosing the Right Network Mode
Docker offers several network modes that can impact the performance of your containerized applications. Understanding and choosing the appropriate network mode for your use case is the first step to optimize network performance.
1. **Bridge Network** (default for standalone containers):
- Suitable for containers on a single Docker host.
- Containers get their own IP addresses within the Docker subnet.
- Provides isolation but might add slight overhead due to NAT.
2. **Host Network**:
- Containers share the host's network stack.
- Removes NAT overhead, resulting in lower latency and increased throughput.
- Suitable when performance is critical and network isolation is less of a concern.
```bash
docker run --network host my_container
None Network:
docker run --network none my_container
Overlay Network:
docker network create --driver overlay my_overlay_network
To further optimize network performance, consider fine-tuning the network interfaces and related settings.
Reduce Network Overhead:
Custom Network Interfaces:
docker network create --subnet=192.168.1.0/24 my_custom_network
docker run --network my_custom_network --ip 192.168.1.100 my_container
Tune Kernel Parameters:
sysctl -w net.core.rmem_max=16777216
sysctl -w net.core.wmem_max=16777216
Efficient DNS resolution can significantly enhance network performance, especially in environments with dynamic container addresses.
Use Internal DNS: Docker’s built-in DNS server facilitates service discovery within the Docker network.
docker run --dns 127.0.0.11 my_container
Cache DNS Resolutions: Implement DNS caching inside containers to reduce lookup times.
Regular monitoring and diagnostics are essential for maintaining optimal network performance.
Network Metrics: Utilize tools like docker stats
and cAdvisor
to monitor network I/O metrics.
docker stats my_container
Network Profiling: Tools like Wireshark or tcpdump
can help profile network traffic and identify bottlenecks.
tcpdump -i eth0 -w trace.pcap
By carefully selecting the right network mode, fine-tuning network interfaces, optimizing DNS settings, and regularly monitoring performance, you can significantly improve the network performance of your Docker containers, ensuring a responsive and efficient application environment.
## Managing Persistent Storage
Efficiently handling persistent storage in Docker containers is crucial for optimizing performance and ensuring data integrity. Containers are ephemeral by nature, and without proper storage management, you risk data loss or suboptimal performance. In this section, we'll discuss best practices for managing persistent storage to strike the perfect balance between speed and reliability.
### Understanding Docker Storage Options
Docker provides several storage options, each suitable for different use cases:
- **Volumes**: Managed by Docker and stored outside the container filesystem. Volumes are the recommended option for persisting data as they offer benefits in performance, flexibility, and portability.
- **Bind Mounts**: Allow you to mount a file or directory from the host filesystem into the container. Bind mounts offer high flexibility but lesser portability.
- **tmpfs Mounts**: Store data in the host system’s memory. Suitable for temporary storage needs that do not require data persistence after the container stops.
### Best Practices for Using Volumes
1. **Use Named Volumes for Persistence**:
Named volumes provide a clear distinction between ephemeral and persistent data. They are managed by Docker and make it easier to back up and restore your data.
```yaml
version: '3.8'
services:
db:
image: postgres:latest
volumes:
- pg_data:/var/lib/postgresql/data
volumes:
pg_data:
Leverage Volume Drivers:
Docker's volume drivers enable you to store volumes on external storage systems like NFS, Amazon EFS, and more. This can enhance resilience and scalability.
volumes:
data:
driver: local
driver_opts:
type: nfs
o: addr=host.docker.internal,rw
device: ":/path/to/dir"
Optimize Volume Performance:
Ensure volumes are mounted with appropriate options. For instance, if using ext4 for a Linux-based host, enabling features like journaling can improve performance.
mkfs.ext4 -o journal_data_writeback /dev/sdX
Keep Host Paths Consistent:
When using bind mounts, ensure the host's directory structure remains consistent across different environments to avoid discrepancies.
version: '3.8'
services:
web:
image: nginx:latest
volumes:
- ./nginx.conf:/etc/nginx/nginx.conf:ro
Limit Bind Mount Usage:
Bind mounts should be used sparingly to avoid potential performance hits and permission issues, reserving them for cases where absolute host control is required.
Use tmpfs for Temporary Data:
Store temp data using tmpfs, which resides in the host’s memory, offering fast access and reducing disk I/O.
version: '3.8'
services:
app:
image: myapp:latest
tmpfs:
- /run
Leverage Docker's Build Cache:
Optimize Docker builds by utilizing multi-stage builds and caching mechanisms to speed up build times and minimize redundant tasks.
FROM node:14 AS build
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
FROM nginx:alpine
COPY --from=build /app/build /usr/share/nginx/html
Regular Backups:
Regularly back up your volumes, especially those storing critical data. This can be automated using various scripts or backup services.
Replication and High Availability:
Consider using replicated storage solutions to ensure high availability and fault tolerance for your data.
By following these best practices, you can efficiently manage persistent storage in Docker containers, ensuring both optimal performance and data reliability. Volumes should be leveraged for most persistent data needs, while bind mounts and tmpfs mounts can be used for specific scenarios.
Remember, proper storage management is a marathon, not a sprint. Regular monitoring and adjustments based on workload and environment changes will keep your system running smoothly.
When optimizing the performance of your Docker containers, it is crucial to ensure that these optimizations do not undermine their security. Performance improvements should be balanced with maintaining a robust security posture. Here are some essential security considerations to keep in mind:
Assign the minimum necessary privileges to your Docker containers. This minimizes the potential damage in case of a security breach:
Running as Non-Root User: By default, Docker containers run as the root user, which poses a significant security risk. Modify the Dockerfile to use a non-root user:
FROM ubuntu:latest
RUN useradd -m myuser
USER myuser
Capabilities: Limit the Linux capabilities granted to the container to the absolute minimum required using the --cap-drop
and --cap-add
flags.
docker run --cap-drop ALL --cap-add NET_BIND_SERVICE my-container
While optimizing network performance, ensure that you are not exposing your containers to unnecessary risks:
Limit Network Exposure: Use network modes like bridge
, host
, or overlay
judiciously. For external-facing services, avoid exposing unnecessary ports.
docker run -p 8080:8080 --network my-bridge-network my-container
Network Policies: Implement network policies to control traffic between containers, especially in Kubernetes environments.
Enhancing the performance of your Docker images should not come at the cost of security:
Trusted Base Images: Always base your Docker images on trusted, official base images. Regularly update your images to include the latest security patches.
Scanning for Vulnerabilities: Use tools like Trivy
or Docker's built-in scanning features to check your images for vulnerabilities:
docker scan my-container
Setting resource limits helps ensure that a single container does not monopolize system resources, but also reinforces security by preventing denial-of-service (DoS) scenarios:
cgroups: Use control groups (cgroups
) to limit and isolate resource usage:
docker run --memory="256m" --cpus="1.0" my-container
Namespaces: Utilize Docker namespaces to provide another layer of isolation, ensuring that containers operate in their own separate environments.
Keep your Docker daemon, images, and supporting infrastructure up to date:
Continuous monitoring and auditing are critical to maintaining security while optimizing performance:
Prometheus
, Grafana
, and Sysdig
for real-time monitoring of container performance and security.Managing secrets such as API keys and passwords securely is essential:
Docker Secrets: Use Docker secrets to manage sensitive data.
echo "my_secret_password" | docker secret create db_password -
Environment Variables: Avoid storing sensitive information in environment variables within Dockerfiles. Use vaults and secret management tools where possible.
Adhering to these security considerations ensures that your performance optimizations do not inadvertently introduce security vulnerabilities into your Docker environment. By taking a balanced approach, you can achieve both high performance and robust security for your containerized applications.
In this guide, we've covered a comprehensive range of best practices and techniques aimed at optimizing the performance of Docker containers. By following these recommendations, you can ensure efficient resource utilization, improved container startup times, and overall better performance for your containerized applications.
To deepen your understanding of Docker container optimization, consider exploring the following resources:
Docker Documentation: Comprehensive resource for all Docker-related features and best practices. Docker Docs
Kubernetes Documentation: In-depth guides and tutorials for managing Kubernetes clusters. Kubernetes Docs
LoadForge Documentation: Learn how to perform advanced load testing with LoadForge. LoadForge Docs
PerfKitBenchmarker: A benchmarking tool that can be used for performance testing cloud infrastructure. PerfKitBenchmarker
Optimizing Docker containers is crucial for maintaining the performance and efficiency of your applications. Proper resource allocation, efficient image creation, robust orchestration, thorough monitoring, and diligent load testing are essential components of a well-tuned Docker environment. By implementing the best practices outlined in this guide, you can achieve a robust, high-performing Docker infrastructure.
Continue exploring and experimenting with these techniques to find the optimal configurations for your specific use case and workload requirements. Happy optimizing!