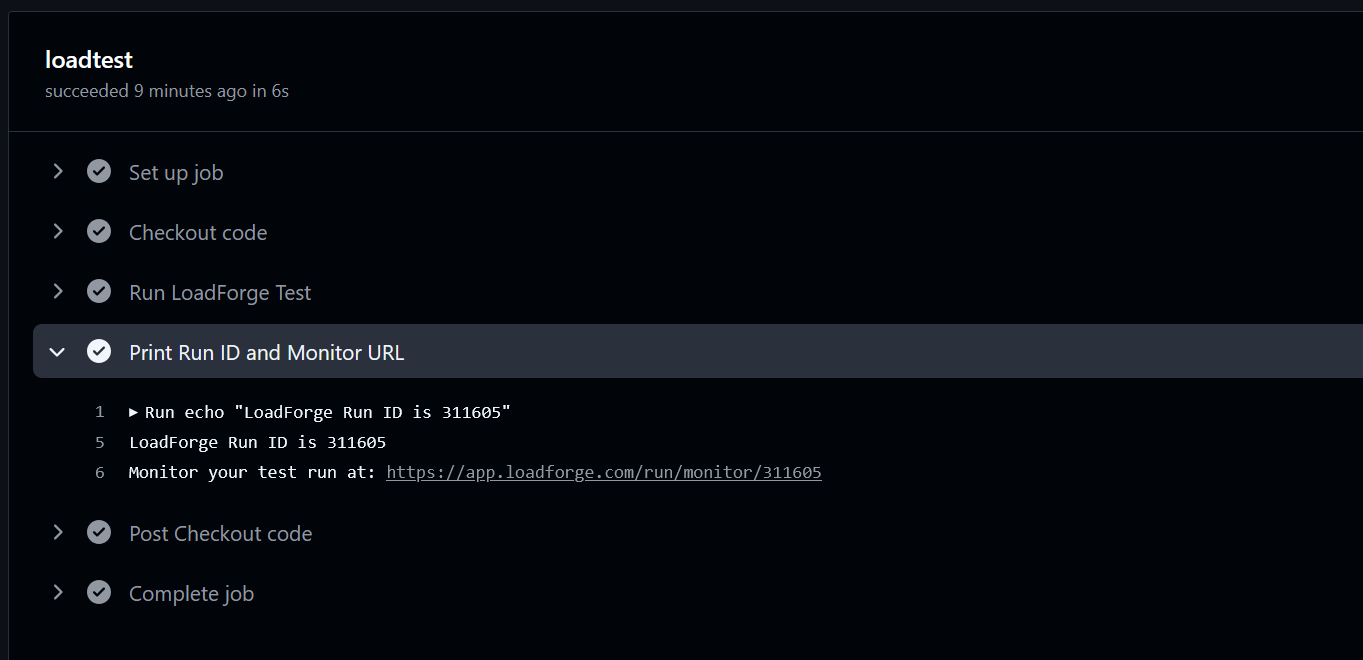
LoadForge GitHub Integration
Performance testing just got a major upgrade. LoadForge is thrilled to announce a seamless GitHub integration that lets you launch...
Learn how to effectively load test a WordPress blog or CMS with LoadForge. This guide will demonstrate simulating real users browsing your blog posts at random using LoadForge and locust.io
You are now browsing the LoadForge locust test directory. You can use these tests as a starting point for your own tests, or use our AI wizard to generate one automatically.
Performance is crucial for ensuring a good user experience, and slow load times can negatively affect your website's SEO and user retention. By load testing (or stress testing), you can identify bottlenecks, server issues, or inefficiencies in your website code or configuration.
LoadForge offers an easy way to do this, and the best part? You can start with a free account!
Automatic Crawling: Once set up, the test will automatically crawl valid URLs from your WordPress blog or CMS. This mimics the behavior of real users who might hop from one blog post to another.
Random Browsing: The test doesn't just stick to one URL. It picks links at random, offering a more realistic simulation of user behavior.
Adaptability: While this guide uses the example of a WordPress blog, the principles can be applied to many types of websites and web applications.
The provided code below is designed to:
/blog/
page of your WordPress site.Moreover, it simulates users accessing certain static contents like images, styles, and scripts which are essential for proper page rendering.
from locust import HttpUser, task, between
from pyquery import PyQuery
import random
class QuickstartUser(HttpUser):
wait_time = between(5, 9)
def index_page(self):
r = self.client.get("/blog/")
pq = PyQuery(r.content)
link_elements = pq(".blog-list-post h2 a")
self.blog_urls = []
for l in link_elements:
if "href" in l.attrib:
self.blog_urls.append(l.attrib["href"])
def on_start(self):
self.index_page()
@task(1)
def static_content(self):
# Fetch images
self.client.get("/img/logo/logo.png")
self.client.get("/img/images/cta_img.png")
self.client.get("/img/images/footer_fire.png")
# Fetch styles
self.client.get("/css/compiled.css?id=629e6d86d0748098936a")
# Fetch scripts
self.client.get("/js/compiled.js?id=1a927dbcfa8d96520959")
@task(3)
def blog_post(self):
url = random.choice(self.blog_urls)
r = self.client.get(url)
@task(3)
def home_page(self):
self.client.get("/blog/")
Did you know? LoadForge is powered by the robust open-source tool, locust.io. If you're already familiar with locust and have scripts ready, it's easy to import them to LoadForge and leverage its advanced testing capabilities.
Tip: Make sure to adjust the static content URLs and the selector used to fetch blog URLs based on your site's structure and design. This ensures the test's accuracy and relevance to your specific WordPress setup.