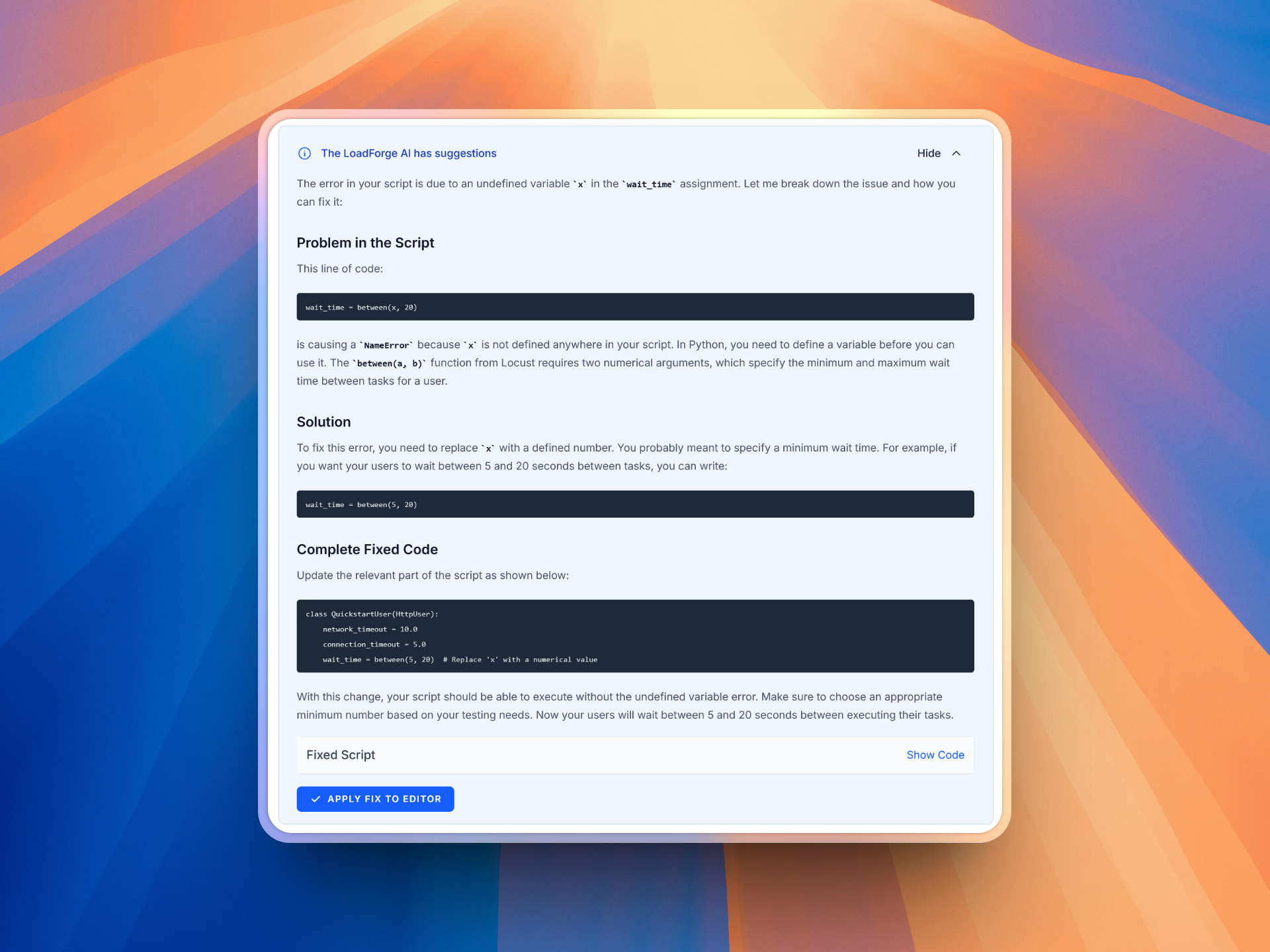
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
In today's fast-paced digital landscape, maintaining a high-performing web application is critical to ensuring a seamless user experience, excellent search engine optimization (SEO), and ultimately, the success of your digital platform. For developers leveraging server-side rendering (SSR) with React, performance...
In today's fast-paced digital landscape, maintaining a high-performing web application is critical to ensuring a seamless user experience, excellent search engine optimization (SEO), and ultimately, the success of your digital platform. For developers leveraging server-side rendering (SSR) with React, performance optimization is an essential consideration. SSR provides significant advantages over client-side rendering (CSR) by delivering fully-rendered HTML content to the user’s browser from the server, improving initial load times and overall site performance.
However, achieving and maintaining optimal performance for SSR React applications presents unique challenges. Factors such as server load, response times, and concurrent user handling capacity must all be finely tuned to meet user expectations. This is where systematic performance testing comes into play.
Effective load testing can simulate real-world user interactions and stress your SSR React application to identify performance bottlenecks and areas for improvement. LoadForge is a powerful tool specifically designed to facilitate this process. By using LoadForge, developers can create realistic load scenarios, monitor server and application behaviors under stress, and derive actionable insights to optimize performance.
This guide aims to provide a comprehensive roadmap for using LoadForge to conduct effective load testing of your SSR React applications. You'll learn how to set up your application, create and run load tests, analyze results, and implement performance optimizations based on empirical data. Whether you're optimizing your app for a global audience or ensuring it scales effectively during peak traffic periods, LoadForge equips you with the tools needed to maintain superior performance standards.
Let's embark on this journey to enhance the performance of your SSR React application with LoadForge. In the sections that follow, we will dive deeper into the fundamentals of SSR in React, the importance of performance, and step-by-step guidance on leveraging LoadForge for your load testing needs.
Server-side rendering (SSR) in React is a technique where the React application is rendered on the server rather than in the client's browser. The server generates the complete HTML for a requested page and sends it to the client, who can then display the content directly. This is in contrast to client-side rendering (CSR), where the browser downloads a minimal HTML and JavaScript bundle and renders the content of the page dynamically on the client’s device.
In SSR, the server processes the React components and issues the final HTML to the browser, creating a faster initial load time and better search engine optimization (SEO). Here’s a step-by-step overview of how SSR works:
SSR offers several advantages, particularly for performance and SEO:
Here are the key differences between SSR and CSR:
Feature | Server-Side Rendering (SSR) | Client-Side Rendering (CSR) |
---|---|---|
Initial Load Time | Faster, as HTML is pre-rendered on server | Slower, as JavaScript must fetch and render data |
SEO | Better, because search engines can index pre-rendered HTML | Worse, as search engines may struggle with JavaScript-rendered content |
Complexity | More complex to set up, requires server configuration | Simpler, often involves a static file server only |
User Experience | Improved due to rapid initial load | Might feel sluggish initially, but fast afterwards |
Interactive Features | Immediate content with subsequent hydration | All interactivity is loaded upfront |
Here's a basic example to illustrate how SSR can be implemented with React:
// server.js
const express = require('express');
const React = require('react');
const { renderToString } = require('react-dom/server');
const App = require('./src/App').default;
const server = express();
server.get('*', (req, res) => {
const appString = renderToString(<App />);
const html = `
<!DOCTYPE html>
<html>
<head>
<title>SSR with React</title>
</head>
<body>
<div id="root">${appString}</div>
<script src="bundle.js"></script>
</body>
</html>
`;
res.send(html);
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
In the example above, the renderToString
method from react-dom/server
is used to render our App
component to a string on the server-side. This string is then embedded into the HTML template, which is sent to the client.
Understanding the fundamentals of SSR in React is essential for optimizing the performance and SEO of your web applications. By leveraging the power of SSR, developers can create faster, more responsive, and SEO-friendly websites. In the next sections, we will delve into the importance of performance, the role of load testing, and how LoadForge can be a crucial tool in ensuring your SSR React application remains performant even under heavy load.
In today's digital landscape, the performance of your Server-Side Rendered (SSR) React application is vital for various reasons: user experience, search engine optimization (SEO), and the overall success of your application. This section elaborates on why performance is crucial and highlights the specific benefits for SSR React apps.
The user experience (UX) is directly tied to the performance of your application. The faster your app responds, the more satisfied your users will be. Here are some key points to consider:
Search engines like Google prioritize fast-loading websites in their search ranking algorithms. An optimized SSR React app can significantly improve your SEO metrics:
Performance can make a tangible difference in the overall success of your application, including business metrics and operational efficiency:
To further illustrate, let’s consider a simple comparison in rendering times between an optimized and a non-optimized SSR React app. Below is a basic example:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
const html = renderToString(<App />);
setTimeout(() => {
res.send(html);
}, 5000); // Simulated delay
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
const express = require('express');
const app = express();
app.get('/', (req, res) => {
const html = renderToString(<App />);
res.send(html); // Immediate response
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this simplified example, the difference in response time is significant—5 seconds vs. 100 milliseconds. This can substantially influence user behavior, SEO ranking, and overall application efficacy.
By understanding why performance matters, particularly in SSR React applications, you can take concrete steps to improve it, thereby ensuring a smooth, reliable, and satisfying user experience. Subsequent sections of this guide will focus on how to achieve this through effective load testing using LoadForge.
Load testing is a critical aspect of ensuring that your server-side rendered (SSR) React applications can handle a variety of stress conditions without compromising performance. Here's a comprehensive look at what load testing entails, why it's crucial, and the typical scenarios where it's necessary, focusing specifically on SSR applications.
Load testing is a type of performance testing that evaluates how a system behaves under an increasing load. Its primary objective is to determine the system's capacity and ensure it can handle high traffic while maintaining optimal performance. During load testing, the following performance characteristics are typically measured:
Load testing is crucial for multiple reasons:
There are several scenarios where load testing becomes essential, including, but not limited to:
For SSR React applications, the goals of load testing are aligned with ensuring that both the client and server-side components can handle stress conditions effectively. Here are key objectives:
By understanding these aspects, you can better prepare your SSR React application for the demands of real-world use. LoadForge offers an integrated solution to help you simulate real-world conditions, measure performance, and uncover areas needing improvement, setting the stage for the next steps in your load testing journey.
In the next sections, we'll delve into how to get started with LoadForge and effectively set up and run load tests for your SSR React application.
In this section, we will dive into the essentials of LoadForge, exploring its core features and understanding why it stands out as an invaluable tool for load testing your Server-Side Rendered (SSR) React applications.
LoadForge is a powerful cloud-based load testing platform designed to evaluate and enhance the performance of web applications. It simulates real-user traffic, helping you uncover potential performance bottlenecks before they affect your end-users. With its user-friendly interface and robust features, LoadForge allows you to seamlessly integrate load testing into your development workflow.
LoadForge offers a variety of features tailored to meet the demands of modern web applications, including SSR React apps:
LoadForge can simulate thousands to millions of concurrent users, allowing you to test your application's limits and understand its behavior under varying load conditions.
With real-time monitoring, you can observe how your SSR React app performs during load tests. Live dashboards provide insights into key metrics such as response times, throughput, and error rates, enabling you to make data-driven decisions.
LoadForge supports the creation of complex load testing scenarios, allowing you to mimic real-world user interactions. You can define user patterns, including login sequences, navigation paths, and form submissions, to ensure comprehensive testing.
Post-test reports offer a deep dive into your application's performance, highlighting critical areas that need improvement. These reports include graphs, charts, and detailed logs, which are crucial for identifying and resolving performance issues.
LoadForge integrates seamlessly with Continuous Integration/Continuous Deployment (CI/CD) pipelines, allowing you to automate load tests as part of your deployment process. This ensures that performance testing is an ongoing part of your development cycle.
SSR React applications have unique performance characteristics due to the server-side rendering process. LoadForge helps ensure that your SSR React app can handle real-world traffic by offering:
To begin using LoadForge for your SSR React application, follow these steps:
Sign Up and Set Up Your Project
Install LoadForge CLI
npm install -g loadforge-cli
Configure Your Load Test
{
"name": "SSR React App Load Test",
"description": "Load test for the SSR React application.",
"scenarios": [
{
"name": "Homepage Load",
"requests": [
{
"method": "GET",
"url": "https://your-ssr-react-app.com/"
}
],
"concurrency": 50,
"duration": "5m"
}
]
}
Run Your Load Test
loadforge run
Monitor and Analyze Results
By leveraging LoadForge's robust features and seamless integration capabilities, you can efficiently load test your SSR React application, ensuring it delivers optimal performance under any load condition.
Setting up your SSR (Server-Side Rendering) React application for load testing is a crucial step in ensuring your application is ready to handle real-world traffic. This involves configuring your environment, preparing your codebase, and implementing best practices to provide an accurate picture of your application's performance under load.
First, you need to ensure that your testing environment closely mirrors your production environment. This includes setting up similar server hardware, network conditions, and software stacks. Here are some key points to consider:
tc
(Traffic Control) on Linux to emulate latency, packet loss, and bandwidth constraints.In preparation for load testing, it's important to ensure your codebase is optimized and ready to handle high traffic loads. Below are the steps to follow:
// Example of lazy loading a non-critical module in React
import React, { lazy, Suspense } from 'react';
const HeavyComponent = lazy(() => import('./HeavyComponent'));
const App = () => (
<Suspense fallback={<div>Loading...</div>}>
<HeavyComponent />
</Suspense>
);
export default App;
Implementing best practices for both your server and application can make a significant difference in performance. Here are some of the most effective strategies:
Setting up automated deployment scripts for your load tests can save time and ensure consistency. Use CI/CD tools like Jenkins, GitHub Actions, or GitLab CI to automate the deployment of your application and infrastructure.
# Example GitHub Actions Workflow
name: Deploy for Load Testing
on: [push]
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/[email protected]
with:
node-version: '14'
- name: Install Dependencies
run: npm install
- name: Build Application
run: npm run build
- name: Deploy to Server
run: |
scp -r ./build/ user@server:/var/www/app
ssh user@server 'sudo systemctl restart app'
Prior to running full load tests, execute warm-up and smoke tests. Warm-up tests help prepare your system by simulating a gradual increase in traffic, while smoke tests check the stability of the deployment.
By thoroughly preparing your SSR React application according to the strategies and best practices outlined above, you set the stage for effective and reliable load testing with LoadForge. Following these steps will help you identify performance bottlenecks and optimize your application to handle scale, ultimately leading to a better user experience and a more robust application.
In this section, we will walk you through the process of creating load tests using LoadForge for your SSR React application. This guide will cover setting up test scenarios, defining user patterns, and establishing success criteria to ensure thorough and effective load testing.
A test scenario in LoadForge is a simulated user journey through your SSR React application. Before creating a test scenario, it’s important to identify the critical user flows and prioritize them based on their impact on the user experience.
User patterns define how users will interact with your application during the test. LoadForge allows you to simulate different types of users with varying behavior patterns.
Success criteria are the metrics that indicate whether your load test has met its goals. It's essential to establish these criteria beforehand to evaluate the results accurately.
Now that you’ve set up your test scenarios and user patterns, it’s time to create the actual load test in the LoadForge platform.
Below is an example configuration snippet for a load test on LoadForge:
{
"testName": "SSR React App Load Test",
"description": "Load test for key user flows in the SSR React app",
"scenarios": [
{
"name": "Home Page Load",
"url": "/home",
"thinkTime": 2000
},
{
"name": "Search Items",
"url": "/search",
"thinkTime": 3000
},
{
"name": "Complete Purchase",
"url": "/purchase",
"thinkTime": 5000
}
],
"userPatterns": {
"initialUsers": 50,
"maxUsers": 500,
"rampUp": "10m"
},
"successCriteria": {
"responseTime": {
"home": "2s",
"search": "3s",
"purchase": "5s"
},
"errorRate": "1%",
"systemMetrics": {
"cpuUsage": "80%",
"memoryUsage": "75%"
}
}
}
Once you have configured the load test, validate the test settings to ensure there are no errors. Then, save the test configuration.
At this point, you have successfully created a load test using LoadForge for your SSR React application. In the next sections, we will discuss how to run these tests and analyze the results to optimize your application's performance.
Once you have set up your SSR React app and created load tests with LoadForge, it's time to execute these tests and delve into the analysis of the results. This section will guide you through the process of running the tests, monitoring performance metrics during the test runs, and interpreting the results to uncover and address performance bottlenecks.
Start the Load Test: To begin running load tests on your SSR React app, log in to your LoadForge account and navigate to the test suite that you have previously set up. Select the specific test scenario you want to execute and hit the "Start Test" button.
Test Parameters: Ensure that all your test parameters such as virtual users, ramp-up time, and duration are configured as per your requirements. For example, you might choose to start with 50 virtual users and gradually increase to 500 over a span of 10 minutes.
Real-time Monitoring: As the test runs, LoadForge provides real-time monitoring of several key performance indicators (KPIs). These include response time, error rates, throughput, and more. Utilize these live metrics to gain immediate insights:
During the execution of the load test, it's crucial to monitor several real-time metrics to assess your application's performance under load:
Resource Utilization: Keep an eye on your server's CPU, memory, and disk I/O usage. High utilization could indicate potential bottlenecks.
top
iostat -x 1
Network Latency: Monitor network latency to ensure that your application's response times are not adversely affected by network delays.
ping yourserver.com
Logs: Tail your server logs to catch any unexpected behaviors or errors during the test.
tail -f /var/log/your_app.log
Once the load test is complete, LoadForge presents a comprehensive analysis of the results. Here's how you can interpret the data to identify performance bottlenecks:
Response Time Distribution: Examine the distribution of response times. A wide spread in response times or a high percentage of slow responses can be an indicator of performance issues.
+------------------+--------+
| Response Time | Count |
+------------------+--------+
| < 1s | 300 |
| 1s - 2s | 150 |
| 2s - 3s | 40 |
| > 3s | 10 |
+------------------+--------+
Error Rates: Identify periods where error rates spike. This can help you pinpoint specific scenarios or load conditions under which your application fails.
{
"timestamp": "2023-10-05T14:48:00Z",
"error_rate": 5.2
}
Throughput Analysis: Evaluate the throughput during the load test. A sudden drop in throughput coupled with rising response times can indicate resource contention or server overload.
Throughput: 250 req/s
Bottleneck Identification: Using LoadForge's detailed reports, identify areas where your SSR React app shows performance degradation. Common bottlenecks include:
Below is a sample report you might see after a test run:
Metric | Value |
---|---|
Total Requests | 10000 |
Successful Requests | 9950 |
Failed Requests | 50 |
Average Response Time | 1.2s |
90th Percentile Response | 1.8s |
Peak Throughput | 300 req/s |
Average Throughput | 250 req/s |
By closely monitoring these metrics and thoroughly analyzing the results provided by LoadForge, you can uncover critical performance issues within your SSR React application. This forms a solid foundation for optimizing your app to handle higher loads, ensuring a smooth and responsive user experience even under heavy traffic conditions.
Once you've gathered the results from your LoadForge load tests, it's time to dive into performance optimization. By analyzing the insights provided by LoadForge, you can pinpoint bottlenecks and systematically enhance the performance of your SSR React app. This section will provide you with effective strategies and tips to achieve this.
Review the server response times as reported by LoadForge. A high response time could indicate issues such as inefficient server code, high database query times, or inadequate server resources.
Action Steps:
The TTFB metric indicates how long it takes for the first byte of a page to reach the user's browser after a request is made. High TTFB often points to server processing delays.
Action Steps:
Render-blocking resources can delay the rendering of your page, increasing the overall load time.
Action Steps:
<link rel="stylesheet" href="styles.css" media="print" onload="this.media='all'">
<noscript><link rel="stylesheet" href="styles.css"></noscript>
Images can significantly impact your page load time if not optimized.
Action Steps:
<img src="placeholder.jpg" data-src="actual-image.jpg" class="lazyload">
srcset
to serve appropriately sized images based on the user's device.<img src="small.jpg" srcset="small.jpg 300w, medium.jpg 768w, large.jpg 1024w" sizes="(max-width: 768px) 100vw, 50vw">
Effective client-side caching can drastically reduce load times for returning users.
Action Steps:
Cache-Control
headers for static assets.Cache-Control: public, max-age=31536000, immutable
CDNs distribute content to servers closer to your users, reducing latency and improving load times.
Action Steps:
<link rel="stylesheet" href="https://cdn.example.com/styles.css">
<script src="https://cdn.example.com/main.js"></script>
After applying these optimizations, it's critical to re-test your application using LoadForge to measure the improvements. Compare the new test results with previous ones to ensure that the changes positively impact performance metrics like server response times, TTFB, and overall load times.
Optimizing your SSR React app based on LoadForge test results involves a continuous process of analyzing, adjusting, and validating performance improvements. By addressing bottlenecks, improving render performance, leveraging caching strategies, and continuously monitoring results, you can significantly enhance your application's responsiveness and user experience.
In the next section, we will discuss maintaining performance over time through continuous performance testing and how to integrate LoadForge into your development workflow for ongoing success.
This structured approach ensures you systematically enhance the performance of your SSR React application, leading to improved user satisfaction and better overall application success.
## Best Practices for Continuous Performance Testing
Continuous performance testing is crucial for maintaining the high performance of your SSR React applications over time. By establishing a routine and integrating LoadForge into your development workflow, you can ensure that your application consistently meets performance benchmarks, scales effectively, and delivers an optimal user experience. Here are some best practices to help you achieve this:
### Set Up Regular Testing Schedules
Regular and consistent testing helps catch performance regressions early. Consider the following strategies to set up an effective testing schedule:
- **Weekly Tests:** Schedule full-scale load tests weekly to identify any emerging performance issues.
- **Daily Smoke Tests:** Run lighter load tests daily to ensure that recent changes haven't adversely affected performance.
- **Before Major Releases:** Execute comprehensive load tests before every major release to validate performance under expected peak loads.
### Automate Load Tests
Automation is key to maintaining an efficient and reliable testing process. Here’s how to automate your load testing with LoadForge:
1. **Use Scripts:** Write scripts to automate the initiation of load tests using LoadForge's CLI or API.
2. **Integrate with CI/CD Tools:** Connect LoadForge with CI/CD tools like Jenkins, GitHub Actions, or GitLab CI to run performance tests as part of your deployment pipeline.
**Example Script for Jenkins:**
```yaml
pipeline {
agent any
stages {
stage('Build') {
steps {
// Your build steps here
}
}
stage('Load Test') {
steps {
sh 'loadforge-cli start-test --test-id YOUR_TEST_ID'
}
}
stage('Analyze Results') {
steps {
script {
def result = sh(script: 'loadforge-cli get-results --test-id YOUR_TEST_ID', returnStdout: true).trim()
echo "Load test results: ${result}"
// Parse and evaluate performance metrics here
if (result.contains('fail')) {
error 'Performance criteria not met'
}
}
}
}
}
}
Continuous performance testing is only as good as your ability to interpret the results. Make sure to:
Use the insights gained from LoadForge tests to make informed optimizations:
Integrating LoadForge into your CI/CD pipeline ensures that performance testing is a seamless part of your development workflow:
Example CI/CD Integration:
jobs:
build-and-test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Build project
run: npm install && npm run build
- name: Run LoadForge tests
run: |
loadforge-cli start-test --test-id ${{ secrets.LOADFORGE_TEST_ID }}
# Wait and fetch results
result=$(loadforge-cli get-results --test-id ${{ secrets.LOADFORGE_TEST_ID }})
echo "Test results: $result"
# Conditionally fail the job
if [[ $result == *"fail"* ]]; then
echo "Performance criteria not met."
exit 1
fi
As your SSR React app evolves, ensure your load testing scenarios reflect the current state of the application:
These best practices for continuous performance testing will help maintain the performance and reliability of your SSR React app over time. By leveraging LoadForge’s powerful tools, automating tests, and integrating performance testing into your CI/CD pipeline, you can ensure your application remains responsive and efficient, delivering an excellent user experience at all times.
In this section, we'll explore real-world examples and case studies where SSR React applications have significantly benefited from using LoadForge for load testing and performance optimization. These case studies highlight the practical applications and tangible results achieved by leveraging the powerful capabilities of LoadForge.
An e-commerce platform built with SSR React was experiencing slow page load times and high server response times during peak shopping seasons. The performance issues were affecting user experience and convergent rates, necessitating a thorough performance evaluation.
Using LoadForge, the development team initiated comprehensive load tests simulating peak traffic conditions, mimicking user behaviors like browsing, searching for products, and checking out.
Scenario Setup:
{
"scenarios": [
{
"name": "Peak Shopping Load Test",
"user_count": 10000,
"duration": "1h",
"steps": [
{
"action": "browse",
"path": "/"
},
{
"action": "search",
"path": "/search?q=electronics"
},
{
"action": "click",
"path": "/product/123456"
},
{
"action": "add_to_cart",
"path": "/cart"
},
{
"action": "checkout",
"path": "/checkout"
}
]
}
]
}
The LoadForge test revealed several bottlenecks:
Based on LoadForge results, the team optimized the SSR React app by:
Post-optimization load tests showed:
A popular news aggregator using SSR React faced issues with slow updates and high latency during high traffic events, particularly during breaking news.
The engineering team used LoadForge to replicate high-traffic events, pushing the limits to observe how the server and application handled sudden spikes.
Scenario Setup:
{
"scenarios": [
{
"name": "Breaking News Load Test",
"user_count": 5000,
"duration": "30m",
"steps": [
{
"action": "load_homepage",
"path": "/"
},
{
"action": "click",
"path": "/news/breaking-news-123"
},
{
"action": "refresh",
"path": "/news/breaking-news-123"
}
]
}
]
}
The LoadForge report indicated:
Optimizations included:
Subsequent tests with LoadForge demonstrated:
A SaaS application providing real-time data analytics and visualizations used SSR React for its frontend. Users reported performance degradation with increased data volumes and simultaneous user interactions.
LoadForge was employed to simulate various user interactions such as data fetching, visualization rendering, and interactive reporting.
Scenario Setup:
{
"scenarios": [
{
"name": "Data Analytics Load Test",
"user_count": 3000,
"duration": "45m",
"steps": [
{
"action": "load_dashboard",
"path": "/dashboard"
},
{
"action": "fetch_data",
"path": "/api/data"
},
{
"action": "render_chart",
"path": "/dashboard/chart/567"
},
{
"action": "download_report",
"path": "/dashboard/report"
}
]
}
]
}
The LoadForge analysis pinpointed:
Enhancements included:
Post-optimization results:
These case studies underscore the importance of using LoadForge for performing detailed load testing. By identifying and addressing performance bottlenecks early, these organizations significantly improved their SSR React apps' responsiveness, scalability, and user satisfaction. LoadForge's detailed analysis and flexible testing scenarios make it an invaluable tool in optimizing SSR React applications for real-world demands.
In this guide, we explored the critical aspects of ensuring peak performance for your server-side rendered (SSR) React applications using LoadForge for load testing. Let's recap the essential points we've covered:
Introduction to SSR and Its Importance: We began by understanding what server-side rendering (SSR) is, its benefits over client-side rendering (CSR), and why performance is a pivotal factor in the success of SSR React applications. Enhanced user experience, better SEO, and faster load times are just a few of the reasons that make SSR a compelling choice for modern web applications.
Significance of Performance in SSR Applications: We delved into why performance matters, discussing how it directly impacts user satisfaction, search engine rankings, and the overall effectiveness of the application. High performance translates to happier users, improved retention, and a competitive edge in the market.
Introduction to Load Testing: We introduced the concept of load testing, explaining its necessity in simulating real-world usage scenarios to identify performance bottlenecks. Common scenarios where load testing is essential include preparing for high traffic events, validating infrastructural changes, and ensuring scalability.
Getting Started with LoadForge: This section highlighted the unique features of LoadForge, demonstrating why it is an ideal tool for load testing SSR React applications. Its user-friendly interface, powerful capabilities, and detailed reporting make it an invaluable resource for developers aiming to optimize their applications.
Setting Up and Creating Load Tests: We provided a comprehensive guide on preparing your SSR React app for load testing, followed by a step-by-step approach to creating effective load tests with LoadForge. Setting up test scenarios, understanding user patterns, and defining success criteria are crucial steps in this process.
Running Tests and Analyzing Results: After setting up the tests, understanding how to run them effectively and analyze the results is critical. We addressed the importance of monitoring during tests and interpreting LoadForge’s detailed reports to uncover performance issues.
Optimizing Performance Based on Results: Using the insights gained from the load tests, we discussed various strategies and tips to optimize the performance of your SSR React app. Optimization can involve fine-tuning server configurations, optimizing database queries, or revisiting your application's architecture.
Best Practices for Continuous Performance Testing: Recognizing that maintaining performance is an ongoing task, we recommended best practices for continuous performance testing. This includes setting up regular testing schedules, automating tests, and integrating LoadForge into your CI/CD pipeline to ensure your application remains performant as it evolves.
Case Studies and Examples: Real-world case studies illustrated how other SSR React applications have successfully utilized LoadForge for load testing and performance optimization, showcasing tangible benefits and improvements.
In conclusion, load testing is not a one-time effort but a continuous process that plays an indispensable role in maintaining the high performance of SSR React applications. Using LoadForge allows you to simulate real-world traffic, identify bottlenecks, and make informed decisions to enhance the performance and reliability of your web application. By following the strategies and best practices outlined in this guide, you can ensure your SSR React application is robust, scalable, and provides the best possible experience for your users. Implement regular load testing with LoadForge to stay ahead of potential issues and keep your application running smoothly under any load conditions.