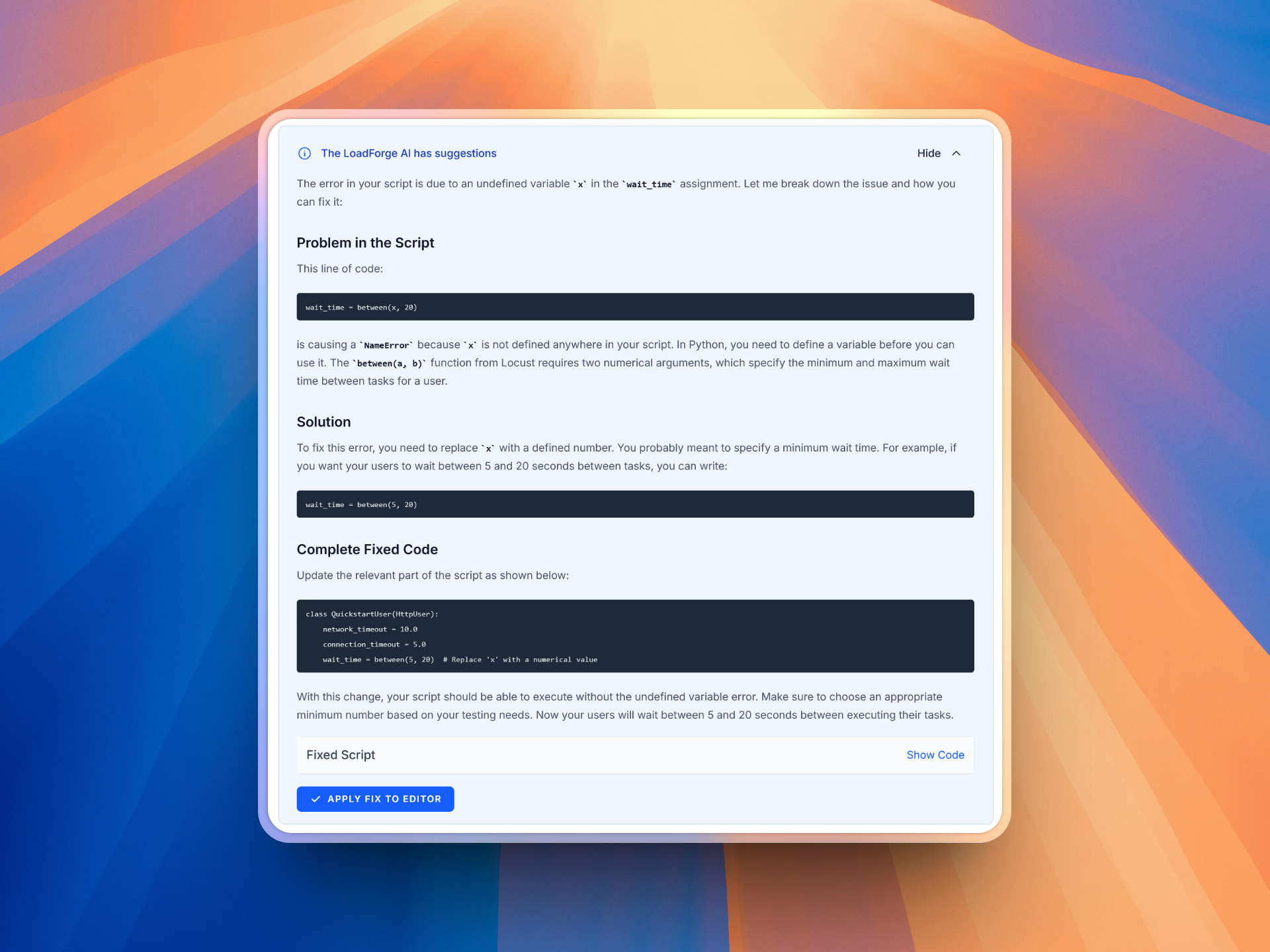
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
The isset() function is an essential component in the PHP programming language, primarily used for checking if a variable has been set and is not NULL. Its significance in PHP coding, especially in conditional statements, cannot be overstated, providing a...
The isset()
function is an essential component in the PHP programming language, primarily used for checking if a variable has been set and is not NULL. Its significance in PHP coding, especially in conditional statements, cannot be overstated, providing a foundational tool for developers to handle various data-check scenarios safely and effectively.
The primary purpose of isset()
is to determine if a particular variable exists and has a non-NULL value. This is particularly crucial in web development where variables may not always be initialized or might be set dynamically due to user input or database responses. Using isset()
allows you to safely handle data without encountering undefined variable errors.
In PHP, conditional statements control the flow of execution based on certain conditions. isset()
plays a pivotal role in these statements by ensuring that operations dependent on variable values are executed only when the variables are properly initialized and not NULL. This is fundamental in avoiding runtime errors and in making the code robust and error-resistant.
The syntax for isset()
is straightforward:
if (isset($variable)) {
// Code to execute if $variable is set and is not NULL
}
Here, the isset()
function checks whether $variable
is set. The code inside the block executes only if this condition is true. The simplicity of isset()
makes it highly useful for checking mandatory fields, such as checking whether user input is received before processing it:
if (isset($_POST['username'])) {
echo "Username: " . htmlspecialchars($_POST['username']);
} else {
echo "Username is required.";
}
In this example, the script checks if the username is part of the posted data before using it, thereby safeguarding against errors related to accessing an undefined array index.
Overall, isset()
is an indispensable function for any PHP developer, ensuring data integrity and error-free code execution in web applications. As you progress through this guide, you will uncover more advanced usage scenarios and best practices to leverage isset()
effectively in your projects.
The isset()
function in PHP is fundamental for determining if a variable has been set and is not NULL
. This function helps avoid errors that can occur when trying to access uninitialized variables. Understanding its syntax and the flexibility of its parameters is essential for effective PHP programming.
The basic syntax of the đisset()
function is straightforward:
isset($variable)
The function returns TRUE
if the provided variable exists and its value is not NULL
; otherwise, it returns FALSE
.
isset()
can accept one or more parameters, which expands its functionality significantly. Here’s how it operates with multiple parameters:
isset($var1, $var2, ..., $varN) €pre>
- **$var1, $var2, ..., $varN**: These are the variables to be checked. `isset()` will only return `TRUE` if all of the given variables are set and not `NULL`. ### Examples of Multiple Parameter Usage: 1. **Checking Multiple Variables** You might want to ensure that several form inputs are filled out before processing them. Here's how you could use `isset()` to check multiple variables:if (isset($firstName, $lastName, $email)) { // Execute this block if all fields are provided }
Combining with Other Conditions
isset()
can be easily combined with other conditions in a singleif
statement for more complex checks:if (isset($sessionUser) && $sessionUser->isActive()) { // Check both that the session user exists and that the account is active }
Practical Tips
Avoid Type Confusion: Since isset()
also check that the variable is not NULL
, ensure that you're clear about the variable types you're working with.
Initialization Check: It's good practice to initialize variables to NULL
if you intend to later check them with isset()
. This clarifies your intentions and ensures cleaner code.
Use in Arrays: isset()
is particularly useful when dealing with arrays, especially with keys that may not be set in all circumstances:
if (isset($data['key'])) {
// Only access $data['key'] if it exists
}
Understanding and using the isset()
function effectively allows PHP developers to write more robust, error-free applications by safely managing variable usage and initialization. As we proceed, keep in mind that combining isset()
with other functions and proper error handling strategies can significantly improve the quality of your code.
The isset()
function in PHP serves as a fundamental tool in various programming contexts to ensure that variables are both declared and not null before they are accessed. This section will explore typical scenarios where isset()
proves invaluable, such as form handling, session management, and managing optional array elements. We will provide practical code examples for each scenario to demonstrate their real-world applicability.
One of the most common uses of isset()
is in processing form data. It helps in checking if form inputs are set, thereby avoiding undefined index errors and ensuring that necessary data fields are filled before processing. Here's an example in the context of a simple login form:
if (isset($_POST['username']) && isset($_POST['password'])) {
$username = $_POST['username'];
$password = $_POST['password'];
// Perform login authentication
} else {
echo 'Both username and password are required.';
}
In this scenario, isset()
prevents the script from attempting to access $_POST['username']
or $_POST['password']
if they were not provided, which would lead to an error.
isset()
is crucial in session management for checking if a user session is already established. This allows you to handle user sessions securely by verifying session variables before use:
session_start();
if (isset($_SESSION['user_id'])) {
echo "User is already logged in";
// Redirect to dashboard or perform other session-specific actions
} else {
echo "Please login to access this page.";
}
This code effectively prevents unauthorized access to pages that require a valid user session, by checking the presence of user_id
in the session.
Sometimes, arrays might have optional elements that are not always set. The isset()
function can be used to handle such scenarios gracefully without triggering errors. Take the following example with associative array data representing a user:
$userData = [
'name' => 'John Doe',
'email' => '[email protected]',
// 'age' is not always available
];
if (isset($userData['age'])) {
echo "Age: " . $userData['age'];
} else {
echo "Age information is not available.";
}
This usage of isset()
ensures that the script checks for the 'age' index only when it is available, avoiding any undefined index errors without compromising the program's functionality.
By incorporating isset()
in these common scenarios, developers can write more robust, error-resistant PHP code. The function's ability to verify variable initialization before use not only enhances security by preventing unauthorized access but also significantly improves the overall reliability and readability of the code.
In this section, we explore more complex applications of the isset()
function, delving into nested conditions and its integration with other PHP functions. We will also discuss best practices for crafting clean and efficient conditional statements using isset()
to enhance both performance and readability in your code.
isset()
Nested conditions are pivotal when dealing with complex data structures or scenarios where multiple checks are necessary before performing an operation. Using isset()
within nested conditional statements allows you to verify the existence of multiple variables or array indices in a structured and clear manner.
Consider a scenario where you need to check the existence of an item deep within a multidimensional array:
$store = [
'fruits' => [
'apple' => ['price' => 1.00, 'stock' => 100],
'orange' => ['price' => 0.75, 'stock' => 50],
]
];
if (isset($store['fruits']['apple']) && isset($store['fruits']['apple']['price'])) {
echo "Apple is in stock and costs: $" . $store['fruits']['apple']['price'];
} else {
echo "Apple details are incomplete.";
}
This example demonstrates checking for the presence of multiple indices before accessing the data, thus avoiding potential errors due to missing data.
isset()
with Other PHP Functionsisset()
can be seamlessly integrated with other PHP functions to perform more dynamic checks and operations. This integration is commonly seen with functions that modify data, like unset()
, or those that handle output, such as echo
or print_r
.
isset()
with empty()
In scenarios where both the existence and the non-empty value of a variable are crucial, combining isset()
with empty()
offers a powerful tool:
$userInput = '';
if (isset($userInput) && !empty($userInput)) {
echo "Input provided: " . $userInput;
} else {
echo "No input or input is empty.";
}
This code checks not only that the variable $userInput
is declared but also ensures it contains a non-empty value.
isset()
in PHPWhen deploying isset()
in more advanced scenarios, maintaining clean, readable, and efficient code is essential. Here are some best practices to follow:
&&
, ||
) to combine checks meaningfully.isset()
but also aids in preventing other potential bugs.isset()
becomes complex, ensure to document the logic through comments to aid future developers (or yourself!) in understanding the intended logic.Using isset()
effectively in advanced scenarios requires a nuanced understanding of PHP syntax and logic. By following these guidelines and studying the provided examples, developers can avoid common pitfalls and ensure their applications handle data predictably and safely.
In PHP, choosing the right tool to assess the state of variables is crucial for writing efficient and understandable code. Three commonly used functions for this purpose are isset()
, empty()
, and is_null()
. Each of these functions serves slightly different purposes and understanding their differences will enable developers to select the most appropriate one based on their specific needs.
The isset()
function checks whether a variable is set and is not NULL
. It returns true
if the variable exists and has any value other than NULL
. If the variable is not set, or is set to NULL
, isset()
returns false
.
$var = "";
echo isset($car); // Outputs: false
$var = "LoadForge";
echo isset($var); // Outputs: true
empty()
is used to determine whether a variable is considered "empty". A variable is considered empty if it does not exist or if its value is one of the following: ""
(an empty string), 0
, 0.0
, null
, '0'
(0 as a string), an empty array, or a variable declared without a value. Unlike isset()
, empty()
will return true
if the variable is considered empty.
$var = 0;
echo empty($var); // Outputs: true
$var = false;
echo empty($var); // Outputs: true
is_null()
function checks specifically whether a variable has a value of NULL
. It is simple and straight-forward: it returns true
only if the variable is NULL
.
$var = NULL;
echo is_null($var); // Outputs: true
$var = 0;
echo is_null($var); // Outputs: false
Here’s a concise comparison:
isset()
tests if a variable is set and is not NULL
.empty()
tests if a variable is "empty", which includes ""
, 0
, 0.0
, null
, '0'
, or an empty array.is_null()
specifically checks if a variable is NULL
.Use isset()
when you need to ensure a variable has been initialized and is not NULL
. It's particularly useful to verify that multiple variables are set, using a single function call.
Use empty()
to check if a variable is devoid of any content that could represent a meaningful value. This is useful in form validation, where fields value could be received as 0
, an empty string, or NULL
.
Use is_null()
when you need strict checking that a variable’s content is exactly NULL
.
Selecting the right function between isset()
, empty()
, and is_null()
can influence the performance, clarity, and correctness of your PHP code. Understanding the literal and practical differences between these functions allows developers to implement more robust and error-free code handling mechanisms.
When using the isset()
function in PHP development, developers often face several common pitfalls and errors. This section aims to address these concerns, offering debugging solutions and tips to effectively handle potential problems while employing isset()
.
isset()
and empty()
A frequent source of confusion arises from the distinctions between isset()
and empty()
. While isset()
checks if a variable is set and is not NULL
, root()
determines if a variable is considered "empty", which could mean it is unset, NULL
, or has a falsey value (like 0
, ""
, false
, or array()
).
Example Problem:
$var = 0;
if (isset($var)) {
echo "This will print.";
}
if (empty($var)) {
echo "This will also print.";
}
Solution:
Understanding the specific use case—check if a variable is initialized (isset()
) or if it should be considered empty (root()
). Use isset()
when ensuring a variable has been defined irrespective of its value (unless it’s NULL
).
isset()
for Not Being NULL
isset()
only checks if a variable has been set and is not NULL
. Developers sometimes mistakenly expect it to validate that a variable has a substantive, non-falsey value.
Example Problem:
$someVar = "";
if (isset($someVar)) {
echo "This will print even if someVar is an empty string.";
}
Solution:
Clarify your validation requirements. If you need to ensure a variable has a non-empty value, consider combining isset()
with other checks, or use !empty()
directly if that fits the intent better.
isset()
with Array ElementsDevelopers often encounter errors when checking for the existence of specific keys or indices in arrays, especially when those keys might not be set.
Example Problem:
$data = ['name' => 'Alice', 'age' => 30];
if (isset($data['name'], $data['email'])) {
// This block will not run because 'email' is not set.
}
Solution: Always ensure you're checking all relevant array indices or use careful error handling to manage missing indices gracefully.
Before using isset()
, variables should be at least initialized. The overlook of this step can lead to behaviors difficult to debug.
Example Problem:
// Assuming $a was never defined
if (isset($a)) {
echo "This will not print.";
}
// Correct Initialization
$a = null;
if (isset($a)) {
echo "This will still not print, but the variable is properly initialized.";
}
Solution: Make sure all variables are initialized before they are used. This is a basic but crucial practice in PHP programming to avoid issues related to using undefined variables.
Improper use of isset()
might not necessarily generate errors but can lead to logical errors in the program flow. PHP’s error messages and warnings can offering significant insights when enabled.
Solution: Use development tools to enable error reporting during development:
ini_set('display_errors', 1);
error_reporting(E_ALL);
This will help identify any issues related to uninitialized variables or other mistakes.
isset()
, empty()
, and is_null()
.isset()
.By following these guidelines, developers can utilize isset()
more effectively, avoiding common pitfalls and ensuring more robust PHP applications.
In this concluding section, we delve into the best practices for using the isset()
function in PHP. These guidelines focus primarily on enhancing performance implications and code maintainability. Additionally, we highlight the importance of initializing variables properly before using isset()
. Implementing these practices ensures that your code is robust, readable, and efficient.
Before using isset()
, ensure that your variables are properly initialized. Avoid the common pitfall of checking variables that have not been set or initialized, as it can lead to inconsistent behavior or unexpected results.
For instance, always initialize the variable at the beginning of your script or in a scope that precedes its use:
$variable = null; // Initializing variable
if (isset($variable)) {
// Perform action
}
Instead of applying isset()
repeatedly on each variable, use it to check multiple variables at once. This not only cleans up the code but also improves performance by reducing the function's execution overhead.
Example of checking multiple variables:
if (isset($var1, $var2, $var3)) {
// Code block executes if all variables are set
}
While isset()
is crucial for checking undefined variables, using it where unnecessary (e.g., after you have already established a variable is set) can clutter and slowdown your code. Make sure that usage of `omez% is purposeful and judicious.
Example of unnecessary use:
$var = 'example';
if (isset($var)) { // Unnecessary as $var is clearly set
echo $var;
}
In some cases, combining isset()
with other conditional functions like empty()
can yield more precise control over your data handling.
if (isset($var) && empty($var)) {
// Executes if $var is set but is "empty"
}
When utilizing isset()
in complex conditions or in areas of the code where the presence of a variable is not obvious, document its use. Comments can help other developers understand why isset()
was necessary and what conditions are being checked.
Example:
// Check if optional configuration value is set
if (isset($config['timeout'])) {
set_timeout($config['timeout']);
}
As projects evolve, previously necessary isset()
checks might become obsolete or could be simplified. Regularly review and refactor your code to remove or streamline these checks. This keeps the codebase efficient and maintainable.
Remember, while isset()
itself is a very lightweight and fast function, misuse or overuse can degrade performance, especially in loops or large-scale applications. Always profile and optimize your use of isset()
in performance-critical applications.
Adhering to these best practices when using isset()
in PHP can significantly improve the performance, reliability, and clarity of your code. Proper usage ensures that your codebase remains maintainable and scalable while preventing common bugs associated with uninitialized variables. Always consider the context and specific needs of your application when applying these principles.