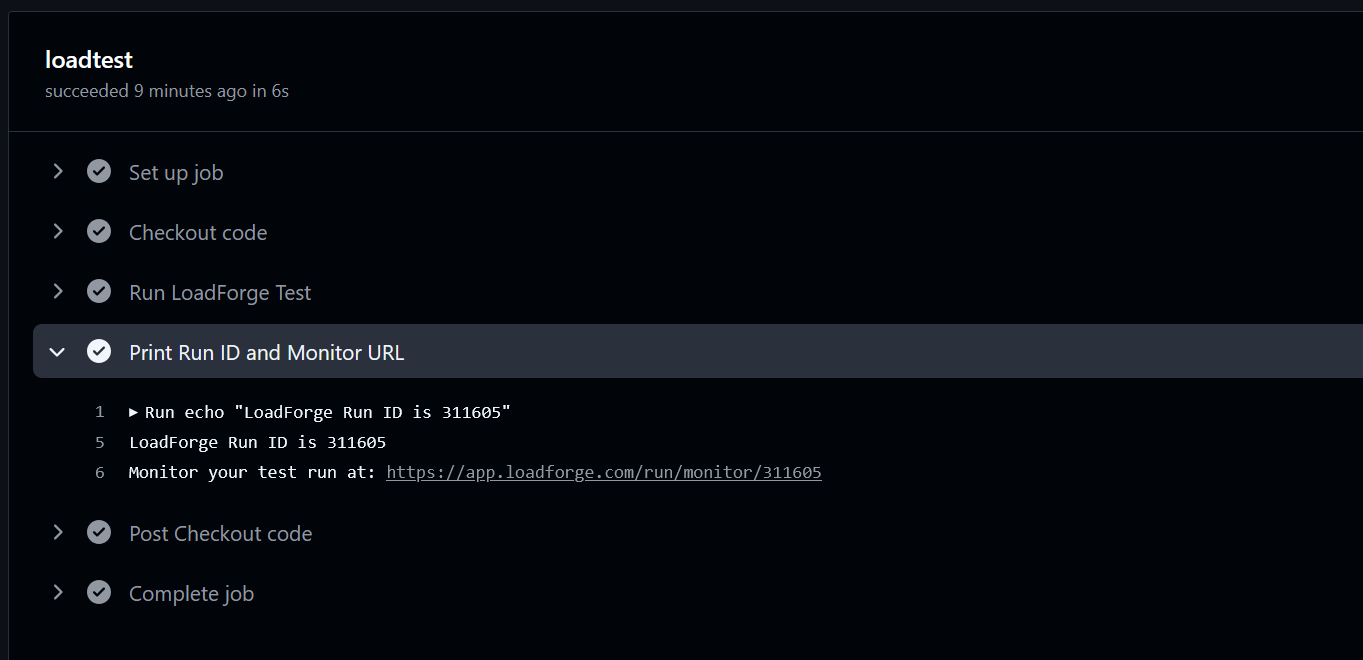
LoadForge GitHub Integration
Performance testing just got a major upgrade. LoadForge is thrilled to announce a seamless GitHub integration that lets you launch...
Caching is a crucial technique in the development of high-performance web applications. It involves storing copies of files or results of expensive computations temporarily in fast access data storage layers, which helps in reducing the time taken to serve content...
Caching is a crucial technique in the development of high-performance web applications. It involves storing copies of files or results of expensive computations temporarily in fast access data storage layers, which helps in reducing the time taken to serve content to the end-users, hence dramatically improving the performance of a website.
When it comes to web development with Laravel, caching can play a transformative role. Laravel, a popular PHP framework known for its elegant syntax and rich features, integrates seamlessly with various caching backends like Redis, Memcached, and even the file system itself. Utilizing caching in Laravel not only accelerates response time but also decreases the load on the web servers and databases.
Laravel provides an expressive, unified API for various caching backends. The default setup is file-based caching; however, Laravel supports several different cache drivers out of the box:
Here’s a simple example to demonstrate caching in Laravel:
<?php
Route::get('/profile', function () {
$user = Cache::remember('user-profile', 60, function () {
return DB::table('users')->find(Auth::user()->id);
});
return view('profile', ['user' => $user]);
});
In the above example, Laravel attempts to retrieve the user-profile
from the cache. If it doesn't exist, it runs the closure passed to the remember
method, retrieves the user from the database, caches it for 60 minutes, and then returns it.
In the next sections, we will delve deeper into how Redis can be configured and utilized in a Laravel environment to effectively handle caching scenarios, along with exploring various caching strategies and their benefits for your Laravel applications.
Redis, standing for Remote Dictionary Server, is an open-source in-memory data structure store that can serve a variety of purposes: it can be used as a database, a caching layer, and a message broker. Its versatility, combined with high performance, makes it a particularly effective tool when integrated with web frameworks like Laravel. Below, we delve into what makes Redis uniquely advantageous for Laravel applications.
Redis operates by storing data in memory, as opposed to disk-based storage, which leads to extremely fast data access times. This is crucial for web applications where response time is critical. In a Laravel context, this means faster retrieval of data for users, which directly contributes to a more responsive application experience.
Unlike simple key-value caches, Redis supports advanced data structures such as strings, lists, sets, hashes, and sorted sets with range queries. This variety allows developers to choose the most appropriate data structure for their specific needs, optimizing both storage and performance. For instance, Laravel can utilize these structures to implement complex caching strategies easily.
Despite being primarily in-memory, Redis provides options for durability via snapshotting and append-only files (AOF). This means that Laravel applications can leverage Redis not just as a cache but as a reliable store without the fear of data loss during unexpected failures.
Redis supports seamless scalability options, which are invaluable for growing Laravel applications. It can run in a master-slave replication setup or as a part of a Redis Cluster configuration to distribute data across multiple nodes. This setup is essential for building high-availability systems that can serve thousands of concurrent users.
Redis facilitates the publishing of messages and subscription to channels, providing a powerful mechanism for inter-process communication. This is particularly useful in Laravel when you need to decouple components and implement asynchronous processes or real-time messaging systems like notifications.
Laravel supports Redis directly through its unified API, which abstracts the complexity of directly dealing with the Redis client. Configuration is straightforward, typically requiring minimal set-up in config/database.php
to define Redis as a cache and session driver:
'redis' => [
'client' => 'predis',
'default' => [
'host' => env('REDIS_HOST', '127.0.0.1'),
'password' => env('REDIS_PASSWORD', null),
'port' => env('REDIS_PORT', 6379),
'database' => 0,
],
],
Furthermore, Laravel’s cache, session, and queue APIs can utilize Redis to enhance performance through simple configuration changes without changing the usage patterns.
Laravel and Redis together can create an effective full-page caching solution. By caching the complete HTML output of a page in Redis, Laravel can serve pages directly from the cache on subsequent requests, dramatically reducing the need for database queries and templating processing.
Redis offers a highly performant, adaptable, and feature-rich solution for enhancing Laravel applications. Its ability to act as a quick data store and a robust caching solution enables Laravel developers to significantly improve the responsiveness and scalability of their applications. The combination of Laravel’s ease of use and Redis’s performance features provides a powerful toolset for building modern web applications.
This robust integration therefore not only simplifies development work but also enhances the overall user experience by speeding up data processing and retrieval operations. Redis, with its comprehensive feature set, proves to be an invaluable addition to any Laravel project aiming for high performance and scalability.
Setting up Redis in your Laravel environment can significantly boost your application's performance by reducing the load on your database and speeding up the response times. Here’s a comprehensive guide to installing and configuring Redis in Laravel.
Before integrating Redis into your Laravel project, ensure you have the following prerequisites installed:
Open your terminal and execute the following commands:
sudo apt update
sudo apt install redis-server
This installs Redis and starts the server automatically.
Using Homebrew, you can install Redis by running:
brew update
brew install redis
After installation, start Redis server with:
brew services start redis
After installation, it’s advisable to configure Redis to ensure it meets your application's requirements. Modify the configuration file, typically located at /etc/redis/redis.conf
on Linux, to adjust parameters such as memory allocation and data persistence.
Laravel utilizes the phpredis
PHP extension for Redis operations. Install it via PECL:
pecl install redis
After installing, ensure you add extension=redis.so
to your php.ini
file to enable the Redis extension.
predis/predis
package using Composer:composer require predis/predis
.env
file in your Laravel project to include Redis settings. Typical settings include:REDIS_HOST=127.0.0.1
REDIS_PASSWORD=null
REDIS_PORT=6379
config/database.php
in your Laravel application and configure Redis as the default cache driver:'cache' => [
'driver' => 'redis',
'connection' => 'default',
],
'redis' => [
'client' => 'predis',
'default' => [
'host' => env('REDIS_HOST', '127.0.0.1'),
'password' => env('REDIS_PASSWORD', null),
'port' => env('REDIS_PORT', 6379),
'database' => 0,
],
],
To ensure that Redis is properly set up with your Laravel app, you can use Tinker to test the connection:
php artisan tinker
>>> cache()->store('redis')->put('Laravel', 'Redis', 10);
>>> cache()->store('redis')->get('Laravel');
If it returns "Redis"
then you've successfully set up Redis caching with your Laravel application.
This step-by-step setup provides a solid foundation for Redis integration in Laravel, paving the way for enhanced application performance through efficient caching mechanisms. Keep this configuration in mind as you continue to optimize and scale your Laravel applications.
Integrating Redis with Laravel for caching purposes involves several key steps, from installing Redis to modifying Laravel's caching configuration. This section provides a detailed guide on how to set Redis as your Laravel application's default cache driver, enhancing your app’s performance.
Before integrating Redis with Laravel, you need to ensure that Redis is installed on your server. You can install Redis using your server's package manager.
For Ubuntu/Debian systems:
sudo apt-get update
sudo apt-get install redis-server
For CentOS/RHEL systems:
sudo yum install redis
sudo systemctl start redis.service
sudo systemctl enable redis.service
After installation, make sure Redis is running by executing:
redis-cli ping
If Redis is running correctly, you should see PONG
as a response.
You will need the Redis PHP extension to allow PHP to communicate with the Redis server. You can install it using pecl or composer:
pecl install redis
or if you are using Composer:
composer require predis/predis
Modify the database.php
configuration file located in your Laravel project’s config
folder. Add or update the Redis configuration array to suit your environment. An example configuration may look like this:
'redis' => [
'client' => env('REDIS_CLIENT', 'predis'),
'default' => [
'url' => env('REDIS_URL'),
'host' => env('REDIS_HOST', '127.0.0.1'),
'password' => env('REDIS_PASSWORD', null),
'port' => env('REDIS_PORT', 6379),
'database' => env('REDIS_DB', 0),
],
],
Make sure the .env
file in your Laravel root directory is updated with the correct Redis server settings:
REDIS_HOST=127.0.0.1
REDIS_PASSWORD=null
REDIS_PORT=6379
REDIS_CLIENT=predis
Edit the .env
file in your Laravel project to set Redis as the default cache driver:
CACHE_STORE=redis
This change tells Laravel to use Redis for all caching operations.
To test if Laravel is properly using Redis for caching, you can perform a simple cache operation. Open a route or controller and add the following code:
Route::get('/test-redis', function () {
Cache::put('testKey', 'This is a value stored in Redis', 10); // Store a value for 10 minutes
return Cache::get('testKey');
});
Visit /test-redis
in your web browser or through a tool like curl. If everything is configured correctly, you should see "This is a value stored in Redis" displayed.
By following these steps, you have successfully integrated Redis with your Laravel application for caching. This setup not only enhances performance but also scales efficiently, handling larger volumes of data and user requests more effectively. With Redis as your caching layer, your Laravel application is now better equipped to deliver high performance and responsive user experiences.
In the context of web application performance, caching is a crucial strategy to reduce load times and improve user experience. Laravel, paired with Redis, offers a potent combination for implementing various caching techniques. This section explores object caching, query result caching, and full-page caching, providing insights on how to effectively implement these strategies in a Laravel environment using Redis.
Object caching involves storing fully constructed objects in the cache so that the application can retrieve them without needing to rebuild them on subsequent requests. This is particularly useful for data that doesn't change often but requires a significant amount of resources to construct.
To implement object caching in Laravel using Redis, you can use the Cache
facade. Here’s a simple example of how you might cache a user object:
use Illuminate\Support\Facades\Cache;
$user = Cache::remember('user:'.$userId, 3600, function () use ($userId) {
return User::find($userId);
});
In this example, the remember
method is used to retrieve the user object from the cache or, if it does not exist, execute the closure, store the result in Redis under the key user:<userId>
, and set an expiration time of one hour (3600 seconds).
Query result caching stores the results of a database query in the cache. This can significantly reduce the response time for subsequent requests that query the same data.
Here’s how you can cache query results in Laravel:
$posts = Cache::remember('posts.index', 3600, function () {
return Post::where('published', true)->get();
});
This snippet caches the result of fetching all published posts. When multiple users access your application, they will be served from the cache rather than hitting the database each time.
Full-page caching refers to caching the entirety of a HTML response generated by Laravel. This is highly effective for improving performance, especially for static pages or pages where the content changes infrequently.
To implement full-page caching, you can use a middleware that checks if a cached response is available for the given URL and serves it directly, bypassing the need to process the route and controller logic. If the response is not cached, it processes the request and stores the response in Redis. Here’s a brief outline of how this can be done:
namespace App\Http\Middleware;
use Closure;
use Illuminate\Support\Facades\Cache;
use Illuminate\Http\Request;
class CachePage {
public function handle(Request $request, Closure $next)
{
$key = 'page:'.md5($request->url());
if (Cache::has($key)) {
return response(Cache::get($key));
}
$response = $next($request);
if ($response->getStatusCode() === 200) {
Cache::put($key, $response->getContent(), 3600);
}
return $response;
}
}
By leveraging Laravel's built-in cache mechanisms and Redis's performance capabilities, you can significantly enhance the responsiveness of your application. Object caching reduces rebuild time, query result caching decreases database load, and full-page caching serves pre-rendered content promptly. Combining these techniques wisely, based on the nature of your Laravel application and the dynamicity of its content, will provide optimal performance enhancements.
Ensuring that Redis operates efficiently within your Laravel application is crucial for maintaining optimal performance and providing a smooth user experience. Monitoring Redis performance and managing its configuration allows developers to identify potential bottlenecks or inefficiencies and take steps to mitigate them. This section outlines practical tips and tools for effectively monitoring and managing Redis performance in a Laravel environment.
Redis comes with built-in commands that help in monitoring its performance directly from the command interface. Here are a few essential commands:
INFO
: Displays information and statistics about the server.MONITOR
: Real-time listing of commands processed by the server.SLOWLOG
: Retrieves logs of commands that have a long execution time.Additionally, you can use tools like Redis-CLI and Redis-stat for more detailed analytics. Redis-CLI provides a command-line interface to interact with Redis, and Redis-stat is a visual tool that shows different metrics updated in real time.
For Laravel applications, the Laravel Telescope provides excellent support for monitoring Redis operations. It offers:
To integrate Redis monitoring in Laravel using Telescope, you can follow these steps:
Install Telescope via Composer:
composer require laravel/telescope
Publish the Telescope assets:
php artisan telescope:install
Access the Telescope dashboard at your application's /telescope
URL.
Optimizing cache hit rates involves ensuring that your cache entries are effectively utilized before they expire or are evicted. Here are some strategies to improve cache utilization:
Encountering issues with Redis in a Laravel application is not uncommon. Here are a few tips to troubleshoot common pitfalls:
config/database.php
matches the Redis server settings.Here's a snippet for setting up Redis connection correctly:
'redis' => [
'client' => env('REDIS_CLIENT', 'predis'),
'options' => [
'cluster' => env('REDIS_CLUSTER', 'redis'),
'prefix' => env('REDIS_PREFIX', Str::slug(env('APP_NAME', 'laravel'), '_').'_database_'),
],
'default' => [
'url' => env('REDIS_URL'),
'host' => env('REDIS_HOST', '127.0.0.1'),
'password' => env('REDIS_PASSWORD', null),
'port' => env('REDIS_PORT', '6379'),
'database' => env('REDIS_DB', '0'),
],
// Other connections...
],
Regularly reviewing and adjusting your Redis setup and usage is key. Scheduled performance audits can help catch inefficiencies early and adapt to changing application requirements.
By implementing these monitoring and management practices, you can significantly improve the performance and reliability of your Laravel application using Redis.
As Laravel applications grow, the demand on system resources and the need for efficient response times increase significantly. To manage scaling effectively, especially in high-traffic scenarios, utilizing Redis for session storage and caching can be crucial. This section delves into the methods for scaling Laravel applications horizontally by leveraging Redis, including potential architectures and scenarios that illustrate the effectiveness of Redis in a scaled environment.
Horizontal scaling involves adding more servers to your pool to handle increased load, as opposed to vertical scaling, which involves adding more power (CPU, RAM) to your existing machine. In the context of Laravel and Redis, horizontal scaling primarily focuses on how Redis can be used to share sessions and cache data across multiple application instances.
When scaling horizontally, session management becomes a challenge because user sessions need to be accessible across multiple server instances. Storing sessions in a centralized Redis instance can solve this:
Configuration: Set up Redis as your session driver in Laravel by changing the SESSION_DRIVER
setting in your .env
file:
SESSION_DRIVER=redis
Session Accessibility: Each instance of your Laravel application can access session data stored in Redis, ensuring session persistence irrespective of which server handles the request.
Using Redis as a cache database when scaling Laravel applications is equally important. This setup enhances performance by reducing the load on the database and speeding up response times by serving cached data from Redis.
Configure Cache Driver: Change the cache driver to Redis in the Laravel configuration file or the .env
file:
CACHE_STORE=redis
Implement Caching Strategy: Use Laravel's caching API to store frequently accessed data, such as query results or API responses, in Redis. Here’s a simple example:
Cache::remember('users', 60, function () {
return DB::table('users')->get();
});
When deploying a Laravel application that uses Redis for session storage and caching in a horizontally scaled environment, consider the following architectures:
Centralized Redis Instance: A single Redis server that all application instances connect to. This approach is simple to implement but can become a bottleneck if not properly configured or if it lacks sufficient resources.
Redis Cluster: A cluster of Redis nodes that offers high availability and partitioning, thus distributing the load and increasing fault tolerance.
Hybrid Solutions: Combining Redis with other technologies like database replication or additional caching layers (e.g., Varnish) for more comprehensive scalability and performance solutions.
Imagine a Laravel e-commerce application experiencing slow response times and frequent database timeouts during peak hours. By implementing Redis for session management and full-page caching, the application can handle more user requests and process transactions faster. The sessions are stored in Redis, allowing user data to be quickly retrieved across multiple servers. Additionally, product pages are cached in Redis, reducing the need to fetch data from the database repeatedly.
Leveraging Redis for session storage and caching in Laravel applications is a proven strategy for scaling horizontally. It not only enhances performance by distributing the load across multiple servers but also maintains data consistency and availability. As traffic grows, consider expanding your Redis implementation by exploring clusters and hybrid solutions to further optimize your Laravel application’s scalability and user experience.
Caching is a critical component in enhancing the performance of web applications. When it comes to Laravel applications, using Redis as a caching backend not only accelerates the response time but also increases the efficiency of data retrieval processes. Below, we discuss several best practices to elevate and maintain the effectiveness of Redis when implemented in a Laravel environment.
Properly configuring Redis is essential for optimizing performance:
Use Persistent Connections: To minimize the connection overhead, adjust Laravel’s Redis configuration to use persistent connections. This can be done by setting the persistent
option in the config/database.php
:
'redis' => [
'client' => 'predis',
'options' => [
'cluster' => 'redis',
'persistent' => true,
],
...
]
Configure Timeouts and Limits: Ensure that timeout settings and memory limits are configured according to your application’s needs to avoid data loss and ensure availability.
When storing data in Redis, the way data is serialized can impact performance. Laravel provides different serialization options that you can configure:
Setting appropriate cache expiration times is crucial for effectively managing the cache storage and ensuring that the data stays up-to-date:
Dynamic Expiry Setting: Use various TTLs (Time To Live) for different types of data depending on how often they change. This can be configured easily in Laravel when setting a cache value:
Cache::put('key', 'value', now()->addMinutes(10));
For better management and invalidation of cache data, use tagging:
Cache Tagging: Redis supports tagging, which can help with grouping related items that can be cleared together when any one of them gets updated.
Cache::tags(['people', 'artists'])->put('John', $john, $minutes);
Cache::tags(['people', 'artists'])->flush();
Securing your Redis instance is critical to prevent unauthorized access:
Authentication and Access Controls: Make sure to enable authentication by setting a strong password in the Redis configuration and use firewalls to restrict access to the Redis server.
Use Encrypted Connections: Where possible, use SSL/TLS to encrypt data in transit between your Laravel application and Redis server.
Keep track of how Redis performs with your Laravel application:
Consider scaling Redis horizontally as your application grows:
Laravel provides Artisan commands that can be handy for managing cache:
Clearing Cache: You can easily clear the Redis cache using Artisan commands which can be crucial during deployments or when making significant updates to your application.
php artisan cache:clear
Implementing these best practices will help ensure that your Laravel application leverages Redis caching effectively, enhancing both performance and scalability. By maintaining security, managing configurations properly, and monitoring system performance, you can optimize the benefits that Redis has to offer in a Laravel setting.
In this section, we delve into real-world case studies where Redis caching has been strategically implemented in Laravel projects. These examples highlight the transformative effects that efficient caching can have on application performance and scalability, offering valuable insights and lessons learned.
Overview:
A mid-sized e-commerce company faced challenges with their Laravel application during peak traffic times, experiencing slow page loads and transaction times which affected customer satisfaction and sales. The implementation of Redis for caching dramatically improved response times and user experience.
Challenge:
Handling high traffic and database load during sales and promotional events, leading to slow page response times.
Solution:
The team integrated Redis to handle session storage and cache database queries. Product data, which remains relatively static, was cached using Redis, reducing the need to fetch data from the primary database on every request.
Implementation:
Configured Laravel to use Redis as the default cache driver:
'default' => env('CACHE_STORE', 'redis'),
Cached frequent database queries:
$products = Cache::remember('products', 60*60, function () {
return Product::all();
});
Utilized Redis for session storage to maintain user sessions across multiple servers in the cloud environment.
Results:
Lessons Learned:
Caching static and semi-static data significantly reduces the load on the database and should be a primary step in optimizing high-traffic applications.
Overview:
A Software as a Service (SaaS) provider utilized Laravel to serve API requests to their clients. They faced issues with API throttling and scalability which were efficiently resolved by implementing Redis.
Challenge:
Managing a high volume of API requests without compromising on the performance and reliability of the service.
Solution: The implementation of Redis allowed for efficient rate limiting, using its increment and expire functionalities to handle API request limits.
Implementation:
Route::middleware('throttle:60,1')->group(function () {
Route::get('/api/user', function () {
// API code here
});
});
Results:
Lessons Learned:
Redis can be an effective tool not just for caching but also for system functionalities such as rate limiting that require quick data access and modification.
Overview:
A financial services application leveraging Laravel needed to process and display real-time data to users, requiring quick update cycles and immediate data availability.
Challenge:
Ensuring that data updates are processed and reflected in real-time on user interfaces without introducing significant latencies.
Solution:
The development team used Redis’ pub/sub messaging system to handle real-time data broadcast, which allowed them to process and push updates instantly to client interfaces.
Implementation:
Redis::publish('updates', json_encode($updateData));
Results:
Lessons Learned:
For applications requiring real-time updates, Redis pub/sub provides an efficient solution for quick data broadcasting across client applications.
Conclusion:
These case studies showcase just a few scenarios where Redis caching in Laravel applications has resulted in significant performance gains and enhanced scalability. The flexibility and speed of Redis make it an invaluable tool in the optimization of Laravel applications, proving that with the right implementation strategies, Redis can tremendously boost application responsiveness and efficiency.
As we have explored in this guide, leveraging Redis for caching in Laravel applications can significantly enhance performance by reducing load times and server strain. Starting with a fundamental understanding of how caching operates within Laravel, we delved into the versatile capabilities of Redis—an advanced in-memory data structure store that serves as an efficient database, cache, and message broker.
We progressed into practical implementations, illustrating how to set up Redis in a Laravel environment and seamlessly integrate it as the default caching backend. Through various caching techniques such as object caching, query result caching, and full-page caching, we demonstrated how to optimize response times and overall user experience.
Monitoring and managing Redis within Laravel ensures that applications remain fast and reliable. Utilizing tools to track performance metrics and troubleshooting common issues are essential for maintaining optimal functionality.
Regarding scalability, Redis offers robust solutions for expanding Laravel applications. By handling sessions and cache databases efficiently, Redis facilitates horizontal scaling, allowing applications to grow and accommodate increasing user demands without sacrificing performance.
Security: Always secure Redis instances with authentication and, if possible, TLS, especially when accessible over public networks.
Monthly diagnostics and updates should be carried out diligently to avoid vulnerabilities.
Maintenance: Regularly update Redis and Laravel packages to harness improvements and security patches.
Use observability tools to continuously monitor Redis performance metrics.
Configuration: Tune Redis settings according to the specific workload and data access patterns of your Laravel application.
Employ appropriate eviction policies based on your application's caching strategy.
The future of caching technologies, particularly with Redis, appears promising as continuous improvements and features are being developed. This evolution will likely offer even more sophisticated mechanisms for data handling, persistence, and replication, which can be seamlessly integrated into Laravel frameworks.
Emerging technologies such as AI and machine learning could also play a crucial role in automating and optimizing caching layers, predicting data access patterns, and preloading frequently accessed data into the cache.
Furthermore, as cloud-native architectures and microservices continue to rise in popularity, Redis's role in distributed caching, session storage, and inter-service communication will become increasingly crucial. Its ability to synchronize data across different components of an application in real-time enhances both the flexibility and scalability of Laravel applications.
In conclusion, by effectively leveraging Redis as part of your Laravel application's caching strategy, you can achieve significant improvements in performance while preparing your application’s architecture for future scalability and technological advancements. As caching technologies evolve, staying informed and adaptable will ensure that your applications remain robust, responsive, and ahead of the curve.