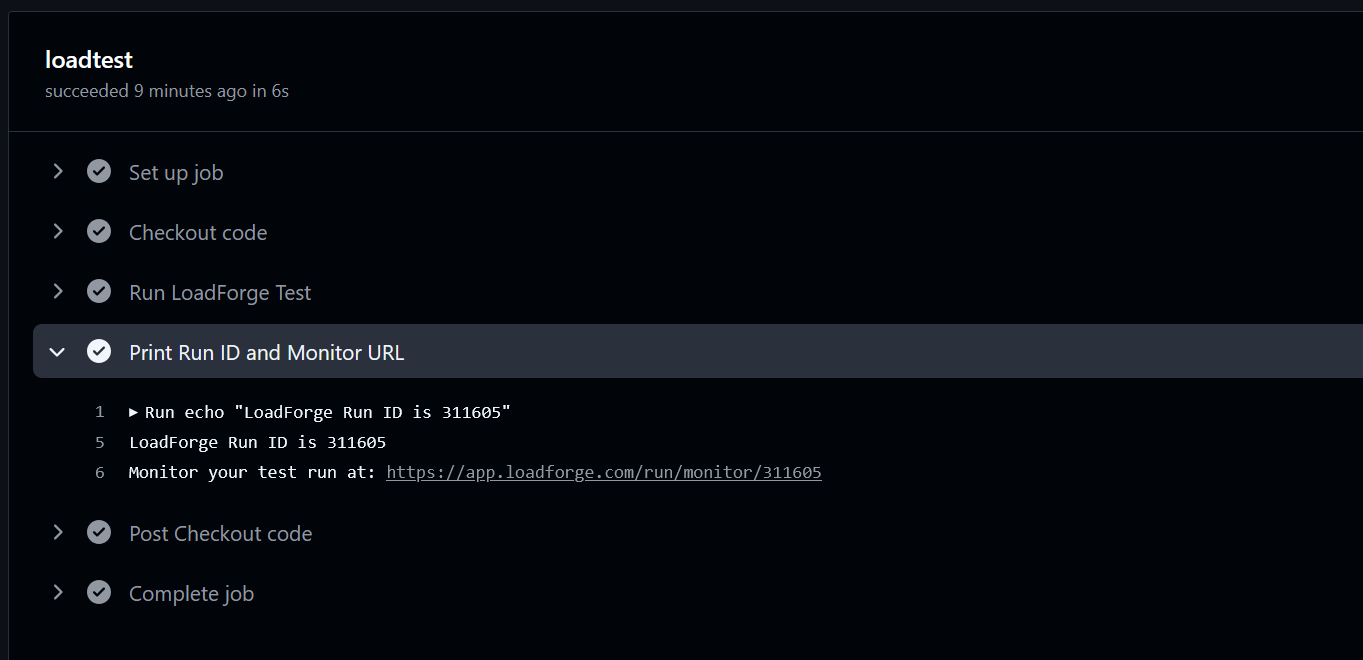
LoadForge GitHub Integration
Performance testing just got a major upgrade. LoadForge is thrilled to announce a seamless GitHub integration that lets you launch...
In the fast-paced digital landscape, user experience and application responsiveness play crucial roles in the success of any web application. Symfony, one of the leading PHP frameworks, is highly regarded for its robustness and ease of use. However, to fully...
In the fast-paced digital landscape, user experience and application responsiveness play crucial roles in the success of any web application. Symfony, one of the leading PHP frameworks, is highly regarded for its robustness and ease of use. However, to fully leverage the power of Symfony, it's essential to pay attention to the performance of its components—chief among them being the database queries managed by Doctrine ORM (Object-Relational Mapping).
Doctrine ORM is a powerful tool that simplifies database interactions by allowing developers to work with PHP objects rather than writing raw SQL queries. While Doctrine significantly enhances productivity and code clarity, it also introduces potential performance bottlenecks if not optimized correctly. Inefficient Doctrine queries can lead to long page load times, high server resource consumption, and ultimately a poor user experience.
Optimizing Doctrine queries is essential for several reasons:
In this guide, we will dive deep into the intricacies of optimizing Doctrine queries in a Symfony application. We will explore best practices, tips, and tricks to enhance query performance, from profiling and analyzing your queries to implementing caching strategies and understanding the nuances of lazy versus eager loading. Additionally, we will cover techniques for batch processing, bulk inserts, and efficient pagination to ensure your database operations are as performant as possible.
Stay tuned as we uncover the path to a high-performance Symfony application, starting with an understanding of Doctrine ORM and progressing through practical optimization techniques. By the end of this guide, you'll be well-equipped to optimize your Symfony applications, ensuring they are both fast and scalable, ultimately delivering an exceptional user experience.
Doctrine ORM (Object-Relational Mapping) is a powerful library that facilitates database interactions in Symfony applications by mapping object-oriented PHP code to relational database tables. Its purpose is to streamline and simplify the database access layer, enabling developers to work with databases in an abstracted and efficient manner. Here, we delve into the core concepts of Doctrine ORM, its benefits, and how it integrates seamlessly with Symfony.
Doctrine ORM is designed to bridge the gap between object-oriented programming and relational databases. By employing a mapping layer, it allows developers to interact with their database using PHP objects, hiding the complexities of SQL syntax. The primary benefits of using Doctrine ORM include:
To understand how Doctrine ORM operates within a Symfony application, it’s crucial to grasp the following core concepts:
Symfony natively supports Doctrine ORM, making the integration process straightforward. Below are the steps to integrate Doctrine ORM into a Symfony project.
composer require symfony/orm-pack
.env
file.
DB_URL="mysql://db_user:[email protected]:3306/db_name" 3. Creating an Entity: Define a new entity using the Symfony console and annotate it with Doctrine ORM mappings.
php bin/console make:entity
This command will prompt for entity name and fields. An example entity might look like:
namespace App\Entity;
use Doctrine\ORM\Mapping as ORM;
/**
* @ORM\Entity(repositoryClass="App\Repository\ProductRepository")
*/
class Product
{
/**
* @ORM\Id
* @ORM\GeneratedValue
* @ORM\Column(type="integer")
*/
private $id;
/**
* @ORM\Column(type="string", length=100)
*/
private $name;
/**
* @ORM\Column(type="decimal", scale=2)
*/
private $price;
// Getter and Setter methods...
}
</code></pre>
- Generating Database Schema: Use Doctrine commands to automatically generate or update the database schema based on your entity definitions.
php bin/console doctrine:schema:update --force
$productRepository = $entityManager->getRepository(Product::class); $product = $productRepository->find($productId);
By understanding and leveraging Doctrine ORM, Symfony developers can achieve more productive, maintainable, and scalable application development. The integration is designed to be seamless, allowing you to focus on building business logic rather than wrestling with database intricacies. In the following sections, we will explore advanced techniques to further optimize Doctrine queries for peak performance.
Optimizing your Symfony application's performance requires a deep understanding of how your Doctrine queries interact with the database. Profiling and analyzing these queries can help you identify bottlenecks and inefficiencies. This section will guide you through the steps to profile Doctrine queries using Symfony Web Profiler, Doctrine Debug Toolbar, and LoadForge for load testing.
Symfony Web Profiler is an indispensable tool for understanding the performance aspects of your Symfony application, including Doctrine queries.
Enable the Web Profiler Bundle:
Make sure the Web Profiler bundle is enabled in your development environment. By default, Symfony includes this bundle in the config_dev.yaml
file.
Accessing the Web Profiler: After running your Symfony application in the browser, click on the "Web Profiler" link at the bottom of the page. This will take you to a detailed dashboard.
Doctrine Query Panel: Navigate to the "Doctrine" panel in the Web Profiler. Here, you will find detailed information on executed queries, including execution times, types, and parameters.
The Doctrine Debug Toolbar is another powerful tool for real-time query profiling directly in your browser.
Install the Debug Toolbar:
Add the doctrine/doctrine-bundle
and symfony/debug-pack
to your composer.json
.
composer require --dev doctrine/doctrine-bundle symfony/debug-pack
Enable the Debug Toolbar in config_dev.yaml
:
Ensure the debug toolbar is enabled in your development configuration.
# config/packages/dev/web_profiler.yaml
web_profiler:
toolbar: true
intercept_redirects: false
Inspect Queries: You will now see a debug toolbar at the bottom of your browser window, displaying a "doctrine" icon. Click this icon to reveal a detailed list of all executed queries, including execution time and memory usage. This is crucial for identifying long-running or unnecessary queries.
Understanding how your queries perform under load can help you anticipate performance issues before they impact your users. LoadForge offers the necessary tools to simulate high-traffic conditions and analyze your application's performance.
Sign Up and Set Up Your Project: Create an account on LoadForge and set up your Symfony project within the platform.
Create a Load Test Scenario: Define a scenario that mimics your application's typical usage pattern, including the routes and endpoints that trigger Doctrine queries.
Run the Load Test: Execute the load test to simulate high traffic. LoadForge will provide real-time analytics and identify potential bottlenecks within your Doctrine queries.
Analyze Results: After the test, LoadForge offers comprehensive reports that highlight query performance issues, allowing you to take targeted optimization actions.
{
"testId": "1234",
"result": {
"totalRequests": 1000,
"failedRequests": 50,
"averageResponseTime": "300ms",
"queries": [
{
"query": "SELECT * FROM users WHERE id = ?",
"averageExecutionTime": "150ms"
},
{
"query": "UPDATE posts SET views = views + 1 WHERE id = ?",
"averageExecutionTime": "200ms"
}
]
}
}
By leveraging the Symfony Web Profiler, Doctrine Debug Toolbar, and LoadForge, you can systematically profile, analyze, and optimize your Doctrine queries to ensure your Symfony application performs at its best, even under demanding conditions.
Doctrine Query Language (DQL) is a powerful and flexible part of the Doctrine ORM, which allows developers to write database queries in an object-oriented way. This section focuses on best practices for writing efficient and optimized DQL, avoiding N+1 query issues, and leveraging joins and fetches to enhance the performance of your Symfony application.
Limit Your Query Scope: Always retrieve only the needed data. Avoid selecting more fields than necessary to minimize data transfer and processing overhead.
// Inefficient Query
$query = $entityManager->createQuery('SELECT u FROM App\Entity\User u');
// Efficient Query
$query = $entityManager->createQuery('SELECT u.username, u.email FROM App\Entity\User u');
Use Indexes for Frequent Searches: Ensure that the fields used frequently in WHERE clauses are indexed. This speeds up search operations.
Avoid Using $resultSetMapping
if Possible: For tightly controlled and frequently used queries, prefer DQL over raw SQL to benefit from the Doctrine ORM’s caching and optimization features.
Leverage Named Queries: Define frequently used queries as named queries to improve readability and reusability.
use Doctrine\ORM\Mapping as ORM;
/**
* @ORM\Entity
* @ORM\NamedQuery(name="findActiveUsers", query="SELECT u FROM App\Entity\User u WHERE u.active = 1")
*/
class User
{
// ...
}
N+1 query issues occur when an application needs to load related entities, resulting in one query to load the primary entity and N additional queries to load each related entity. To avoid this:
Use JOINs in DQL: Join related entities within the query to reduce the total number of queries.
// Inefficient: Causes N+1 issue
$users = $entityManager->getRepository(User::class)->findAll();
foreach ($users as $user) {
echo $user->getProfile()->getBio();
}
// Efficient: Solving N+1 issue with JOIN
$query = $entityManager->createQuery(
'SELECT u, p FROM App\Entity\User u JOIN u.profile p'
);
$users = $query->getResult();
foreach ($users as $user) {
echo $user->getProfile()->getBio();
}
Use Fetch Joins: Eagerly fetch related entities using fetch joins to minimize the number of queries executed.
$query = $entityManager->createQuery(
'SELECT u, p FROM App\Entity\User u JOIN FETCH u.profile p'
);
$users = $query->getResult();
Properly leveraging joins and fetches not only avoids N+1 issues but also enhances query performance as it minimizes unnecessary database round-trips.
INNER JOIN vs LEFT JOIN: Use INNER JOIN when you are sure that related entities will exist, as it is generally more performant than LEFT JOIN, which accounts for NULL values.
Conditional Fetching: Fetch only necessary related entities conditionally to keep the resource usage optimized.
$query = $entityManager->createQuery(
'SELECT u, p FROM App\Entity\User u LEFT JOIN FETCH u.profile p WHERE u.status = :status'
);
$query->setParameter('status', 'active');
$users = $query->getResult();
Transforming Data Efficiently: Use partial objects or array results when you do not require full entity objects, reducing memory usage and overhead.
$query = $entityManager->createQuery(
'SELECT PARTIAL u.{id, username}, PARTIAL p.{bio} FROM App\Entity\User u JOIN u.profile p'
);
$result = $query->getArrayResult();
Optimization of Doctrine queries is crucial for maintaining a high-performance Symfony application. By following the best practices outlined above, using JOINs effectively, and avoiding N+1 query issues through fetch strategies, developers can ensure efficient data retrieval and improved application performance. Properly optimized DQL not only meets performance expectations but also enhances the scalability and user experience of Symfony applications.
Query caching is an essential optimization technique for enhancing the performance of your Symfony application when using Doctrine ORM. Properly implemented, it can drastically reduce the load on your database by reusing the results of frequent and repetitive queries. In this section, we will delve into how query caching works in Doctrine, its benefits, and provide a detailed guide on implementing it in your Symfony project.
Doctrine ORM supports various types of caching mechanisms, including:
For performance improvements, the Query Cache and Result Cache are particularly relevant. The Query Cache stores the SQL generated from DQL, allowing repeated queries to be handled faster. The Result Cache stores the results of database queries, enabling instant retrieval of data without hitting the database.
Integrating query caching in your Symfony project involves configuring your cache provider and modifying your query code to leverage the cache. Here's a step-by-step guide to get you started.
Doctrine supports multiple cache providers like Redis
, Memcached
, and APCu
. For simplicity, we'll use the APCu
cache in this example.
Add the APCu
extension to your Symfony project:
composer require symfony/cache
Configure the cache in your config/packages/doctrine.yaml
file:
doctrine:
orm:
metadata_cache_driver:
type: service
id: doctrine.system_cache_provider
query_cache_driver:
type: service
id: doctrine.system_cache_provider
result_cache_driver:
type: service
id: doctrine.result_cache_provider
services:
doctrine.system_cache_provider:
class: Symfony\Component\Cache\Adapter\ApcuAdapter
doctrine.result_cache_provider:
class: Symfony\Component\Cache\Adapter\ApcuAdapter
With the cache provider configured, you can now cache your query results. Use the useResultCache
method in your Doctrine query builder.
// src/Repository/ProductRepository.php
use Doctrine\ORM\EntityRepository;
use Doctrine\Common\Cache\ApcuCache;
class ProductRepository extends EntityRepository
{
public function findAllProducts()
{
return $this->createQueryBuilder('p')
->select('p')
->getQuery()
->useResultCache(true, 3600) // Enable result cache, cache for 1 hour
->getResult();
}
}
In this example:
useResultCache(true, 3600)
enables the result cache for this query and sets a TTL (time-to-live) of 3600 seconds (1 hour).By effectively implementing query caching, you can significantly enhance the performance of your Symfony application with Doctrine ORM, making your application more responsive and scalable.
In the context of Doctrine ORM and Symfony applications, understanding the difference between lazy loading and eager loading is crucial to optimizing query performance. Each strategy has its advantages and trade-offs, and knowing when to use each can significantly impact the responsiveness of your application.
Lazy loading is a design pattern used to defer the initialization of an object until the point at which it is needed. In Doctrine, this means associated entities are fetched from the database only when their properties are accessed.
By default, Doctrine uses lazy loading for associations unless explicitly instructed otherwise. Here’s an example of how lazy loading works:
/**
* @Entity
*/
class Product
{
/**
* @OneToMany(targetEntity="Review", mappedBy="product", fetch="LAZY")
*/
private $reviews;
}
In this example, the reviews
association for a Product
entity is lazy-loaded. The associated Review
entities are not retrieved from the database until the reviews
property is accessed.
Eager loading is the opposite of lazy loading; it fetches the associated entities at the same time as the main entity, typically through joins.
You can define eager loading with the fetch="EAGER"
attribute or via Doctrine Query Language (DQL):
/**
* @Entity
*/
class Product
{
/**
* @OneToMany(targetEntity="Review", mappedBy="product", fetch="EAGER")
*/
private $reviews;
}
Or, using DQL for fine-grained control:
$query = $entityManager->createQuery(
'SELECT p, r FROM App\Entity\Product p
JOIN p.reviews r'
);
$products = $query->getResult();
To optimize performance, decide between lazy and eager loading based on the specific needs of your application:
By wisely choosing between lazy and eager loading, you can strike a balance that keeps your application both responsive and efficient.
Knowing when to use lazy versus eager loading will help ensure that your Symfony application performs optimally under various scenarios. Combined with the other optimization strategies discussed in this guide, you can significantly enhance the efficiency and speed of your Doctrine queries.
Handling large volumes of data efficiently is a crucial aspect of optimizing Doctrine queries in your Symfony application. Batch processing and bulk inserts are techniques designed to minimize the performance overhead associated with multiple database operations. Properly implementing these strategies can significantly enhance the performance and responsiveness of your system.
Executing numerous individual database operations can lead to excessive transaction overhead, increased load times, and can slow down your application considerably. By grouping these operations into batches, you can:
Doctrine provides a straightforward mechanism to handle batch processing. The primary approach involves persisting and flushing entities in chunks. Below is a step-by-step guide to implementing batch processing effectively:
Define the batch size: Determine the optimal batch size based on your application's requirements and database capabilities.
Iterate over your data: Loop through your dataset and persist entities in batches.
Flush and clear the EntityManager: After each batch, flush the changes to the database and clear the EntityManager to free up memory.
Here’s a sample implementation in Symfony:
// Sample code for batch processing
$batchSize = 20;
for ($i = 1; $i <= 1000; ++$i) {
$user = new User();
$user->setName('User '.$i);
$entityManager->persist($user);
if (($i % $batchSize) === 0) {
$entityManager->flush();
$entityManager->clear();
}
}
$entityManager->flush(); // Flush the remaining objects
$entityManager->clear(); // Clear the EntityManager
Bulk inserts can further enhance performance by reducing the overhead introduced by repeated insert operations. This is especially useful when dealing with large datasets.
The DoctrineBatchUtils library provides tools to perform efficient bulk inserts. Here’s how you can incorporate it into your Symfony application:
Install the DoctrineBatchUtils package:
composer require doctrine/doctrine-batch-utils
Use the package to perform bulk inserts:
// Sample code for bulk inserts using Doctrine BatchUtils use DoctrineBatchUtils\BatchProcessing\SimpleBatchProcessor;
// Create a batch processor $processor = new SimpleBatchProcessor($entityManager);
for ($i = 1; $i <= 1000; ++$i) { $user = new User(); $user->setName('User '.$i);
$processor->persist($user);
if (($i % $batchSize) === 0) {
$processor->flushAndClear();
}
} $processor->flushAndClear(); // Flush and clear the remaining objects
By implementing efficient batch processing and bulk inserts, you can significantly reduce the overhead on your database operations and improve the overall performance of your Symfony application.
Pagination is a crucial feature in web applications, particularly those that handle large datasets. Efficiently implementing pagination can significantly improve the performance of your Symfony application by reducing the amount of data processed and transferred in each request. Below are some tips and tricks to help you achieve efficient pagination in your Doctrine queries.
The fundamental approach to pagination in Doctrine is using the setFirstResult()
and setMaxResults()
methods. These methods allow you to specify an offset (the starting point of your query) and a limit (the maximum number of results to return).
For example:
public function getPaginatedResults(int $page, int $pageSize)
{
$query = $this->createQueryBuilder('e')
->setFirstResult(($page - 1) * $pageSize)
->setMaxResults($pageSize)
->getQuery();
return $query->getResult();
}
In this code snippet:
setFirstResult(($page - 1) * $pageSize)
sets the starting point of the query.setMaxResults($pageSize)
limits the number of results returned by the query.Counting the total number of records can be expensive, especially for large datasets. If the total count isn't strictly necessary, consider omitting it or using an estimated count.
If you must perform a count:
$totalItems = $this->createQueryBuilder('e')
->select('COUNT(e.id)')
->getQuery()
->getSingleScalarResult();
Ensure that your database tables have appropriate indexes, especially on columns used in the ORDER BY
clause and any WHERE
conditions. This will greatly improve the efficiency of your paginated queries by reducing the amount of data the database needs to scan.
Ordering paginated results is another critical aspect of performance. Always prefer indexed columns for ordering. Avoid ordering by columns with high cardinality such as text fields.
If you're fetching related entities, consider using joins and fetch joins to reduce the number of separate queries executed. However, be cautious with fetch joins as they can lead to huge result sets if not controlled properly.
For instance:
public function getPaginatedResultsWithAssociations(int $page, int $pageSize)
{
$query = $this->createQueryBuilder('e')
->leftJoin('e.relatedEntity', 'r')
->addSelect('r')
->setFirstResult(($page - 1) * $pageSize)
->setMaxResults($pageSize)
->getQuery();
return $query->getResult();
}
Cursor-based pagination can be more efficient than offset-based pagination for large datasets. Instead of using limit and offset, you pass a "cursor," typically the ID of the last item on the previous page.
Example:
public function getCursorBasedPaginatedResults(int $lastItemId, int $pageSize)
{
$query = $this->createQueryBuilder('e')
->where('e.id > :lastItemId')
->setParameter('lastItemId', $lastItemId)
->setMaxResults($pageSize)
->getQuery();
return $query->getResult();
}
Implementing efficient pagination in Doctrine helps significantly in handling large datasets. Combining the strategies of limit and offset, effective indexing, efficient ordering, and considering cursor-based pagination can lead to noticeable performance improvements in your Symfony application. Whether you're displaying a list of items on a webpage or providing data for an API, understanding and applying these techniques will help you ensure that your application remains performant and scalable.
Optimizing your database design and indexing strategy is essential for achieving efficient query execution in your Symfony application. Proper indexing can drastically reduce query execution time, while a well-thought-out database design ensures scalability and maintainability. In this section, we will explore guidelines and best practices for indexing and designing your database to support optimal performance with Doctrine.
Indexes are special lookup tables that the database search engine can use to speed up data retrieval. Without an index, the database must scan the entire table to find the relevant rows, leading to slower queries as the table grows.
Identify Frequently Queried Columns: Begin by identifying columns that are frequently used in WHERE
clauses, join conditions, and sorting operations. These columns are prime candidates for indexing.
Use Composite Indexes: When queries often filter using multiple columns, consider creating composite indexes. Ensure the order of columns in the composite index aligns with the order in which they are used in queries.
Avoid Over-Indexing: While indexes improve read performance, they can degrade write performance. Each index needs to be updated on insert, update, and delete operations. Strike a balance by indexing only those columns that significantly benefit query performance.
Use Unique Indexes: If a column should contain unique values, leverage unique indexes. This not only improves search performance but also enforces data integrity.
Monitor and Refine Indexes: Use database tools to monitor index usage and adjust your indexing strategy as query patterns evolve.
Doctrine allows you to define indexes directly within your entity annotations or YAML/XML mappings. Here is an example of how to define indexes using annotations:
/**
* @Entity
* @Table(name="users", indexes={
* @Index(name="search_idx", columns={"email", "username"})
* })
*/
class User
{
/**
* @Id
* @GeneratedValue(strategy="AUTO")
* @Column(type="integer")
*/
private $id;
/**
* @Column(type="string", length=255)
*/
private $email;
/**
* @Column(type="string", length=255)
*/
private $username;
// Other fields and methods...
}
Normalization: Normalize your database to avoid data redundancy. However, balance normalization with performance needs. In some cases, denormalization might be necessary to optimize read-heavy operations.
Use Appropriate Data Types: Choose the smallest data type that can adequately store your data. This minimizes storage requirements and improves performance. For example, use TINYINT
instead of INT
if the number range allows it.
Foreign Keys: Define foreign keys for relationships to ensure data integrity. While foreign keys add overhead on write operations, they are crucial for maintaining consistent and reliable data.
Partitioning: If working with large datasets, consider partitioning your tables. Partitioning helps in breaking down large tables into more manageable pieces, speeding up query execution.
Database Schema Migrations: Use Doctrine Migrations to manage schema changes effectively. This helps in maintaining consistent database schemas across different environments.
Consider a blogging platform with a normalized design for users, posts, and comments:
users
+----+---------+
| id | name |
+----+---------+
| 1 | Alice |
| 2 | Bob |
+----+---------+
posts
+----+---------+---------+
| id | user_id | title |
+----+---------+---------+
| 1 | 1 | Post A |
| 2 | 2 | Post B |
+----+---------+---------+
comments
+----+---------+---------+
| id | post_id | text |
+----+---------+---------+
| 1 | 1 | Nice! |
| 2 | 2 | Great! |
+----+---------+---------+
In this design:
user_id
and post_id
columns are foreign keys, ensuring referential integrity.By following these indexing and database design guidelines, you can significantly enhance the performance of your Symfony application using Doctrine. Remember to regularly profile and test your queries to adapt your strategies as your application's needs evolve.
Load testing is a crucial step in the optimization journey of any Symfony application. By simulating real-world usage scenarios and applying stress to your application, you can identify performance bottlenecks and validate the impact of your Doctrine query optimizations. In this section, we will explore how to use LoadForge to perform effective stress testing on your Symfony application.
LoadForge is a powerful load testing tool that allows you to create, manage, and execute sophisticated load tests with ease. To get started with LoadForge, follow these steps:
To effectively test your Symfony application, you should configure LoadForge to mimic real-world usage patterns. This includes setting appropriate request rates, concurrency levels, and durations. Here is an example configuration:
{
"scenarios": [
{
"name": "Homepage Load",
"requests": [
{
"method": "GET",
"url": "https://your-symfony-app.com/"
}
]
},
{
"name": "API Load",
"requests": [
{
"method": "GET",
"url": "https://your-symfony-app.com/api/data"
}
]
}
],
"config": {
"concurrency": 100,
"ramp_up": 5,
"duration": 60
}
}
Once your scenarios are defined and configured, click on "Run Test" to start the load testing process. LoadForge will launch multiple virtual users to execute your test scenarios concurrently. During the test, LoadForge will collect essential metrics such as response times, request rates, and error rates.
After the test is completed, you can analyze the results in the LoadForge dashboard. Key metrics to focus on include:
By reviewing the results, you can identify performance bottlenecks in your Symfony application. Look for endpoints with high response times or error rates and correlate them with your Doctrine queries. For instance, if you notice slow response times on a particular API endpoint, investigate the underlying Doctrine query for potential optimization opportunities.
Once you have made optimizations to your Doctrine queries, rerun your LoadForge tests to validate their impact. Compare the new test results with the previous ones to ensure that your optimizations have led to measurable performance improvements.
Consider the following example where a heavy query is causing performance issues:
$repository = $entityManager->getRepository(Entity::class);
$query = $repository->createQueryBuilder('e')
->leftJoin('e.relatedEntity', 'r')
->addSelect('r')
->where('e.someField = :value')
->setParameter('value', 'test')
->getQuery();
$result = $query->getResult();
After optimizing the query to reduce unnecessary joins and fetching only required fields, you can re-test using LoadForge to validate the improvement.
Load testing with LoadForge is an indispensable part of maintaining a high-performance Symfony application. By systematically applying stress to your application, analyzing the results, and iteratively optimizing your queries, you can ensure that your Symfony application is well-tuned and capable of handling real-world traffic efficiently.
## Conclusion
Maintaining an optimized, high-performance Symfony application requires a thorough understanding of how Doctrine ORM operates and the various strategies available to enhance its performance. Here’s a summary of the key points discussed:
1. **Understanding Doctrine ORM**: Knowing the inner workings and purpose of Doctrine ORM is the first step in leveraging its full potential within Symfony. Proper integration ensures a streamlined data management process.
2. **Profiling and Analyzing Queries**: Utilizing tools like Symfony Web Profiler, Doctrine Debug Toolbar, and LoadForge for load testing are essential in identifying performance bottlenecks. Profiling provides the insights needed to make informed optimizations.
3. **Optimizing DQL**: Writing efficient DQL queries is paramount. Avoiding N+1 query issues, using joins and fetches properly, and adhering to best practices ensures minimal database load and quicker data retrieval.
4. **Using Query Caching**: Implementing query caching can significantly reduce database load and speed up query execution. Ensuring that your project makes use of Doctrine’s caching capabilities will lead to substantial performance gains.
5. **Lazy vs Eager Loading**: Understanding when to use lazy loading versus eager loading is critical. While lazy loading can save memory, eager loading might reduce the number of queries executed, impacting your application’s performance based on context.
6. **Batch Processing and Bulk Inserts**: Handling large datasets efficiently is key. Implementing batch processing and bulk inserts can help minimize the performance overhead associated with multiple database operations.
7. **Efficient Pagination**: Properly implementing pagination helps in managing and displaying large sets of data without overwhelming the database. Efficient pagination techniques ensure smooth user experiences and optimized database queries.
8. **Indexing and Proper Database Design**: Designing a well-structured database with proper indexing ensures that queries are executed efficiently. A well-indexed database enhances query performance significantly.
9. **Load Testing with LoadForge**: Continuous performance validation using LoadForge helps in identifying and addressing performance bottlenecks, ensuring your optimizations are effective under real-world load conditions.
In conclusion, optimizing Doctrine queries is not a one-time task but an ongoing process. Regular profiling, careful query optimization, strategic use of caching and loading techniques, and robust database design are essential practices. Combining these with consistent load testing using LoadForge ensures that your Symfony application remains performant and scalable. Being proactive about performance will help maintain a smooth, efficient, and high-performing Symfony application.