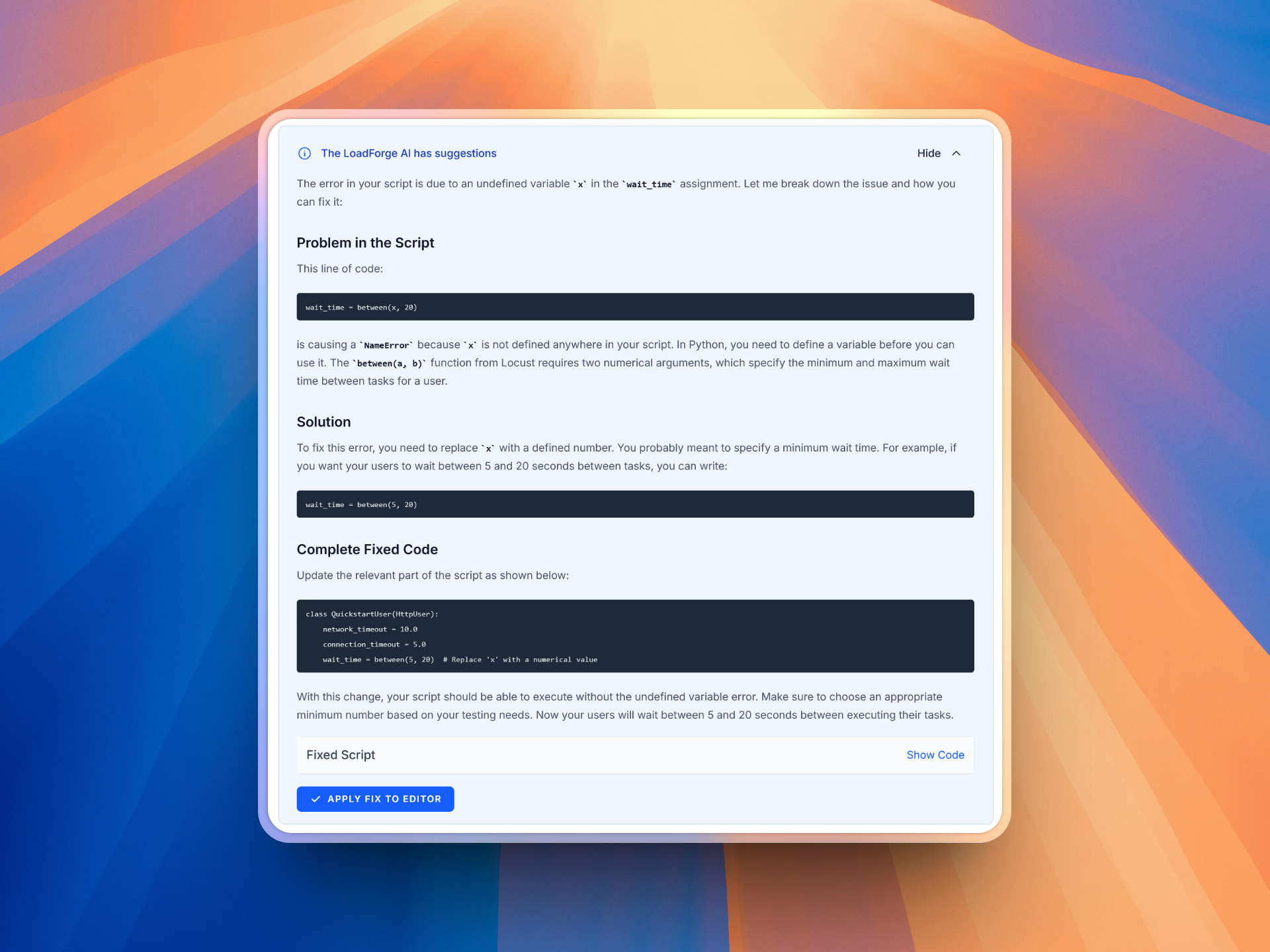
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
Welcome to our comprehensive guide on Optimizing Docker Images for Faster Performance. In the era of microservices and containerized applications, Docker has emerged as a cornerstone technology, facilitating rapid deployment, consistency, and scalability. However, as your applications grow in complexity...
Welcome to our comprehensive guide on Optimizing Docker Images for Faster Performance. In the era of microservices and containerized applications, Docker has emerged as a cornerstone technology, facilitating rapid deployment, consistency, and scalability. However, as your applications grow in complexity and scale, the performance of your Docker containers can become a critical factor. Optimizing Docker images is essential to ensure fast build times, efficient resource utilization, and smooth operation in production environments.
In this guide, we will explore various strategies to optimize your Docker images, helping you achieve:
By the end of this guide, you'll have a deep understanding of the best practices and techniques to fine-tune your Docker images for peak performance. Here's a preview of what each section will cover:
Our journey begins here, with a foundation that will arm you with the knowledge to optimize your Docker images effectively. Whether you are a seasoned developer or just starting with Docker, this guide will serve as a valuable resource in enhancing your containerized applications.
Let's dive in and start optimizing!
One of the most straightforward ways to optimize your Docker containers for faster performance is to start with a minimal base image. Using a slim base image reduces bloat, decreases attack surface, and improves both build and run-time performance by minimizing the resources required. In this section, we'll explore the benefits of minimal base images and provide examples of commonly used minimalist images.
Alpine Linux is a popular choice for a minimal Docker base image due to its small size and comprehensive package repository. A typical Alpine image is around 5 MB, making it an excellent option for reducing the footprint of your Docker containers.
Usage Example:
# Dockerfile using Alpine as the base image
FROM alpine:latest
RUN apk add --no-cache python3 \
&& python3 -m ensurepip \
&& rm -r /usr/lib/python*/ensurepip \
&& pip3 install --no-cache --upgrade pip setuptools
CMD ["python3"]
The scratch
base image is an entirely empty image, typically used for building statically compiled binaries to run within Docker. It provides the ultimate minimal environment.
Usage Example:
# Dockerfile using Scratch as the base image
FROM scratch
COPY hello /hello
CMD ["/hello"]
Google's Distroless images provide a minimal runtime environment while still including essential runtime dependencies for specific languages. It's a good balance between minimalism and usability for certain applications.
Usage Example:
# Dockerfile using Distroless for a Node.js application
FROM node:12 as build
WORKDIR /app
COPY . .
RUN npm install && npm run build
FROM gcr.io/distroless/nodejs:12
COPY --from=build /app /app
CMD ["node", "/app/dist/app.js"]
When choosing a minimal base image, consider your application's requirements and runtime dependencies. While Alpine and Distroless provide a balance of minimalism and functionality, scratch
is an excellent choice for highly optimized, statically compiled applications. Matching your base image to your application’s needs is key to achieving the best performance optimization.
In summary, starting with a minimal base image is a foundational step in optimizing your Docker containers. This choice not only trims down the image size but also enhances security and performance. In the following sections, we will build on this foundation to further refine and streamline your Docker build processes.
Layering your Dockerfile efficiently is crucial for minimizing image size and reducing build times. A well-structured Dockerfile can make a significant difference in the performance of your Docker images. This section will walk you through best practices for combining steps and ordering commands to optimize your Dockerfile layers.
In Docker, each command in a Dockerfile creates a new layer. Layers are like incremental changes that Docker builds and caches. If a layer changes, all subsequent layers are rebuilt. Therefore, carefully ordering and combining commands can help reuse cached layers and avoid unnecessary rebuilds.
One of the simplest techniques to reduce the number of layers is to combine multiple commands into one. While readability is important, strategic command combination can drastically improve performance.
RUN apt-get update && apt-get install -y \
python \
python-pip \
&& apt-get clean \
&& rm -rf /var/lib/apt/lists/*
This single RUN
command ensures that all these operations are executed in one layer, reducing the overall number of layers.
Docker caches intermediate results to speed up builds. To make the most of this, place less frequently changed commands early in the Dockerfile and commands that change frequently later. For example:
FROM python:3.9-slim
# Install system packages
RUN apt-get update && apt-get install -y build-essential
# Add requirements separately to leverage Docker cache
COPY requirements.txt /app/requirements.txt
# Install dependencies
RUN pip install --no-cache-dir -r /app/requirements.txt
# Copy application code
COPY . /app
CMD ["python", "/app/main.py"]
In this example, COPY requirements.txt
is placed before installing Python dependencies. This way, if only the application code changes, Docker can reuse the previous cached layer where dependencies were installed, speeding up the build process.
Multi-stage builds help keep your final image lean by copying only necessary artifacts from one stage to another. Here's an example:
FROM golang:1.17 AS builder
WORKDIR /app
COPY . .
RUN go build -o myapp
FROM alpine:latest
WORKDIR /root/
COPY --from=builder /app/myapp .
CMD ["./myapp"]
In this example, the Go application is built in the builder
stage, but only the final binary (myapp
) is copied into the final image. This removes the need for build tools like golang
in the final image, keeping it minimal.
.dockerignore
to Avoid Unnecessary FilesAdding a .dockerignore
file to exclude files and directories from being copied to the Docker image can reduce the image size and speed up the build.
# .dockerignore
node_modules
*.log
.git
This file ensures that unnecessary files, such as Git repositories and local dependencies, are not included in the Docker build context, leading to a more efficient build process.
Optimizing your Dockerfile involves strategic layering by combining commands, ordering them to maximize cache reuse, and using multi-stage builds. By applying these best practices, you can significantly improve your Docker container's build times and reduce image sizes, resulting in faster and more efficient deployments.
Docker’s layer caching mechanism is a powerful feature that can significantly speed up your build times and enhance your workflow efficiency. By understanding how Docker's caching works and strategically ordering your commands, you can maximize cache reuse and reduce unnecessary building steps. This section will walk you through the mechanisms of Docker caching and provide best practices to make the most out of this feature.
Docker builds images in layers. Each command in the Dockerfile creates a new layer. Docker caches these layers so that if it encounters a command it has already executed (with the same context and state), it can reuse the cached layer instead of executing the command again. This caching can save a considerable amount of time during the build process.
Docker determines whether a layer can be cached based on a specific set of conditions:
FROM
statement is unchanged and the base image is available locally.ENV
variables should not change.COPY
and ADD
should remain unaltered.To make the most out of Docker's caching mechanism and ensure that your builds are speedy, follow these best practices:
Place commands that change frequently lower in the Dockerfile, and commands that rarely change higher up. For example:
# Install dependencies: rarely changes
RUN apt-get update && apt-get install -y \
build-essential \
curl
# Copy package and install: changes more frequently
COPY package.json /app/
RUN npm install
# Copy the rest of the application code
COPY . /app/
Keep environment-specific configurations separate to avoid breaking the cache for unrelated commands. Use .dockerignore
to exclude files that don't need to be re-copied into the image:
# Copy only what is necessary for cacheable commands first
COPY package.json yarn.lock /app/
RUN yarn install
# Copy everything else that may change more frequently
COPY . /app/
When dealing with dependencies and build artifacts, consider using multi-stage builds to keep your images clean and small:
# Stage 1: Builder
FROM node:14 AS builder
WORKDIR /app
COPY package.json yarn.lock ./
RUN yarn install
COPY . .
RUN yarn build
# Stage 2: Final
FROM nginx:alpine
COPY --from=builder /app/build /usr/share/nginx/html
Sometimes, you need to pass build arguments that might change often. It’s a good practice to organize commands so changes in these arguments don’t invalidate the entire cache:
# ARG before frequently changing steps
ARG NODE_VERSION=14
FROM node:${NODE_VERSION}
WORKDIR /app
COPY package.json yarn.lock ./
RUN yarn install
COPY . .
RUN yarn build
By thoughtfully structuring your Dockerfile and understanding Docker's layer caching mechanism, you can drastically reduce your image build times. Remember to:
Utilizing these strategies will help ensure that you’re taking full advantage of Docker’s caching capabilities, leading to faster builds and a more efficient development process.
Efficiently managing dependencies is crucial to achieving faster build times and smaller Docker images. In this section, we'll explore strategies to ensure your dependencies are handled in the most optimal way possible. We'll cover multistage builds and intelligent use of package managers to streamline your Docker image creation process.
Multistage builds are a powerful feature in Docker that allow you to use multiple FROM
statements in your Dockerfile. This lets you create a temporary build environment and then copy only the necessary artifacts to the final image, which keeps it lean and efficient.
Here's an example of a Dockerfile using multistage builds:
# Stage 1: Build
FROM golang:1.16-alpine AS builder
WORKDIR /app
COPY . .
RUN go build -o myapp
# Stage 2: Package
FROM alpine:latest
WORKDIR /app
COPY --from=builder /app/myapp .
CMD ["./myapp"]
In the example above, the golang:1.16-alpine
image is used to build the Go application in a temporary build stage. Only the resulting binary (myapp
) is copied to the final image (alpine:latest
), which reduces the image size significantly by excluding build tools and other unnecessary files.
Using package managers wisely can also make a big difference. It is important to:
Here's how you can implement these practices in your Dockerfile:
FROM python:3.9-slim
# Install necessary packages
RUN apt-get update && apt-get install -y --no-install-recommends \
build-essential \
libssl-dev \
&& rm -rf /var/lib/apt/lists/*
# Set work directory
WORKDIR /app
# Install Python dependencies
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
# Copy the rest of the application code
COPY . .
CMD ["python", "app.py"]
Key points to note in this example:
--no-install-recommends
: This flag tells apt-get
to only install essential packages, reducing bloat.rm -rf /var/lib/apt/lists/*
command removes unnecessary package manager files after package installation.--no-cache-dir
with pip install
: This prevents pip
from caching packages, saving space in the final image.Combining the use of multistage builds and intelligent package management can lead to substantial improvements in both build time and image size. For instance, you can use a build stage to compile dependencies and then copy only the necessary files to the final image, while also following best practices for package management.
Here's a combined approach in a Dockerfile:
# Stage 1: Build
FROM node:14 AS builder
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
# Stage 2: Package
FROM node:14-slim
WORKDIR /app
COPY --from=builder /app/dist ./dist
COPY --from=builder /app/node_modules ./node_modules
CMD ["node", "dist/index.js"]
In this example:
node:14-slim
) and only copies the necessary files (dist
and node_modules
), resulting in a much smaller final image.By effectively managing dependencies through these strategies, you ensure that your Docker images are optimized for performance, both in terms of build times and runtime efficiency.
One of the crucial steps in optimizing Docker images for faster performance involves cleaning up intermediate files that are no longer needed once the build process has completed. These files can include temporary build artifacts, package manager caches, and other miscellaneous files that contribute to unnecessary bloat in your final Docker image. By meticulously removing these files, you can significantly reduce the size of your Docker images, which in turn can lead to faster build times and improved runtime performance.
Package managers often leave behind caches that are not needed after the installation of software packages. Removing these caches can save a significant amount of space.
For example, when using apt-get
in Debian-based images:
RUN apt-get update && apt-get install -y \
some-package \
another-package && \
rm -rf /var/lib/apt/lists/*
For Alpine-based images using apk
:
RUN apk --no-cache add \
some-package \
another-package
The --no-cache
flag ensures that the package index is not stored locally, keeping the image size to a minimum.
Temporary files created during the build process should be explicitly removed in your Dockerfile.
RUN ./configure \
&& make \
&& make install \
&& rm -rf /tmp/*
In this example, we make sure to clean up the /tmp
directory after the installation process is complete.
Each RUN
instruction in a Dockerfile creates a new layer. Combining commands within a single RUN
instruction can help reduce the number of layers and, consequently, the size of the final image.
RUN wget -qO- https://example.com/install.sh | bash && \
rm -rf /var/tmp/*
Here, the downloading and execution of a script are combined into a single step, and the temporary files are removed immediately afterward.
RUN
instruction to clean up intermediate files right after they are no longer needed.--no-install-recommends
for apt-get
.FROM golang:alpine AS builder
WORKDIR /app
COPY . .
RUN go build -o myapp .
FROM alpine:latest
WORKDIR /root/
COPY --from=builder /app/myapp .
CMD ["./myapp"]
In this example, the build stage uses a base image that includes all necessary tools (like Golang), while the final image contains only the built application, without any build artifacts.
Cleaning up intermediate files is a vital step in crafting efficient Docker images. By removing unnecessary files and combining commands effectively, you can produce leaner, more secure, and better-performing Docker containers. Keep these techniques in mind as you build your Docker images to ensure optimal performance.
In the next section, we'll discuss ways to optimize the runtime environment of your Docker containers, focusing on configurations that can further enhance performance.
Once you have optimized your Docker images, the next step is to fine-tune the runtime environment for optimal container performance. This section provides actionable strategies to help you configure resource limits and adjust environment variables to achieve better performance for your Docker containers.
One of the crucial aspects of optimizing the runtime environment is setting appropriate resource limits for your Docker containers. By properly managing resources such as CPU and memory, you ensure that containers do not consume more than their fair share and cause performance issues.
You can control the CPU usage of your Docker containers using the --cpus
flag. This flag allows you to specify the number of CPU cores that a container can use:
docker run --cpus="1.5" myimage
In this example, the container is restricted to using at most 1.5 CPU cores.
To prevent a container from consuming too much memory, you can use the --memory
flag. This flag lets you set the maximum amount of memory a container can use:
docker run --memory="512m" myimage
Here, the container is limited to using 512 MB of memory.
Environment variables can play a significant role in the performance of your Docker containers. Properly set environment variables can control application behavior, tuning it for better efficiency and resource usage.
JAVA_OPTS: If you are running Java applications, setting the JAVA_OPTS
environment variable can optimize JVM performance. For example:
docker run -e JAVA_OPTS="-Xms512m -Xmx1024m" myimage
This example sets the initial and maximum heap size for the JVM.
NODE_ENV: When running Node.js applications, set the NODE_ENV
environment variable to production
for optimized performance:
docker run -e NODE_ENV=production myimage
DB_URL: Ensure that your application is connected to optimized database endpoints by setting the DB_URL
environment variable appropriately.
docker-compose
When using Docker Compose, you can define resource constraints and environment variables for each service in the docker-compose.yml
file:
version: '3'
services:
myapp:
image: myimage
deploy:
resources:
limits:
memory: 512M
cpus: '1.5'
environment:
- JAVA_OPTS=-Xms512m -Xmx1024m
- NODE_ENV=production
This configuration file achieves the same effect as running docker run
commands with resource limits and environment variables.
Optimizing the runtime environment of your Docker containers is essential for ensuring consistency, efficiency, and optimal resource usage. By configuring resource limits and fine-tuning environment variables, you can significantly improve the performance of your Dockerized applications. Next, we'll explore monitoring and performance testing techniques, including the use of LoadForge for load testing, to help you make data-driven decisions in your optimization process.
Once you have optimized your Docker images and their build processes, the next step is to ensure that your performance improvements hold up under real-world conditions. Monitoring and performance testing are crucial for identifying bottlenecks, ensuring scalability, and validating that your optimizations are effective. This section will introduce techniques and tools for monitoring container performance, with a strong emphasis on load testing using LoadForge to make data-driven decisions.
Effective monitoring helps you understand the performance characteristics of your Docker containers in real-time. It enables you to track resource usage, identify performance issues, and make informed decisions.
Docker provides a built-in mechanism for accessing real-time metrics. You can use the docker stats
command to monitor container performance metrics like CPU, memory usage, network I/O, and block I/O.
Example:
docker stats <container_id_or_name>
For more detailed and customizable monitoring, integrating Prometheus and Grafana with your Docker setup is a powerful solution. Prometheus collects metrics from Docker containers, and Grafana visualizes this data, making it easier to identify trends and potential issues.
Prometheus Docker configuration in docker-compose.yml
:
version: '3.7'
services:
prometheus:
image: prom/prometheus
volumes:
- ./prometheus.yml:/etc/prometheus/prometheus.yml
ports:
- "9090:9090"
Grafana Docker configuration in docker-compose.yml
:
version: '3.7'
services:
grafana:
image: grafana/grafana
ports:
- "3000:3000"
While monitoring gives you a snapshot of container performance, load testing simulates traffic to evaluate how your application behaves under stress. LoadForge is an excellent tool for conducting load tests that can generate detailed performance metrics.
Account Creation and Setting Up Tests: Sign up for a LoadForge account if you haven't already. Setting up a test is straightforward—input the target URL, configure the number of concurrent users, and specify the duration.
Running Your Load Tests: After configuring your test, start it from the LoadForge dashboard. The system will simulate traffic to your application and collect performance data for analysis.
Interpreting LoadForge Results: LoadForge provides detailed metrics, including response times, error rates, and throughput. Here's a quick guide to interpret the key results:
Suppose you execute a LoadForge test with the following results:
A 2% error rate indicates that the container struggles under certain conditions, while a peak response time of 1200ms shows potential bottlenecks. These insights help you decide whether to optimize further or scale your containers horizontally.
Based on the data gathered from monitoring and load testing, here are steps you can take:
By monitoring your containers and conducting thorough load tests with LoadForge, you ensure that your Dockerized applications can handle traffic efficiently under various conditions.
Ensuring your Docker images are secure is not only crucial for protecting your applications and data but can also contribute to improved performance. A secure container minimizes potential vulnerabilities, reduces the attack surface, and can lead to more efficient use of resources. Here, we’ll discuss best practices to enhance both your Docker image's security and performance.
One of the most effective ways to secure a Docker container is to minimize its attack surface. This involves starting with a minimal base image and only including essential components. Unnecessary packages and files not only increase the size of your Docker image but also introduce potential security vulnerabilities.
For example, opting for a minimal base image like Alpine can be an excellent choice:
FROM alpine:latest
RUN apk add --no-cache [your-required-packages]
This ensures that only essential packages are included, reducing both the potential attack surface and the image size.
Regularly updating your base image and dependencies ensures that any known vulnerabilities are patched, reducing the risk of exploitation. Schedule periodic updates and rebuild your Docker images to incorporate the latest security patches and enhancements.
Here’s a simple illustration of updating a base image:
# Use the latest official Ubuntu base image
FROM ubuntu:latest
# Update package list and upgrade all packages to the latest versions
RUN apt-get update && apt-get upgrade -y
Running containers with the least privileges necessary can dramatically reduce the impact of potential security breaches. Wherever possible, avoid running your applications as the root user. Instead, create a new user with minimal permissions required for the application to function.
# Use an existing minimal base image
FROM nginx:alpine
# Add a new user and group with non-root privileges
RUN adduser -D myuser
USER myuser
# Copy application files and set permissions
COPY . /app
WORKDIR /app
RUN chown -R myuser:myuser /app
Avoid hardcoding sensitive information such as API keys and passwords directly into your Dockerfile. Utilize Docker secrets and environment variables to manage sensitive data securely.
# An example of how to use environment variables
FROM node:14
# Set environment variables for sensitive information
ENV DB_USER=myuser
ENV DB_PASSWORD=mypassword
# Copy application files and install dependencies
COPY . /app
WORKDIR /app
RUN npm install
Regularly scan your Docker images for vulnerabilities using tools like Docker's built-in security scanning capabilities or third-party solutions like Clair or Trivy. This practice helps identify and address potential vulnerabilities promptly.
Secure configurations often lead to better resource utilization. For example, limiting the resources available to a container can prevent Denial of Service (DoS) attacks and ensure your container runs efficiently. You can configure resource constraints directly within your Docker Compose file or Docker run commands.
# Example Docker Compose configuration with resource limits
version: '3.7'
services:
webapp:
image: myapp:latest
deploy:
resources:
limits:
cpus: '0.5'
memory: 512M
Incorporating security best practices is essential for building robust Docker images. By minimizing the attack surface, regularly updating and patching, adhering to the principle of least privilege, managing secrets securely, performing vulnerability scanning, and efficiently utilizing resources, you can build Docker images that are not only secure but also optimized for performance.
This section complements other Docker image optimization techniques discussed in this guide to help ensure that your applications run securely and efficiently. For ongoing monitoring, load testing, and performance testing, consider using tools like LoadForge to make data-driven optimization decisions, further enhancing both security and performance.
In this guide, we've explored a comprehensive set of strategies and best practices to optimize Docker images for faster performance. From foundational concepts to advanced techniques, let's summarize the key points covered:
Choosing a Minimal Base Image:
alpine:latest
debian:slim
Layering Your Dockerfile Effectively:
RUN
instruction.Leveraging Docker Caching:
.dockerignore
to exclude unnecessary files.Managing Dependencies Efficiently:
Cleaning Up Intermediate Files:
RUN apt-get clean && rm -rf /var/lib/apt/lists/*
Optimizing Runtime Environment:
Monitoring and Performance Testing:
Security Considerations:
docker scan
can help maintain a secure and efficient Docker environment.For those interested in delving deeper into Docker optimization and performance, here are some valuable resources:
By continuously applying these practices, you can maintain optimal performance and security for your Dockerized applications. Remember, performance tuning is an ongoing process that involves regular monitoring and updates. The habits you develop today will keep your containers running smoothly and efficiently in the long run.