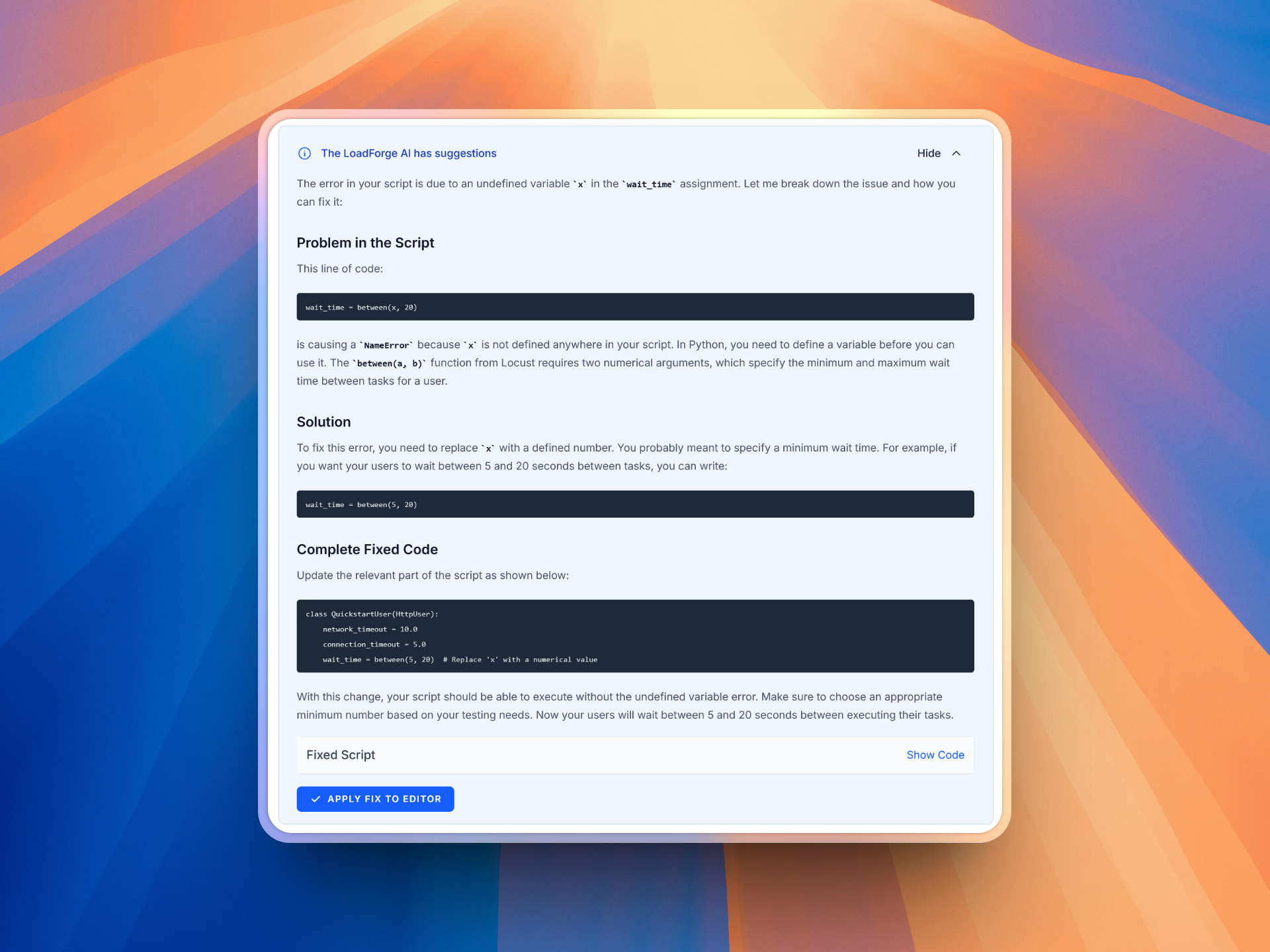
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
In the dynamic world of web development, performance is paramount. For Ruby on Rails applications, one of the critical areas affecting performance is database queries. Inefficient database queries can lead to slow response times, frustrated users, and ultimately, a loss...
In the dynamic world of web development, performance is paramount. For Ruby on Rails applications, one of the critical areas affecting performance is database queries. Inefficient database queries can lead to slow response times, frustrated users, and ultimately, a loss in traffic and revenue. Conversely, well-optimized queries can enhance the application's performance, improve scalability, and provide a seamless user experience.
Rails, with its convention-over-configuration philosophy, offers a range of tools and practices that can help optimize database queries. However, it's essential to understand and implement these effectively to reap their full benefits. Throughout this guide, we will explore various strategies to fine-tune your database interactions in a Ruby on Rails environment.
Optimizing database queries is crucial for several reasons:
In this guide, we will delve into specific areas where you can optimize your database queries in Ruby on Rails, including:
By the end of this guide, you will have a comprehensive understanding of the best practices and tools available to optimize database queries in Ruby on Rails. This will ensure your application runs smoothly under heavy load, provides an excellent user experience, and scales efficiently as your user base grows. Let’s get started with understanding one of the most common performance pitfalls: the N+1 query problem.
The N+1 query problem is a common performance pitfall in Ruby on Rails applications that can occur when an application retrieves records from a database. This issue arises when Rails executes a separate query for each associated object in a one-to-many relationship, resulting in an excessive number of database queries and significantly impacting performance.
In an N+1 query scenario, the application first issues a query to load the primary records (N) and then issues an additional query for each associated record (1 for each). For example, consider a scenario where you have a User
model, each of which has many Posts
:
@users = User.all
@users.each do |user|
puts user.posts.count
end
In this example, Rails will execute the following queries:
One query to load all the User
records:
SELECT * FROM users;
An additional query for each user to load their associated posts
:
SELECT * FROM posts WHERE user_id = 1;
SELECT * FROM posts WHERE user_id = 2;
...
SELECT * FROM posts WHERE user_id = N;
This results in N+1
queries, where N
is the number of User
records.
N+1 queries can drastically reduce the performance of your application due to the overhead of multiple database round trips. The increased number of queries puts additional load on your database server and can lead to slower response times, especially as the number of primary records grows.
Bullet Gem
The Bullet gem is a valuable tool for identifying N+1 queries in your Rails application. Bullet will notify you when your application is making inefficient queries and suggest optimizations.
To install Bullet, add it to your Gemfile:
gem 'bullet'
Then, configure Bullet in an initializer file, like config/initializers/bullet.rb
:
if defined?(Bullet)
Bullet.enable = true
Bullet.alert = true
Bullet.bullet_logger = true
Bullet.rails_logger = true
Bullet.add_footer = true
end
Bullet will alert you in the browser, log files, or logs when it detects an N+1 query.
LoadForge
Using LoadForge, you can conduct load testing to observe how your application performs under increased traffic—a practical way to identify the impact of N+1 queries. LoadForge simulates multiple users interacting with your application, allowing you to identify performance bottlenecks, including inefficient database queries.
To integrate LoadForge for identifying N+1 issues:
Understanding the N+1 query problem is crucial for maintaining the performance and scalability of your Ruby on Rails application. By leveraging tools like Bullet to identify N+1 queries and conducting load testing with LoadForge, you can spot performance bottlenecks early and apply the necessary optimizations to ensure efficient database interactions. In the following sections, we'll explore techniques for resolving N+1 queries and implementing other best practices for database performance optimization in Rails.
One of the most effective ways to optimize database queries in Ruby on Rails applications is through eager loading. Eager loading helps batch database queries and reduces the overall number of queries executed against the database, thereby mitigating performance issues such as the N+1 query problem.
Eager loading is a technique where associated records are retrieved in the same query as the main record(s), instead of making multiple queries to fetch each associated record. In Rails, eager loading can be implemented using the includes
, preload
, or joins
methods.
includes
The includes
method is one of the most straightforward ways to implement eager loading. It retrieves the specified associations in a single query. Here's an example:
# Without eager loading
posts = Post.all
posts.each do |post|
puts post.comments.count
end
# With eager loading
posts = Post.includes(:comments).all
posts.each do |post|
puts post.comments.count
end
In the above example, the second approach with includes
ensures that all comments for the posts are loaded in one go, reducing the number of SQL queries significantly.
preload
The preload
method is similar to includes
but guarantees that two separate queries are made: one for the main records and one for the associated records. It's generally used when you have more complex conditions or calculations to perform on the associations.
# Using preload
posts = Post.preload(:comments).all
posts.each do |post|
# Perform some complex calculations with post.comments
end
joins
The joins
method produces an inner join SQL query and can be used with conditions. It allows you to filter records based on associated tables but doesn’t necessarily load the associated records into memory.
# Using joins
posts = Post.joins(:comments).where(comments: { status: 'approved' })
If you need to access the associated records after filtering, it’s a good practice to follow joins
with a call to includes
:
# Combining joins with includes
posts = Post.joins(:comments).where(comments: { status: 'approved' }).includes(:comments)
posts.each do |post|
# Now we can safely access post.comments without additional queries
end
includes
combines eager loading and joins, making one query when possible, while preload
forces two separate queries and joins
only performs SQL joins without loading associations.By following these practices and understanding the different methods for eager loading, you can significantly improve the performance of your Rails application, ensuring that database queries are optimized and your user experience remains smooth and fast.
Effective caching strategies are critical for optimizing the performance of Ruby on Rails applications. By reducing the number of database queries, caching can significantly lower the database load and improve response times. In this section, we will explore various caching techniques available in Rails, including Rails cache, fragment caching, and Russian doll caching.
Rails provides a built-in caching mechanism that makes it easy to store the results of expensive database queries. You can use the Rails.cache
to store and retrieve cached data.
Using Rails.cache
To cache a query result:
# Cache a query result for 5 minutes
posts = Rails.cache.fetch('posts', expires_in: 5.minutes) do
Post.all.to_a
end
In this example, the query results are cached under the key posts
for 5 minutes. If the key does not exist in the cache, the block will be executed, and the result will be stored in the cache.
Fragment caching allows you to cache parts of a view. This is useful when certain parts of your page do not change often and can be cached separately.
Using Fragment Caching in Views
Here’s how to use fragment caching in views:
<% cache @post do %>
<%= @post.title %>
<%= @post.body %>
<% end %>
In this example, the HTML markup for the @post
is cached. When the cache is expired or does not exist, Rails will execute the block and store the rendered HTML.
Russian doll caching is an advanced form of fragment caching that caches nested components. This technique minimizes the amount of caching invalidation needed when one fragment changes.
Using Russian Doll Caching
Here’s an example of Russian doll caching:
<% cache @post do %>
<%= @post.title %>
<%= @post.body %>
<% cache [@post, @post.comments] do %>
Comments
<%= render @post.comments %>
<% end %>
<% end %>
In this example, the outer cache block caches the entire post, including the comments section. The inner cache block caches the comments for the specific post. If the post changes, only the outer cache is expired. If the comments change, only the inner cache is expired.
Implementing effective caching strategies in your Ruby on Rails application can lead to substantial performance improvements. By using Rails cache, fragment caching, and Russian doll caching, you can minimize database load and enhance response times. Remember to review and invalidate cache appropriately to ensure users always have access to the most accurate data.
Writing efficient SQL queries is crucial for the performance of your Ruby on Rails application. Poorly optimized queries can lead to slow response times and a degraded user experience. Here, we explore best practices to optimize SQL queries in Rails, focusing on using select
, pluck
, and database-specific features to create efficient and performance-optimized queries.
select
for Optimal Data RetrievalThe select
method in Rails allows you to specify the columns you need from the database, which can reduce the amount of data loaded into memory and improve performance.
Instead of selecting all columns with User.all
, only fetch the necessary columns:
users = User.select(:id, :name, :email)
This technique decreases payload size and speeds up query execution by limiting the data returned from the database.
pluck
for Array RetrievalWhen you only need one or two columns and no ActiveRecord objects, pluck
is an efficient way to retrieve raw data from the database as an array.
To retrieve user emails, use:
user_emails = User.pluck(:email)
pluck
skips the instantiation of ActiveRecord objects, resulting in significant performance improvements for simple read operations.
Utilizing database-specific functionalities can further enhance the performance of your queries. For instance, PostgreSQL offers several extensions and functions that can be used directly within ActiveRecord queries.
Example of using PostgreSQL's jsonb
functions:
User.where("metadata->>'active' = ?", 'true')
This leverages PostgreSQL's JSONB indexing capabilities, delivering faster query results on JSON data.
exists?
To check if a record exists without loading it, use exists?
as it only returns a boolean flag, which is more efficient than loading the entire object:
user_exists = User.exists?(email: '[email protected]')
COUNT(*)
For count queries, avoid the overhead of COUNT(*)
when not necessary. Use size
, count
, or length
appropriately based on your use case:
relation.size
for loaded relationsrelation.count
for unassociated countsrelation.length
for eagerly loaded collectionsAggregate SQL functions like SUM
, AVG
, and MAX
can be directly translated into ActiveRecord queries, reducing the need for complex Ruby enumerations:
Calculate the average age of users:
User.average(:age)
This shifts the computational load to the database, which is optimized for such operations.
Here’s an example that combines several optimization techniques:
User.select("users.name, COUNT(posts.id) AS post_count")
.joins(:posts)
.group("users.id")
.order("post_count DESC")
.limit(10)
This query retrieves the top 10 users with the most posts, utilizing select
, joins
, and group
to produce an optimized and efficient SQL query.
By following these best practices, you can ensure your SQL queries are executed more efficiently, reducing database load and improving your Rails application's performance.
Database indexing is a crucial optimization technique that can dramatically enhance the performance of your Ruby on Rails application. Proper indexing ensures that database queries execute more quickly, significantly improving the responsiveness of your application.
When you query a database without an index, the database engine must scan through every row of the table to locate the relevant data. This full table scan can be particularly time-consuming for large datasets. Indexing helps by creating a data structure that enables the database to quickly locate and retrieve the data, just like an index in a book helps you quickly find information.
Benefits of database indexing include:
WHERE
clauses), and joining tables.In Ruby on Rails, creating indexes involves adding them to your database migrations. Here’s how you can add an index to a table column:
class AddIndexToUsersEmail < ActiveRecord::Migration[6.1]
def change
add_index :users, :email, unique: true
end
end
This migration creates a unique index on the email
column of the users
table. Unique indexes ensure that all values in the indexed column are distinct.
WHERE
Clauses: Index columns that frequently appear in queries' WHERE
conditions to speed up data retrieval.For example, to create a composite index on first_name
and last_name
:
class AddCompositeIndexToUsers < ActiveRecord::Migration[6.1]
def change
add_index :users, [:first_name, :last_name]
end
end
To illustrate the impact of indexing, consider a simple query before and after indexing:
Without Index:
User.where(email: '[email protected]')
The database engine performs a full table scan, checking each row's email
field.
With Index:
class AddIndexToUsersEmail < ActiveRecord::Migration[6.1]
def change
add_index :users, :email
end
end
With the index in place, the database engine quickly locates the rows with the email
value of '[email protected]' using the index structure, typically a B-tree.
Employing the right indexing strategies can have a profound impact on the performance of your Rails application. The appropriate indexes can transform slow, inefficient queries into rapid, responsive ones, thus providing a smoother user experience and improving the overall scalability of your application. By understanding the importance of indexing and how to implement it effectively, you can ensure that your application remains performant even as your dataset grows.
In the next sections, we will delve into more optimization techniques such as caching and efficient SQL query writing, further bolstering the performance of your Rails application.
## Pagination and Limiting Results
Handling large datasets efficiently is crucial for the performance and user experience of your Ruby on Rails application. Without proper pagination and limiting techniques, your application may slow down significantly, resulting in longer load times and a poor user experience. In this section, we'll explore several techniques for paginating results and limiting the number of records returned in a query using methods like `limit`, `offset`, and the `will_paginate` gem.
### Using `limit` and `offset`
Rails ActiveRecord provides `limit` and `offset` methods to control the number of records returned and the starting point for the query. These methods are particularly useful for implementing custom pagination logic.
- **`limit`**: Specifies the maximum number of records to return.
- **`offset`**: Specifies the number of records to skip before starting to return records.
Here's an example of how to use `limit` and `offset`:
```ruby
# Fetch 10 records starting from the 20th record
@records = Model.limit(10).offset(20)
Custom pagination allows you to have more control over the pagination logic. Suppose you want to paginate through Post
records by displaying 10 posts per page. Here’s how you can achieve this:
class PostsController < ApplicationController
def index
@page = params[:page] || 1
@per_page = 10
@posts = Post.limit(@per_page).offset((@page.to_i - 1) * @per_page)
end
end
In the view, you can create simple navigation links:
<%= link_to 'Previous', posts_path(page: @page.to_i - 1) unless @page.to_i == 1 %>
<%= link_to 'Next', posts_path(page: @page.to_i + 1) %>
will_paginate
GemThe will_paginate
gem is a popular pagination library for Rails, making it easier to implement pagination without writing custom logic.
Add will_paginate
to your Gemfile:
gem 'will_paginate', '~> 3.3'
Run the bundle command to install it:
bundle install
To paginate Post
records, use the paginate
method in your controller:
class PostsController < ApplicationController
def index
@posts = Post.paginate(page: params[:page], per_page: 10)
end
end
In the view, render the pagination controls:
<%= will_paginate @posts %>
This will automatically generate next and previous links, along with page numbers, making navigation between pages seamless.
Paginating and limiting results is vital for managing large datasets efficiently in Ruby on Rails applications. Whether you decide to implement custom pagination using limit
and offset
or utilize a gem like will_paginate
, understanding these techniques will help you improve query performance and enhance the overall user experience. By efficiently handling large datasets, your application can remain responsive and performant regardless of the data volume it manages.
In Ruby on Rails, keeping web requests fast and responsive is crucial for delivering a seamless user experience. One effective strategy to achieve this is by offloading time-consuming or resource-intensive tasks to background jobs. This approach frees up your web server to handle incoming requests promptly while deferring heavy processing to background workers. This section will guide you through setting up and using background jobs using popular libraries like Sidekiq, Resque, and Active Job.
Heavy processing activities such as sending emails, generating reports, or performing complex calculations can significantly slow down your application if executed within the request-response cycle. Offloading these tasks to background jobs helps in:
Active Job is a framework for declaring jobs and making them run on a variety of queueing backends. Here’s how to set it up:
Create a Job: You can generate a new job using the Rails generator:
rails generate job ExampleJob
Define the Job:
Open the generated job file (app/jobs/example_job.rb
) and define the tasks to be executed asynchronously:
class ExampleJob < ApplicationJob
queue_as :default
def perform(*args)
# Do something later
UserMailer.welcome_email(args.first).deliver_now
end
end
Enqueue the Job: You can enqueue the job from anywhere in your application:
ExampleJob.perform_later(User.first)
Sidekiq is a popular choice for background processing in Rails applications due to its performance and simplicity. Follow these steps to integrate Sidekiq with Rails:
Add Sidekiq Gem: Add Sidekiq to your Gemfile:
gem 'sidekiq'
Run bundle install
to install the gem.
Configure Sidekiq:
Create a Sidekiq configuration file (config/sidekiq.yml
):
:queues:
- default
Modify your config/application.rb
file to integrate Sidekiq with Rails:
config.active_job.queue_adapter = :sidekiq
Create a Worker: Define a new Sidekiq worker:
class ExampleWorker
include Sidekiq::Worker
def perform(user_id)
user = User.find(user_id)
UserMailer.welcome_email(user).deliver_now
end
end
Enqueue the Job: You can enqueue the worker from your application code:
ExampleWorker.perform_async(User.first.id)
Resque provides Rails applications with Redis-backed backend jobs processing. Here’s how to set it up:
Add Resque Gem: Add Resque to your Gemfile:
gem 'resque'
Run bundle install
to install the gem.
Configure Resque:
Add Resque configuration in config/initializers/resque.rb
:
require 'resque/server'
Rails.application.config.active_job.queue_adapter = :resque
Create a Worker:
Define a Resque worker in app/workers/example_worker.rb
:
class ExampleWorker
@queue = :default
def self.perform(user_id)
user = User.find(user_id)
UserMailer.welcome_email(user).deliver_now
end
end
Enqueue the Job: Enqueue the worker job from your application code:
Resque.enqueue(ExampleWorker, User.first.id)
Here are some best practices for using background jobs effectively:
Background jobs are indispensable for maintaining the performance and responsiveness of your Ruby on Rails application. Whether you choose Sidekiq, Resque, or Active Job, implementing background jobs can significantly enhance the efficiency and scalability of your application.
Effective database connection management is critical for the performance and scalability of any Ruby on Rails application. One of the primary techniques to achieve this is through database connection pooling. This section will explain what database connection pooling is, how to configure it in Rails, and the benefits it provides in terms of performance and resource management.
Database connection pooling is a method of creating and managing a pool of database connections that can be reused for multiple database requests. Instead of creating and closing a new connection for each request, which is resource-intensive, connection pooling allows a set number of connections to be maintained and reused. This can significantly improve the performance and responsiveness of a Rails application, especially under load.
Rails makes it straightforward to configure connection pooling through the database.yml
configuration file. You can specify the pool
size which determines the maximum number of database connections that can be maintained.
Here’s an example configuration:
default: &default
adapter: postgresql
encoding: unicode
pool: 5 # Change this number according to your requirements
username: your_username
password: your_password
host: localhost
development:
<<: *default
database: myapp_development
test:
<<: *default
database: myapp_test
production:
<<: *default
database: myapp_production
pool: 15 # Higher pool size for production environment
In the configuration above:
pool: 5
indicates that Rails will maintain up to 5 connections in the development environment.15
to handle more simultaneous connections, which is often necessary to accommodate higher traffic.By configuring and appropriately managing database connection pooling in Rails, you can significantly enhance the performance and resource management of your application. This enables your application to better handle growing traffic and ensures a smooth and fast user experience.
In the next sections, we will further explore strategies for optimizing database interactions, including caching queries, optimizing SQL, and more.
Ensuring that your Ruby on Rails application can handle increased traffic requires rigorous monitoring and performance testing. This involves identifying bottlenecks, analyzing query performance, and simulating real-world traffic. Let's explore some essential tools and methodologies to achieve this.
New Relic: New Relic is a powerful application performance monitoring tool that provides real-time insights into your application's performance metrics. It tracks database queries, response times, and throughput, helping you identify slow or inefficient queries.
# Gemfile
gem 'newrelic_rpm'
Scout: Scout offers detailed performance monitoring for Rails applications. With features like transaction tracing and custom instrumentation, Scout helps pinpoint database-related performance issues.
# Gemfile
gem 'scout_apm'
Bullet: Bullet is a gem that helps detect N+1 queries and unused eager loading, making it invaluable for optimizing database interactions.
# Gemfile
gem 'bullet'
PgHero: Specifically designed for PostgreSQL databases, PgHero provides insights into query performance, database indexes, and overall health.
# Gemfile
gem 'pghero'
To identify bottlenecks, monitor these metrics regularly:
Load testing is crucial to ensure your application performs well under increased traffic. LoadForge is an excellent tool for conducting load tests on your Rails application. It simulates real-world traffic and stress-tests your application so you can catch performance issues before they affect users.
Configure LoadForge: Sign up for a LoadForge account and set up your testing parameters. Define the number of concurrent users, test duration, and specific URLs/endpoints to test.
Create a Test Script: Write a test script that simulates user interactions with your application. LoadForge supports various scripting languages and offers an intuitive interface for creating tests.
- description: "User login and fetch dashboard"
steps:
- url: "http://yourapp.com/login"
method: "POST"
data:
username: "testuser"
password: "password"
- url: "http://yourapp.com/dashboard"
method: "GET"
Run the Load Test: Execute the test and monitor the results in real-time. LoadForge will provide detailed metrics on response times, error rates, and throughput.
Analyze Results: Post-test, analyze the results to identify slow queries, high error rates, or any other performance issues. Use this data to optimize your application further.
By combining continuous monitoring with periodic load testing, you can maintain optimal database performance and ensure your Rails application is prepared for spikes in traffic. Regularly review New Relic or Scout metrics, address issues flagged by Bullet, and schedule LoadForge tests to maintain a robust and responsive application.
Implementing these monitoring and performance testing tools will help you keep a close eye on your database's health and your application's performance, ensuring a seamless user experience even as your traffic scales.
Optimizing database queries in Ruby on Rails is crucial for improving the performance, scalability, and overall user experience of your application. By addressing common inefficiencies and leveraging Rails' powerful tools and techniques, you can significantly enhance your app’s responsiveness and reduce server load.
Understanding and Solving the N+1 Query Problem: Identifying and resolving N+1 query issues using tools like Bullet and performing load testing with LoadForge can prevent unnecessary database hits.
Eager Loading:
Adopting eager loading techniques with includes
, preload
, and joins
to batch database queries helps in minimizing the number of queries executed against the database, thus speeding up your application.
Caching Database Queries: Implementing caching strategies such as Rails cache, fragment caching, and Russian doll caching can drastically reduce database load and improve response times. A well-cached application is always more performant from a user’s perspective.
Optimizing SQL Queries:
Writing efficient SQL queries using methods like select
and pluck
and utilizing database-specific features can help in reducing query complexity. Always aim for lean and optimized queries.
Database Indexing: Proper indexing of database tables can yield substantial performance gains. Ensure you have the right indexes in place to speed up query execution times.
Pagination and Limiting Results:
Efficiently handle large datasets by paginating results and using limit
and offset
. Incorporating gems like will_paginate
can provide a seamless user experience while managing backend load effectively.
Background Jobs for Resource-Intensive Tasks: Offloading heavy processing tasks to background jobs using tools like Sidekiq, Resque, or Active Job prevents slow web requests, keeping the application responsive.
Database Connection Pooling: Configuring database connection pooling helps in efficient resource management and avoids the overhead of establishing new database connections on each request.
Monitoring and Performance Testing: Continuous performance monitoring and conducting regular load testing using LoadForge can help you identify bottlenecks early and ensure your application remains performant under varying loads.
It's important to recognize that optimizing database queries is not a one-time task but an ongoing process. Applications evolve, new features are added, and usage patterns change over time. Regularly revisiting your database interactions, monitoring performance, and adjusting your strategies accordingly will ensure that your application continues to run efficiently.
By integrating these practices into your development workflow, you will achieve a more robust, scalable, and high-performance Rails application. Keep leveraging advanced monitoring tools and perform load testing frequently to stay ahead of performance issues and provide an exceptional user experience.