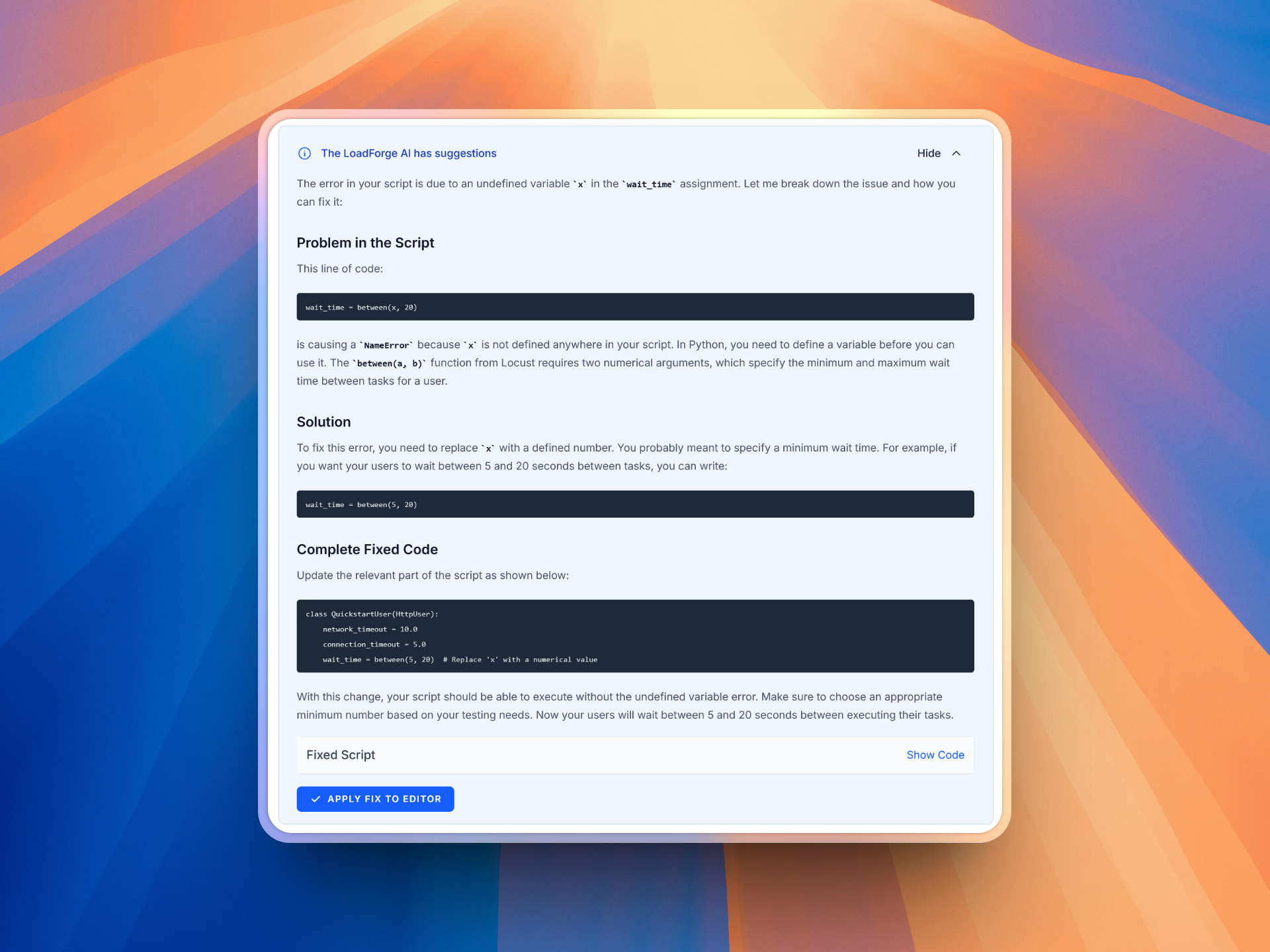
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
In the realm of Spring applications, the performance and scalability of your system hinge critically on how effectively you manage database connections. Database connection optimization isn't just a matter of best practices; it's a necessity for creating responsive, reliable, and...
In the realm of Spring applications, the performance and scalability of your system hinge critically on how effectively you manage database connections. Database connection optimization isn't just a matter of best practices; it's a necessity for creating responsive, reliable, and high-performing applications. This section will provide an overview of why optimizing database connections is crucial and discuss the adverse impacts of poor connection management.
Database connections serve as the lifeline between your Spring application and the database. Every request that necessitates data retrieval or manipulation relies on these connections. A well-optimized connection strategy ensures:
Failing to optimize database connections can have a dramatic effect on your application's performance and may lead to several critical issues.
Connection Leaks:
// Example of a potential connection leak
try (Connection conn = dataSource.getConnection()) {
// Perform database operations
} catch (SQLException e) {
// Handle exceptions
}
Excessive Connection Creation:
Unoptimized Pool Size:
# Example configuration for optimal connection pool sizing in Spring
spring.datasource.hikari.minimum-idle=5
spring.datasource.hikari.maximum-pool-size=20
spring.datasource.hikari.idle-timeout=30000
Timeout Issues:
# Configuring timeouts to ensure optimal performance
spring.datasource.hikari.connection-timeout=30000
spring.datasource.hikari.max-lifetime=1800000
Poor Exception Handling:
Understanding and addressing these potential issues through effective database connection optimization will lay a solid foundation upon which we can build a more responsive and scalable Spring application. In the coming sections, we will delve deeper into connection pooling, configuration, best practices, monitoring, and advanced topics to ensure that your Spring application's database interactions are as efficient and reliable as possible.
Database connection pooling is a critical concept in the development and maintenance of high-performance Spring applications. This section delves into what connection pooling is, how it works, and why it’s crucial for scaling your Spring application. We'll also introduce HikariCP, a popular connection pooling library.
Connection pooling is a technique used to manage database connections efficiently by maintaining a cache of reusable connection objects. Instead of opening and closing a new connection each time a request is made, which is resource-intensive and slow, a pool of connections is kept available for reuse. This reduces the overhead associated with managing database connections and significantly enhances the application's performance.
Here's a step-by-step breakdown of how connection pooling operates:
Connection pooling provides several benefits essential for scaling Spring applications:
HikariCP is one of the most popular connection pooling libraries due to its high performance and lightweight nature. It is known for its low overhead, fast response time, and robust stability. Here’s why HikariCP stands out:
For those getting started, integrating HikariCP into a Spring Boot application is straightforward. Here’s a basic configuration:
In your pom.xml
or build.gradle
, add HikariCP as a dependency:
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>5.0.0</version>
</dependency>
implementation 'com.zaxxer:HikariCP:5.0.0'
Then, configure your application.properties
file:
spring.datasource.url=jdbc:mysql://localhost:3306/yourdatabase
spring.datasource.username=yourusername
spring.datasource.password=yourpassword
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.hikari.maximum-pool-size=10
spring.datasource.hikari.minimum-idle=2
spring.datasource.hikari.idle-timeout=30000
spring.datasource.hikari.connection-timeout=30000
spring.datasource.hikari.max-lifetime=1800000
With these configurations, your Spring application will utilize HikariCP for database connection pooling, enhancing the performance and scalability of your application.
Understanding and implementing connection pooling is fundamental for any developer looking to optimize their Spring application's performance. By using efficient connection pooling libraries like HikariCP, you can ensure that your application manages database connections optimally, providing a robust foundation for scalability and high performance.
This section has provided a comprehensive look into connection pooling, its workings, and the advantages it offers to Spring applications, setting the stage for further deep dives into more specific configurations and best practices in subsequent sections of this guide.
Effective management of database connections is essential for enhancing the performance and scalability of your Spring application. Connection pooling is a technique that allows applications to reuse existing connections instead of creating new ones, thus saving time and resources. In this section, we will walk you through the steps required to configure connection pools in a Spring application using the popular HikariCP library, and how to set optimal pool size parameters.
HikariCP is a fast, reliable, and widely-used connection pooling library. Integrating HikariCP into a Spring Boot application is straightforward as Spring Boot provides built-in support for it.
First, ensure that your pom.xml
file includes the necessary dependencies. If you are using Maven, add the following snippet to include HikariCP:
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>5.0.0</version>
</dependency>
For Gradle users, add the following to your build.gradle
file:
dependencies {
implementation 'com.zaxxer:HikariCP:5.0.0'
}
Next, configure the datasource properties in your application.properties
or application.yml
file. Here is an example configuration in application.properties
:
spring.datasource.url=jdbc:mysql://localhost:3306/yourdatabase
spring.datasource.username=yourusername
spring.datasource.password=yourpassword
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
# HikariCP settings
spring.datasource.hikari.minimum-idle=5
spring.datasource.hikari.maximum-pool-size=20
spring.datasource.hikari.idle-timeout=30000
spring.datasource.hikari.connection-timeout=20000
spring.datasource.hikari.max-lifetime=1800000
And the equivalent configuration in application.yml
:
spring:
datasource:
url: jdbc:mysql://localhost:3306/yourdatabase
username: yourusername
password: yourpassword
driver-class-name: com.mysql.cj.jdbc.Driver
hikari:
minimum-idle: 5
maximum-pool-size: 20
idle-timeout: 30000
connection-timeout: 20000
max-lifetime: 1800000
Choosing the right pool size for your application is crucial for balancing performance and resource usage. Here are some guidelines for setting optimal pool size parameters:
Minimum Idle Connections (spring.datasource.hikari.minimum-idle
): This is the minimum number of idle connections that HikariCP tries to maintain. Setting this value ensures that your application doesn't waste time waiting for a new connection when the load starts increasing.
Maximum Pool Size (spring.datasource.hikari.maximum-pool-size
): This is the maximum number of connections that HikariCP will keep in the pool. If all connections are in use and the max pool size is reached, additional connection requests will be queued until a connection becomes available.
Connection Timeout (spring.datasource.hikari.connection-timeout
): This is the maximum time HikariCP will wait for a connection to be available before throwing an exception. Setting this ensures that your application can handle situations where the database is under heavy load.
Idle Timeout (spring.datasource.hikari.idle-timeout
): This is the maximum amount of time that a connection is allowed to sit idle in the pool. Connections that sit idle for longer than this timeout will be removed from the pool.
Max Lifetime (spring.datasource.hikari.max-lifetime
): This is the maximum lifetime of a connection in the pool. Connections that live longer than this will be closed and removed from the pool.
If you prefer to configure the connection pool programmatically, you can do so by defining a DataSource
bean in your Spring configuration:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.sql.DataSource;
@Configuration
public class DataSourceConfig {
@Bean
public DataSource dataSource() {
HikariConfig hikariConfig = new HikariConfig();
hikariConfig.setJdbcUrl("jdbc:mysql://localhost:3306/yourdatabase");
hikariConfig.setUsername("yourusername");
hikariConfig.setPassword("yourpassword");
hikariConfig.setDriverClassName("com.mysql.cj.jdbc.Driver");
hikariConfig.setMinimumIdle(5);
hikariConfig.setMaximumPoolSize(20);
hikariConfig.setIdleTimeout(30000);
hikariConfig.setConnectionTimeout(20000);
hikariConfig.setMaxLifetime(1800000);
return new HikariDataSource(hikariConfig);
}
}
By following these steps and configuring HikariCP correctly, you can significantly enhance the performance and reliability of your Spring applications. Proper connection pooling not only optimizes resource use but also ensures your application can scale efficiently to accommodate growing user demands.
Effective management of database connections is crucial for ensuring optimal performance and maintaining the reliability of your Spring application. Poor connection management can lead to resource exhaustion, unresponsive applications, and degraded user experiences. Below, we present a comprehensive list of best practices for managing database connections in Spring applications.
Connection timeouts are essential for preventing applications from waiting indefinitely for a database connection. Properly set timeouts ensure that your application gracefully handles situations when connections are not available. Here are the key timeouts to consider:
spring.datasource.hikari.connection-timeout=30000
spring.datasource.hikari.idle-timeout=600000
spring.datasource.hikari.max-lifetime=1800000
Exception handling is vital to maintain application stability. Ensure that exceptions related to database connections are caught and handled appropriately. Common exceptions include SQL exceptions, timeout exceptions, and connection pool exhaustion exceptions.
Use try-catch blocks to manage exceptions and log them for further analysis:
try (Connection connection = dataSource.getConnection()) {
// Perform database operations
} catch (SQLException e) {
// Log exception and take necessary actions
logger.error("Database connection error: ", e);
}
Understanding and managing the lifecycle of database connections help in optimizing and maintaining a healthy pool. Below are some lifecycle events to consider:
Spring's DataSource
can be configured to validate connections:
spring.datasource.hikari.validation-timeout=3000
spring.datasource.hikari.test-query=SELECT 1
Configuring the right pool size is vital for optimizing performance. A pool too small may lead to resource contention, while a pool too large may lead to excessive resource usage. Calculate and set the optimal pool size based on your application's load and database capacity:
spring.datasource.hikari.minimum-idle=10
spring.datasource.hikari.maximum-pool-size=50
Monitoring tools help to keep track of the state and performance of the connection pool. HikariCP provides metrics that can be integrated with monitoring tools like Prometheus and Grafana:
@Configuration
public class HikariMetricsConfiguration {
@Bean
public HikariMetricsTrackerFactory hikariMetricsTrackerFactory() {
return new PrometheusMetricsTrackerFactory();
}
}
Connection leaks can severely impact the performance and stability of your application. Enabling leak detection helps in identifying and resolving these leaks:
spring.datasource.hikari.leak-detection-threshold=2000
For read-only operations, ensure to utilize connections configured specifically for read-only transactions. This helps in load distribution and enhances performance:
@SpringBootApplication
public class MyApplication {
@Bean
@ConfigurationProperties("app.datasource.readonly")
public DataSource readOnlyDataSource() {
return DataSourceBuilder.create().build();
}
@Bean
public JdbcTemplate readOnlyJdbcTemplate(DataSource readOnlyDataSource) {
return new JdbcTemplate(readOnlyDataSource);
}
}
By following these best practices, you can significantly enhance the performance and reliability of your Spring application. Optimizing database connections not only improves response times but also ensures efficient resource utilization and robust application performance.
Effective monitoring and tuning of database connection pools is critical for maintaining the optimal performance of your Spring application. By keeping a close eye on your connection pools, you can identify potential bottlenecks and adjust configurations to better handle varying loads. Here’s a comprehensive guide on how to monitor and tune your database connection pools for peak performance.
Monitoring your connection pools allows you to gain insights into the performance metrics and usage patterns of your database connections. Here are some common methods and tools you can use:
HikariCP, a popular connection pool library, provides built-in metrics that can help you observe the behavior of your connection pool. These metrics can be integrated with monitoring tools like Prometheus, Grafana, or any Java Management Extensions (JMX) based tool.
To enable HikariCP metrics, include the following configuration in your application.properties
or application.yml
:
# application.properties
spring.datasource.hikari.metrics.enabled=true
With this enabled, HikariCP exposes several useful metrics, such as:
Prometheus can be seamlessly integrated with HikariCP to scrape and store metrics. You can set up a Grafana dashboard to visualize these metrics for real-time monitoring. To configure Prometheus to scrape HikariCP metrics, add the following snippet to your prometheus.yml
:
scrape_configs:
- job_name: 'spring-actuator'
scrape_interval: 5s
metrics_path: '/actuator/prometheus'
static_configs:
- targets: ['localhost:8080']
JMX is another powerful tool for monitoring Java applications. HikariCP’s metrics can be exposed via JMX, offering a plethora of diagnostic options. To enable JMX, add the following to your Spring configuration:
# application.properties
spring.datasource.hikari.register-mbeans=true
Once you have a good grasp of your connection pool's performance metrics, you can fine-tune the pool to fit your application’s workload. Here are some key parameters and tips:
Maximum Pool Size (maximumPoolSize
)
Minimum Idle Connections (minimumIdle
)
Connection Timeout (connectionTimeout
)
Idle Timeout (idleTimeout
)
Here’s an example of configuring these parameters in a Spring Boot application:
# application.properties
spring.datasource.hikari.maximumPoolSize=10
spring.datasource.hikari.minimumIdle=5
spring.datasource.hikari.connectionTimeout=30000
spring.datasource.hikari.idleTimeout=600000
Perform Regular Load Testing
Analyze Connection Usage Patterns
Configure Timeouts Appropriately
Monitor for Connection Leaks
By diligently monitoring and fine-tuning your database connection pools, you can significantly enhance the performance and reliability of your Spring application.
Connection leaks are one of the most insidious performance issues in any database-backed application, including those built on Spring. These occur when database connections are not released back to the pool after usage, resulting in an exhausted pool and subsequent connection failures. This section will discuss common causes of connection leaks, how to detect them, and best practices to resolve them effectively in Spring applications.
To resolve connection leaks, it’s crucial first to detect them. Here are some techniques:
One effective way to detect leaks in HikariCP is by setting a leak detection threshold. When a connection in the pool is not closed within the specified time, a stack trace is logged to help identify where the leak is occurring.
spring.datasource.hikari.leak-detection-threshold=2000 // in milliseconds
Regularly monitor your connection pool metrics to spot any unusual patterns such as a high number of active connections or low available connections. Tools like Prometheus and Grafana can be integrated to visualize these metrics.
management:
endpoints:
web:
exposure:
include: "hikaricp"
try-with-resources
StatementJava's try-with-resources
statement helps ensure that each resource is closed at the end of the statement. It’s the most straightforward way to manage connections.
try (Connection conn = dataSource.getConnection()) {
// Your database operations
} catch (SQLException e) {
// Handle exceptions
}
If not using try-with-resources
, ensure connections are closed in a finally
block to guarantee they are returned to the pool even if an exception is thrown.
Connection conn = null;
try {
conn = dataSource.getConnection();
// Your database operations
} catch (SQLException e) {
// Handle exceptions
} finally {
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
// Handle close exception
}
}
}
Set appropriate timeouts to manage long-lived transactions and async tasks effectively. Ensure connections are only held as long as necessary.
spring.datasource.hikari.connection-timeout=30000 // in milliseconds
Logging can be configured to provide detailed insights into the lifecycle of your database connections.
HikariCP can log connection acquisition and release events, which are useful for tracing connection usage patterns.
logging.level.com.zaxxer.hikari=DEBUG
Implement custom logging in your DAO or repository classes to log when connections are opened and closed.
// Inside your data access method
Logger logger = LoggerFactory.getLogger(YourClass.class);
try (Connection conn = dataSource.getConnection()) {
logger.debug("Connection opened: " + conn);
// Your database operations
logger.debug("Connection will be closed: " + conn);
} catch (SQLException e) {
// Handle exceptions
}
Effectively managing database connections is paramount to maintaining the performance and stability of your Spring applications. By understanding the causes, detection mechanisms, and resolutions for connection leaks, you can significantly reduce the risk of pool exhaustion and improve overall application performance. Implement the practices discussed in this section meticulously to ensure your connection pools are robust, efficient, and leak-free.
Optimizing read-only transactions is crucial for maintaining a high-performance Spring application. This section delves into strategies for configuring database connections specifically for read-only operations, and the advantages of implementing dedicated read-only data sources.
When dealing with transactions that only read data and do not modify it, you should explicitly mark them as read-only. This can improve performance and, in some cases, help the database engine optimize query execution.
In Spring, you can specify read-only transactions at a method level using the @Transactional
annotation. For example:
@Service
public class UserService {
@Transactional(readOnly = true)
public User findUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
}
A read-only data source can be configured to facilitate operations that don't require write access, helping balance the load more efficiently across your database resources. Here are some advantages:
To implement read-only data sources in a Spring application, consider the following approach:
DataSource
beans for the primary (write) and read-only (replica) databases.@Configuration
public class DataSourceConfig {
@Bean
@Primary
@ConfigurationProperties("spring.datasource.primary")
public DataSource primaryDataSource() {
return DataSourceBuilder.create().build();
}
@Bean
@ConfigurationProperties("spring.datasource.readonly")
public DataSource readOnlyDataSource() {
return DataSourceBuilder.create().build();
}
}
DataSource
to dynamically switch between the primary and read-only data sources based on the transaction type.public class RoutingDataSource extends AbstractRoutingDataSource {
@Override
protected Object determineCurrentLookupKey() {
return TransactionSynchronizationManager.isCurrentTransactionReadOnly() ? "readOnly" : "primary";
}
}
RoutingDataSource
to route based on the transaction context.@Configuration
public class DataSourceRoutingConfig {
@Bean
public DataSource dataSource() {
RoutingDataSource routingDataSource = new RoutingDataSource();
Map<Object, Object> dataSourceMap = new HashMap<>();
dataSourceMap.put("primary", primaryDataSource());
dataSourceMap.put("readOnly", readOnlyDataSource());
routingDataSource.setTargetDataSources(dataSourceMap);
routingDataSource.setDefaultTargetDataSource(primaryDataSource());
return routingDataSource;
}
}
@Transactional(readOnly = true)
to clearly indicate the read-only nature.Properly configuring read-only transactions and implementing dedicated read-only data sources can greatly enhance the performance and scalability of your Spring application. By distributing read and write operations efficiently, you can achieve better load balancing, higher throughput, and improved database reliability.
Effective session management is crucial for optimizing database interaction in Spring applications. Properly managing sessions ensures that resources are efficiently utilized, transactions are handled correctly, and overall application performance is enhanced. In this section, we will cover best practices for session management, including the importance of correctly opening and closing sessions within transactions.
In Spring, database interactions are often wrapped within transactions to ensure data integrity and consistency. Spring's transaction management capabilities allow you to demarcate the boundaries of transactions programmatically or declaratively.
Declarative transaction management, achieved using the @Transactional
annotation, is preferred for its simplicity and readability. Here is an example:
@Service
public class TransactionalService {
@Transactional
public void transactionalMethod() {
// Business logic involving database operations
}
}
In this example, the @Transactional
annotation ensures that all database operations within transactionalMethod
are part of a single transaction. If any operation fails, the transaction will be rolled back, maintaining data integrity.
Optimize Session Scope: The default scope of a Hibernate session is the duration of a transaction. It’s essential to keep the scope of sessions as short as possible to reduce resource contention and improve performance.
Explicit Session Management:
In some cases, explicit session management is needed. For instance, when dealing with deferred execution. Use Spring’s SessionFactory
to manage sessions manually:
@Autowired
private SessionFactory sessionFactory;
public void manageSessionManually() {
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
try {
// Database operations
tx.commit();
} catch (Exception e) {
if (tx != null) tx.rollback();
throw e;
} finally {
session.close();
}
}
Avoid Long Transactions: Long-running transactions hold locks and can lead to contention, deadlocks, and resource exhaustion. Break long transactions into smaller ones and use batching for bulk operations if necessary.
Use Connection Pooling: Integrate connection pooling (e.g., HikariCP) to manage the lifecycle of connections efficiently. This helps in reducing the overhead associated with opening and closing connections frequently.
Proper Session Closing: Always ensure sessions are closed after operations are complete to release database connections back to the pool. This can be achieved manually or by using try-with-resources statements:
try (Session session = sessionFactory.openSession()) {
Transaction tx = session.beginTransaction();
// Database operations
tx.commit();
} catch (Exception e) {
// Handle exception
}
Transaction Timeouts: Set appropriate transaction timeouts to avoid long waits and potential deadlocks. This can be configured declaratively:
@Transactional(timeout=5) // 5 seconds timeout
public void transactionalMethod() {
// Business logic involving database operations
}
Spring and Hibernate support lazy loading, which defers fetching associated entities until they are actually needed. However, if the session scope is not managed correctly, lazy loading can lead to LazyInitializationException
. To avoid this:
Use @Transactional
:
Ensure the session is active for the duration of lazy loading by wrapping the operations within a @Transactional
method.
DTOs and Eager Fetching: Consider using Data Transfer Objects (DTOs) or configuring eager fetching strategies where appropriate to avoid lazy loading issues.
By following these best practices for session management, you can significantly enhance the database interaction performance of your Spring applications. Proper transaction management, optimized session scope, and efficient resource utilization are key to maintaining robust and performant database operations.
## Load Testing Database Performance
Understanding how your database performs under varying loads is crucial for maintaining the stability and efficiency of your Spring application. Load testing allows you to simulate different user scenarios and evaluate how well your database manages these situations. This section delves into the importance of load testing and introduces you to LoadForge, an essential tool for load testing your Spring application's database connections.
### The Importance of Load Testing
Load testing helps identify bottlenecks, performance degradation, and potential downtimes under stress conditions. Here are a few key reasons why load testing is significant:
- **Capacity Planning**: Determine how many concurrent users your database can handle before performance starts to degrade.
- **Bottleneck Identification**: Pinpoint slow queries and inefficient database operations.
- **Scalability Verification**: Ensure your database scaling strategies are effective.
- **Resilience Testing**: Evaluate how your system behaves under high load, including recovery times post-failure.
### Introducing LoadForge
LoadForge is a powerful load testing tool tailored for modern web applications, including those built with Spring. By simulating real-world traffic and environmental conditions, LoadForge helps you understand your application's behavior under various load scenarios.
### Using LoadForge for Load Testing Database Connections
To get started with load testing your Spring application using LoadForge, follow these steps:
1. **Sign Up and Set Up**: Create an account on the LoadForge platform and set up a new load testing project.
2. **Define Test Scenarios**: Configure test scenarios to simulate different levels of database connection load. This can include simulating peak user loads, concurrent transactions, and specific query loads.
3. **Execute Load Tests**: Run the load tests and use LoadForge’s comprehensive reporting features to gather performance data.
4. **Analyze Results**: Examine the results to identify any performance issues, such as connection pool exhaustion, slow query performance, or high latency.
#### Sample Load Test Configuration
Here's a sample configuration for LoadForge to simulate 1000 concurrent users, each making multiple database requests:
<pre><code>
loadTest:
name: "SpringApp Database Load Test"
scenario:
rampUp: 5m
holdFor: 30m
rampDown: 5m
users: 1000
steps:
- type: http
method: GET
url: "https://your-spring-app/api/endpoint"
headers:
Authorization: "Bearer YOUR_ACCESS_TOKEN"
checks:
- type: response
status: 200
</code></pre>
### Key Metrics to Monitor
When analyzing the performance data from LoadForge, pay close attention to these key metrics:
- **Response Time**: Time taken for the database to respond to queries.
- **Throughput**: Number of transactions processed per second.
- **Error Rate**: Percentage of failed or problematic queries.
- **Connection Pool Usage**: Utilization levels of your database connection pool.
- **Latency**: Delays in data processing and query execution.
### Tips for Effective Load Testing
- **Start Small**: Gradually increase the load to understand how your application performs under different conditions.
- **Simulate Real-World Scenarios**: Design test scenarios that mirror real user behavior and traffic patterns.
- **Monitor Continuously**: Regularly perform load testing, especially after significant changes to your application or infrastructure.
- **Automate Testing**: Utilize tools like LoadForge’s scripting capabilities to automate and schedule regular load tests.
### Conclusion
Load testing with LoadForge is an invaluable step in optimizing your Spring application's database performance. By rigorously testing and analyzing how your database handles various load conditions, you can ensure your application remains robust, responsive, and scalable, ultimately delivering a better experience to your users.
## Conclusion and Summary
Optimizing database connections is a cornerstone for the performance and scalability of Spring applications. Poor connection management can lead to a myriad of performance issues, such as thread bottlenecks, application latency, and even downtime. Throughout this guide, we explored several key aspects of database connection optimization to ensure your Spring application runs efficiently and scales appropriately.
### Key Takeaways
1. **Importance of Database Connection Optimization**:
- Effective management of database connections enhances application responsiveness and stability.
- Poor connection handling can severely impact application performance and user experience.
2. **Connection Pooling**:
- Utilizes a pool of pre-established database connections to efficiently manage and reuse connections.
- Libraries like HikariCP simplify the implementation of connection pooling and are essential for scaling Spring applications.
3. **Configuring Connection Pool in Spring**:
- Integrating HikariCP into Spring Boot applications is straightforward and offers significant performance benefits.
- Properly setting connection pool parameters such as minimumIdle, maximumPoolSize, and connectionTimeout ensures optimal resource utilization.
<pre><code>
spring.datasource.hikari.minimum-idle=5
spring.datasource.hikari.maximum-pool-size=20
spring.datasource.hikari.connection-timeout=30000
</code></pre>
4. **Best Practices for Managing Database Connections**:
- Setting appropriate timeouts and handling exceptions gracefully prevent application stalls.
- Proper management of connection lifecycle events is critical for maintaining efficient database interactions.
5. **Monitoring and Tuning Database Connection Pools**:
- Regularly monitor connection pool metrics and use profiling tools to identify performance bottlenecks.
- Iteratively tune connection pool settings based on real-time data for maximum efficiency.
6. **Handling Connection Leaks**:
- Connection leaks can be mitigated by ensuring all connections are closed after use and by implementing proper logging strategies.
- Tools and libraries can assist in detecting and addressing connection leaks effectively.
7. **Read-Only Transactions and Connection Strategies**:
- Leveraging read-only transactions where applicable reduces load on the primary database.
- Consider implementing read-only data sources to segregate reads from writes for better performance.
8. **Session Management for Improved Database Interaction**:
- Proper session management within transactions ensures efficient and cohesive database interactions.
- Opening and closing sessions correctly reduces the risk of performance issues and resource leaks.
9. **Load Testing Database Performance**:
- Load testing is crucial for understanding how your database handles varying loads and identifying potential performance issues before they impact users.
- Using LoadForge facilitates robust load testing practices, ensuring your application's database connection management is sound under stress.
### Final Thoughts
Optimizing database connections in Spring applications is not just about improving performance—it’s about ensuring the scalability and reliability of your entire application stack. By implementing the strategies and best practices discussed in this guide, you can significantly enhance your application's efficiency and user experience. Load testing with tools like LoadForge should be an integral part of your optimization workflow, giving you the confidence to scale seamlessly.
Remember, consistent monitoring, tuning, and adherence to best practices are key to maintaining an optimized database connection environment. Your users will thank you for the increased performance and reliability, and your application will be well-poised to handle growth and varying loads effectively.