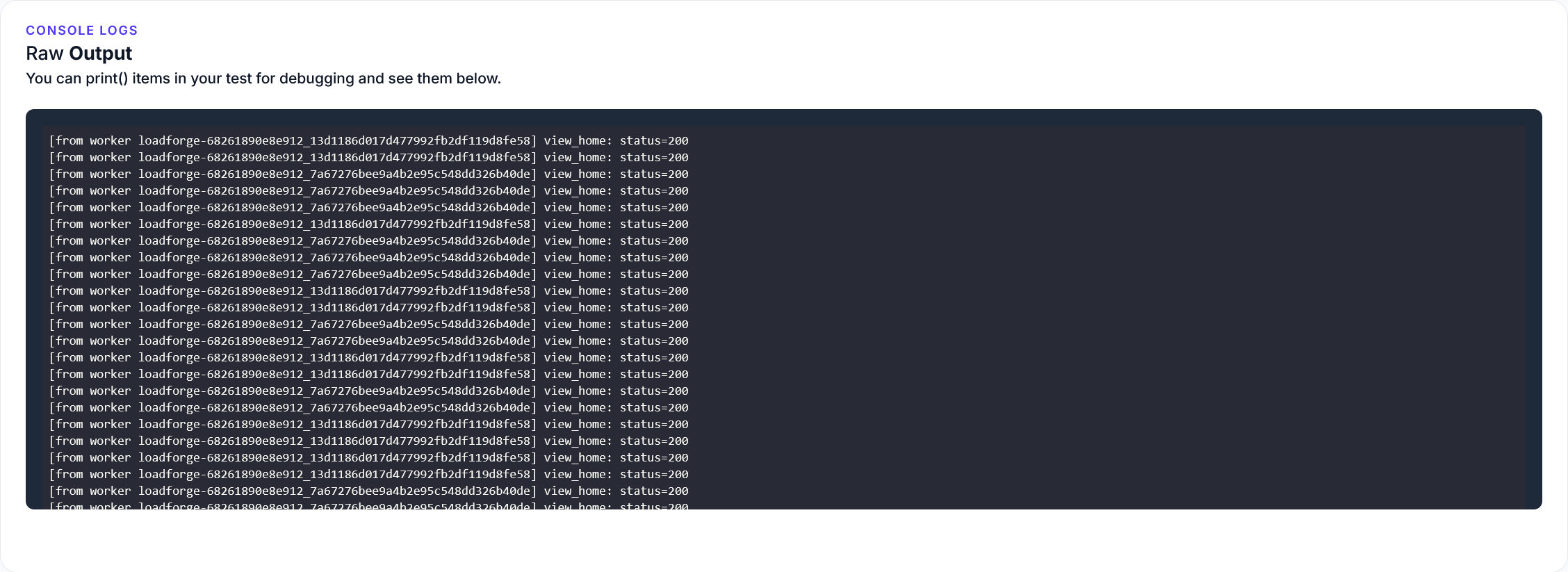
Easier Debug Logging
We're excited to announce a powerful new feature for LoadForge users: comprehensive debug logging for test runs. This highly requested...
In the realm of PHP development, performance optimization plays a crucial role in ensuring that applications serve requests swiftly and efficiently. One of the key tools in optimizing PHP applications is the Opcode Cache, commonly known as OpCache. This feature...
In the realm of PHP development, performance optimization plays a crucial role in ensuring that applications serve requests swiftly and efficiently. One of the key tools in optimizing PHP applications is the Opcode Cache, commonly known as OpCache. This feature significantly enhances PHP performance by eliminating the need for PHP scripts to be compiled on every request.
OpCache improves PHP performance by storing precompiled script bytecode in shared memory, thereby removing the need for PHP to load and parse scripts on each request. This mechanism helps in reducing the execution time and increasing the responsiveness of PHP applications.
The compilation of PHP scripts can be resource-intensive, particularly for complex applications. By caching the compiled bytecode, OpCache eliminates the compilation overhead, which can lead to substantial performance gains. These enhancements are particularly noticeable in high-traffic environments where the same PHP scripts are requested frequently.
OpCache works by intercepting PHP's compilation process. When a PHP script is executed for the first time, OpCache takes the PHP code that is usually compiled into opcodes (operation codes), and stores it in the cache. For subsequent executions, PHP fetches this cached opcode hence bypassing the compilation phase.
Here’s a simple illustration of how OpCache processes PHP scripts:
<?php
// Sample PHP script
echo "Hello, World!";
?>
Without OpCache, the above script would need to be compiled into bytecode every time it is requested. With OpCache enabled, the bytecode is compiled and cached during the first request, speeding up all subsequent requests.
By compiling PHP script bytecode into optimized execution commands that are stored for quick retrieval, OpCache substantially cuts down the time and resources associated with executing PHP scripts. This introduction sets the stage for further exploration into how to enable, configure, and effectively utilize OpCache to achieve optimal performance in PHP applications. The following sections will delve deeper into how to harness the full potential of OpCache through proper configuration, monitoring, and troubleshooting practices.
OpCache improves PHP performance by storing precompiled script bytecode in shared memory, thereby eliminating the need for PHP to load and parse scripts on each request. To enable OpCache on your server, you will need to edit your PHP configuration file (php.ini
). This file is typically located in the php
directory of your server, but its location can vary depending on your operating system and server setup.
To enable OpCache, open your php.ini
file and search for the following line:
;zend_extension=opcache.so
For Windows servers, the extension might be named opcache.dll
. Remove the semicolon (;
) at the beginning of the line to uncomment it, thus enabling the extension:
zend_extension=opcache.so
After enabling the extension, restart your web server for the changes to take effect. For Apache, you can usually restart it using:
sudo service apache2 restart
For Nginx:
sudo service nginx restart
Once OpCache is enabled, you can configure it to optimize its performance. Here are some of the key OpCache configuration directives and what they control:
opcache.memory_consumption
opcache.memory_consumption=128
opcache.interned_strings_buffer
opcache.interned_strings_buffer=8
opcache.max_accelerated_files
opcache.max_accelerated_files=10000
opcache.revalidate_freq
0
, OpCache will check for updates on every request, which might degrade performance under heavy load. A practical value could be 2
seconds.opcache.revalidate_freq=2
opcache.enable_cli
0
), but enabling it (1
) can improve performance for CLI-based applications like cron jobs.opcache.enable_cli=1
Once you have made the necessary changes to your php.ini
file, you can verify that OpCache is configured correctly by creating a simple PHP info file and checking the output in a web browser:
phpinfo.php
with the following content:
<?php phpinfo(); ?>
By properly configuring and enabling OpCache, your PHP applications can see significant improvements in performance due to reduced compile times and lower CPU usage. This setup ensures that your applications can handle higher traffic volumes more efficiently while maintaining fast response times.
Optimizing the configuration of OpCache is crucial for maximizing the performance of your PHP applications. This section outlines recommended practices for tuning OpCache to achieve optimal performance, focusing on key configuration directives, cache resetting, and effective memory management.
Several directives in the php.ini
file must be tuned for optimal performance:
opcache.memory_consumption
: This directive controls the amount of memory OpCache uses to store compiled scripts. A higher value increases the number of files that can be cached, reducing compilation frequency and improving performance. A typical setting might be 128 MB or 256 MB, depending on your application's size and your server's total memory.
opcache.memory_consumption=256
opcache.interned_strings_buffer
: This setting allocates memory for interned strings—a method PHP uses to optimize resource usage by storing identical strings in a single buffer. Depending on your application, increasing this can significantly reduce memory overheads.
opcache.interned_strings_buffer=16
opcache.max_accelerated_files
: Determines the maximum number of PHP files, which can be stored in the cache. Set this number based on the count of PHP script files on your server. A sensible value could be calculated (e.g., 10000 if you have a large application).
opcache.max_accelerated_files=10000
Resetting the OpCache is sometimes necessary, especially during development or when deploying updates to ensure that changes are reflected immediately:
Development Environment: Use opcache_reset()
function in your PHP script to clear the cache programmatically when scripts change frequently.
Production Environment: Consider using automatic cache reset settings upon updates. This can be managed via deployment scripts or hooks that call opcache_reset()
.
Proper memory management ensures that OpCache runs efficiently without exhausting server resources:
Monitoring Usage: Regularly check memory usage to adjust the memory_consumption
setting. Use tools like opcache_get_status()
for detailed insight into OpCache memory usage.
$status = opcache_get_status();
echo $status['memory_usage']['used_memory'];
Avoiding Cache Saturation: Ensure that memory_consumption
and max_accelerated_files
are balanced so that the cache does not become saturated. Saturated cache leads to cache eviction, which can degrade performance.
Consistent Optimization: Consistency in performance can be maintained by periodical review and tweaking of OpCache settings based on ongoing monitoring results.
Adopting these best practices for configuring OpCache can lead to significant improvements in your PHP application's performance. Continuously monitor and tweak the settings as your application scales and as server load varies to maintain optimal efficiency.
Effective monitoring is key to maximizing the performance benefits from PHP OpCache. This helps in understanding how well the cache is functioning and aids in proactive optimization to avoid potential issues. By leveraging both built-in tools within PHP and utilizing third-party monitoring solutions, you can gain valuable insights into OpCache’s effectiveness and efficiency.
PHP offers several built-in functions that can provide a snapshot of OpCache's status and its current metrics. The primary tool is opcache_get_status()
, which retrieves an array of information about OpCache's state and statistics.
Here’s a simple example of how to use this function:
<?php
$status = opcache_get_status(false);
echo "<pre>";
print_r($status);
echo "</pre>";
?>
This function returns extensive data, including:
These details help in assessing the utilization and performance impacts of OpCache, enabling administrators to tweak configurations based on actual usage patterns.
While the built-in status functions provide substantial data, integrating with a full-fledged monitoring solution allows for continuous tracking, historical data analysis, and more sophisticated insights. Tools such as New Relic and Datadog offer PHP monitoring capabilities, and configuring them to include OpCache data involves additional setup steps specific to each tool.
For example, setting up OpCache monitoring with New Relic involves:
Graphical visualization of OpCache metrics can significantly enhance your ability to interpret performance data quickly. Tools like Grafana can be used in conjunction with time-series databases like Prometheus to create intuitive dashboards that display:
Additionally, configuring alerting based on certain thresholds (like high memory usage or low hit rates) can preempt performance bottlenecks before they impact your application.
Monitoring the performance of OpCache is not just about ensuring it is functioning but optimizing its setup to suit the specific needs of your application. Regular monitoring using the built-in functions along with an integration of third-party tools can provide a comprehensive view, ensuring your PHP applications run at their peak potential. By tweaking settings based on real-time and historical performance data, you can ensure efficient resource utilization and consistent application performance.
When working with OpCache to enhance PHP performance, several common issues may arise. This section provides advice on identifying and resolving these frequent problems, focusing on cache stampeding, inconsistency in performance, and script caching issues.
Cache stampeding occurs when many requests for a single piece of data arrive simultaneously to an empty cache, causing multiple executions of slow backend processes.
How to Identify:
How to Resolve:
Example of enabling lock mechanism in PHP:
opcache.enable_file_override=1
Performance inconsistency may arise due to improper configuration or conflicts between the cached scripts.
How to Identify:
How to Resolve:
opcache.revalidate_freq
and opcache.validate_timestamps
to ensure proper balance between performance and dynamic PHP script updates.opcache.memory_consumption
setting if frequent cache eviction is noticed.These issues typically occur when scripts are not properly cached or prematurely evicted from the cache.
How to Identify:
How to Resolve:
opcache.max_accelerated_files
is adequately sized to hold all your PHP scripts. Increase it if necessary.opcache.interned_strings_buffer
and opcache.memory_consumption
values to ensure they are set high enough to store all necessary scripts and strings without frequent evictions.Configuring OpCache properly:
opcache.max_accelerated_files=10000
opcache.memory_consumption=256
opcache.interned_strings_buffer=16
To prevent these issues from cropping up, regular monitoring and maintenance of the OpCache configuration and performance are crucial:
opcache_get_status()
.By being proactive and aware of how to troubleshoot these common issues, you can ensure that OpCache runs smoothly and continues to provide significant improvements to your PHP application's performance.
When dealing with high traffic websites and applications, optimizing your PHP performance through OpCache can drastically reduce response times and resource consumption. However, as we scale our environments to accommodate greater loads, there are advanced techniques that can be implemented to enhance the efficiency of OpCache further, particularly in cluster environments and when integrating with other technologies. Here, we will explore some of these sophisticated strategies.
In cluster environments, where multiple servers are set up to handle the application load, it’s crucial that OpCache settings are synchronized across all nodes to avoid inconsistencies.
Shared Cache: One effective approach is using a shared file system for the cached scripts. Although OpCache is inherently local (caching files in the memory of the individual server), configurations can be adapted so that all cluster nodes point to a centralized cache storage. However, this might introduce latency due to network file system overheads.
Cache Consistency: To maintain consistency across nodes, it's advised to deploy new changes in an atomic way. This often involves clearing the OpCache at the same time across all nodes, which can be managed via a deployment script that ssh's into each node and resets the OpCache.
# Sample script to reset OpCache across multiple servers
for server in server1 server2 server3; do
ssh $server "php -r 'opcache_reset();'"
done
Staggered Resets: To avoid a massive performance hit from resetting the cache simultaneously across all servers, consider using staggered cache resets where each node’s cache is reset at slightly different times.
OpCache does not have to function in isolation; it can be combined with other performance-enhancing technologies to maximize PHP application efficiency:
Content Delivery Networks (CDNs): While OpCache optimizes PHP execution on the server-side, using a CDN can help offload static content (CSS, JS, Images) delivery to a distributed network, reducing the overall load on your application servers.
Database Caching: Technologies like Redis or Memcached can be employed for database query result caching, which complements the OpCache by reducing database load and speeding up data retrieval.
Full-Page Caching: Tools like Varnish can be used to cache entire pages in memory. When integrated with PHP OpCache, this strategy allows for not just faster script execution but also quicker content delivery, making it extremely effective for high-traffic sites.
Real-User Monitoring (RUM): Integrating RUM can help in assessing the impact of OpCache optimization in real-world scenarios by providing insights into actual performance experienced by end-users, helping further tune the settings.
Advanced OpCache techniques involve thoughtful integration and strategic configuration adjustments, particularly in complex environments like multi-server clusters. By employing comprehensive strategies that include other performance technologies, OpCache can significantly contribute to reducing latency, improving load times, and ultimately enhancing user satisfaction. As with any optimization technique, monitoring and continuous improvement are key to achieving the best results.
In this section, we explore various real-world scenarios where enabling and tuning PHP OpCache has significantly improved the performance of PHP applications. These case studies highlight the practical benefits of optimizing OpCache settings, demonstrating its impact on reducing response times and enhancing server throughput.
Background: A popular e-commerce website was experiencing slow loading times during peak traffic hours, which negatively affected customer experience and sales.
Challenge: The platform was built on PHP and used complex scripts that were CPU-intensive, leading to high TTFB (Time to First Byte) and overall page load times.
Solution: The development team enabled OpCache and optimized its configuration:
opcache.memory_consumption
from the default 64MB to 256MB.opcache.max_accelerated_files
to 10000 to cover all the platform's scripts.opcache.revalidate_freq
to 60 seconds to balance between performance and script update needs.Results: After implementing the changes, the website saw a 50% reduction in TTFB and a 40% faster page load time during high traffic periods. These enhancements contributed to a better user experience and an increase in conversion rates.
Background: An API developed in PHP for a mobile application was struggling with latency issues, particularly when handling simultaneous requests from numerous users.
Challenge: The server was recalculating the same scripts repeatedly, leading to unnecessary processing and increased response times.
Solution: The API's backend adopted OpCache with specific settings tailored to an API environment:
opcache.memory_consumption
to 128MB to accommodate the script size.opcache.interned_strings_buffer
to 16MB to optimize string storage.opcache.validate_timestamps
set to 0
during production to avoid constant disk checks.Results: These adjustments decreased the average API response time by 65%, greatly improving the experience for end-users and reducing server load.
Background: A CMS used by a news portal was slow, affecting the editorial team's ability to publish content swiftly.
Challenge: High server load during peak news hours and inefficient script execution.
Solution: Implementation of OpCache with a focus on high traffic scenarios:
opcache.memory_consumption
was increased to 192MB.opcache.max_accelerated_files
set to 8000, covering all CMS and custom modules.opcache_reset()
) during low-traffic hours to maintain cache freshness.Results: Post-optimization, the CMS showed a performance improvement of over 35% in backend operations and a 25% faster page load for readers, significantly enhancing both editor and visitor satisfaction.
These case studies illustrate the dramatic enhancements that can be achieved by properly configuring and managing PHP OpCache. Optimizing OpCache not only boosts the performance of PHP applications but also improves resource utilization, thereby supporting scalability and reliability in dynamic and high-traffic environments. By understanding and implementing the right OpCache settings, developers and system administrators can significantly uplift the efficiency of their PHP applications.