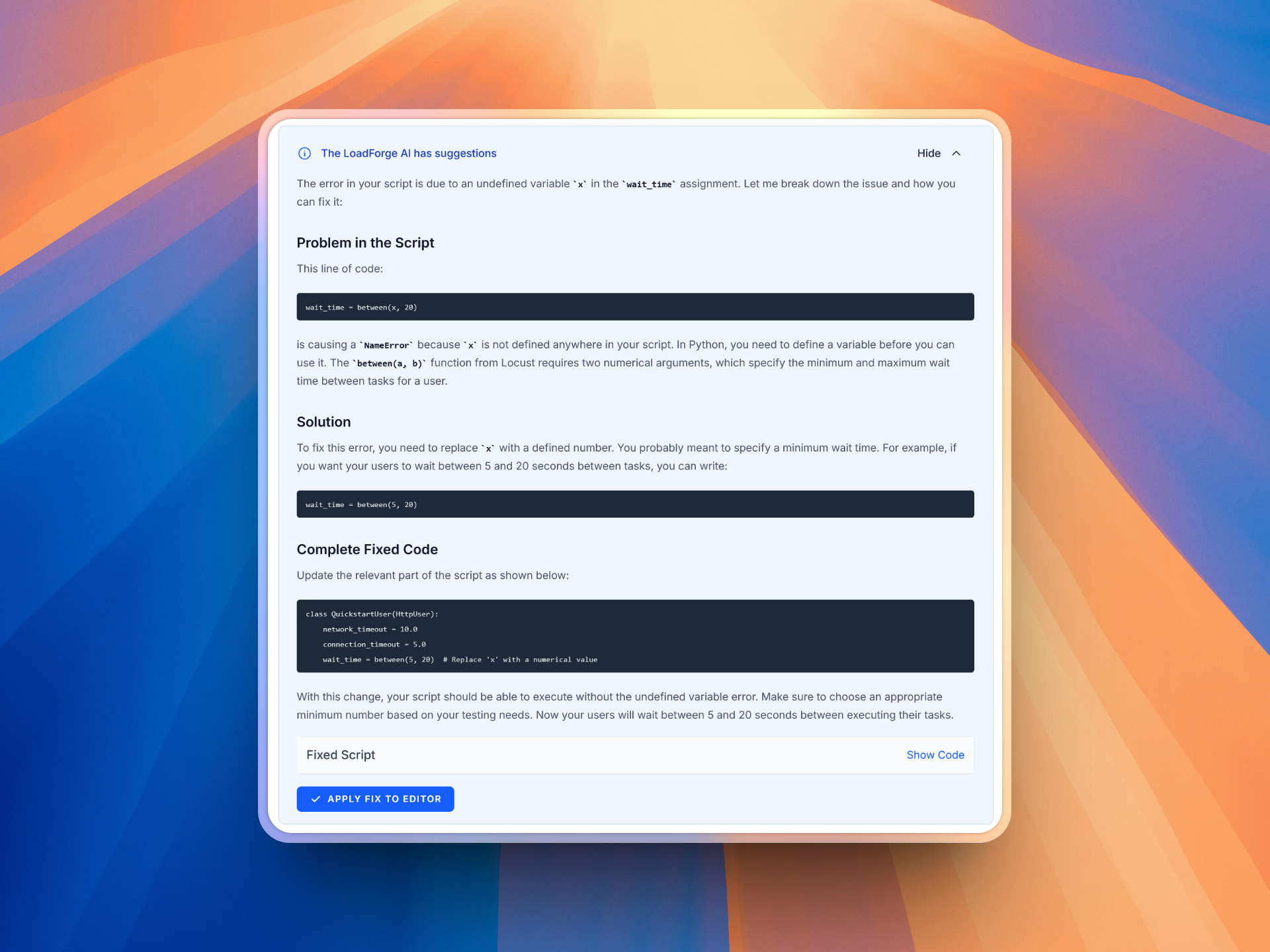
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
In the realm of database management, MySQL stands out as one of the most popular relational database management systems. Its importance cannot be understated, particularly in applications ranging from small-scale projects to large, high-traffic web applications. Effective MySQL performance tuning...
In the realm of database management, MySQL stands out as one of the most popular relational database management systems. Its importance cannot be understated, particularly in applications ranging from small-scale projects to large, high-traffic web applications. Effective MySQL performance tuning can drastically enhance the responsiveness of applications, leading to improved user experiences and reduced server costs. This section aims to illuminate the pivotal role of MySQL performance, setting the groundwork for advanced tuning techniques discussed in subsequent sections of this guide.
MySQL’s performance impacts several facets of technology infrastructure:
The performance of MySQL can be the bottleneck in many applications, particularly those which are data-intensive or have high concurrency requirements. The database's ability to handle multiple connections, execute queries swiftly, and return responses without delay is critical. Performance issues in MySQL can lead to slow page loads, timeout errors, and in worst-case scenarios, system crashes. These issues not only degrade user experience but can also affect an organization's reputation and revenue.
Throughout this guide, we will explore various aspects of MySQL performance tuning, including:
The forthcoming sections will delve deeply into each area, equipped with practical examples and best practices. This holistic approach will furnish you with the knowledge to not only solve immediate performance issues but also to strategize long-term improvements for scaling and maintaining your MySQL environments effectively.
By understanding and applying the performance tuning principles discussed in this guide, developers and database administrators can ensure that their MySQL instances are robust, efficient, and ready to meet the challenges of modern web and enterprise applications.
Indexing is one of the fundamental aspects of database optimization in MySQL. Proper indexing strategy drastically improves the performance of a database system by enabling quicker data retrieval operations, thereby reducing the load on database resources. This section explores the different types of indexes available in MySQL, their importance, and appropriate scenarios for their application.
MySQL supports several types of indexes that cater to different needs and scenarios. Here are the most commonly used:
Primary Key Index: Automatically created when a primary key is defined. There is only one primary key index per table, enforcing uniqueness for the column or set of columns.
Unique Index: Ensures that all values in the index are unique. They are used not only to enforce uniqueness but to enhance search performance.
Index on Foreign Key: Used in association with foreign key constraints, aiding in quick join operations and maintaining referential integrity.
Full-text Indexes: Designed for full-text searches. Only available for CHAR
, VARCHAR
, and TEXT
columns.
Composite Indexes: These indexes involve multiple columns, useful for queries involving multiple columns.
Spatial Indexes: Used for spatial data such as geometry, and are useful for queries that involve spatial operations.
Indexes are vital for enhancing database performance:
Understanding when and how to use indexes can greatly improve the efficiency of MySQL operations:
To efficiently use indexes in MySQL, follow these best practices:
Given these insights into MySQL indexing, database administrators and developers can make informed decisions on how to best implement and utilize indexes for their specific needs, balancing load and optimizing the performance of their database systems.
Optimizing SQL queries is crucial to enhancing the performance of a MySQL database. Effective query optimization can significantly reduce server load, decrease response times, and increase throughput. In this section, we discuss best practices for writing optimized SQL queries and highlight common pitfalls to avoid in query design.
Rather than using SELECT *
, which retrieves all columns from a table, specify only the columns you need. This reduces the amount of data that MySQL has to process and transfer over the network, which can substantially decrease query times.
Example:
SELECT firstName, lastName FROM users;
Using explicit joins, rather than implicit syntax, can make queries more readable and often more efficient. It also helps prevent Cartesian joins that can occur due to missing join conditions.
Example:
SELECT users.name, orders.amount
FROM users
INNER JOIN orders ON users.id = orders.user_id;
Ensure that columns used in WHERE
, JOIN
, ORDER BY
, and GROUP BY
clauses are indexed. Correct indexing can dramatically speed up data retrieval times by allowing the database engine to quickly locate the data without scanning through every row in a table.
Applying functions on indexed columns in your WHERE clause can negate the performance benefit of indexing. Avoid using functions on indexed columns in conditions unless necessary.
Example:
// Less efficient
SELECT * FROM users WHERE DATE(createdAt) = '2021-01-01';
// More efficient
SELECT * FROM users WHERE createdAt >= '2021-01-01' AND createdAt < '2021-01-02';
Subqueries can often be replaced with joins, which are usually more efficient. If you must use a subquery, ensure it is optimized and does not run for each row of the main query.
When you only need a specific number of rows from a query, use the LIMIT
clause to restrict the numbers of rows returned. This is particularly useful for queries with potentially large result sets.
Example:
SELECT * FROM users LIMIT 10;
When using LIKE
statements, avoid leading wildcards, which can result in full table scans. If a leading wildcard is necessary, consider full-text searches for larger datasets.
Example:
// Avoid
SELECT * FROM users WHERE username LIKE '%smith';
// Better
SELECT * FROM users WHERE username LIKE 'smith%';
Minimize the overhead by combining multiple queries into a single query, where appropriate, and by avoiding repeated queries in loops.
Make use of the EXPLAIN
statement to gain insight into how MySQL executes your SQL queries. This can help spot inefficiencies and potential optimizations in the execution plan.
Example:
EXPLAIN SELECT * FROM users WHERE id = 101;
Query optimization is both an art and a science, requiring a deep understanding of both the data you're working with and how MySQL handles queries. By following these best practices, you can achieve significant improvements in your database's performance and scalability.
Configuring your MySQL server correctly can dramatically enhance the performance of your database system. This section outlines essential settings and parameters you should tune based on the workload and specific requirements of your applications. Proper tuning of these configurations helps in optimizing resource utilization, reducing latency, and speeding up data retrieval.
This setting defines the amount of memory allocated to InnoDB for caching data and indexes of your tables. It's one of the most crucial settings for InnoDB performance. The general recommendation is to set this parameter to up to 70% of your total RAM on a dedicated database server.
[mysqld]
innodb_buffer_pool_size = 10G # Adjust size according to your server's RAM
This parameter determines the maximum number of connections that MySQL can handle simultaneously. Setting this value too low could cause “Too many connections” errors, while too high a value might lead to excessive memory use.
[mysqld]
max_connections = 150 # Depends on your application's requirement
For versions of MySQL before 8.0, setting the query_cache_size
could improve performance for certain workloads. This setting specifies the amount of memory allocated for caching the results of old queries.
[mysqld]
query_cache_size = 256M
This parameter indicates the maximum number of open tables in the cache. Increasing this value can increase the performance when you have many tables.
[mysqld]
table_open_cache = 2000
These settings define the maximum size of internal in-memory temporary tables. If an in-memory table exceeds this size, MySQL will automatically convert it to an on-disk table.
[mysqld]
tmp_table_size = 32M
max_heap_table_size = 32M
Proper management of client connections is critical. Utilize the back_log
and wait_timeout
parameters to manage connection queues and how long the server should wait for a non-interactive connection before closing it.
[mysqld]
back_log = 100
wait_timeout = 300 # 300 seconds
MySQL supports multiple storage engines, each suitable for different types of workloads. The most commonly used storage engines are InnoDB and MyISAM. InnoDB supports transactions and is preferred for high-volume read and write operations. MyISAM, on the other hand, is simpler and could perform faster in read-mostly scenarios. Choosing the right storage engine based on your need is an easy yet effective tweak:
[mysqld]
default-storage-engine = InnoDB
These tweaks are starting points and should be adjusted based on the specific performance metrics and workload patterns observed. Use tools like MySQL Workbench or command-line utilities to monitor the impact of your changes. Moreover, consider regular load testing to determine the scalability and responsiveness of your MySQL server under different configurations.
As databases grow in size and complexity, traditional deployment methods often become inadequate to meet heightened performance demands. Scaling MySQL efficiently is crucial to maintaining performance, ensuring data availability, and providing timely responses to client queries, especially in high-load environments. This section will explore effective strategies such as partitioning, replication, and utilizing MySQL clusters to manage large databases.
Partitioning is a technique to distribute a large database into smaller, more manageable pieces while maintaining its logical integrity. MySQL supports several types of partitioning, such as range, list, hash, and composite partitioning.
Range partitioning: Useful when you can easily divide data into ranges. For instance, data could be partitioned by date range (e.g., year, quarter).
List partitioning: Ideal for distributing data based on a predefined list of values. This might be suitable for geographic regions or distinct categories.
Hash partitioning: This method uses a hashing function to uniformly distribute data among partitions. It’s particularly useful when no logical distinction in data ranges or lists exists.
Composite partitioning: A combination of the above methods, such as range-hash or list-hash, to fine-tune data distribution.
Partitioning helps by improving query performance through a reduction in index size, enabling faster index scans and data retrieval. It also facilitates easier maintenance and faster data access in specific scenarios. Here is a simple SQL example for range partitioning:
CREATE TABLE orders (
order_id INT AUTO_INCREMENT,
order_date DATE NOT NULL,
amount DECIMAL(10 , 2 ) NOT NULL,
PRIMARY KEY (order_id, order_date)
)
PARTITION BY RANGE( YEAR(order_date) ) (
PARTITION p0 VALUES LESS THAN (1991),
PARTITION p1 VALUES LESS THAN (1992),
PARTITION p2 VALUES LESS THAN (1993),
PARTITION p3 VALUES LESS THAN (1994)
);
Replication involves copying and distributing data and database objects from one database to another and synchronizing between databases to maintain consistency. Using replication, you can enhance database performance and achieve:
Data redundancy and increased availability: Ensuring that a copy of your data is always available reduces the risk of downtime.
Load balancing: Distribute read queries among multiple slave servers to improve the application's responsiveness.
Disaster recovery: Maintain copies of data in geographically dispersed locations to safeguard against site-specific failures.
MySQL supports several types of replication settings:
Master-Slave Replication: The most common type, where the master database handles writes and one or more slaves are used for read-only queries.
Master-Master Replication: Each server acts as both a master and a slave, allowing data writes to occur on any server and providing high availability.
Group Replication: A plugin that provides fault-tolerant systems by ensuring that servers coordinate their transactions as a group, providing fault tolerance and consistency.
MySQL Cluster is a technology providing shared-nothing clustering and auto-sharding for the MySQL database management system. It focuses on high availability and scalability, with real-time performance and the ability to handle large volumes of transactions and queries.
Real-time performance: MySQL Cluster uses an in-memory row-based storage system that provides low latency data access.
Automated failover and recovery: Ensures high availability and durability by automatically detecting node failures and rerouting node traffic as necessary.
Geographical replication: Facilitates spreading data across multiple sites for disaster tolerance and localized performance optimization.
Here is a simplified view on configuring cluster replication:
[ndbd default]
NoOfReplicas=2 # Number of replicas
[ndb_mgmd]
# Management node settings
hostname=mgm_host
[ndbd]
# Data node settings
hostname=ndb1_host
[ndbd]
hostname=ndb2_host
[mysqld]
# SQL node settings
hostname=mysql_host
Efficiently scaling large MySQL databases demands the implementation of strategies like partitioning, replication, and clustering. Each has its unique strengths, and the choice of a specific technology or a combination depends on the specific requirements like data size, expected load, consistency needs, and availability targets. By intelligently implementing these strategies, organizations can ensure robust, scalable, and high-performance database solutions.
Maintaining optimal performance in MySQL databases not only requires setup and configuration but also demands continuous monitoring and regular maintenance routines. Consistent maintenance ensures the database functions efficiently, reduces the likelihood of unexpected downtime, and prevents performance degradation over time. Here, we outline essential maintenance practices that should be routinely executed.
Over time, as data is added, removed, or updated within your MySQL database, the physical storage of this data can become fragmented. This fragmentation often leads to inefficient data retrieval, which in turn can degrade the performance of the database.
To handle this, regularly scheduled table optimization should be performed. This process can be done using the OPTIMIZE TABLE
command, which reorganizes the physical storage of table data and associated index data, to reduce storage space and improve I/O efficiency.
OPTIMIZE TABLE table_name;
Replace table_name
with the name of your database table. For databases with large tables, consider running this during off-peak hours to minimize the impact on database performance.
MySQL uses statistical information about the distribution of the values in each index to optimize queries. Accurate statistics help MySQL's query optimizer make better choices about query plans. However, these statistics can become outdated as the database changes.
To update these statistics, the ANALYZE TABLE
command can be used. This command updates the key distribution for the table, which in turn aids MySQL in optimizing searches based on key columns.
ANALYZE TABLE table_name;
Routine updates to index statistics can ensure that the query optimization process remains efficient, especially in dynamic environments where data changes frequently.
MySQL provides several logs that are useful for regular maintenance, including:
Regular monitoring of these logs helps in recognizing patterns that could indicate performance issues, like slow-running queries or frequent errors. Tools like awk
and grep
can be valuable in analyzing these logs:
grep "specific_error" /path/to/mysql/error.log
By implementing regular maintenance routines such as defragmenting tables, updating statistics, and monitoring logs, you can ensure that your MySQL databases continue to operate at peak performance. These tasks help identify potential issues early, mitigate risks, and uphold the response time and reliability of your database systems.
Schedule these maintenance tasks during periods of low activity and automate them as much as possible to maintain consistent database performance and reliability.
In the realm of MySQL database management, mastering a few advanced optimization techniques can lead to significant performance improvements, especially in high-load environments. This section explores query caching, tuning the InnoDB storage engine, and implementing performance-enhancing tools and plugins.
Query caching is a powerful way to speed up data retrieval operations in MySQL. When enabled, MySQL stores the result set of a query in the cache; subsequent identical queries can be served from this cache, drastically reducing query time and decreasing database load.
To enable and manage query caching, you can adjust the following parameters in your MySQL configuration:
query_cache_size
: This parameter specifies the amount of memory allocated to the cache. For example, setting it to 100MB:
SET GLOBAL query_cache_size = 104857600;
query_cache_type
: This parameter controls the type of queries that are cached. Set it to ON
to enable caching for all query types.
SET GLOBAL query_cache_type = ON;
query_cache_limit
: This limits the maximum size for a cached result set.
SET GLOBAL query_cache_limit = 1048576; # Caches results up to 1MB
InnoDB is a storage engine for MySQL, known for its high reliability and performance. Optimizing InnoDB involves adjusting several parameters:
Buffer Pool Size: The buffer pool is where data and indexes are cached. Setting this to a high value allows more data to be stored in RAM, reducing disk I/O.
[mysqld]
innodb_buffer_pool_size = 12G # depends on available server memory
Log File Size: This determines the size of the log files in the InnoDB transaction log. Larger log files minimize disk I/O due to checkpointing.
[mysqld]
innodb_log_file_size = 512M
Flush Method: Adjusting the flush method can balance performance with reliability:
[mysqld]
innodb_flush_method = O_DIRECT
Several tools and plugins can be used to further optimize MySQL performance:
Performance Schema: This feature helps monitor MySQL server execution at a low level. Enable it in your my.cnf:
[mysqld]
performance_schema = ON
Percona Toolkit: This collection of advanced command-line tools helps perform a variety of MySQL and system tasks that are too difficult or complex to perform manually.
MySQLTuner: A script written in Perl that allows you to review a MySQL installation quickly and make adjustments to increase performance and stability.
Implementing these advanced optimizations requires careful testing and incremental changes. Always backup your database before making any significant configurations, and monitor the impact using a robust toolset.
For instance, before and after making the changes, you might want to conduct load tests using tools like LoadForge to gauge the impact of each adjustment. This helps in precise tuning based on the system's response to real-world simulated traffic.
Exploring these advanced techniques will equip you with the knowledge to handle complex scenarios and optimize MySQL performance to meet and exceed the demands of modern applications.
In this section, we explore various practical, real-world examples of how performance optimization techniques have been successfully applied to MySQL databases. These case studies underscore the effectiveness of strategies discussed in previous sections, from indexing and query optimization to advanced server configuration and maintenance.
An e-commerce company faced challenges with slow response times during high-traffic periods which significantly impacted customer experience and sales. The problem was traced to several poorly optimized queries that were key to product searches and listings.
-- Example of optimized query
SELECT product_id, product_name, price FROM products
USE INDEX (product_search_index)
WHERE product_category = 'Electronics' AND in_stock = true;
A popular media outlet experienced database lag that was not attributable solely to query inefficiency but to suboptimal MySQL server configuration.
innodb_buffer_pool_size
to utilize 80% of available system memory.max_connections
to handle more simultaneous user sessions.innodb_file_per_table
to improve I/O performance.A Software-as-a-Service (SaaS) platform providing real-time data analytics was dealing with decreasing performance issues as their data grew.
A financial services firm handling millions of transactions daily required an overhaul in their database management strategy to handle increasing loads efficiently.
query_cache_limit
and query_cache_size
.These examples demonstrate the tangible benefits of applying meticulous MySQL performance optimization techniques. By addressing specific challenges through strategic adjustments at various levels—be it query structure, server configuration, or regular maintenance—an optimized MySQL database not only performs better but also provides a more stable and efficient environment for handling data-intensive applications.
When it comes to enhancing the performance of MySQL databases, having the right tools can significantly ease the process of monitoring, diagnosing, and optimizing. In this section, we'll explore a variety of tools and resources that are essential for anyone looking to improve their MySQL performance.
MySQL Workbench: MySQL Workbench is a unified visual tool for database architects, developers, and DBAs. It provides data modeling, SQL development, and comprehensive administration tools for server configuration, user administration, and much more. The Performance Dashboard and Query Statistics sections allow you to monitor MySQL using customizable dashboards.
Percona Monitoring and Management (PMM): PMM is an open-source platform for managing and monitoring MySQL performance. It facilitates observing database performance in real-time with dashboards and graphs related to query analytics, metrics monitor, and more.
Performance Schema: Included in MySQL, the Performance Schema helps collect and report performance data. It captures data about server execution at a low level and provides valuable insights without a significant performance hit.
Sys Schema: A set of objects that helps DBAs and developers interpret data collected by the Performance Schema. Sys Schema simplifies queries for common diagnostic tasks, such as finding inefficient queries, checking for unused indexes, and other operational analytics.
MySQL Tuner: A script written in Perl that you can run against your MySQL server and quickly get a variety of recommendations for optimization. It provides suggestions concerning configurations, schema, and other variables that could help improve performance.
pt-query-digest: Part of the Percona Toolkit, this tool is excellent for reviewing SQL queries that are most demanding on your server. It breaks down the queries by resource usage, and lifetime, and can even help identify potential slow queries before they become a significant issue.
Percona Server for MySQL: Enhanced, drop-in replacement for MySQL that includes performance and scalability enhancements not found in vanilla MySQL. It includes features such as improved query performance, Thread Pooling, and advanced diagnostics that go beyond what traditional MySQL offers.
MySQL Enterprise Monitor: This is Oracle’s commercial solution tailored for managing MySQL environments more efficiently. It includes real-time monitoring and alerts, performance tuning recommendations, and comprehensive security features.
Monitoring Query Performance:
SHOW STATUS LIKE 'Handler_read_rnd_next';
This command helps monitor the read requests for table scanning activities, which can indicate inefficient queries needing optimization.
Utilizing MySQL Tuner:
perl mysqltuner.pl
Running this script will analyze your MySQL server and output suggestions for memory, storage, and other settings that could be optimized to improve performance.
Empowering yourself with the right set of tools can profoundly impact the performance of your MySQL databases. From in-built utilities like Performance Schema to sophisticated monitoring solutions such as MySQL Enterprise Monitor, each tool serves a specific purpose that complements your tuning efforts. Regularly utilizing these tools not only assists in maintaining optimal database operation but also in foreseeing potential issues before they escalate.
Throughout this guide, we've explored a wide array of strategies to boost MySQL performance, from effective indexing and query optimization to deep dives into server configuration and advanced technical adjustments. As we consolidate our learning, it's essential to highlight that the key to sustaining high performance in MySQL databases lies in a balanced combination of best practices, regular maintenance, and continuous assessment.
Below, we summarize the critical best practices alongside final thoughts to empower you with a robust approach toward optimizing and scaling your MySQL databases:
Implement Proper Indexing
EXPLAIN
to identify missing indexes and eliminate unused or duplicate ones.Optimize Queries
LIKE
pattern and strive to write queries that target as few rows as possible.Configure for Performance
my.cnf
/my.ini
file can lead to substantial performance improvements.Scale Intelligently
Perform Regular Maintenance
OPTIMIZE TABLE
, and updating statistics to help the optimizer choose the best query plans.Leverage Advanced Techniques
Continuous Monitoring and Testing
MySQL Workbench
, Percona Monitoring and Management
, and Prometheus
coupled with Grafana
can offer extensive monitoring capabilities.<pre><code>
# Example: Basic monitoring loop using Bash
while true; do
mysqladmin status | egrep -o "Queries:[^ ]+"
sleep 10
done
</code></pre>
Implementing these best practices within MySQL environments is not a one-time task but a continuous process of refinement and improvement. Emerging technologies and evolving business requirements will necessitate periodic revisits to your database strategy. With a commitment to these principles, you are well-equipped to maintain, optimize, and scale your MySQL databases effectively, ensuring robust performance and reliability in the long run.