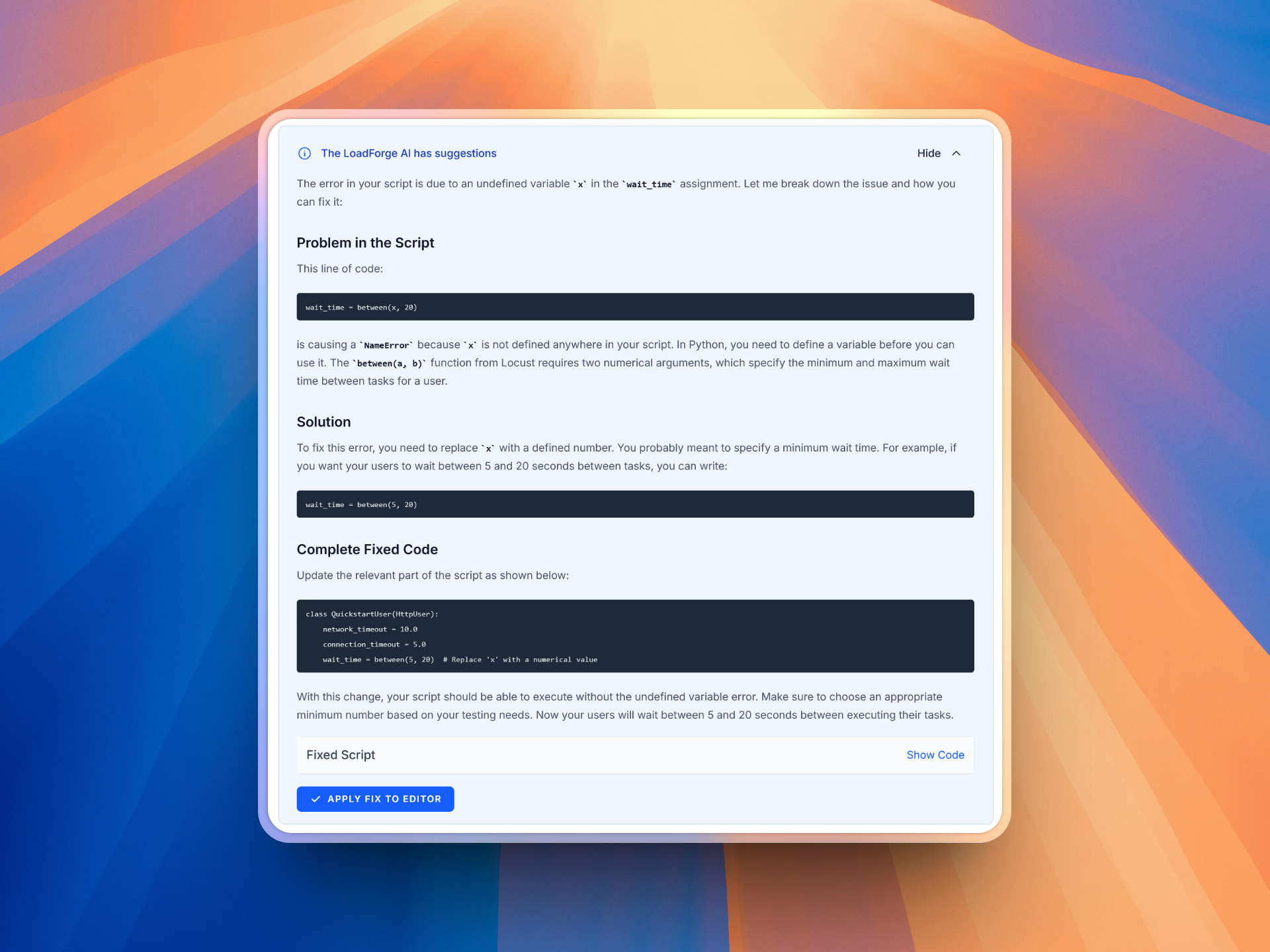
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
Caching is a fundamental technique in web development that stores copies of files or computational results to deliver faster responses to user requests. In the context of Laravel, a robust PHP framework, caching plays a crucial role in enhancing both...
Caching is a fundamental technique in web development that stores copies of files or computational results to deliver faster responses to user requests. In the context of Laravel, a robust PHP framework, caching plays a crucial role in enhancing both the performance and the user experience of web applications.
Laravel seamlessly integrates caching as part of its architecture, providing a straightforward and flexible way to store frequently accessed data such as database query results, HTML views, or JSON data. This mechanism reduces the need to execute expensive operations such as database queries or complicated business logic on every request, thereby dramatically speeding up application response times and lowering server load.
Improved Performance: By temporarily storing data that is expensive to fetch or compute, caching reduces the processing overhead on servers. This is vital for enhancing the speed of the application, which is directly linked to user satisfaction and service efficiency.
Scalability: Effective caching reduces the number of operations performed on the web server, thus enabling the application to handle a larger number of simultaneous users without degrading performance. This is particularly beneficial for applications expecting high traffic volumes.
Cost Efficiency: Reducing the computational load on servers can also lower operational costs by decreasing the resources required to serve the same amount of traffic.
Better User Experience: Faster web applications translate into a smoother, more responsive user experience. This can be critical for maintaining user engagement and satisfaction, especially in competitive digital environments.
Laravel provides an expressive, unified API for various caching backends. The framework supports popular cache drivers out of the box, including:
The choice of cache driver can be configured easily via Laravel's .env
file, allowing developers to tailor the caching mechanism to their specific needs and environment constraints.
Here is a simple example of setting and retrieving a cached value in Laravel:
use Illuminate\Support\Facades\Cache;
// Setting a cache value
Cache::put('key', 'value', $seconds);
// Retrieving a cached value
$value = Cache::get('key');
This caching logic can be integrated into any part of a Laravel application, ensuring that developers can optimize critical sections of their system efficiently.
In summary, caching in Laravel is a powerful approach to enhancing application performance and scalability. By understanding and implementing appropriate caching strategies, developers can ensure that their applications remain fast and robust regardless of user load or data volume, ultimately leading to improved user interaction and satisfaction.
Caching is a critical component in enhancing the performance of web applications by storing frequently accessed data, reducing the need to access slower backend sources repeatedly. Laravel provides robust, easy-to-implement caching solutions that can significantly improve your application's speed and responsiveness. This guide walks you through the basic configurations of Laravel's caching systems, including file, database, and in-memory caches such as Redis and Memcached.
Laravel supports various cache drivers out of the box. The cache configuration is located in config/cache.php
. In this file, you'll find several options for cache configurations including file
, database
, array
, memcached
, and redis
.
Before proceeding, make sure the cache settings are properly set in your .env
file:
CACHE_STORE=file
This configuration utilizes the file system for caching. To change the cache driver, simply modify the CACHE_STORE
value to redis
, memcached
, database
, or any other supported driver.
File caching is the default cache driver for Laravel, storing cached items in the filesystem. It's simple to set up and does not require additional services:
Ensure the CACHE_STORE
in your .env
file is set to file
.
Set the path
in config/cache.php
under the stores
array to define where the cache files should be stored:
'file' => [
'driver' => 'file',
'path' => storage_path('framework/cache/data'),
],
To cache data in the database, you need to set up a table to store the cache items:
Set CACHE_STORE
in your .env
file to database
.
Run the following Artisan command to create the necessary migration:
php artisan cache:table
After creating the migration, run it to create the cache table:
php artisan migrate
Configure the connection and table in config/cache.php
:
'database' => [
'driver' => 'database',
'table' => 'cache',
'connection' => null,
],
Redis is an advanced key-value store that is very fast for operations involving in-memory data sets. To set up Redis caching:
Install Redis on your server and the PhpRedis PHP extension or the predis package.
Set CACHE_STORE
in your .env
file to redis
.
Configure Redis connection settings in config/database.php
in the redis
section:
'redis' => [
'client' => 'predis',
'default' => [
'host' => env('REDIS_HOST', '127.0.0.1'),
'password' => env('REDIS_PASSWORD', null),
'port' => env('REDIS_PORT', 6379),
'database' => 0,
],
],
Memcached is another potent in-memory caching system. To enable Memcached caching:
Install Memcached on your server and ensure the Memcached PHP extension is installed.
Set CACHE_STORE
in your .env
file to memcached
.
Configure the Memcached servers in config/cache.php
:
'memcached' => [
'driver' => 'memcached',
'servers' => [
[
'host' => env('MEMCACHED_HOST', '127.0.0.1'),
'port' => env('MEMCACHED_PORT', 11211),
'weight' => 100,
],
],
],
Setting up caching in Laravel is straightforward, whether you are using file, database, Redis, or Memcached drivers. Proper configuration and choosing the right cache driver based on your application's specific needs will significantly impact performance. Make sure to test the cache configurations and monitor their performance to ensure optimal results.
In subsequent sections, we will delve deeper into using these configurations effectively within your Laravel applications, leveraging advanced techniques and ensuring your cache strategy scales efficiently under load.
In Laravel, caching is a pivotal aspect of enhancing the application performance by reducing the load on the database and speeding up the response time. Laravel supports various cache drivers, each suited for different types of environments and specific use cases. Selecting the right cache driver is crucial for optimizing the performance of your Laravel application. Below, we compare the most commonly used Laravel cache drivers, outline their characteristics, and provide recommendations based on application needs.
Usage: File cache is the simplest form of caching that Laravel offers. It serializes the cache data and stores it directly on the filesystem.
Pros:
Cons:
Recommendation: Use file cache for local development or small applications where setup simplicity is more important than cache performance.
Usage: Stores cache items in a database table. Laravel uses either the default database connection or a separate connection you configure.
Pros:
Cons:
Recommendation: Suitable for applications that have light to moderate caching needs and are already utilizing robust database systems that can handle additional load.
Usage: An in-memory data structure store, used as a distributed, in-memory key-value database, cache and message broker.
Pros:
Cons:
Recommendation: Ideal for high-performance applications requiring quick read/write operations and complex data types. Best for real-time applications.
Usage: A high-performance, distributed memory object caching system intended for use in speeding up dynamic web applications by alleviating database load.
Pros:
Cons:
Recommendation: Best used in situations where simple caching and high performance are required, particularly where cache size is large.
Usage: Alternative PHP Cache (APC) is suitable for a single server setup where no cache synchronization between instances is required.
Pros:
Cons:
Recommendation: Use APC if you have a standalone server and need faster performance without the need for distributed caching.
Choosing the right cache driver largely depends on your application's specific needs:
In all cases, consider the impact of your choice not only on performance but also on the maintainability of your application. As your Laravel application scales, regularly revisiting your caching strategy is crucial.
Caching in Laravel can significantly enhance your application's performance by reducing the load on your database and speeding up the response time. This section provides a comprehensive step-by-step guide on how to implement caching in various parts of your Laravel application, focusing on route, configuration, and results caching.
Route caching in Laravel is a powerful feature for improving the performance of your application. It is particularly useful for applications with a large number of routes or complex configuration. To implement route caching, follow these steps:
Optimize Your Routes
Before caching, ensure your routes are optimized. Group middleware that applies to many routes, and avoid using closures in routes as they cannot be cached.
Cache Your Routes
To cache all your routes, run the following Artisan command:
php artisan route:cache
This command will compile your routes into a PHP array, improving the performance of route registration.
Clear Route Cache
If you make changes to your routes, remember to clear the route cache using:
php artisan route:clear
Then, re-run the route caching command to update the cache.
Laravel allows you to cache all your configuration settings into a single file, which can drastically reduce the number of file reads needed on each request. Here’s how to cache your configuration settings:
Cache Configuration
To cache the configuration, run:
php artisan config:cache
This will merge all configuration files into one and speed up configuration loading.
Clear Configuration Cache
After updating configuration files or .env variables, clear the cache by running:
php artisan config:clear
Remember to re-run the config cache command after making changes.
For database result caching, Laravel provides a straightforward approach to store query results in cache, reducing database load. Below is how to cache database queries:
Cache a Query Result
Use the Cache
facade to store the result of a query. For example, caching a query result for 30 minutes might look like this:
$posts = Cache::remember('posts.all', 30, function () {
return Post::all();
});
Clear Cache When Necessary
Ensure that your cache is invalidated when data changes. For example, if a new post is created, clear the cache to allow the list of posts to update:
Cache::forget('posts.all');
Alternatively, use the Cache
tags for more flexible cache invalidation:
Cache::tags('posts')->flush();
By applying route, configuration, and result caching correctly in your Laravel applications, you help ensure a smoother and faster user experience while reducing server load and response times.
When developing complex applications with Laravel, simply implementing basic caching is often not enough. Advanced caching techniques can significantly enhance the performance and scalability of your applications. This section explores some of the sophisticated caching strategies such as tagging, prefetching, and invalidation. These methods can help you manage cache more effectively, especially in dynamic environments where content changes frequently.
Cache tagging is essential for maintaining a highly granular control over cache entries. In Laravel, tagging allows you to assign tags to cached responses and then perform operations on all cached items that share a tag. This is particularly useful in scenarios where multiple cache items need to be invalidated simultaneously.
Here’s how you can use cache tagging in Laravel:
// Storing tagged cache entries
Cache::tags(['product', 'inventory'])->put('product_1', $productDetails, $expiration);
// Accessing a tagged cache entry
$product = Cache::tags(['product', 'inventory'])->get('product_1');
// Clearing all entries tagged with 'product'
Cache::tags('product')->flush();
Prefetching involves loading data into the cache before it is actually requested. This proactive approach can reduce latency and improve the user experience by anticipating future requests based on user behavior patterns or application workflows.
Implementing prefetching requires a good understanding of your application's usage patterns. For instance, if you know a user typically accesses certain data after logging in, you can prefetch this data as part of the login process.
Example of prefetch implementation might look like this:
if (auth()->check()) {
// User is logged in, prefetch essential user-specific data
$userDashboard = Cache::remember('user_dashboard_'. auth()->id(), now()->addMinutes(10), function () {
return queryDashboardData(auth()->user());
});
}
Invalidation is the process of removing outdated data from the cache. Effective invalidation ensures that users do not receive stale data, which is crucial for maintaining data accuracy and trust, especially in applications dealing with frequently changing data.
In Laravel, you can invalidate cache in several ways:
Time-Based Expiration: Set a TTL (Time To Live) for cache entries that causes them to be automatically removed after a certain period.
Cache::put('key', 'value', now()->addMinutes(10)); // Cache expires after 10 minutes
Manual Invalidation: Explicitly remove a cache item when you know it has been updated.
Cache::forget('key'); // Manually remove the cache item
Conditional Invalidation: Use events and listeners to invalidate cache in response to specific actions or triggers.
Event::listen(UserProfileUpdated::class, function ($event) {
Cache::forget('user_profile_' . $event->userId);
});
Advanced caching techniques such as tagging, prefetching, and invalidation are powerful tools in a developer’s arsenal. By implementing these strategies, you can ensure that your Laravel application remains efficient, even as the complexity grows. Remember, the key to effective caching is not just storing data but managing the lifecycle of that cached information responsibly and intelligently.
Effective monitoring and management of cache performance are crucial for maintaining the efficiency and reliability of your Laravel application. This section provides guidance on the tools and techniques that can be employed to monitor cache effectiveness and troubleshoot common cache-related issues.
Laravel Telescope is an elegant debug assistant for Laravel that provides insight on requests, exceptions, database queries, and more. It includes a cache watcher that monitors key cache events:
To set up Telescope in your Laravel project, you can follow these steps:
composer require laravel/telescope
php artisan telescope:install
php artisan migrate
Once installed, you can access Telescope through your application's /telescope
endpoint and navigate to the "Cache" tab to see real-time data on cache usage.
If you are using Redis as your cache driver, tools like RedisInsight or Redis Commander can be invaluable. These tools provide a more in-depth view of Redis operations, memory usage, and performance metrics.
Laravel's built-in logging capabilities can also be utilized to track cache operations. You can log cache activities by customizing the log stack in config/logging.php
and using Laravel's logging facilities within your caching logic:
Cache::remember('key', $seconds, function () use ($key) {
Log::info('Cache miss for key: ' . $key);
return computeExpensiveResource();
});
Cache Configuration Checks: Ensure that your cache configuration in config/cache.php
is correctly set for the intended driver and connection settings. Mistyped or incorrect settings can lead to unexpected behavior.
Testing Cache Locally: Before deploying changes that affect caching, test them in a local development environment. This helps avoid disrupting the live application and allows you to fine-tune cache settings without impact.
Clearing Cache Strategically: Sometimes, issues can arise due to stale or corrupted cache data. Using Laravel's Artisan commands can help clear the cache selectively or entirely, which can be useful during troubleshooting:
Clear specific cache keys:
php artisan cache:forget 'key'
Clear the entire cache:
php artisan cache:clear
Performance Metrics Analysis: Use performance metrics such as response time and memory usage to understand the impact of caching. Slow cache operations might indicate a need for optimizing the cache storage or querying logic.
Cache Layer Visibility: Enhancing visibility into the cache layer by adding more detailed monitoring or debugging statements around cache operations can help identify and resolve issues more quickly.
Monitoring and managing cache performance effectively requires a combination of right tools and techniques. With Laravel's built-in capabilities and some external tools, developers can gain substantial insights into how caching impacts their application and take informed actions based on real-time metrics and logs. By following these guidelines, you can ensure that your caching strategy is robust, responsive, and reliable.
Caching is a powerful feature to enhance the performance of web applications, including those built with Laravel. However, improperly managed cache systems can introduce significant security vulnerabilities, especially when handling sensitive information. This section explores key security considerations and provides practical tips on securing cache data in Laravel applications.
The most prevalent risks associated with caching sensitive data include unauthorized access and data leakage. These risks are heightened when cache stores are compromised or when cache data is improperly invalidated.
Examples of Cache Security Risks:
To mitigate these risks, follow these best practices for implementing secure caching strategies in your Laravel applications:
Use Encrypted Cache Data
Crypt
facade.use Illuminate\Support\Facades\Cache;
use Illuminate\Support\Facades\Crypt;
// Encrypt and store in cache
Cache::put('key', Crypt::encrypt('Sensitive Data'), $minutes);
// Retrieve and decrypt from cache
$decrypted = Crypt::decrypt(Cache::get('key'));
Limit Cache Lifespan
// Store data for 10 minutes
Cache::put('user_session', $data, now()->addMinutes(10));
Cache Data Isolation
Strict Access Controls
Regular Cache Auditing and Monitoring
Proper Cache Invalidation
// Correctly invalidate cache
Cache::forget('key');
HTTPS Usage
While caching is essential for performance, it must not compromise the security of the application. By following the practices outlined above, developers can ensure that their Laravel applications handle cache data securely, minimizing the risks associated with sensitive information exposure. Always balance performance improvements with potential security implications to maintain the overall integrity and trustworthiness of your application.
Scaling and optimizing the caching layer of a Laravel application is crucial to handling increased load and ensuring the application remains responsive under high traffic scenarios. This section offers practical tips and strategies for effectively scaling and optimizing the Laravel caching system.
In distributed environments, load balancers play a pivotal role in managing incoming traffic across multiple server instances. Implementing load balancers helps distribute requests evenly, ensuring no single server becomes a bottleneck due to uneven cache hits.
Setting up a clustered cache environment, where cache data is synchronized across multiple nodes, can significantly enhance data retrieval times and load distribution.
Example configuration for a Redis cluster in Laravel:
'cache' => [
'stores' => [
'redis' => [
'driver' => 'redis',
'connection' => 'default',
'options' => [
'cluster' => 'redis',
'nodes' => [
'tcp://127.0.0.1:6379',
'tcp://127.0.0.1:6380',
],
],
],
],
],
Preloading commonly accessed data into the cache can reduce latency and database load during peak times.
Efficient cache invalidation is crucial to ensure the consistency of data served to users. Implement strategies to handle cache invalidation smartly to prevent stale data.
Beyond setup, it’s essential to periodically test the robustness and performance of your cache strategy under simulated high traffic using a tool like LoadForge. LoadForge can help you:
Effective scaling and optimization of the Laravel caching layer include understanding and implementing advanced caching strategies, using tools to monitor and test system performance, and continuously refining your approach based on new insights and technologies. By following these guidelines, developers can ensure their Laravel applications are prepared to handle high loads efficiently and reliably.
Testing the performance of your Laravel cache setup is crucial to ensure it can handle high traffic scenarios effectively. LoadForge is an excellent tool for simulating these scenarios and measuring the resilience of your cache configuration. By following the steps below, you can gauge how well your application performs under stress and optimize it accordingly.
Before you can effectively test your Laravel cache, you need to set up a test on LoadForge. This involves:
Creating a new test script: LoadForge provides a powerful DSL (Domain Specific Language) that allows you to define the specifics of your test.
Defining user behavior: Specify how many users you want to simulate and what actions they should perform. For testing cache performance, focus on actions that heavily rely on cached data.
Here is a basic example of a test script that would simulate users retrieving cached content:
from loadforge import http as lf_http
class User(lf_http.User):
@task
def index(self):
self.client.get("/cached-route")
After setting up your test in LoadForge:
Once the test is complete, LoadForge provides detailed analytics that will help you understand the performance of your cache setup:
Use the data from LoadForge to optimize your cache configuration:
After making adjustments, it’s important to run additional tests to see if the changes have improved the performance:
By systematically utilizing LoadForge to test and adjust your Laravel caching strategies, you can ensure that your application is optimally configured to handle real-world loads. This process helps in achieving a balance between performance and resource usage, ultimately leading to a smoother user experience.
With these steps, LoadForge can be an invaluable tool in your performance testing arsenal, helping you to ensure that your Laravel application is as robust and efficient as possible under diverse and challenging conditions.
In this comprehensive guide, we've explored the pivotal role of caching in enhancing the performance and scalability of Laravel applications. Implementing efficient caching can significantly improve response times, reduce database load, and provide a smoother user experience.
Understanding Caching: Caching is crucial for optimizing web applications by storing frequently accessed data temporarily in a fast-access storage layer. This reduces the need to retrieve or compute this data repeatedly from slower storage layers.
Configuring Cache: Laravel supports several cache drivers, and it's vital to choose one that best aligns with your application's needs. Whether it's file-based, in-memory with Redis, or distributed using Memcached, proper configuration is key.
Cache Drivers Choice: Selecting the right cache driver depends on your application requirements. In-memory caches like Redis are typically faster and more suited for high-demand applications, though they require more robust infrastructure management.
Implementation Strategies: Implement caching thoughtfully by caching routes, configuration data, and query results where frequent access patterns are observed. This selective caching helps in optimizing resource utilization effectively.
Advanced Techniques: Employ advanced strategies like tagging for invalidation, prefetching data proactively, and auto-scaling caches in response to demand spikes to handle complex scenarios and high traffic volumes effectively.
Monitoring and Managing: Continuously monitor cache performance and effectiveness. Tools built into Laravel, or external monitoring services, can provide insightful metrics that guide cache optimization and troubleshooting.
Security in Caching: Always secure cache data, especially when it involves sensitive information. Implement access controls, use encryption as needed, and ensure cached data does not leak between users or sessions.
Scalable Caching: Scale the caching layer by integrating with load balancers and employing clustered environments to accommodate growing application demands and ensure high availability.
Regular Testing: Regularly test your cache implementation with tools like LoadForge to ensure that it performs under different load conditions. This can help predict and mitigate potential bottlenecks before they impact users.
loadforge test --config laravel-cache-load.json
Optimize Cache Lifetimes: Set appropriate cache lifetimes; not too short to negate the benefits of caching, and not too long to serve stale data. Dynamic cache invalidation strategies can help manage this balance adeptly.
Use Environment-Based Configuration: Utilize Laravel’s ability to handle environment-specific configurations to tweak caching strategies across development, staging, and production environments. This ensures optimized settings relevant to each stage.
Document and Review: Maintain documentation on your caching strategies and configurations. Regularly review and update the caching logic as the application evolves and new features are added.
Incorporate Feedback Loops: Utilize feedback from real-world usage to tune and adjust caching strategies. Performance metrics and user feedback are invaluable for iterative improvements.
In conclusion, effectively leveraging Laravel's caching capabilities requires a thoughtful approach from setup to ongoing management. By adhering to these best practices and continuously monitoring and testing your cache system, you can ensure that your Laravel application remains fast, reliable, and scalable. Remember, cache optimization is an ongoing process that plays a critical role in the overall performance of your web applications.