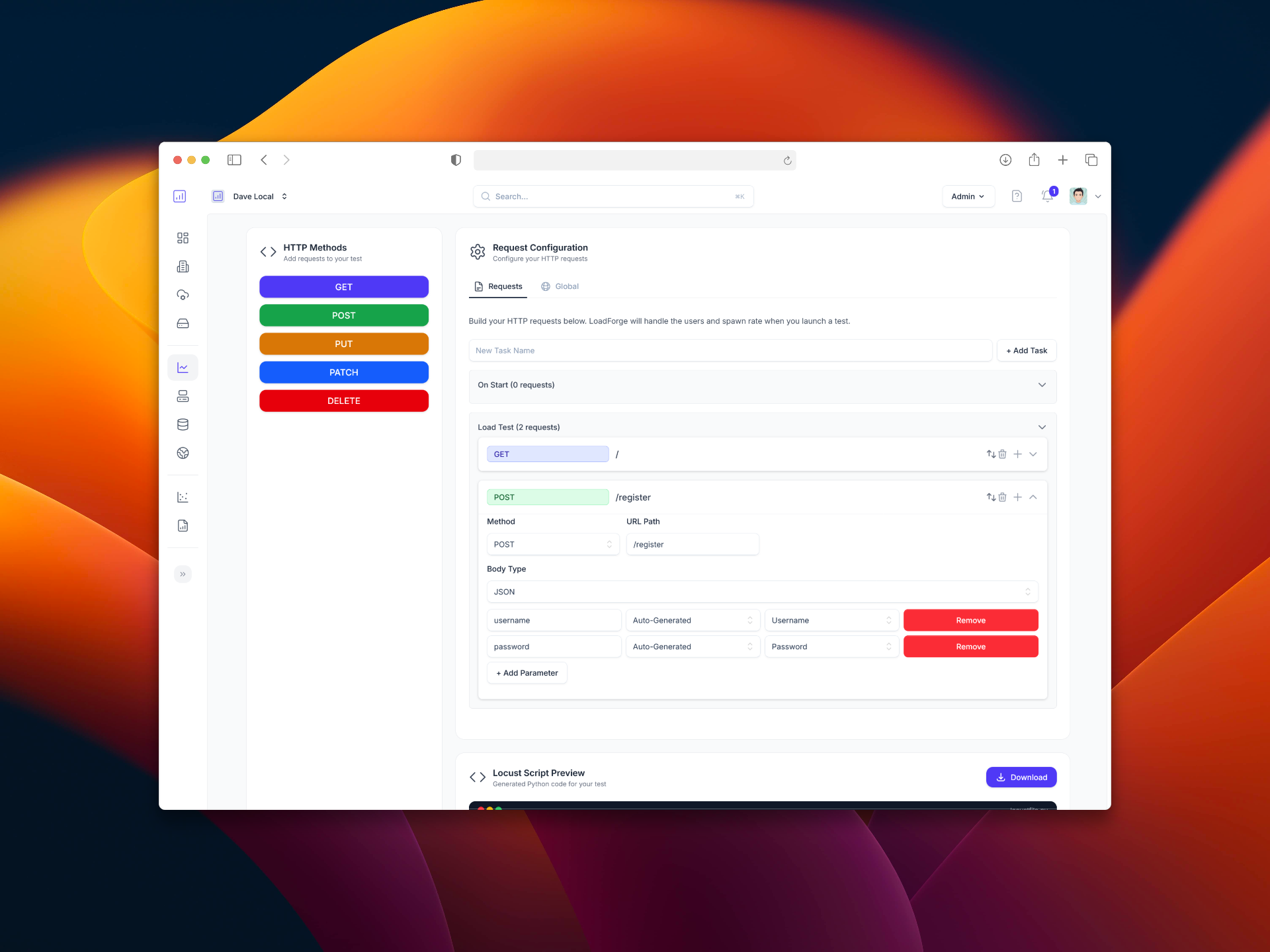
Jira Integration & Graphical Test Builder
We are proud to announce the release of two new features, available immediately. 🚀 Jira Integration: Automate Issue Tracking for...
In the dynamic world of web development, Ruby on Rails stands out for its ease of use, developer-friendly environment, and powerful framework capabilities. However, like any web application, a Rails-based system's real test comes under the pressure of heavy user...
In the dynamic world of web development, Ruby on Rails stands out for its ease of use, developer-friendly environment, and powerful framework capabilities. However, like any web application, a Rails-based system's real test comes under the pressure of heavy user traffic. This is where load testing becomes not just valuable, but essential.
Load testing is the process of simulating real-world stress on software systems to evaluate their performance in high-demand situations. For Ruby on Rails applications, this kind of testing is crucial to ensure that your application can not only survive but thrive under peak loads. It helps identify bottlenecks in your infrastructure, database, or codebase that could lead to slow response times or even system failures during critical times.
Scalability refers to the capability of a system to handle a growing amount of work without compromising on performance. In the context of Ruby on Rails, scalability means that as more users access your application, it can manage the increased load without hiccups or downtime. Here’s why scalability is particularly critical:
Through effective load testing, you can prepare your Rails application to handle unexpected spikes in traffic gracefully, ensuring a smooth and consistent user experience. Tools like LoadForge empower developers by providing an extensive platform to simulate and test various load scenarios, which helps in making informed decisions about scalability needs.
This guide delves deeper into the art and science of load testing using LoadForge, specifically tailored for Ruby on Rails applications. It offers a practical roadmap from setting up your environment, through writing and running tests, to analyzing and optimizing based on the results. By understanding and implementing load testing, you ensure that your Rails application is not just functional but truly scalable.
Load testing is a type of performance testing used to determine how a system behaves when it is accessed by multiple users at the same time. This involves simulating real-world usage conditions to ensure that the application can sustain its intended load while still functioning effectively. For Ruby on Rails applications, which are dynamic and often data-intensive, load testing becomes crucial in validating the application's performance and scalability under peak traffic conditions.
Ruby on Rails applications, characterized by their 'Convention over Configuration' design, benefit greatly from targeted load testing due to their structured framework. The modular architecture of Rails supports scalable application development, which can be tested effectively with load simulations:
Routes: Testing can target specific Rails routes to simulate user interactions with different parts of the application, such as logging in, posting data, or querying the database.
Database: Simulating heavy read and write operations to test how well the application's database handles concurrent access.
Assets: Testing the load times of static and dynamic assets to evaluate the efficiency of content delivery networks and asset pipelines.
LoadForge is an ideal tool for carrying out such load tests on Ruby on Rails applications. It leverages the power of Locust, an open-source load testing tool, allowing for scripting in Python to create customizable test scenarios. Here’s how LoadForge facilitates detailed and scalable load testing:
By integrating LoadForge in your development pipeline, you ensure that your Ruby on Rails application is not only ready for the current user load but also prepared for future growth. This proactive approach helps in maintaining a smooth and efficient user experience, thus safeguarding the reputation and reliability of your web application.
To effectively load test your Ruby on Rails application using LoadForge, setting up your testing environment correctly is critical. This step-by-step guide will walk you through selecting the right configuration and ensuring your application is prepared for the stresses of load testing.
Before initiating any load tests, it's essential to ensure your application is in a test-ready state:
LoadForge offers a command-line interface (CLI) to manage your tests easily from your local machine. Install the CLI using pip:
pip install loadforge-cli
Configuration plays a crucial role in the accuracy and effectiveness of your load tests:
Here is an example snippet to configure a basic test in your locustfile:
class UserBehavior(TaskSet):
@task
def index_page(self):
self.client.get("/")
class WebsiteUser(HttpUser):
tasks = [UserBehavior]
min_wait = 5000
max_wait = 9000
Ensure that all necessary environment variables and secrets (like API keys, database passwords, etc.) are set up for your test environment. Use secure methods to manage these settings, avoiding hard-coding sensitive information directly in your locustfiles.
Before deploying full-scale load tests, run a small-scale smoke test to ensure that the environment and your locustfile are properly set up:
loadforge --locustfile my_locustfile.py --clients 10 --hatch-rate 2 --run-time 1m
This command runs a one-minute test with ten users hatching at a rate of two per second, which helps in identifying any glaring issues with the setup.
Finally, log in to your LoadForge dashboard to select and adjust settings more specifically:
This stepwise preparation of your environment is crucial for executing meaningful and effective load tests. Proper configuration not only ensures that tests run smoothly but also guarantees that the findings and data you get out are reliable and actionable.
When load testing a Ruby on Rails application, creating an effective locustfile is a crucial step. This file serves as the blueprint for your load test, defining the user behavior and Requests that simulate traffic towards your application. Below, we provide detailed guidance on crafting a locustfile tailored specifically for Ruby on Rails applications.
Before we begin writing our locustfile, ensure that you have Locust installed. You can install Locust using pip:
pip install locust
Start your locustfile by importing the necessary modules from Locust. In most cases, you'll need HttpUser
, task
, and between
from the locust
library:
from locust import HttpUser, task, between
In Locust, user behavior is modeled through classes. Create a class that inherits from HttpUser
and define the tasks within it. The wait_time
method can be used with between
, which specifies the time between the execution of tasks.
class WebsiteUser(HttpUser):
wait_time = between(1, 5) # User waits between 1 to 5 seconds between tasks
Each function decorated with the @task
decorator represents a specific user action. In a Ruby on Rails application, these actions typically include retrieving web pages or submitting forms.
Assuming you have a blog application, here are a few examples:
@task
def view_posts(self):
self.client.get("/posts")
@task(3) # higher weight, performed more frequently
def view_post(self):
post_id = 42 # assuming an existing post with id 42
self.client.get(f"/posts/{post_id}")
@task
def create_post(self):
self.client.post("/posts", json={
"title": "Load Testing with LoadForge",
"content": "Learning how to implement locustfiles is exciting!"
})
If you need to simulate more complex user behavior that involves multiple types of users, you can define additional classes akin to the one above or modify user task ratios to represent different types of user interactions with your application.
Once your tasks are defined, your basic locustfile for a Ruby on Rails application should look like this:
from locust import HttpUser, task, between
class WebsiteUser(HttpUser):
wait_time = between(1, 5) # Waits between 1 to 5 seconds between tasks
@task
def view_posts(self):
self.client.get("/posts")
@task(3)
def view_post(self):
self.client.get("/posts/42") # Views the post with id 42
@task
def create_post(self):
self.client.post("/posts", json={
"title": "Load Testing with LoadForge",
"content": "Learning how to implement locustfiles is exciting!"
})
After crafting your locustfile, you are ready to upload it to LoadForge and set up your test. You can adjust configurations like the number of users, spawn rate, and testing duration according to the needs of your specific simulation scenario.
This locustfile serves as your foundation in load testing your Ruby on Rails application, aiming to mimic real-world usage as closely as possible to ensure that your application can handle expected traffic levels efficiently.
Once you have crafted your locustfile to suit your Ruby on Rails application’s needs, the next step is running your load test using LoadForge. This section will guide you through the process from uploading your locustfile to interpreting the initiatory outcomes of your test. Here is how you can set up and run your load tests effectively.
Before you can start your test, you need to upload the locustfile that you’ve created. This file describes the actions that simulated users will take on your application during the test.
Upload
and select the locustfile from your computer.After uploading your file, configure your test parameters. This includes the number of users (clients), spawn rate, and test duration among other settings.
### Step 3: Initiate the Load Test
Now that your locustfile is uploaded and the test is properly configured, you can start the test:
1. Scroll to the bottom of the test setup page on LoadForge.
2. Click on the “Launch Test” button. This will queue your test for execution, and LoadForge will begin deploying resources to run your test.
### Step 4: Monitoring the Test Execution
While your test is running, LoadForge provides a real-time dashboard where you can monitor the progress and view initial results. This includes:
- **Requests per Second**: How many requests your application is handling per second.
- **Response Times**: Average, median, and max response times during the test.
- **Error Rates**: Percentage and type of failed requests.
### Step 5: Test Completion
Once the test is complete, LoadForge will automatically compile the results. You can view detailed metrics, download reports, and analyze the performance under load. This information is crucial for understanding how your application behaves under stress and what might be the bottlenecks.
Running load tests with LoadForge is straightforward but powerful. By following these steps and utilizing LoadForge’s capabilities, you can significantly enhance the scalability and performance of your Ruby on Rails application. Continue refining your testing strategy as needed based on the results and insights gained from each test.
## Analyzing the Results
After successfully conducting a load test on your Ruby on Rails application using LoadForge, analyzing the outcome is crucial. This section explores how to interpret the test results, understand vital metrics, and use this data to guide your scalability improvements.
### Understanding Key Metrics
LoadForge provides a comprehensive set of metrics that help you understand the performance and health of your Ruby on Rails application under stress. Here are some of the critical metrics to focus on:
- **Requests Per Second (RPS):** This indicates the number of requests your application can handle each second. A higher RPS usually signifies better performance.
- **Response Time:** This includes the average, median, and 95th percentile response times. It shows how quickly your application responds to requests during the test. Shorter response times are generally preferable.
- **Error Rate:** This metric shows the percentage of failed requests. An ideal load test has an error rate as close to zero as possible.
- **Number of Users:** Indicates the concurrency level, i.e., how many simulated users are interacting with the application simultaneously.
### Interpreting the Results
1. **Baseline Performance Understanding**
Start by establishing a baseline of how your app performs under normal conditions. Gather metrics such as average RPS and response times without any load. Compare these with the metrics obtained during the load test to identify performance degradation.
2. **Response Times Analysis**
Examine the response times in detail. If the 95th percentile response time significantly exceeds the average, your application may have scalability issues under high loads. This indicates that while most requests are handled efficiently, a small percentage are taking much longer, which could negatively impact user experience.
3. **Error Rate Scrutiny**
Investigate any error responses. Common Ruby on Rails issues under load include database timeouts, full memory, or asset delivery bottlenecks. Understanding the errors will guide you in pinpointing areas needing optimization.
4. **Comparison Against User Thresholds**
Compare your current performance against the expected number of users. If your application cannot handle the current user base without performance issues, it's a clear sign that improvements are needed.
### Visualizing the Data
LoadForge provides graphs and charts that can help visualize the data for easier interpretation. Here's how you can leverage them:
- **Timeline Graphs:** Shows how metrics like RPS and response times change over time during the test. Look for any spikes or drops that correlate with changes in load.
- **Error Graphs:** Helps you visualize when most errors occurred during the testing period. You might find that errors spike at certain load levels which can be critical for determining your app's capacity.
Here is an example visualization command that might be used to generate a response time graph:
<pre><code>
# Example: Plotting response time over the test duration in Python with matplotlib
import matplotlib.pyplot as plt
# Assuming 'results' is a loaded data containing the test results
plt.figure(figsize=(10, 5))
plt.plot(results['timestamp'], results['response_time'])
plt.title('Response Time Over Test Duration')
plt.xlabel('Time (s)')
plt.ylabel('Response Time (ms)')
plt.show()
</code></pre>
Based on the analysis:
By understanding these metrics and analyzing them effectively, you will be well-positioned to enhance the scalability and performance of your Ruby on Rails application.
After conducting comprehensive load testing on your Ruby on Rails application using LoadForge, you identify areas that could be improved to enhance system performance. The fine-tuning of your application involves both code-level and infrastructure optimizations. Implementing these changes can lead to significant increases in responsiveness and throughput, thereby ensuring a seamless user experience during high traffic periods. Here, we outline various strategies to optimize your Ruby on Rails performance post-load testing.
One of the most common areas for improvement in Ruby on Rails applications is making database interactions more efficient. Consider the following adjustments:
includes
method in ActiveRecord to retrieve all associated records you need in one query.
@orders = Order.includes(:user, :products).where(active: true)
Implementing caching strategies can drastically reduce the time taken to load pages by avoiding repeated computations and database calls.
Use Low-Level Caching: For data that seldom changes but is frequently accessed, consider using Rails caching.
Rails.cache.fetch("daily_specials") { Special.where('date = ?', Date.today).to_a }
Fragment Caching: Cache complex partial views that require intensive queries and computations, significantly reducing render time on subsequent requests.
Distribute system responsibilities across multiple servers:
Scale out by adding more machines to your pool of resources to handle increased load:
version: '3'
services:
web:
image: my-rails-app
deploy:
replicas: 4
resources:
limits:
cpus: '0.5'
memory: 1024M
By methodically applying these optimization strategies, your Ruby on Rails application can significantly boost its efficiency and scalability. Regular reviews and updates to your application's code and architecture, informed by ongoing load testing data, ensure the sustained performance necessary to handle growing user demands.
In the realm of load testing Ruby on Rails applications, real-world examples can vividly illustrate the transformative impact of targeted optimizations based on comprehensive test results. The following case studies demonstrate successful load testing scenarios facilitated by LoadForge, showcasing significant performance enhancements before and after implementing recommended changes.
A popular e-commerce platform built with Ruby on Rails experienced significant slowdowns during peak shopping periods, affecting user experience and sales.
Ensure the platform could handle up to 100,000 concurrent users during peak times, with a page load time not exceeding two seconds.
Post-optimization, the platform not only met but exceeded the initial performance goals, ensuring smooth operations during sales events.
A social media application built on Ruby on Rails saw performance dips during evening hours when user activity spiked.
Maintain a stable response time below three seconds during peak user activity hours, which typically sees a user base increase by four times.
The application was able to sustain four times the original load with improved response times and stability during peak hours.
These case studies underscore the effectiveness of using LoadForge for load testing and scalability improvements in Ruby on Rails applications. Continuous monitoring and testing, coupled with strategic interventions based on data, can lead to outstanding improvements in application performance and user satisfaction.
As Ruby on Rails applications evolve, so too should the strategies utilized to test their performance under various conditions. Beyond basic load testing, it's essential to employ more intricate techniques that simulate real-life scenarios—often more extreme—that your application might face. Here we'll explore three advanced load testing strategies crucial for robust Rails applications: stress testing, spike testing, and testing for long-term performance degradation.
Stress testing involves pushing your application to its limits to determine the maximum capacity it can handle. The goal is not just to observe when performance issues arise but to understand how the system fails under extreme stress and how gracefully it recovers, if at all. This can highlight synchronization issues, memory leaks, and other vulnerabilities that are not apparent under normal load conditions.
Here's an example of a simple stress test using LoadForge:
from locust import HttpUser, task, between
class StressTestUser(HttpUser):
wait_time = between(1, 2)
@task
def index_page(self):
self.client.get("/")
@task
def heavy_load(self):
self.client.get("/reports/heavy-data")
The key for stress testing is to incrementally increase the number of concurrent users (or load) until the system breaks. Configure LoadForge to gradually increase the load and observe how your Rails application handles it.
Spike testing examines the behavior of your Ruby on Rails application during sudden surges in traffic. This is particularly useful for applications that may experience periodic spikes due to promotional events or specific features becoming temporarily popular. It helps ensure your application can handle unexpected increases in load without degradation in performance or downtime.
For example, you might simulate a user spike with this configuration:
from locust import HttpUser, task, between
class SpikeTestUser(HttpUser):
wait_time = between(1, 3)
@task
def submit_form(self):
self.client.post("/submit", {"data": "value"})
In this test, you could configure LoadForge to suddenly increase the number of users from 100 to 1000 for a short period and then drop back down. This helps to test how quickly your application can scale, and how effectively resources are managed both during and after the spike.
Long-term performance degradation, often referred to as "Soak Testing", involves evaluating the application's performance when subjected to a typical load over an extended period. This can reveal issues that might not cause immediate failures but can lead to gradual performance degradation, such as slow memory leaks or database connection saturation.
For a soak test, you might use a Locust file like this:
from locust import HttpUser, task, between
class SoakTestUser(HttpUser):
wait_time = between(5, 10)
@task
def read_operations(self):
self.client.get("/resource")
Set LoadForge to run this test continuously over a period, maybe even a weekend, monitoring for any decline in response times or increase in error rates.
Advanced load testing strategies like stress testing, spike testing, and long-term performance degradation tests are invaluable for ensuring that your Ruby on Rails application can handle not just everyday traffic but also extraordinary conditions. These tests help preemptively fix potential issues before they affect users, ultimately aiding in providing a seamless user experience no matter what happens.
In this guide, we have explored the multifaceted approach needed to ensure that your Ruby on Rails application can sustain its performance and scalability under varying loads. From understanding the theoretical underpinnings of load testing to practically applying these concepts with LoadForge, each section has contributed to a comprehensive strategy aimed at enhancing your application's robustness and responsiveness.
Regular load testing using LoadForge has been emphasized as not merely an option but a necessity for maintaining an optimal user experience as your application grows and evolves. Implementing the locustfiles tailored for Ruby on Rails, as demonstrated, allows you to simulate and analyze real-world user interactions, which is crucial for identifying potential bottlenecks before they impact your users.
Continuous monitoring is another critical component that we discussed. It ensures that your application not only withstands occasional stress tests but also performs consistently well under everyday conditions. The integration of LoadForge's testing results into your ongoing performance optimization strategies means that you're not just fixing problems, but proactively enhancing your application's infrastructure.
Here is a quick recap of key practices to follow:
Successful application of these strategies not only secures a seamless user experience but also bolsters your infrastructure against future growth and unexpected surges in web traffic. Case studies detailed earlier illustrate the tangible benefits of embracing a rigorous load testing regimen, spotlighting significant improvements in handling real-world loads.
Load testing and performance optimization in Ruby on Rails is an ongoing journey, not a one-time task. By regularly employing the tools and techniques discussed, you'll sustain not just the performance, but also the success and reliability of your application in the long run.