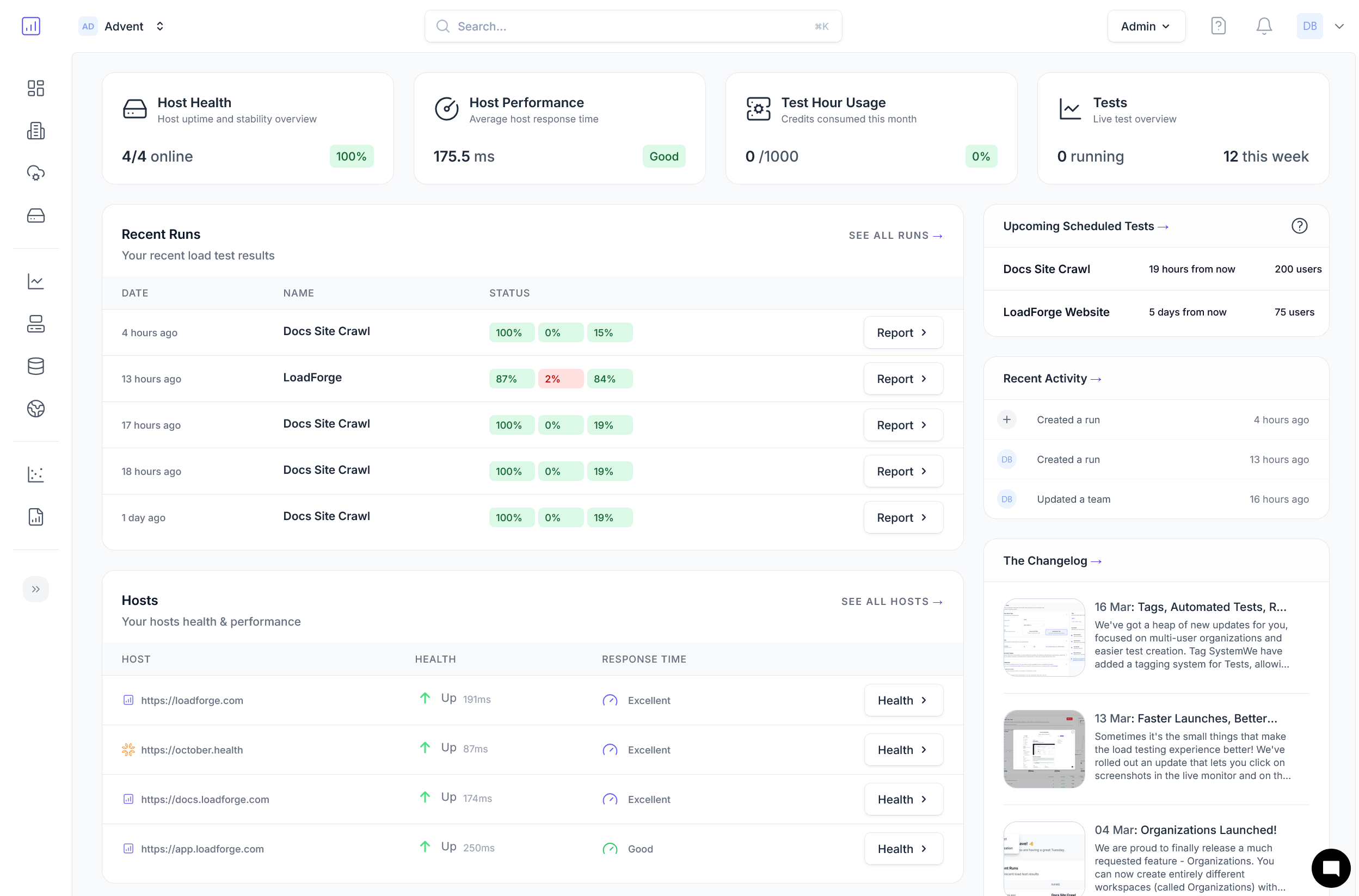
Updated UX & Activity Logging
We’ve rolled out a fresh update to LoadForge, focused on enhancing usability, improving how data is presented, and making the...
GraphQL, developed by Facebook in 2012 and released publicly in 2015, represents a powerful alternative to traditional RESTful APIs. Unlike REST, which uses a standardized but rigid approach to data retrieval and manipulation, GraphQL offers a flexible and efficient method...
GraphQL, developed by Facebook in 2012 and released publicly in 2015, represents a powerful alternative to traditional RESTful APIs. Unlike REST, which uses a standardized but rigid approach to data retrieval and manipulation, GraphQL offers a flexible and efficient method for interacting with web servers. This flexibility and efficiency stem from GraphQL's ability to allow clients to specify exactly what data they need, reducing both the number of requests and the volume of data transferred.
GraphQL is a query language for APIs and a runtime for executing those queries by using a type system you define for your data. Rather than multiple endpoints that return fixed data structures, a GraphQL server only exposes a single endpoint and responds to queries with precisely the data the client requested.
Feature | GraphQL | REST |
---|---|---|
Data Fetching | Single request to get multiple resources and nested resources | Multiple requests to different endpoints |
Over-fetching | Mitigated as clients request only the data they need | Common, as servers define returned data |
Under-fetching | Rare due to nested resource querying | Frequent, often requires additional requests |
API versioning | Not required, new fields and types can be added without impacting existing queries | Often needed, leading to versioned endpoints |
Data organization | Organized around a graph structure | Typically organized around endpoints |
Understanding these core principles of GraphQL is essential for planning effective load tests. By knowing where GraphQL excels and where it might face performance bottlenecks, such as in complex nested queries or large single requests, testers can craft more targeted and meaningful tests, ensuring that APIs perform well under the expected load conditions of real-world applications. This foundational knowledge will guide us as we delve deeper into the specifics of load testing for GraphQL APIs in the following sections.
Load testing is an essential aspect of developing robust web services, ensuring that APIs can handle high user loads without compromising on performance. For GraphQL, a powerful query language designed to make APIs fast, flexible, and developer-friendly, specific load testing considerations must be addressed due to its unique operational characteristics.
Unlike RESTful APIs, where endpoints return fixed data structures, GraphQL allows clients to request exactly the data they need, nothing more and nothing less. This flexibility, though powerful, introduces distinct challenges in load testing:
Complex and Varied Queries: Each GraphQL request can be vastly different, with some queries requesting numerous fields nested deeply. This variability can lead to significant differences in performance, which can be challenging to anticipate and test effectively.
Variable Response Times: Due to the nature of GraphQL allowing customizable queries, response times can greatly vary from one query to another. A query asking for minimal data can be swift, while a more complex nested query can be substantially slower.
Over and Under Fetching: While GraphQL aims to solve the issues of over-fetching and under-fetching (common in RESTful APIs), poorly designed queries can still result in inefficient operations that burden the server.
N+1 Problem: This is a common issue where a query leads to multiple round trips to a database or backend, degrading performance. It occurs when a field needs to be resolved by making additional requests based on the result of a parent field.
These unique features of GraphQL necessitate a tailored approach to load testing.
Performance bottlenecks in GraphQL can emerge from several areas:
Query Complexity: Complex queries can consume more resources. Tools like query cost analysis can help in setting complexity limits on queries.
Database Load: GraphQL can intensify load on your database, especially with complex nested queries.
API Layer: The layer that interprets GraphQL queries can become a bottleneck, particularly if caching strategies are ineffective or if the schema is poorly designed.
Network Performance: Since GraphQL can reduce the number of requests by enabling more precise data retrieval, the impact of network latency may be heightened for sprawling queries.
Effective load testing of GraphQL APIs requires simulating real-world usage scenarios. Key considerations include:
Query Variability: Tests should cover a range of query types, from simple to highly complex, to effectively gauge performance across different user demands.
Concurrent Users: Simulate scenarios with varying numbers of concurrent users making requests to understand how well the API scales.
Persistence Layer Stress: Since GraphQL can lead to complex interactions with the database, stress testing this layer will reveal potential degradation points.
Error Rate Analysis: Monitor and analyze errors and timeouts to improve error handling and resilience under high load.
By acknowledging these factors and integrating them into your load testing strategy, you can ensure that your GraphQL service remains responsive and efficient under various real-world conditions. This preparatory work not only optimizes performance but also enhances the user experience by creating a more stable and reliable API service.
Before diving into load testing your GraphQL APIs, it's crucial to set up a suitable environment that allows for effective simulation and analysis of your application's performance under stress. This setup ranges from creating a LoadForge account to ensuring that all necessary local development tools and dependencies are properly configured. Let's walk through the preliminary steps needed to commence load testing utilizing the LoadForge platform.
To begin, you will need to create an account on LoadForge. This is a straightforward process:
Once you have an account, familiarize yourself with the dashboard as you will use it to create and manage your load tests.
Next, you'll want to ensure that your local development environment is set up properly. For testing GraphQL APIs, you’ll need the following tools:
Python: Load testing scripts for LoadForge are written in Python, necessitating its presence on your machine. Install Python from the official Python website.
Locust: This is the core tool used for scripting load tests in LoadForge. Install it using pip:
pip install locust
IDE or Text Editor: Utilize an IDE or text editor that you are comfortable with for writing Python scripts, such as VSCode, PyCharm, or Sublime Text.
Ensure you have proper access to the GraphQL endpoint you will be testing. This may require:
If your GraphQL implementation relies on particular schemas or specific queries, make sure you have them downloaded and easily accessible. These will aid in creating realistic test cases.
If the GraphQL API requires authentication, you'll need to manage this within the tests:
Here is an example snippet to configure authentication in your Locustfile:
from locust import HttpUser, task, between
class AuthenticatedGraphQLUser(HttpUser):
wait_time = between(1, 5)
def on_start(self):
self.client.headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
@task
def execute_query(self):
query = '{ users { id name email posts { title } } }'
self.client.post('/graphql', json={'query': query})
Finally, before running intensive load tests, make sure your local or staging environment is running and accessible. It is usually better to avoid testing on production systems to prevent any disruptions.
With these steps completed, your environment is now ideally configured to start writing the specific locust tests for your GraphQL APIs. This foundational setup ensures that subsequent stages of testing execution and analysis can proceed smoothly without interruptions.
Designing effective load tests for GraphQL APIs involves several crucial steps, from selecting the right queries to simulate user actions to determining the optimal load levels and deciding on the pertinent metrics to track. In this section, we will guide you through designing robust tests to ensure your GraphQL APIs can perform under pressure and deliver the expected user experience.
The first step in designing your test is to identify which queries are pivotal to your application's functionality. Testing these queries ensures that your most critical operations can withstand the anticipated load. Consider the following types of queries for inclusion in your tests:
Example of a simple query test in a locustfile:
from locust import HttpUser, task, between
class GraphQLUser(HttpUser):
wait_time = between(1, 2) # Simulate real user wait time between tasks
@task
def fetch_users(self):
# A simple query to fetch users data
query = '''
{
users {
id
name
email
}
}
'''
self.client.post('/graphql', json={'query': query})
Determining the load levels involves understanding how much traffic your API must handle. This could range from a few users to thousands depending on your use case. Key considerations include:
Monitoring the right metrics will help you gauge the health and responsiveness of your GraphQL API under load. The crucial metrics to monitor include:
Here's an example snippet for adding custom logging to your locustfile, which can help in capturing the above metrics:
from locust import events
@events.request.add_listener
def log_request(request_type, name, response_time, response_length, exception, **kwargs):
if exception:
print(f"Request failed: {exception}")
else:
print(f"Response time: {response_time}ms, Throughput: {response_length} bytes")
Properly designing your load tests by selecting significant queries, carefully planning the test loads, and focusing on critical performance metrics will provide a robust framework to ensure your GraphQL APIs can handle real-world usage scenarios. This structured approach to testing will help illuminate potential performance issues before they impact your users, leading to a more reliable and efficient application.
Writing an effective Locustfile is crucial for conducting meaningful load tests on your GraphQL APIs. In this section, we will guide you through crafting a Locustfile tailored for GraphQL, focusing on query payloads, variable management, and authentication handling.
One of the key elements in load testing a GraphQL API is creating the correct query payload. In GraphQL, unlike REST, you send a single query that can fetch an extensive amount of related data. Here’s how you can structure a basic GraphQL query in your Locustfile:
from locust import HttpUser, task, between
class GraphQLUser(HttpUser):
wait_time = between(1, 5)
@task
def execute_query(self):
query = """
{
users {
id
name
email
posts {
title
}
}
}
"""
self.client.post('/graphql', json={'query': query})
Sometimes, you need to test queries that require input variables. Managing these variables effectively can help simulate more realistic and varied test cases. Here’s how you integrate variables into your queries:
@task
def execute_variable_query(self):
query = """
query GetUser($userID: ID!) {
user(id: $userID) {
id
name
email
}
}
"""
variables = {'userID': '1'}
self.client.post('/graphql', json={'query': query, 'variables': variables})
Many GraphQL APIs require authentication. Handling authentication in your Locust tests can vary depending on the method (e.g., token-based, OAuth). Below is an example of how to handle token-based authentication:
class AuthenticatedGraphQLUser(HttpUser):
wait_time = between(1, 5)
def on_start(self):
# You might retrieve the token via an API call or environment variable
self.token = 'your_access_token'
@task
def execute_auth_query(self):
query = """
{
authenticatedEndpoint {
id
protectedField
}
}
"""
headers = {'Authorization': f'Bearer {self.token}'}
self.client.post('/graphql', headers=headers, json={'query': query})
'query'
and optional 'variables'
fields. Make sure to structure your JSON correctly depending on the GraphQL server’s requirements.self.client.post
.wait_time
and task frequency according to realistic usage patterns. Real-world users don’t hit servers at constant intervals, so between
helps simulate this irregularity.By following these guidelines and varying the complexity and structure of your queries, you will be able to compose a comprehensive Locustfile that effectively tests your GraphQL API's performance under load.
Once you've designed your Locustfile for testing GraphQL APIs, deploying and running your load test with LoadForge is your next essential step. This section walks you through the process of executing your test, scaling your simulations to different user loads, and interpreting the preliminary results.
Upload Your Locustfile: Begin by uploading your Locustfile to your LoadForge account. Ensure the script includes all necessary queries, configurations, and user behaviors that you're planning to test.
Configure Test Settings:
Example of test settings:
Number of Users: 500
Spawn Rate: 50 users per second
Host URL: https://api.yourgraphqlservice.com/graphql
Start the Test: With everything configured, click the "Run Test" button on LoadForge. Your test will begin executing, and LoadForge will start logging the performance of your GraphQL API under load.
Scaling your tests involves simulating different levels of user load to observe how your application behaves under varying conditions. You may start with a small number of users to ensure basic stability before escalating the numbers to stress test your system's limits.
Once a test completes, LoadForge provides comprehensive reports that help you understand how your GraphQL API performed under stress. Key metrics to consider include:
Review the detailed graphs and logs provided by LoadForge to spot trends and anomalies. For instance, significantly longer response times for specific queries can indicate a need for query optimization or increased resource allocation.
Running your load test efficiently requires a careful balance between realism in user simulation and the insightful analysis of the results. By scaling your tests thoughtfully and interpreting the data judiciously, you can ensure that your GraphQL API is robust and responsive under various load conditions. This proactive approach will help in sustaining optimal API performance and enhancing user satisfaction in real-world scenarios.
After successfully executing your GraphQL API load tests on LoadForge, the next crucial step is to analyze the outcomes. This step is essential to understand how your system behaves under stress and to identify potential bottlenecks or performance issues. This section provides an overview of how to interpret the detailed reports generated by LoadForge, pinpoint potential problem areas, and optimize your API performance accordingly.
Once your load test concludes, LoadForge provides comprehensive reports that include various metrics. Key metrics to focus on include:
Understanding these metrics helps in evaluating the scalability and reliability of your GraphQL API under various load conditions.
The next step involves drilling down into the data to identify specific bottlenecks. Some common areas where bottlenecks may occur include:
Use the detailed report graphs and logs provided by LoadForge to locate these bottlenecks. For example, if a particular query consistently shows slow response times across different load levels in the graph, it’s likely a candidate for optimization.
Based on the analysis, consider the following common performance optimizations:
Optimizing performance is an iterative process. After making changes based on your initial analysis, it's important to run additional load tests to evaluate the impact of those changes. This will help you understand whether the optimizations have had the desired effect or if further adjustments are needed.
Analyzing the results from your load tests through LoadForge allows you to not only assure your GraphQL API’s performance under various conditions but also offers actionable insights into optimizing and scaling your application effectively. By continually assessing and optimizing, you can ensure that your API remains robust and responsive, providing a seamless experience to your users.
Load testing GraphQL APIs can bring to light a variety of challenges and issues that may not be evident during regular API testing. Below are some of the common pitfalls you might encounter, along with practical advice on troubleshooting and resolving these issues.
One frequently encountered problem when load testing GraphQL APIs is receiving timeout errors. These can occur if the server takes too long to resolve a request due to complex queries or slow database responses.
Troubleshooting steps:
Optimize the GraphQL queries by reducing the fields requested or breaking down complex queries into smaller, simpler ones.
Increase timeout settings in your Locustfile, but be cautious as this could mask underlying performance problems. For example:
class GraphQLUser(HttpUser):
timeout = 30 # Timeout set to 30 seconds
@task
def execute_query(self):
query = '{ users { id name email } }'
self.client.post('/graphql', json={'query': query}, timeout=self.timeout)
Consider improving database performance or using caching mechanisms to reduce response times.
Complex queries in GraphQL can significantly impact the performance of your API, leading to errors related to query depth or complexity.
Troubleshooting steps:
With GraphQL APIs, authentication issues can arise, especially under load when tokens or session data may not persist as expected.
Troubleshooting steps:
Ensure authentication tokens are managed correctly in your Locust tasks. Use session handling to maintain token validity:
class GraphQLUser(HttpUser):
@task
def login(self):
response = self.client.post('/login', json={'username': 'user', 'password': 'pass'})
self.token = response.json()['token']
@task
def execute_query(self):
headers = {'Authorization': f'Bearer {self.token}'}
query = '{ users { id } }'
self.client.post('/graphql', headers=headers, json={'query': query})
Review the API server’s authentication logs to identify failed attempts or token rejections.
Under high load, your GraphQL server might become overwhelmed, leading to slow responses or outright failure.
Troubleshooting steps:
Sometimes, issues arise intermittently due to network latency or third-party services failing under load.
Troubleshooting steps:
By identifying these common issues and implementing the associated troubleshooting steps, you can ensure that your GraphQL API remains robust and performs efficiently under various load conditions. Always aim to simulate real-world usage scenarios as closely as possible during your tests to uncover and mitigate potential issues effectively.
Comprehensive Query Coverage: Ensure your load tests cover a wide range of GraphQL queries, mutations, and subscriptions that are representative of real-world usage. This helps in identifying weaknesses across all parts of your API.
Variable Load Levels: Test with varying levels of load to understand how your system behaves under different conditions. Start with a low number of users and gradually increase the count to assess scalability and performance thresholds.
Realistic Data Volumes: Utilize realistic data volumes in your testing environment. The performance can significantly differ when handling large volumes of data, which is common in production.
Efficient Query Design: Optimize GraphQL queries to fetch only the necessary data. Avoid excessive nested queries in tests unless they reflect actual usage scenarios, as these can skew performance metrics.
State Management: Consider the state of the application during testing. Stateful interactions, where queries depend on previous data mutations, should be accurately represented in your locustfiles.
Monitor and Analyze: Use LoadForge's detailed analytics to monitor key metrics like response times, error rates, and throughput. Establish baseline performance metrics and compare them after each test to measure improvements or regressions.
Nested Queries: Handling deeply nested queries can be particularly challenging. These queries can generate massive and deeply nested JSON responses, putting a strain on both the server and the network. When designing locustfiles for these scenarios, ensure you measure the impact extensively.
from locust import HttpUser, task, between
class NestedQueryUser(HttpUser):
wait_time = between(1, 5)
@task
def nested_query(self):
query = '''
{
user(id: "1") {
posts {
title
comments {
content
author {
name
}
}
}
}
}
'''
self.client.post('/graphql', json={'query': query})
Hybrid Environment Testing (REST and GraphQL): Many modern applications use a combination of RESTful APIs and GraphQL. It’s important to test how these interact and affect each other's performance. Design scenarios where REST and GraphQL requests are made in parallel to mimic real user interactions.
Authentication and Authorization: Testing authenticated queries is crucial as it adds additional overhead to the server. Simulate authenticated sessions properly in your locustfiles to gauge the impact of security mechanisms on performance.
Subscription Testing: Unlike queries and mutations, GraphQL subscriptions maintain a steady connection to push updates to the client. Use LoadForge’s capabilities to test subscriptions by simulating WebSocket or similar persistent connections, which are essential for real-time functionalities.
Error Handling: Introduce errors in your queries to test the robustness of the GraphQL server. Ensuring your system gracefully handles invalid queries or data helps in maintaining stability under adverse conditions.
By following these best practices and exploring more complex scenarios, you'll be better equipped to ensure your GraphQL APIs are not just functioning but are also optimized and resilient under varied and intense load conditions. Continually refine your approach based on test results, and keep up with new GraphQL features and best practices to maintain peak performance.