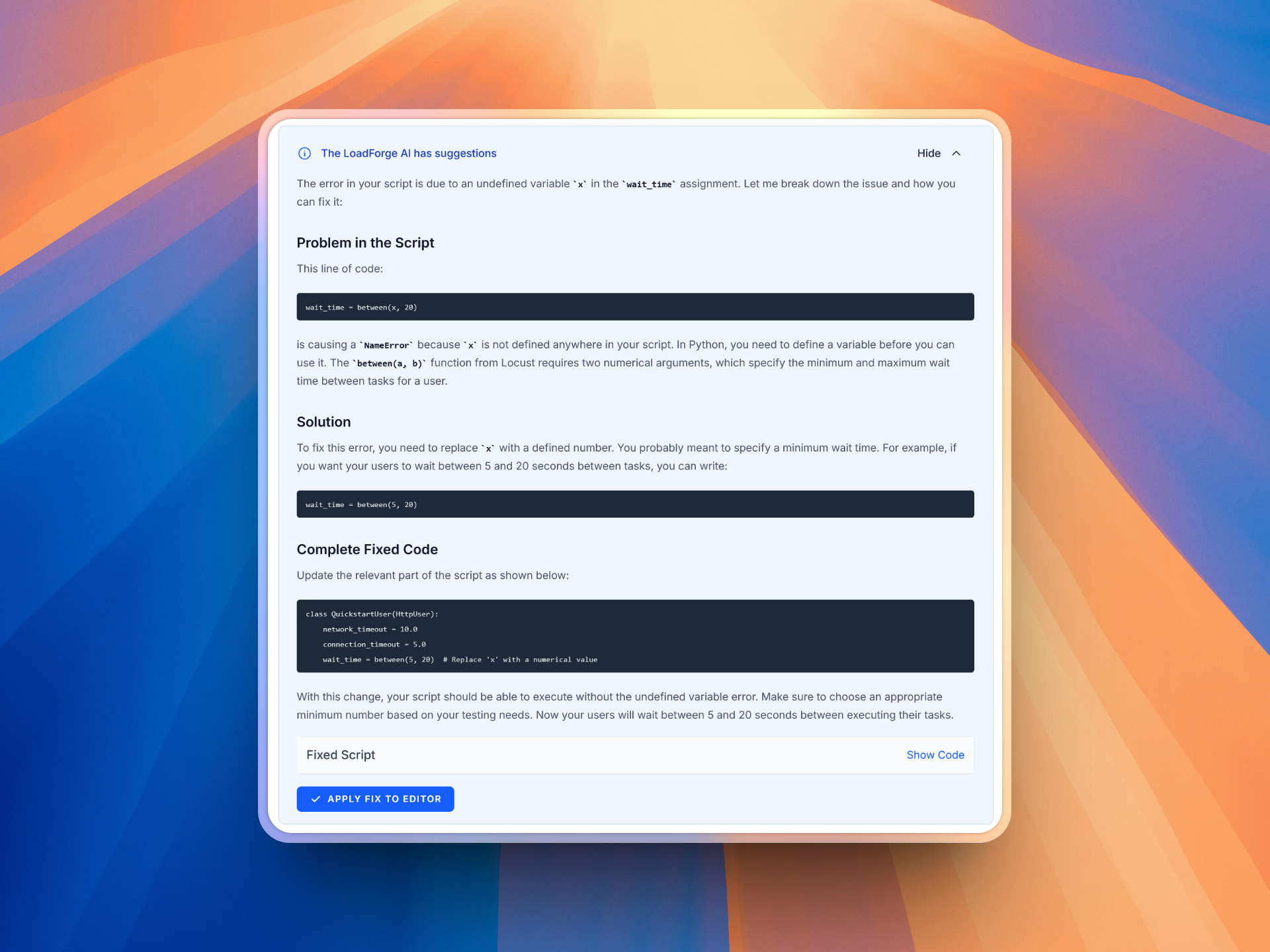
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
## Understanding Spring HTTP Server Basics In order to effectively optimize the performance of your Spring HTTP server, it is essential to first have a solid understanding of its core components, architecture, and request-handling mechanisms. This foundational knowledge will not...
In order to effectively optimize the performance of your Spring HTTP server, it is essential to first have a solid understanding of its core components, architecture, and request-handling mechanisms. This foundational knowledge will not only help identify potential bottlenecks but also guide you in making informed decisions when applying optimization techniques. In this section, we'll explore these key areas to set the stage for why performance optimization is crucial.
The Spring HTTP server is built on several fundamental components that work together to handle HTTP requests and responses. These components include:
Consider a basic controller example:
@RestController
@RequestMapping("/api")
public class ExampleController {
@GetMapping("/greeting")
public ResponseEntity greet() {
return ResponseEntity.ok("Hello, World!");
}
}
In this example, the DispatcherServlet routes a GET request to /api/greeting
to the greet
method in ExampleController
.
The architecture of a Spring HTTP server generally follows the layered architecture pattern, which provides separation of concerns and promotes maintainability. The primary layers include:
The following diagram represents this layered architecture:
+--------------------+
| Presentation Layer |
| (Controllers) |
+--------------------+
|
+--------------------+
| Service Layer |
+--------------------+
|
+--------------------+
| Repository Layer |
+--------------------+
Spring HTTP servers use a multi-step process to handle requests efficiently. The typical steps include:
DispatcherServlet
.DispatcherServlet
consults HandlerMapping
to determine the appropriate controller for the request.DispatcherServlet
.ViewResolver
and View
components together render the final response to be sent back to the client.Here is how these steps are translated into code:
// Controller
@RestController
@RequestMapping("/api")
public class ExampleController {
private final GreetingService greetingService;
public ExampleController(GreetingService greetingService) {
this.greetingService = greetingService;
}
@GetMapping("/greeting")
public ResponseEntity greet() {
String greeting = greetingService.getGreeting();
return ResponseEntity.ok(greeting);
}
}
// Service Layer
@Service
public class GreetingService {
public String getGreeting() {
return "Hello, World!";
}
}
Understanding the basics of the Spring HTTP server illuminates why performance optimization is fundamental. As traffic grows and workloads increase, inefficiencies in request handling, slow data access, and suboptimal configurations can significantly degrade performance, leading to:
By thoroughly grasping these core concepts, you'll be better equipped to implement the strategies discussed in subsequent sections. Performance optimization isn't just about fine-tuning—it's about ensuring a seamless, scalable, and efficient experience for your users.
## Evaluating Performance Metrics
Understanding and evaluating performance metrics is crucial for optimizing your Spring HTTP server. Key metrics such as response time, throughput, CPU usage, and memory consumption offer insights into how well your server performs under load. Measuring and analyzing these metrics enables you to identify bottlenecks, allocate resources more effectively, and provide a smoother user experience.
### Key Performance Metrics
#### Response Time
Response time is the duration it takes for the server to process a request and send a response back to the client. Lower response times typically indicate better performance and improved user satisfaction.
**How to Measure:**
Use tools like Spring Boot Actuator and Micrometer to capture response time metrics.
<pre><code>
@Configuration
public class MonitoringConfig {
@Bean
public MeterRegistryCustomizer<MeterRegistry> responseTimeMetrics() {
return registry -> registry.config()
.commonTags("application", "spring-http-server");
}
}
</code></pre>
#### Throughput
Throughput measures the number of requests the server can handle per second. Higher throughput is generally a sign of a more efficient and capable server.
**How to Measure:**
Monitor the total number of requests processed over time using JMX (Java Management Extensions) or tools like Prometheus and Grafana.
<pre><code>
@RestController
public class MonitoringController {
@GetMapping("/metrics/throughput")
public String getThroughput() {
long throughput = ... // Logic to calculate throughput
return "Throughput: " + throughput + " requests per second";
}
}
</code></pre>
#### CPU Usage
CPU usage indicates how much processing power the server is using. High CPU usage can degrade performance, especially under heavy load. Balancing CPU utilization ensures that your server can handle multiple requests efficiently.
**How to Measure:**
Use JVM tools or application performance monitoring solutions like New Relic, AppDynamics, or Dynatrace to capture CPU usage metrics.
<pre><code>
@SpringBootApplication
public class CPUMonitoringApp {
public static void main(String[] args) {
SpringApplication.run(CPUMonitoringApp.class, args);
OperatingSystemMXBean osBean = ManagementFactory.getPlatformMXBean(
OperatingSystemMXBean.class);
System.out.println("CPU Load: " + osBean.getSystemCpuLoad());
}
}
</code></pre>
#### Memory Consumption
Memory consumption refers to the amount of RAM your Spring HTTP server uses. Efficient memory management is key to preventing issues like memory leaks and providing consistent performance.
**How to Measure:**
Monitor heap and non-heap memory usage through the JVM’s memory management tools or advanced monitoring platforms.
<pre><code>
public class MemoryMetrics {
public static void main(String[] args) {
MemoryMXBean memoryBean = ManagementFactory.getMemoryMXBean();
System.out.println("Heap Memory Usage: " + memoryBean.getHeapMemoryUsage());
System.out.println("Non-Heap Memory Usage: " + memoryBean.getNonHeapMemoryUsage());
}
}
</code></pre>
### Importance of Evaluating Performance Metrics
Evaluating these metrics allows you to:
- **Diagnose Problems:** Identify and rectify performance bottlenecks like slow database queries or inefficient code.
- **Optimize Resources:** Allocate CPU and memory more effectively to handle concurrent requests smoothly.
- **Improve Scalability:** Ensure the server can handle increased load without compromising performance.
- **Enhance User Experience:** Deliver faster response times which directly translate to better user satisfaction.
Understanding these metrics and regularly monitoring them provides a solid foundation for any further optimization efforts.
In the following sections of this guide, we will delve into specific techniques and best practices to improve these key performance metrics, ensuring a robust and responsive Spring HTTP server.
Effective thread pool management is essential for maximizing the performance of Spring HTTP servers. A well-tuned thread pool can ensure that your server handles concurrent requests efficiently, reduces latency, and improves overall throughput. In this section, we will explore key configurations for thread pools, including max pool size, queue capacity, and thread keep-alive time. We will also discuss best practices and common pitfalls to avoid.
Spring HTTP servers typically use a thread pool to manage concurrent request processing. The thread pool is responsible for allocating threads to handle incoming HTTP requests, thus enabling parallel processing and efficient resource utilization. Below are the essential thread pool configurations:
Using Spring Boot, you can configure your thread pool in the application.properties
or application.yml
file. Here is an example of configuring a thread pool using application.properties
:
spring.task.execution.pool.core-size=10
spring.task.execution.pool.max-size=50
spring.task.execution.pool.queue-capacity=100
spring.task.execution.pool.keep-alive=60s
Alternatively, using application.yml
:
spring:
task:
execution:
pool:
core-size: 10
max-size: 50
queue-capacity: 100
keep-alive: 60s
Determine Optimal Pool Size:
Optimal Thread Pool Size = Number of Available Cores * (1 + (Wait time / Service time))
.Setting Core and Max Pool Size:
core-size
to a value that ensures sufficient threads are available for steady-state workloads.max-size
to handle peak loads while avoiding resource exhaustion.Tuning Queue Capacity:
Adjusting Keep-Alive Time:
Overprovisioning Threads:
max-size
too high can lead to excessive context switching and resource contention, degrading performance.Ignoring Workload Characteristics:
Incorrect Queue Selection:
SynchronousQueue
) vs. a bounded queue (LinkedBlockingQueue
) should be chosen based on the nature of the workload and performance targets.Consider a Spring Boot application handling HTTP requests that are mainly I/O-bound. Below is an example configuration for such a scenario:
spring.task.execution.pool.core-size=20
spring.task.execution.pool.max-size=100
spring.task.execution.pool.queue-capacity=200
spring.task.execution.pool.keep-alive=120s
In this setup, we are preparing to handle up to 100 concurrent threads with a core size of 20 for steady-state operations and a queue capacity of 200 to buffer incoming requests during peak times. The keep-alive time of 120 seconds ensures that the threads do not terminate too quickly during brief periods of inactivity.
Optimizing thread pool settings is a vital aspect of Spring HTTP server performance tuning. By carefully configuring the thread pool size, queue capacity, and keep-alive time, you can enhance your server's ability to manage concurrent requests efficiently. Always consider your specific workload characteristics and perform iterative tuning to achieve the best results. In the following sections, we will delve deeper into other performance optimization strategies to further enhance your Spring HTTP server's efficiency.
Implementing efficient caching mechanisms within a Spring HTTP server is crucial for reducing load and improving response times. Caching can help minimize database calls, reduce network latency, and compress data. In this section, we will explore various caching techniques in Spring, including the use of EhCache, Redis, and HTTP caching headers.
EhCache is a widely used, Java-based caching solution that is easy to integrate with Spring. It can store frequently accessed data in memory, thereby reducing the time it takes to retrieve data from the database.
First, include the EhCache dependency in your pom.xml
:
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>2.10.6</version>
</dependency>
Next, configure EhCache in your Spring application context:
<bean id="cacheManager" class="org.springframework.cache.ehcache.EhCacheCacheManager">
<property name="cacheManager" ref="ehcache"/>
</bean>
<bean id="ehcache" class="org.springframework.cache.ehcache.EhCacheManagerFactoryBean">
<property name="configLocation" value="classpath:ehcache.xml"/>
</bean>
In the ehcache.xml
file:
<ehcache>
<cache name="myCache"
maxEntriesLocalHeap="1000"
timeToLiveSeconds="3600">
</cache>
</ehcache>
You can now use the cache in your Spring components:
@Service
public class MyService {
@Cacheable("myCache")
public String getData(String key) {
// Simulate a time-consuming operation
return "Data for " + key;
}
}
Redis is a powerful, in-memory key-value store that acts as a low-latency cache. It can be used to store API responses, session data, and other read-heavy data structures.
Include the Redis dependency in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
Configure Redis in your application.properties
:
spring.redis.host=localhost
spring.redis.port=6379
Create a Redis configuration class:
@Configuration
@EnableCaching
public class RedisConfig extends CachingConfigurerSupport {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
return new LettuceConnectionFactory();
}
@Bean
public RedisTemplate<String, Object> redisTemplate() {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory());
return template;
}
@Bean
@Override
public CacheManager cacheManager() {
return RedisCacheManager.builder(redisConnectionFactory())
.cacheDefaults(RedisCacheConfiguration.defaultCacheConfig().entryTtl(Duration.ofHours(1)))
.build();
}
}
Use the cache in your service:
@Service
public class MyService {
@Cacheable(value = "myCache", key = "#key")
public String getData(String key) {
// Simulate a time-consuming operation
return "Data for " + key;
}
}
HTTP caching headers allow client-side caches (browsers, proxies) to store responses and reuse them without contacting the server.
Use @ControllerAdvice
and ResponseEntity
to set cache headers for specific endpoints:
@RestController
public class MyController {
@GetMapping("/data/{key}")
public ResponseEntity<String> getData(@PathVariable String key) {
HttpHeaders headers = new HttpHeaders();
headers.setCacheControl(CacheControl.maxAge(60, TimeUnit.SECONDS));
return ResponseEntity.ok()
.headers(headers)
.body("Data for " + key);
}
}
ETags (Entity Tags) are a way to validate resources. They help in avoiding transmission of the same data if it hasn't changed.
@GetMapping("/data/{key}")
public ResponseEntity<String> getData(@PathVariable String key, HttpServletRequest request) {
String data = "Data for " + key;
String eTag = Integer.toHexString(data.hashCode());
if (eTag.equals(request.getHeader("If-None-Match"))) {
return ResponseEntity.status(HttpStatus.NOT_MODIFIED).eTag(eTag).build();
}
return ResponseEntity.ok().eTag(eTag).body(data);
}
Efficient use of caching plays a significant role in optimizing the performance of Spring HTTP servers. By integrating in-memory caches like EhCache and Redis, along with leveraging HTTP caching headers effectively, you can drastically reduce load times and server load, providing a smoother experience for users.
Remember to validate your caching strategy for your specific use case and continuously monitor its effectiveness.
Continue to the next section to learn more about optimizing your database connection pools for better performance.
Efficient database connection management is critical to the performance of any Spring HTTP server. Improper handling of database connections can lead to sluggish response times, increased latency, and resource exhaustion. In this section, we'll discuss optimizing database connections through the use of connection pools, with a particular focus on HikariCP, a popular and high-performance JDBC connection pool. We'll delve into key connection pool settings, help you choose appropriate pool sizes, and outline strategies for avoiding common bottlenecks.
A connection pool is a cache of database connections. By reusing connections from this pool, we can avoid the overhead of creating and closing connections repeatedly, thus enhancing performance and scalability. HikariCP is known for its reliability, speed, and simplicity, making it an excellent choice for Spring applications.
Spring Boot simplifies the process of integrating HikariCP. By default, if HikariCP is available in the classpath, Spring Boot uses it for database connections. Here is a basic configuration in application.properties
:
# HikariCP Configuration
spring.datasource.hikari.maximum-pool-size=10
spring.datasource.hikari.minimum-idle=5
spring.datasource.hikari.idle-timeout=30000
spring.datasource.hikari.connection-timeout=20000
spring.datasource.hikari.max-lifetime=1800000
Let's break down essential HikariCP settings and how they impact your application:
Determining the right pool size depends on various factors such as the nature of your application, database capacity, and expected load. Here are some guidelines:
Here are some best practices to avoid common connection pool-related bottlenecks:
Monitor Connection Pool Metrics: Use Spring Actuator or other monitoring tools to keep an eye on the performance metrics of your connection pool. Look for signs of contention or starvation.
Connection Leak Detection: Enable leak detection to identify connections that are not closed properly. In HikariCP, you can configure this using:
spring.datasource.hikari.leak-detection-threshold=2000
Optimize Queries: Inefficient SQL queries can keep connections busy for longer than necessary. Optimize your queries and use indexes wisely.
Connection Validation: Ensure connections are validated before use to avoid processing requests with a broken connection.
In conclusion, tuning your database connection pool is a crucial step towards achieving optimal performance in a Spring HTTP server. By carefully configuring HikariCP and continuously monitoring your application's database interactions, you can prevent bottlenecks and ensure high responsiveness and reliability. Make use of load testing with LoadForge to validate your optimizations and achieve a well-rounded, high-performance setup.
When dealing with large payloads in a Spring HTTP server, it’s essential to adopt strategies that maintain performance and ensure resource efficiency. This section presents key techniques for effectively managing large payloads, including chunked transfer encoding, using InputStream
for large file uploads, and optimizing JSON serialization. Effectively handling heavy loads prevents server bottlenecks and contributes to a smooth user experience.
Chunked transfer encoding allows the server to send a response in chunks rather than as a single block, which can be beneficial for large payloads by reducing the memory footprint and enabling clients to start processing data sooner. To enable chunked transfer encoding in Spring:
Controller Configuration:
Ensure your Spring controller returns a ResponseEntity
or any other OutputStream
-based response.
@GetMapping("/largePayload")
public ResponseEntity<StreamingResponseBody> getLargePayload() {
StreamingResponseBody stream = outputStream -> {
// Write large data to outputStream
for (int i = 0; i < 1000; i++) {
outputStream.write(("Chunk " + i).getBytes());
outputStream.flush();
}
};
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_TYPE, MediaType.TEXT_PLAIN_VALUE)
.body(stream);
}
Client-Side Implementation: Ensure the client can handle chunked responses effectively.
InputStream
for Large File UploadsUploading large files can be memory-intensive if not handled properly. Using InputStream
for file uploads can help manage resources efficiently. Spring provides support for file uploads using MultipartFile
which can be converted to an InputStream
.
Controller for File Upload:
@PostMapping("/upload")
public ResponseEntity<String> handleFileUpload(@RequestParam("file") MultipartFile file) {
try (InputStream inputStream = file.getInputStream()) {
// Process the input stream as needed
} catch (IOException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("File upload failed");
}
return ResponseEntity.ok("File upload successful");
}
File Processing: Process the file in a way that minimizes memory usage, such as reading it in smaller chunks.
Handling large JSON payloads efficiently requires optimizing serialization and deserialization processes to improve performance and reduce memory usage. Best practices include:
Use Jackson Streaming API:
For large JSON payloads, use Jackson's streaming API (JsonParser
and JsonGenerator
) to process data as a stream.
@PostMapping("/processJson")
public ResponseEntity<String> processLargeJson(@RequestBody InputStream inputStream) {
try (JsonParser parser = new ObjectMapper().getFactory().createParser(inputStream)) {
while (parser.nextToken() != null) {
// Process JSON token
}
} catch (IOException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("JSON processing failed");
}
return ResponseEntity.ok("JSON processing successful");
}
Configure Jackson for Performance:
Tweak Jackson's default settings for better performance with large payloads by enabling features like USE_BIG_DECIMAL_FOR_FLOATS
.
ObjectMapper mapper = new ObjectMapper();
mapper.enable(DeserializationFeature.USE_BIG_DECIMAL_FOR_FLOATS);
Effectively managing large payloads is critical for maintaining the performance and reliability of a Spring HTTP server. By leveraging chunked transfer encoding, utilizing InputStream
for file uploads, and optimizing JSON serialization, you can ensure your Spring HTTP server handles heavy loads efficiently. Implement these strategies to enhance server responsiveness and resource usage, providing a smoother experience for end users.
As web applications grow, managing server loads efficiently becomes increasingly crucial. One powerful technique for enhancing the scalability and responsiveness of your Spring HTTP server is leveraging asynchronous request processing. By using asynchronous operations, you can handle more requests concurrently without blocking server threads, leading to better resource utilization and improved overall performance.
Asynchronous requests are particularly beneficial in scenarios where:
Spring provides extensive support for asynchronous request processing. Below are practical steps and examples to help you configure and implement this in your Spring HTTP server.
First, ensure that your Spring application has asynchronous support enabled. This typically involves annotating your configuration class with @EnableAsync
:
@Configuration
@EnableAsync
public class AsyncConfig {
// ThreadPool configuration and other settings (optional)
}
Next, define a custom Executor
bean to manage asynchronous tasks. This executor can be fine-tuned based on your application's requirements:
@Configuration
@EnableAsync
public class AsyncConfig {
@Bean(name = "taskExecutor")
public Executor taskExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(5);
executor.setMaxPoolSize(10);
executor.setQueueCapacity(25);
executor.setThreadNamePrefix("Async-");
executor.initialize();
return executor;
}
}
Use the @Async
annotation to mark methods that should run asynchronously. These methods will be executed in a separate thread managed by the configured Executor
:
@Service
public class AsyncService {
@Async("taskExecutor")
public CompletableFuture asyncMethod(String input) {
// Simulate long-running task
Thread.sleep(5000);
return CompletableFuture.completedFuture("Processed: " + input);
}
}
Finally, update your controllers to leverage these asynchronous methods. The return type should be CompletableFuture
:
@RestController
public class AsyncController {
@Autowired
private AsyncService asyncService;
@GetMapping("/process")
public CompletableFuture process(@RequestParam String input) {
return asyncService.asyncMethod(input);
}
}
exceptionally()
in CompletableFuture
to handle exceptions gracefully.CompletableFuture.supplyAsync(() -> {
// Long-running task
})
.orTimeout(30, TimeUnit.SECONDS)
.exceptionally(ex -> {
// Handle timeout or exception
return "Task timed out";
});
By integrating asynchronous processing into your Spring HTTP server, you can efficiently manage resources, scale effortlessly, and enhance the overall response times, ensuring a smooth and responsive user experience.
Continue exploring advanced configurations and real-world implementations to make the most of Spring’s powerful asynchronous features.
## Load Testing with LoadForge
Load testing is crucial for understanding how your Spring HTTP server behaves under different levels of traffic. With LoadForge, you can simulate real-world traffic, identify performance bottlenecks, and derive actionable insights to optimize your server. This section will guide you through the process of performing load tests on your Spring HTTP server using LoadForge.
### Setting Up LoadForge
First, you need to have a LoadForge account. Sign up if you haven't already.
1. **Access LoadForge**: Log in to your LoadForge account at [LoadForge Dashboard](https://app.loadforge.com).
2. **Create a New Project**: Click on 'Create New Project' and provide a name and description for your load testing project.
### Configuring Your Load Test
1. **Define your HTTP Endpoints**: Specify the HTTP endpoints of your Spring HTTP server that you want to test.
- **URL**: The full URL of the endpoint.
- **Method**: HTTP method (GET, POST, PUT, DELETE).
- **Headers** and **Parameters**: Any necessary headers and query parameters.
2. **Set Up Test Scenarios**: Configure scenarios that mimic realistic user behavior.
- **Concurrent Users**: Define the number of virtual users that will simulate real-world traffic.
- **Ramp-Up Period**: Set the time over which the number of concurrent users will increase to the maximum value.
- **Test Duration**: Specify how long the test will run.
3. **Advanced Configuration**: Use advanced settings for specific needs.
- **Think Time**: Add simulated user 'think time' to mimic the delay between actions.
- **Failure Criteria**: Define conditions that determine when the test should fail (e.g., response time exceeds a certain threshold).
### Running the Load Test
Once your test configuration is ready:
1. **Start the Test**: Click the 'Run Test' button. LoadForge will start sending traffic to your Spring HTTP server based on the parameters you defined.
2. **Monitor the Test in Real-Time**: Use the LoadForge dashboard to monitor real-time metrics like response times, throughput, error rates, and more.
### Analyzing Test Results
After the test completes, LoadForge provides a comprehensive report with detailed insights.
1. **Response Times**: Check average, median, and percentile response times to understand the latency.
2. **Throughput**: Evaluate requests per second to gauge how well the server handles the load.
3. **Error Rates**: Identify any HTTP errors that occurred during the test to spot potential issues.
4. **Resource Utilization**: Analyze CPU and memory consumption if available through integration with application performance monitoring (APM) tools.
### Identifying Bottlenecks
Use the test results to pinpoint performance bottlenecks:
1. **High Latency**: If response times are high, investigate if thread pool sizes, connection pool settings, or garbage collection pauses are the culprit.
2. **Errors**: HTTP errors could indicate issues like database connection saturation or memory leaks.
3. **CPU/Memory Spikes**: Sudden increases could point to inefficient request processing or resource handling.
### Actionable Insights
Based on the test analysis, implement the following to optimize performance:
1. **Adjust Thread Pools**: Tweak the core and max pool sizes, and queue capacity.
2. **Optimize Database Connections**: Reconfigure connection pools like HikariCP settings.
3. **Improve Caching**: Introduce caching mechanisms to reduce the load on your server.
### Example: LoadForge Configuration
Below is an example configuration setup for a POST request load test in LoadForge:
<pre><code>
{
"url": "https://your-spring-server.com/api/data",
"method": "POST",
"headers": {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_TOKEN"
},
"body": "{\"key1\": \"value1\", \"key2\": \"value2\"}",
"scenarios": {
"concurrentUsers": 100,
"rampUpPeriod": 300,
"testDuration": 900
}
}
</code></pre>
### Conclusion
Load testing with LoadForge provides invaluable insights into your Spring HTTP server's performance under stress. By simulating real-world usage patterns, identifying bottlenecks, and implementing optimization strategies, you can ensure your server performs reliably and efficiently under varying loads.
## Utilizing HTTP/2
HTTP/2 is the newer version of the HTTP protocol, designed to improve web performance by addressing some of the inefficiencies present in HTTP/1.1. It introduces features such as multiplexing, header compression, and server push, which can significantly enhance the performance of your Spring HTTP server. In this section, we will explore the benefits of HTTP/2 and how to enable and configure it on a Spring server.
### Benefits of HTTP/2
1. **Multiplexing**: Allows multiple requests and responses to be sent over a single TCP connection, reducing latency and improving loading times.
2. **Header Compression**: Compresses HTTP headers to reduce overhead and improve response times, especially beneficial for applications with verbose headers.
3. **Server Push**: Enables the server to send resources to the client preemptively, reducing the need for multiple round-trip requests.
### Enabling HTTP/2 in Spring Boot
Enabling HTTP/2 in a Spring Boot application is straightforward. Below are the steps to configure your Spring Boot application to use HTTP/2.
#### 1. Prerequisites
Ensure you have the following prerequisites before enabling HTTP/2:
- Java 9 or higher
- Spring Boot 2.0 or higher
- HTTP/2-capable server (Jetty, Tomcat, or Undertow)
#### 2. Configure Application Properties
To enable HTTP/2 in your Spring Boot application, you need to set the appropriate properties in your `application.properties` or `application.yml` file. Here's an example for `application.properties`:
<pre><code>
# Enable HTTP/2 for embedded Tomcat
server.http2.enabled=true
</code></pre>
If you prefer `application.yml`, the equivalent configuration looks like this:
<pre><code>
server:
http2:
enabled: true
</code></pre>
#### 3. Configuring Embedded Servlet Containers
HTTP/2 support is provided natively by popular embedded servlet containers like Tomcat, Jetty, and Undertow. Ensure you have the correct dependencies included in your `pom.xml` if you are using Maven:
For Tomcat:
<pre><code>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Optional if not already included -->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-core</artifactId>
</dependency>
</code></pre>
For Jetty:
<pre><code>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jetty</artifactId>
</dependency>
</code></pre>
For Undertow:
<pre><code>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-undertow</artifactId>
</dependency>
</code></pre>
#### 4. Configuring SSL/TLS
HTTP/2 requires an SSL/TLS configuration. Here's an example of setting up SSL for embedded Tomcat:
<pre><code>
# application.properties
server.port=8443
server.ssl.key-store=classpath:keystore.jks
server.ssl.key-store-password=changeit
server.ssl.key-password=changeit
server.ssl.key-alias=springboot
</code></pre>
Add the necessary SSL configuration settings based on your chosen container. If you don't have a keystore, you can create one using the `keytool` command:
<pre><code>
keytool -genkey -alias springboot -storetype PKCS12 -keyalg RSA -keysize 2048 -validity 3650 -keystore keystore.p12
</code></pre>
Make sure to place the keystore file in the `src/main/resources` directory or adjust the path accordingly.
### Summary
Enabling HTTP/2 in a Spring Boot application introduces several performance improvements, including multiplexing, header compression, and server push. By modifying your `application.properties` or `application.yml` and ensuring your servlet container and SSL are appropriately configured, you can leverage the benefits of HTTP/2 to optimize your Spring HTTP server's performance.
This configuration can lead to faster page loads, reduced latency, and an overall better user experience, making it a valuable addition to your performance optimization toolkit.
In this section, we'll explore a collection of settings and tweaks specific to Spring Boot that can lead to significant performance gains. We'll cover various aspects including JVM parameters, garbage collection settings, and strategies for optimizing Spring Boot startup time.
Tuning the JVM is critical for maximizing the performance of your Spring Boot application. Here are some recommended JVM options for optimizing performance:
-Xms512m -Xmx1024m # Set initial and maximum heap size
-XX:+UseG1GC # Use the G1 garbage collector
-XX:MetaspaceSize=128m # Set initial metaspace size
-XX:MaxMetaspaceSize=256m # Set maximum metaspace size
-XX:+HeapDumpOnOutOfMemoryError # Enable heap dump in case of OOM error
-XX:HeapDumpPath=/path/to/dumps # Directory to save heap dumps
These parameters establish a balanced memory use strategy and enable efficient garbage collection, crucial for maintaining performance under load.
Choosing the right garbage collection (GC) strategy can dramatically impact the performance of your Spring Boot application. The G1 Garbage Collector is a popular choice for modern applications due to its predictable behavior and efficiency. Here's how to configure it:
-XX:+UseG1GC
-XX:MaxGCPauseMillis=200 # Target maximum GC pause time
-XX:G1HeapRegionSize=16M # Fine-tune the size of G1 regions
-verbose:gc # Enable verbose GC logging
-XX:+PrintGCDetails # Print detailed GC logging
-XX:+PrintGCDateStamps # Add timestamps to GC logging
-XX:+PrintGCTimeStamps # Add GC timing information
-XX:+PrintGCApplicationStoppedTime # Print application stop time
-XX:+PrintGCApplicationConcurrentTime # Print concurrent application time
These flags help to reduce GC overhead and pause times, thereby enhancing the application's responsiveness.
A fast startup time is crucial for instances where you may need to rapidly scale your application up or down. Here are some strategies to optimize Spring Boot startup:
Ensure that only necessary beans are loaded at startup by configuring your components wisely:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication application = new SpringApplication(Application.class);
// Disable command line property rewriting
application.setAddCommandLineProperties(false);
application.run(args);
}
}
@Lazy
InitializationUse the @Lazy
annotation to delay the initialization of beans until they are needed:
@Component
@Lazy
public class ExpensiveBean {
// Bean initialization logic
}
Load configurations specific to the active profile to minimize unnecessary context loading:
spring:
profiles:
active: prod
Spring Boot 2.x supports parallel bean initialization which can speed up the startup process:
spring:
main:
allow-bean-definition-overriding: true
lazy-initialization: true
web-environment: true
concurrency:
initial-contexts: 4
max-initialization-threads: 4
Combining the optimizations, your Spring Boot application configuration may look something like this:
spring:
main:
banner-mode: "off"
lazy-initialization: true
server:
jetty:
threads:
max: 200
min: 10
idle-timeout: 120
compression:
enabled: true
mime-types: application/json, application/xml, text/html, text/xml, text/plain
min-response-size: 1024
management:
endpoint:
health:
show-details: "always"
endpoints:
web:
exposure:
include: "*"
logging:
level:
org:
springframework:
web: INFO
com:
myapp: DEBUG
Configuring Spring Boot for high performance involves tweaking the JVM parameters, selecting efficient garbage collection settings, and optimizing startup time through smart application configuration. Implementing these strategies can significantly enhance the performance and responsiveness of your Spring Boot application, ensuring that it scales efficiently and handles load effectively.
Maintaining the high performance of your Spring HTTP server isn't a one-time task; it requires ongoing monitoring and continuous improvement. In this section, we'll explore best practices for performance monitoring, tools you can leverage, and how to implement a cycle of continuous performance assessment and tuning.
To ensure your Spring HTTP server is performing optimally, keep an eye on the following key performance metrics:
Several tools can assist in monitoring your Spring HTTP server's performance:
Spring Boot Actuator: Provides a suite of production-ready features to help you monitor and manage your application. It includes endpoints for health checks, metrics, and application information.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Prometheus and Grafana: A powerful monitoring and alerting toolkit paired with a visualization solution.
management.metrics.export.prometheus.enabled=true
management.endpoints.web.exposure.include=prometheus
JMX (Java Management Extensions): Useful for monitoring JVM-related metrics such as garbage collection, memory pools, and thread usage.
LoadForge: For conducting regular load testing to simulate real-world traffic and uncover performance bottlenecks.
Here’s an example of setting up Prometheus and Grafana for monitoring:
Add Spring Boot Actuator and Prometheus dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
Configure Prometheus:
management.endpoints.web.exposure.include: "*"
management.metrics.export.prometheus.enabled: true
Set up Prometheus server configuration:
scrape_configs:
- job_name: 'spring'
scrape_interval: 5s
static_configs:
- targets: ['localhost:8080']
Visualize in Grafana: Import Prometheus as a data source in Grafana and use it to create dashboards for visualizing metrics.
With tools like LoadForge, you can perform regular load testing to simulate different traffic patterns and user behaviors. LoadForge’s features enable you to:
Set up automated alerts that notify you of performance degradation or resource threshold breaches. Prometheus and Grafana support alerting rules, which can be configured as follows:
alerting:
alertmanagers:
- static_configs:
- targets: ['localhost:9093']
rule_files:
- "alert.rules"
alert: InstanceDown
expr: up == 0
for: 5m
labels:
severity: page
annotations:
summary: "Instance {{ $labels.instance }} down"
By integrating robust monitoring practices and committing to continuous improvement, you can maintain optimal performance for your Spring HTTP server and ensure a seamless experience for users.
In this guide, we've undertaken a comprehensive journey through the various aspects of optimizing Spring HTTP server performance. From understanding the basics of Spring HTTP server architecture to delving into specific performance metrics, we've equipped you with the knowledge needed to diagnose, analyze, and enhance the efficiency of your Spring-based applications.
Understanding Spring HTTP Server Basics: We began with a foundational overview of the Spring HTTP server, its core components, and the architecture. This set the stage for understanding why performance optimization is crucial.
Evaluating Performance Metrics: We discussed crucial performance metrics such as response time, throughput, CPU usage, and memory consumption, providing the essential tools to measure and understand your server’s performance.
Optimizing Thread Pool Settings: Detailed advice was provided on configuring thread pools, including considerations for max pool size, queue capacity, and thread keep-alive time, to ensure optimal server performance.
Efficient Use of Caching: We explored various caching mechanisms like EhCache and Redis, and how to effectively use HTTP caching headers to reduce load and optimize response times.
Database Connection Pool Tuning: We covered best practices for optimizing database connections through efficient utilization of connection pools such as HikariCP.
Handling Large Payloads: Strategies were shared on managing large payloads, including techniques like chunked transfer encoding and optimized JSON serialization, to handle heavy loads smoothly.
Leveraging Asynchronous Requests: Insight was provided into the use of asynchronous request processing to enable non-blocking I/O and improve the server’s scalability.
Load Testing with LoadForge: We introduced LoadForge as a vital tool for performing load tests on Spring HTTP servers to simulate real-world traffic scenarios and identify performance bottlenecks.
Utilizing HTTP/2: The benefits of HTTP/2, such as multiplexing and header compression, were explored, along with steps on enabling and configuring it on a Spring server.
Configuring Spring Boot for High Performance: We concluded with a collection of settings and tweaks in Spring Boot, including JVM parameters and garbage collection settings, to achieve significant performance gains.
Monitoring and Continuous Improvement: Highlighting the importance of continuous monitoring, we discussed tools and best practices for ongoing performance assessment and tuning.
For those eager to dive deeper into Spring HTTP server performance optimization, here are some recommended resources:
Spring Documentation:
Books:
Online Courses:
Articles and Blog Posts:
Tools and Libraries:
By following these best practices and continually educating yourself with these resources, you will ensure that your Spring HTTP server delivers peak performance, scales effectively, and provides a robust user experience.
Thank you for reading, and happy optimizing!