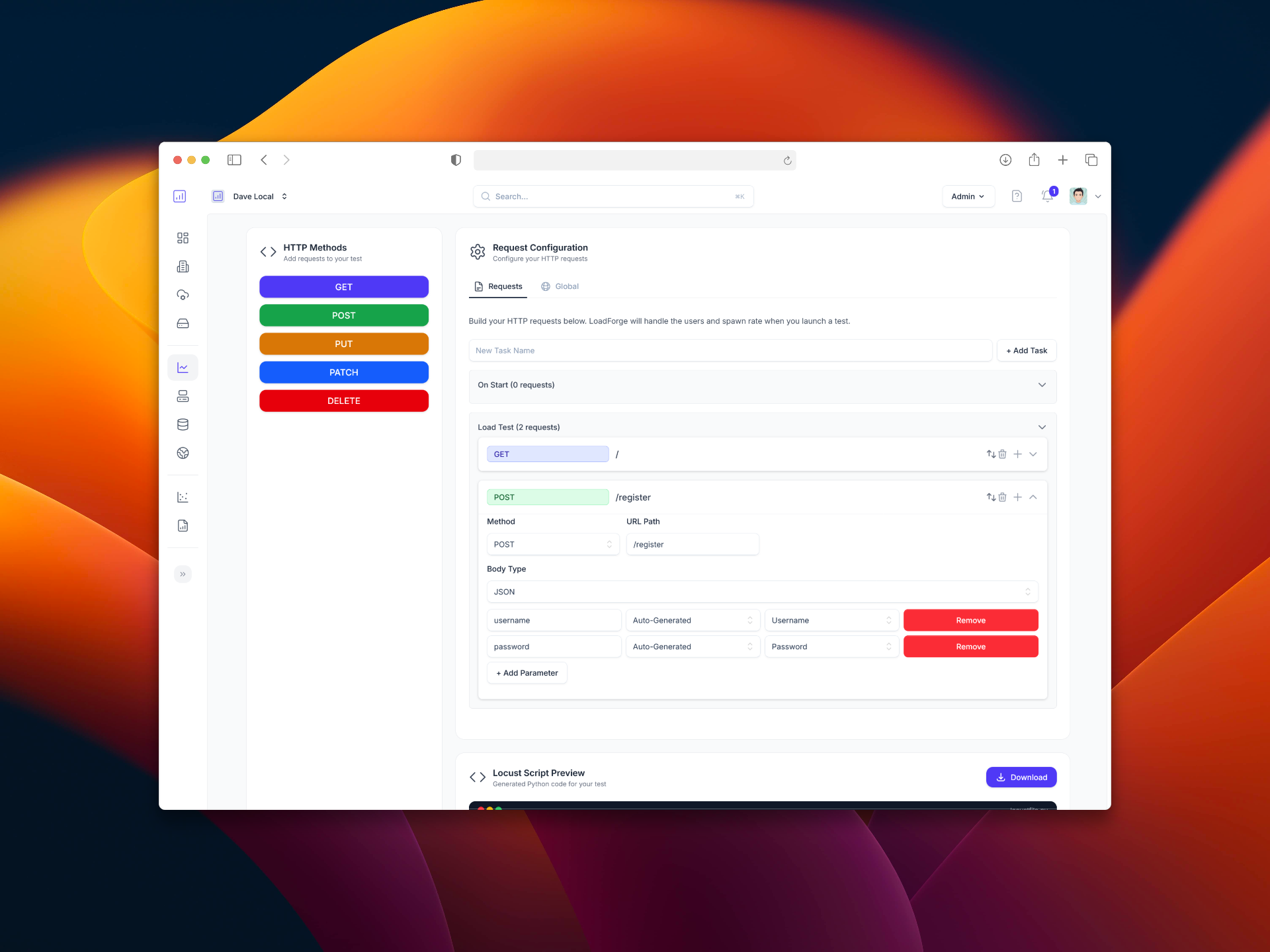
Jira Integration & Graphical Test Builder
We are proud to announce the release of two new features, available immediately. 🚀 Jira Integration: Automate Issue Tracking for...
In the digital world, ensuring the reliability and security of your web applications is paramount. One critical aspect of this is implementing rate limiting and throttling to manage the flow of requests to your application. But what exactly are these...
In the digital world, ensuring the reliability and security of your web applications is paramount. One critical aspect of this is implementing rate limiting and throttling to manage the flow of requests to your application. But what exactly are these terms, and why are they so essential?
Rate limiting is a technique used to control the amount of incoming and outgoing traffic to or from a network. It restricts the number of requests a client can make to your server within a given timeframe. This is generally done to prevent abuse, ensure fair usage, and avoid overloading the server.
Throttling, on the other hand, often refers to temporarily slowing down the rate of request processing. It's a broader term usually aimed at controlling data throughput over a network.
Rate limiting and throttling are vital for several reasons:
In this step-by-step guide, we aim to provide you with a comprehensive understanding and practical implementation of rate limiting in your FastAPI application. Here’s what you’ll learn:
slowapi
or fastapi-limiter
to help implement rate limiting.Implementing effective rate limiting and throttling can protect your FastAPI application and ensure a smoother user experience. Let’s dive in and start building a resilient system.
Before diving into the implementation of rate limiting in FastAPI, there are a few prerequisites you'll need to meet. This section lists the essential requirements you'll need to follow along with this guide successfully.
Python Environment: Ensure you have Python 3.7 or higher installed on your machine. You can download the latest version of Python from the official website. Verify your installation by running:
python --version
FastAPI: Install FastAPI, which is the core framework we will be using. You can install it using pip:
pip install fastapi
Uvicorn: Since FastAPI is an ASGI framework, a compatible ASGI server like Uvicorn is needed to run the application. Install it using pip:
pip install uvicorn
Rate Limiting Packages: For implementing rate limiting, we will be using the slowapi
package. Install it using pip:
pip install slowapi
Alternatively, you can use fastapi-limiter
, which requires additional dependencies like Redis. For the purposes of this guide, we'll focus on slowapi
.
Ensure your project directory is well-organized. Create a new directory for your FastAPI application (if you haven't already) and navigate into it:
mkdir fastapi-rate-limit
cd fastapi-rate-limit
Within this directory, create two essential files:
main.py
: This will contain the main application code.requirements.txt
: This file will list all the required dependencies.Populate your requirements.txt
file with the following lines:
fastapi
uvicorn
slowapi
Run the following command to install all dependencies listed in requirements.txt
:
pip install -r requirements.txt
With your environment set up and the necessary packages installed, you're now ready to progress through the guide. In the next section, we'll set up a basic FastAPI application to serve as the foundation for our rate limiting implementation.
Remember to keep this guide open as you proceed, and feel free to refer back to this prerequisites section if you encounter any issues with setup and installation.
In this section, we'll guide you through setting up a basic FastAPI application. This will provide the groundwork for implementing rate limiting and will help you understand the project structure and minimal code needed to get started.
Before diving in, ensure you have the following prerequisites:
If you haven’t installed FastAPI and Uvicorn yet, you can do so using the following commands:
pip install fastapi uvicorn
Here's a basic directory structure for your FastAPI application:
fastapi-rate-limit/
├── app/
│ ├── main.py
│ └── __init__.py
├── requirements.txt
├── README.md
└── .gitignore
Let's start by writing the minimal code for our FastAPI application.
main.py
inside the app
directory.from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"message": "Welcome to FastAPI!"}
@app.get("/items/{item_id}")
def read_item(item_id: int, q: str = None):
return {"item_id": item_id, "q": q}
requirements.txt
in the project root. Add the necessary dependencies.fastapi
uvicorn
Now, let's run the application to ensure everything is set up correctly. Navigate to the project root and use Uvicorn to run the app:
uvicorn app.main:app --reload
You should see output indicating that Uvicorn is running the server. By default, it runs on http://127.0.0.1:8000
. Open a browser and navigate to that URL; you should see a JSON response with the message "Welcome to FastAPI!"
.
To verify that other endpoints work, you can navigate to:
http://127.0.0.1:8000/items/1?q=test
You should see a response similar to:
{
"item_id": 1,
"q": "test"
}
Congratulations! You have successfully set up a basic FastAPI application. In the upcoming sections, we will build upon this foundation to implement rate limiting and throttling to protect your application from abuse and ensure fair usage.
In this section, we will delve deeper into the concepts of rate limiting and throttling. We will explore why these mechanisms are essential, how they work, and the various strategies and algorithms for implementing them. This will provide you with a solid foundation to apply effective rate limiting to your FastAPI applications.
Rate limiting and throttling are techniques used to control the amount of incoming and outgoing traffic to and from a network or application. They ensure that no single user can overwhelm the system by making too many requests in too little time. Here’s a brief explanation of each:
Implementing rate limiting and throttling helps in:
Understanding different strategies and algorithms used in rate limiting helps you choose the right one for your FastAPI application. Below are popular strategies you might consider:
The Token Bucket algorithm is one of the most commonly used rate limiting strategies. It works by adding tokens to a bucket at a fixed rate. Each incoming request consumes a token. When the bucket is empty, requests are either delayed or dropped until more tokens are added.
Example Pseudocode:
class TokenBucket:
def __init__(self, capacity, refill_rate):
self.capacity = capacity
self.tokens = capacity
self.refill_rate = refill_rate
self.last_refill_timestamp = current_time()
def allow_request(self):
self.refill_tokens()
if self.tokens > 0:
self.tokens -= 1
return True
return False
def refill_tokens(self):
now = current_time()
elapsed = now - self.last_refill_timestamp
tokens_to_add = elapsed * self.refill_rate
self.tokens = min(self.capacity, self.tokens + tokens_to_add)
self.last_refill_timestamp = now
The Leaky Bucket algorithm works similarly to water leaking from a bucket at a constant rate. Incoming requests are added to the bucket, and they are processed at a fixed rate. If the bucket overflows, the requests are dropped.
Example Pseudocode:
class LeakyBucket:
def __init__(self, capacity, leak_rate):
self.capacity = capacity
self.leak_rate = leak_rate
self.queue = []
self.last_leak_timestamp = current_time()
def allow_request(self, request):
self.leak()
if len(self.queue) < self.capacity:
self.queue.append(request)
return True
return False
def leak(self):
now = current_time()
elapsed = now - self.last_leak_timestamp
leaks = elapsed * self.leak_rate
for _ in range(int(leaks)):
if self.queue:
self.queue.pop(0)
self.last_leak_timestamp = now
The Fixed Window Counter strategy divides time into fixed-size windows and counts the number of requests in the current window. If the count exceeds the limit for the current window, additional requests are denied until the next window.
Example Pseudocode:
class FixedWindow:
def __init__(self, limit, window_size):
self.limit = limit
self.window_size = window_size
self.counter = 0
self.window_start = current_time()
def allow_request(self):
if current_time() >= self.window_start + self.window_size:
self.window_start = current_time()
self.counter = 1
return True
elif self.counter < self.limit:
self.counter += 1
return True
return False
By understanding the different strategies and algorithms for rate limiting and throttling, you can better protect your FastAPI application from abuse and ensure fair usage among all users. The next sections will guide you through installing the necessary dependencies and implementing these strategies in your FastAPI application.
In order to implement rate limiting in your FastAPI application, you will need to install a few essential Python packages. For this guide, we will focus on two popular libraries: slowapi
and fastapi-limiter
. These packages simplify the process of integrating rate limiting mechanisms into your FastAPI app.
slowapi
slowapi
is a useful library that provides a straightforward way to enforce rate limits. Follow these steps to install slowapi
and its dependencies:
Install slowapi
First, you need to install the slowapi
package. Ensure you are in your project's virtual environment, if applicable, and run the following command:
pip install slowapi
Additional Dependencies
slowapi
requires limits
. You can install it using:
pip install limits
Optionally, you may also want to install a Redis client if you plan to use Redis as the backend for rate limiting storage:
pip install aioredis
fastapi-limiter
fastapi-limiter
is another robust library for implementing rate limits in FastAPI. Here are the steps to install fastapi-limiter
:
Install fastapi-limiter
Again, ensure you are within your project's virtual environment and run the following command:
pip install fastapi-limiter
Redis Server
fastapi-limiter
leverages Redis for storing rate limits. You need to have Redis installed and running. To install Redis on your local machine, you can follow the instructions from the Redis official website.
Alternatively, you can use a hosted Redis service such as Amazon ElastiCache, Azure Cache for Redis, or Redis Labs.
To verify that the required dependencies have been installed correctly, you can create a small Python script and import the installed packages:
try:
from slowapi import Limiter
from slowapi.util import get_remote_address
from fastapi_limiter import FastAPILimiter
import redis
print("All packages are installed successfully!")
except ImportError as e:
print(f"Error: {e}")
Run the script:
python verify_dependencies.py
If you see the message "All packages are installed successfully!" then you have correctly installed the necessary dependencies for implementing rate limiting in your FastAPI application.
With the dependencies in place, you are now ready to integrate rate limiting into your FastAPI app. In the next sections, we will guide you through the actual implementation using these packages to ensure fair usage and protect your application from abuse.
In this section, we will walk through the detailed steps of implementing rate limiting in a FastAPI application using the SlowAPI package. SlowAPI is a user-friendly library designed specifically for handling rate limiting in FastAPI applications. Follow along carefully to ensure you configure the middleware correctly and set appropriate limits for your endpoints.
Before we begin, install the SlowAPI package. You can do this using pip:
pip install slowapi
First, import the necessary components from SlowAPI and FastAPI. Add the SlowAPI middleware to your FastAPI application.
from fastapi import FastAPI, Request
from slowapi import Limiter, _rate_limit_exceeded_handler
from slowapi.util import get_remote_address
# Create a Limiter instance
limiter = Limiter(key_func=get_remote_address)
# Initialize the FastAPI app
app = FastAPI()
# Add the Limiter middleware and exception handler to the app
app.state.limiter = limiter
app.add_exception_handler(429, _rate_limit_exceeded_handler)
Now, let’s configure rate limits for your endpoints. You can specify the rate limits using decorators provided by SlowAPI.
from fastapi import Depends
from slowapi.decorators import limit
@app.get("/items")
@limit("5/minute")
async def read_items():
return {"message": "This endpoint is rate limited to 5 requests per minute"}
@app.post("/submit")
@limit("2/minute")
async def submit_item():
return {"message": "This endpoint is rate limited to 2 requests per minute"}
You can also define more granular rate limits based on different strategies. For instance, applying different limits based on user roles or IP addresses.
@app.get("/user-data")
@limit("10/minute", key_func=lambda request: request.state.user_role)
async def read_user_data(request: Request):
return {"message": f"This endpoint is rate limited to 10 requests per minute based on user roles"}
If you want to apply a global rate limit to all endpoints, you can set it up in the middleware configuration.
limiter = Limiter(
key_func=get_remote_address,
default_limits=["200 per day", "50 per hour"]
)
app = FastAPI()
app.state.limiter = limiter
app.add_exception_handler(429, _rate_limit_exceeded_handler)
You have now successfully implemented rate limiting in your FastAPI application using SlowAPI. By following these steps, you can ensure that your endpoints are protected against abuse and maintain fair usage among your users.
Continue to the next section to learn how to test your rate limits using LoadForge.
Once you've implemented rate limiting in your FastAPI application, it's crucial to test whether these constraints are functioning as intended. Proper testing ensures that your rate limits prevent abuse without hindering legitimate traffic. In this section, we'll explore different methods and tools to test your rate limits, specifically focusing on using LoadForge for load testing. We'll also discuss how to interpret the results and make any necessary adjustments.
There are several methods you can use to test your rate limits:
curl
or Postman.LoadForge is a powerful load testing tool designed to simulate real-world traffic and help you understand how your FastAPI application performs under stress. Here’s how you can use LoadForge to test your rate limits:
Sign Up and Set Up a Test on LoadForge:
Configure the Test Script:
import requests
url = "http://your-fastapi-app.com/endpoint"
def load_test():
for _ in range(100):
response = requests.get(url)
print(response.status_code)
Run the Load Test:
Analyze the Results:
429 Too Many Requests
responses, which indicate that your rate limit is being triggered correctly.After running your load test, you’ll receive a variety of metrics. Here’s how to interpret them:
429 Too Many Requests
responses will indicate how often users are hitting the rate limit. If this number is very high, you might need to adjust your rate limits.429
to ensure that your rate limiting doesn’t introduce new issues.Based on the test results, you might need to tweak your rate limiting configurations. Here are a few tips:
Adjust Rate Limits:
Improve Performance:
Monitor and Iterate:
Testing your rate limits is an essential step to ensure they perform as designed under real-world traffic. Using LoadForge for load testing provides a robust and controlled way to simulate various scenarios and gather actionable insights. By interpreting the results and making informed adjustments, you can protect your FastAPI application from abuse while maintaining a smooth user experience.
When implementing rate limits in your FastAPI application, it is crucial to handle cases where clients exceed their allowed requests gracefully. Appropriate handling provides a good user experience, conforms to SEO best practices, and ensures clients are aware of their rate limits and how to manage them. In this section, we'll explore how to configure responses for rate-limited requests, best practices for user experience, and considerations for SEO.
When a client hits the rate limit, we should return a clear and informative HTTP response. Typically, this involves using the HTTP 429 Too Many Requests
status code. Along with the status code, the response body should provide useful information, such as the time until the rate limit resets.
Here's an example of how to handle rate limit exceeded responses using the SlowAPI
package:
from slowapi.errors import RateLimitExceeded
from slowapi.extension import Limiter
from fastapi import FastAPI, Request
from slowapi.util import get_remote_address
from starlette.responses import JSONResponse
app = FastAPI()
limiter = Limiter(key_func=get_remote_address)
app.state.limiter = limiter
@app.exception_handler(RateLimitExceeded)
async def rate_limit_exceeded_handler(request: Request, exc: RateLimitExceeded):
retry_after = int(exc.description.split(' ')[-1])
response_body = {
"detail": "Rate limit exceeded. Please try again later.",
"retry_after_seconds": retry_after
}
return JSONResponse(status_code=429, content=response_body, headers={"Retry-After": str(retry_after)})
@app.get("/limited_endpoint")
@limiter.limit("5/minute")
async def limited_endpoint():
return {"message": "This is a rate-limited endpoint"}
In the code snippet above, the rate_limit_exceeded_handler
function is set up to handle RateLimitExceeded
exceptions. The response includes a Retry-After
header, which indicates to the client how many seconds to wait before making a new request.
Informative Responses: Always provide detailed and helpful information in the response body. Let the user know what happened and how long they need to wait before they can make another request.
HTTP Headers: Use appropriate HTTP headers to communicate rate limiting information. The Retry-After
header is particularly useful for informing clients about how long to wait before retrying.
UI Feedback: If your FastAPI application includes a frontend, ensure that the UI provides clear feedback when users exceed rate limits. Display a user-friendly message and possibly a countdown timer indicating when they can try again.
Documentation: Ensure your API documentation includes details about rate limits. Clearly specify the limits, the responses users can expect when limits are exceeded, and any strategies for clients to handle these responses.
From an SEO perspective, it’s important to handle rate limit responses in a way that search engine crawlers can understand and respect. Here are a few tips:
Status Codes: Use the 429 Too Many Requests
status code to indicate rate limiting. This status code is understood by search engines and helps them identify why certain requests are being denied.
Retry-After Header: Always include the Retry-After
header in rate-limited responses. This header informs search engine crawlers about when they should attempt to crawl the page again, helping to ensure that your site is crawled efficiently and not penalized.
Avoid Blocking Important Pages: Be cautious about rate-limiting requests to critical pages that are essential for SEO. Ensure that search engine bots have optimal access to these pages or consider implementing higher rate limits for them.
Here’s an example JSON response for a rate limit exceeded scenario:
{
"detail": "Rate limit exceeded. Please try again later.",
"retry_after_seconds": 60
}
In this example, the "retry_after_seconds"
field indicates the number of seconds clients must wait before making another request.
By handling rate limit exceeded responses effectively, you can ensure that your FastAPI application maintains a positive user experience, adheres to SEO best practices, and communicates rate limit policies clearly to clients.
Rate limiting is a crucial aspect of protecting and optimizing your FastAPI application, but basic rate limiting might not be sufficient for all use cases. In this section, we'll explore more advanced rate limiting techniques, including distributed rate limiting, user-based limits, and IP-based limits. These techniques will give you greater flexibility and control in managing how resources are accessed in your application.
When your application is deployed across multiple servers or instances, implementing a distributed rate limiting mechanism can help maintain consistent limits across the infrastructure. This approach requires storing the rate limiting state in a centralized data store such as Redis.
Example using Redis and SlowAPI:
from slowapi import Limiter
from slowapi.util import get_remote_address
from slowapi.middleware import SlowAPIMiddleware
from fastapi import FastAPI, Request
from redis import Redis
import uvicorn
# Initialize Redis and SlowAPI Limiter
redis = Redis(host='localhost', port=6379)
limiter = Limiter(key_func=get_remote_address, storage_uri="redis://localhost:6379")
app = FastAPI()
app.state.limiter = limiter
app.add_middleware(SlowAPIMiddleware)
@app.get("/")
@limiter.limit("5/minute")
async def root(request: Request):
return {"message": "Hello, World!"}
if __name__ == "__main__":
uvicorn.run(app, host="0.0.0.0", port=8000)
In this example, the rate limier state is shared via Redis, ensuring consistent rate limiting across multiple instances of the FastAPI application.
User-based rate limiting is particularly useful for managing API usage on a per-user basis. This technique ensures that each user has a dedicated quota, which can help prevent a single user from consuming all available resources.
Example using SlowAPI with user-based limits:
from slowapi import Limiter
from slowapi.util import get_remote_address
from slowapi.middleware import SlowAPIMiddleware
from fastapi import FastAPI, Request, Depends
from pydantic import BaseModel
limiter = Limiter(key_func=lambda: "global")
app = FastAPI()
app.state.limiter = limiter
app.add_middleware(SlowAPIMiddleware)
class User(BaseModel):
username: str
def get_current_user() -> User:
# Dummy function returning a user, replace with auth logic
return User(username="test_user")
@app.get("/")
@limiter.limit("10/minute", key_func=lambda request: get_current_user().username)
async def root(request: Request, user: User = Depends(get_current_user)):
return {"message": f"Hello, {user.username}!"}
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
This example attaches the rate limit to each unique username, ensuring that each user can make up to 10 requests per minute.
IP-based rate limiting restricts access based on the client's IP address, which is useful for preventing abuse from a particular IP address or range of addresses. This method is often used for public APIs to mitigate DDoS attacks and other malicious activities.
Example using SlowAPI with IP-based limits:
from slowapi import Limiter
from slowapi.util import get_remote_address
from slowapi.middleware import SlowAPIMiddleware
from fastapi import FastAPI, Request
import uvicorn
limiter = Limiter(key_func=get_remote_address)
app = FastAPI()
app.state.limiter = limiter
app.add_middleware(SlowAPIMiddleware)
@app.get("/")
@limiter.limit("15/minute")
async def root(request: Request):
return {"message": "Hello from IP-limited endpoint!"}
if __name__ == "__main__":
uvicorn.run(app, host="0.0.0.0", port=8000)
In this example, the rate limiting is applied based on the client's IP address, allowing a maximum of 15 requests per minute.
Combining these techniques can offer even finer control over your API usage. For example, you might want to use user-based limits for authenticated endpoints and IP-based limits for public endpoints.
@app.get("/public")
@limiter.limit("20/minute")
async def public_endpoint(request: Request):
return {"message": "Public endpoint with IP-based limiting"}
@app.get("/private")
@limiter.limit("5/minute", key_func=lambda request: get_current_user().username)
async def private_endpoint(request: Request, user: User = Depends(get_current_user)):
return {"message": f"Private endpoint for {user.username} with user-based limiting"}
By understanding and implementing these advanced rate limiting techniques, you can ensure fair and efficient usage of your FastAPI application, safeguard its resources, and offer a better user experience.
When implementing rate limiting in your FastAPI application, it’s crucial to understand its impact on performance and to ensure that you're not trading off too much in terms of speed and resource efficiency. This section will discuss the potential performance implications of rate limiting and offer tips for optimizing server resources to maintain smooth operation under load.
Rate limiting acts as a gatekeeper, controlling the flow of requests to your application. While beneficial for preventing abuse and ensuring fair usage, it adds an additional layer of checks that can impact performance. Here are some of the key areas affected:
To ensure your rate limiting implementation doesn't degrade your application's performance significantly, consider the following optimization techniques:
Efficient Algorithms:
Redis for Storage:
from redis import Redis
from slowapi import Limiter
from slowapi.util import get_remote_address
from fastapi import FastAPI, Depends
app = FastAPI()
redis = Redis(host='localhost', port=6379, db=0)
limiter = Limiter(key_func=get_remote_address, storage_uri="memory://")
@app.on_event("startup")
def startup():
limiter.set_storage_uri(app, "redis://localhost:6379")
@app.get("/home")
@limiter.limit("5/minute")
async def home():
return {"message": "Homepage"}
Asynchronous I/O:
@app.get("/async_endpoint")
@limiter.limit("10/minute")
async def async_endpoint():
# Simulate a long-running I/O operation
await asyncio.sleep(1)
return {"message": "Asynchronous endpoint"}
Cache Headers:
from fastapi.responses import JSONResponse
@app.get("/data")
@limiter.limit("10/minute")
async def get_data():
response = JSONResponse(content={"data": "sample data"})
response.headers["Cache-Control"] = "public, max-age=60"
return response
Optimize Middlewares:
To maintain smooth operation under load, consider the following:
Load Testing:
# Example LoadForge configuration for testing
loadforge test \
--url=https://api.example.com/endpoint \
--rate=1000rps \
--duration=60s
Monitoring and Alerts:
Graceful Degradation:
By understanding and mitigating the performance impacts of rate limiting, you can effectively protect your FastAPI application while ensuring a seamless experience for your users. Optimal algorithms, efficient storage solutions, and strategic load testing will help maintain high performance even under demanding conditions.
To effectively enforce rate limiting in your FastAPI application, it's crucial to implement robust monitoring and logging mechanisms. These will not only help you gain insights into how your rate limiting is performing but also assist in identifying and resolving potential issues. In this section, we will explore best practices, tools, and strategies for monitoring and logging rate limiting events.
Monitoring and logging rate limiting events are essential for:
Here are some best practices to follow:
First, let's add basic logging to our FastAPI application. Python's built-in logging
module will be used to print log messages.
import logging
from fastapi import FastAPI, Request
from slowapi import Limiter, _rate_limit_exceeded_handler
from slowapi.errors import RateLimitExceeded
# Initialize FastAPI and SlowAPI
app = FastAPI()
limiter = Limiter(key_func=lambda request: request.client.host)
app.state.limiter = limiter
app.add_exception_handler(RateLimitExceeded, _rate_limit_exceeded_handler)
# Configure Logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
@app.middleware("http")
async def log_requests(request: Request, call_next):
logger.info(f"Request: {request.method} {request.url}")
response = await call_next(request)
logger.info(f"Response: {response.status_code}")
return response
@app.get("/rate-limited-endpoint")
@limiter.limit("5/minute")
async def rate_limited_endpoint():
return {"message": "This is a rate-limited endpoint"}
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
Prometheus and Grafana can be powerful tools for real-time monitoring. You can export metrics from your FastAPI application using prometheus_client
.
Install Prometheus Client:
pip install prometheus_client
Integrate Prometheus with FastAPI:
from fastapi import FastAPI, Response
from prometheus_client import Counter, generate_latest, CONTENT_TYPE_LATEST
# Initialize Prometheus metrics
REQUEST_COUNT = Counter('request_count', 'Application Request Count')
RATE_LIMIT_EXCEEDED_COUNT = Counter('rate_limit_exceeded_count', 'Rate Limit Exceeded Count')
app = FastAPI()
@app.middleware("http")
async def prometheus_metrics(request: Request, call_next):
REQUEST_COUNT.inc()
response = await call_next(request)
return response
@app.add_exception_handler(RateLimitExceeded)
async def rate_limit_exceeded_handler(request: Request, exc: RateLimitExceeded):
RATE_LIMIT_EXCEEDED_COUNT.inc()
return Response("Rate limit exceeded", status_code=429)
@app.get("/metrics")
def metrics():
return Response(generate_latest(), media_type=CONTENT_TYPE_LATEST)
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
Besides Prometheus and Grafana, other tools like ELK, Splunk, and Datadog can be used for logging and monitoring. Choose one that best fits your infrastructure and requirements.
Effective monitoring and logging of rate limiting events are vital for maintaining the reliability and performance of your FastAPI application. By following the best practices and utilizing the right tools, you can gain deep insights into your rate limiting policies, optimize performance, and ensure fair usage across your user base.
Implementing rate limits in FastAPI can be a smooth process, but there are common pitfalls and issues that developers might encounter. This section provides a guide to those potential obstacles and how to resolve them effectively.
Problem: One of the most common issues is incorrect configuration of the rate-limiting middleware, which can lead to it not being applied to your FastAPI application at all.
Solution: Ensure that the middleware is correctly added to the FastAPI application. For example, if you're using SlowAPI
, the middleware should be included as follows:
from fastapi import FastAPI
from slowapi import Limiter, _rate_limit_exceeded_handler
from slowapi.middleware import SlowAPIMiddleware
app = FastAPI()
limiter = Limiter(key_func=get_ipaddr)
app.state.limiter = limiter
app.add_exception_handler(RateLimitExceeded, _rate_limit_exceeded_handler)
app.add_middleware(SlowAPIMiddleware)
Problem: Incorrect or inefficient key function for identifying unique clients can lead to misapplied rate limits, either too lenient or too strict.
Solution: Ensure the key_func
provided to the rate limiter middleware accurately identifies unique clients. A common approach is based on the client's IP address:
from slowapi.util import get_remote_address
limiter = Limiter(key_func=get_remote_address)
Problem: Applying rate limits too broadly or narrowly—by either setting global limits that affect all endpoints uniformly or failing to set endpoint-specific limits where necessary.
Solution: Carefully define both global and endpoint-specific rate limits according to your application's requirements:
@app.get("/resource")
@limiter.limit("5/minute")
async def limited_resource():
return {"message": "This resource is rate limited to 5 requests per minute"}
Problem: Rate limit exceptions not being properly handled, leading to uninformative errors being returned to users.
Solution: Customize the exception handler to provide user-friendly error messages and proper HTTP status codes. This can be done by modifying the rate limit exceeded handler:
from fastapi.responses import JSONResponse
from slowapi.errors import RateLimitExceeded
@app.exception_handler(RateLimitExceeded)
async def rate_limit_exceeded_handler(request, exc):
return JSONResponse(
status_code=429,
content={"detail": "Rate limit exceeded. Please try again later."},
)
Problem: Applying rate limits without considering performance implications, which can lead to slow responses or increased latency.
Solution: Profile your application and consider optimizing your rate limiting logic, for instance using an efficient in-memory store like Redis for maintaining rate limit counters:
from redis import Redis
from slowapi.storage import RedisStorage
storage = RedisStorage(Redis())
limiter = Limiter(key_func=get_remote_address, storage=storage)
Problem: Not monitoring or logging rate limit events, making it hard to diagnose issues or understand usage patterns.
Solution: Integrate logging and monitoring for rate-limited requests to gain insights and promptly address issues. For instance, using the logging
module:
import logging
logger = logging.getLogger("rate_limit")
@app.exception_handler(RateLimitExceeded)
async def rate_limit_exceeded_handler(request, exc):
client_ip = request.client.host
logger.warning(f"Rate limit exceeded for IP: {client_ip}")
return JSONResponse(
status_code=429,
content={"detail": "Rate limit exceeded. Please try again later."},
)
Problem: Insufficient or improper testing of rate limits can lead to unexpected behavior in production.
Solution: Utilize tools like LoadForge to simulate traffic and test your rate limits under realistic conditions. Make sure to interpret the results and adjust your rate limits accordingly.
These common pitfalls and troubleshooting steps will help you mitigate issues and ensure the smooth implementation of rate limiting in your FastAPI application.
In this guide, we explored the concept of rate limiting and throttling within FastAPI, diving deep into both their necessity and implementation. These mechanisms are pivotal in protecting your application from abuse, ensuring fair resource usage, and maintaining a high-quality user experience.
Introduction to Rate Limiting and Throttling: We began with a primer on what rate limiting and throttling are, defining their significance in safeguarding your FastAPI application.
Prerequisites: Required software and knowledge needed to follow this guide, including FastAPI, Python, and necessary libraries.
Setting Up a Basic FastAPI Application: We guided you through setting up a foundational FastAPI application to serve as a base for implementing rate limits.
Understanding Rate Limiting and Throttling: A comprehensive examination of the different strategies and algorithms that can be utilized, such as Token Bucket, Leaky Bucket, and Fixed Window Counter.
Installing Required Dependencies:
Detailed instructions were provided to install all necessary dependencies like slowapi
to implement these rate limiting strategies.
Implementing Rate Limiting with SlowAPI: A step-by-step guide to applying rate limits in your FastAPI app, including middleware configuration and setting endpoint-specific limits.
Testing Your Rate Limits: Techniques and tools, including LoadForge, to rigorously test your rate limits to ensure they function as intended. We also discussed how to interpret test results and adjust configurations as needed.
Handling Rate Limit Exceeded Responses: Best practices were shared on handling responses when rate limits are exceeded, prioritizing good user experience and SEO implications.
Advanced Rate Limiting Techniques: We delved into more advanced topics, including distributed rate limiting, user-based limits, and IP-based limits to cater to complex scenarios.
Performance Considerations: Discussions on the performance impacts of rate limiting and ways to optimize server resources, ensuring smooth operations even under load.
Monitoring and Logging: We outlined best practices for monitoring and logging rate limit events, optimizing how you gain insights and react to rate limit activities.
Common Pitfalls and Troubleshooting: Guidance on avoiding and resolving common issues encountered during rate limit implementation.
Implementing rate limiting isn't a one-time task but a continuous process. It requires regular testing, monitoring, and tweaking to align with your application's evolving needs and traffic patterns. LoadForge can be a valuable tool in this ongoing process, helping you stress-test your application under different scenarios.
We encourage you to apply what you've learned and experiment with different rate limiting configurations. Share your experiences, challenges, and insights with the community or reach out with any questions. Continuous learning and community support are key to mastering any technical skill.
Thank you for following along with this guide. We're excited to see how you implement rate limiting in your FastAPI applications and look forward to your feedback.
By diligently applying the techniques and best practices discussed, you can significantly fortify your FastAPI application against abuse, ensuring a robust, fair, and user-friendly service.