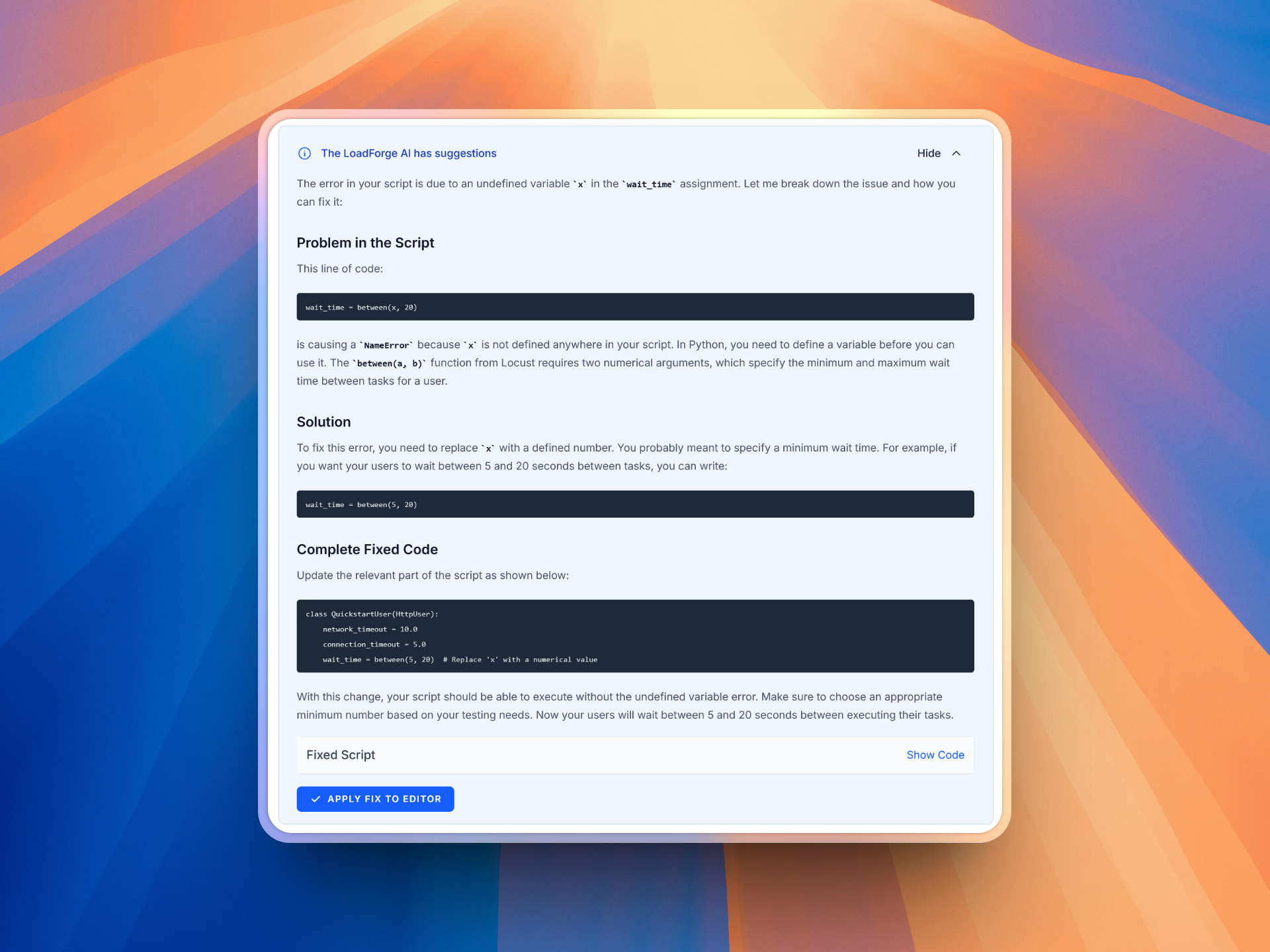
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
Caching is a critical performance optimization technique in web development that involves storing data so that future requests can be served faster. By reducing the amount of time and resources needed to retrieve data, caching significantly enhances the responsiveness and...
Caching is a critical performance optimization technique in web development that involves storing data so that future requests can be served faster. By reducing the amount of time and resources needed to retrieve data, caching significantly enhances the responsiveness and scalability of your application. In the context of a Spring HTTP Server, caching can transform a sluggish system into a high-performance machine capable of handling large volumes of traffic with ease.
Caching provides several key benefits, including:
Spring HTTP Server supports several caching strategies, each suited to different use-cases and requirements. Understanding these strategies is crucial for implementing the most effective caching solutions for your application.
In-Memory Caching: This strategy stores cache data in the application's memory, providing the fastest access times. It is ideal for scenarios where quick data retrieval is essential, but the data set is relatively small.
Examples: Caffeine, Ehcache
@Cacheable("books")
public Book findBookByIsbn(String isbn) {
// Method implementation
}
Distributed Caching: In this approach, cache data is stored across multiple servers, allowing for better scalability and fault tolerance. This strategy is suitable for large-scale applications where data must be shared among different instances of the application.
Example: Redis
@Bean
public RedisCacheManager cacheManager(RedisConnectionFactory factory) {
return RedisCacheManager.create(factory);
}
Client-Side Caching: HTTP headers like Cache-Control
, ETag
, and Last-Modified
can be used to store cache data on the client side. This reduces the number of requests made to the server, offloading work from your backend systems.
HTTP Headers Example:
@GetMapping("/resource")
public ResponseEntity<Resource> getResource() {
HttpHeaders headers = new HttpHeaders();
headers.setCacheControl("max-age=3600");
return new ResponseEntity<>(resource, headers, HttpStatus.OK);
}
Caching is an indispensable technique for optimizing the performance of a Spring HTTP Server. By understanding and implementing various caching strategies, you can improve your application's speed, reduce load on your backend systems, and enhance overall scalability. The subsequent sections will delve into the specific implementation details and best practices for each caching strategy. Stay tuned to learn how to set up and leverage these caching mechanisms to their full potential.
Caching is a critical technique for optimizing the performance of your Spring HTTP Server. By temporarily storing copies of frequently accessed data, caching reduces the load on your backend, improves response times, and enhances the overall user experience. There are several caching strategies you can implement in a Spring HTTP Server application, each with its unique benefits and use-cases. In this section, we will explore three main caching strategies: in-memory caching, distributed caching, and client-side caching.
In-memory caching stores data in the memory (RAM) of your application server. This type of caching offers fast read and write operations since data is stored close to the application logic.
Example with Spring Cache and Caffeine:
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import com.github.benmanes.caffeine.cache.Caffeine;
import org.springframework.cache.caffeine.CaffeineCacheManager;
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CaffeineCacheManager cacheManager() {
CaffeineCacheManager cacheManager = new CaffeineCacheManager();
cacheManager.setCaffeine(Caffeine.newBuilder().maximumSize(100).expireAfterWrite(10, TimeUnit.MINUTES));
return cacheManager;
}
}
Distributed caching involves storing cached data across multiple nodes in a cluster. This ensures that the cache is scalable and highly available, which is essential for large-scale applications.
Example with Spring Boot and Redis:
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.cache.RedisCacheManager;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.lettuce.LettuceConnectionFactory;
import org.springframework.data.redis.serializer.GenericJackson2JsonRedisSerializer;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
@EnableCaching
public class RedisCacheConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
return new LettuceConnectionFactory();
}
@Bean
public RedisCacheManager cacheManager(RedisConnectionFactory connectionFactory) {
RedisCacheManager.RedisCacheManagerBuilder builder = RedisCacheManager.builder(connectionFactory);
builder.cacheDefaults(RedisCacheConfiguration.defaultCacheConfig()
.serializeKeysWith(RedisSerializationContext.SerializationPair.fromSerializer(new StringRedisSerializer()))
.serializeValuesWith(RedisSerializationContext.SerializationPair.fromSerializer(new GenericJackson2JsonRedisSerializer())));
return builder.build();
}
}
Client-side caching leverages HTTP headers to instruct browsers and clients to cache responses locally. This helps reduce the number of requests that reach your server, indirectly enhancing server performance.
Example of HTTP headers for caching:
@RestController
public class ResourceController {
@GetMapping("/resource")
public ResponseEntity<String> getResource() {
HttpHeaders headers = new HttpHeaders();
headers.setCacheControl(CacheControl.maxAge(30, TimeUnit.MINUTES).cachePublic());
headers.setETag("\"12345\"");
headers.setLastModified(Instant.now());
return new ResponseEntity<>("Resource Content", headers, HttpStatus.OK);
}
}
By understanding and implementing these caching strategies, you can significantly improve the performance and scalability of your Spring HTTP Server application. Each strategy has its advantages and ideal use-cases, and sometimes a combination of these may provide the best results for your specific needs.
In-memory caching is one of the most efficient ways to speed up your Spring HTTP server by storing frequently accessed data in memory. Spring Cache provides robust support for various cache providers, including Caffeine and Ehcache. In this section, we will walk through configuring and implementing in-memory caching using Spring Cache with these two popular providers.
To get started with in-memory caching, you'll first need to add the necessary dependencies to your pom.xml
(for Maven) or build.gradle
(for Gradle) file.
Add the following dependencies for Spring Cache and cache providers:
<dependencies>
<!-- Spring Cache Dependency -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<!-- Caffeine Cache Dependency -->
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
</dependency>
<!-- Ehcache Dependency -->
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
</dependencies>
For Gradle users, include the following dependencies in your build.gradle
:
dependencies {
// Spring Cache
implementation 'org.springframework.boot:spring-boot-starter-cache'
// Caffeine Cache
implementation 'com.github.ben-manes.caffeine:caffeine'
// Ehcache
implementation 'org.ehcache:ehcache'
}
Next, enable caching in your Spring Boot application by adding the @EnableCaching
annotation to your main application class.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cache.annotation.EnableCaching;
@SpringBootApplication
@EnableCaching
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
To configure Caffeine as your cache provider, create a configuration class:
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.cache.caffeine.CaffeineCache;
import org.springframework.cache.concurrent.ConcurrentMapCacheManager;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import com.github.benmanes.caffeine.cache.Caffeine;
import java.util.concurrent.TimeUnit;
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public ConcurrentMapCacheManager cacheManager() {
ConcurrentMapCacheManager cacheManager = new ConcurrentMapCacheManager();
cacheManager.setCacheNames(List.of("users", "products"));
cacheManager.setCacheDefaults(defaultCacheConfig());
return cacheManager;
}
@Bean
public Caffeine<Object, Object> defaultCacheConfig() {
return Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(100);
}
}
For Ehcache, set up a configuration class and include the ehcache.xml
configuration file in your src/main/resources
directory.
import org.ehcache.jsr107.Eh107Configuration;
import org.springframework.cache.CacheManager;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.cache.jcache.JCacheCacheManager;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.cache.Cache;
import javax.cache.Caching;
import javax.cache.configuration.MutableConfiguration;
import javax.cache.spi.CachingProvider;
@Configuration
@EnableCaching
public class EhcacheConfig {
@Bean
public CacheManager cacheManager() {
CachingProvider provider = Caching.getCachingProvider();
javax.cache.CacheManager cacheManager = provider.getCacheManager(
getClass().getResource("/ehcache.xml").toURI(),
getClass().getClassLoader()
);
return new JCacheCacheManager(cacheManager);
}
@Bean
public Cache<Object, Object> defaultCache() {
MutableConfiguration<Object, Object> configuration = new MutableConfiguration<>()
.setStatisticsEnabled(true);
Cache<Object, Object> cache = cacheManager().getCache("defaultCache", Object.class, Object.class);
if (cache == null) {
cacheManager().createCache("defaultCache", Eh107Configuration.fromEhcacheCacheConfiguration(configuration));
}
return cache;
}
}
ehcache.xml
Upload an ehcache.xml
file to the src/main/resources
directory with the following content:
<config xmlns:xsi='http://www.w3.org/2001/XMLSchema-instance'
xmlns='http://www.ehcache.org/v3'
xsi:schemaLocation="http://www.ehcache.org/v3 http://www.ehcache.org/schema/ehcache-core-3.8.xsd">
<cache alias="defaultCache">
<expiry>
<ttl unit="minutes">10</ttl>
</expiry>
<resources>
<heap unit="entries">100</heap>
</resources>
</cache>
</config>
Finally, use the @Cacheable
annotation to specify which methods you'll cache. For example:
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Cacheable(value = "users", key = "#userId")
public User getUserById(Long userId) {
// Simulated method to fetch user from the database
return new User(userId, "John Doe");
}
}
By following these steps, you can effectively set up in-memory caching using Spring Cache with Caffeine or Ehcache. This setup will help reduce server load and improve your Spring application's performance. In the next section, we will delve into implementing distributed caching with Redis to further enhance the scalability and robustness of your caching strategy.
In this section, we will explore how to set up distributed caching using Redis in a Spring application. Distributed caching is crucial for scalable caching as it allows multiple instances of your application to share a common cache, ensuring consistency and improving performance across the board. Redis, an in-memory data structure store, is a popular choice for this purpose due to its high performance and ease of use.
First, ensure that your project has the necessary dependencies for using Redis with Spring. You can add these dependencies to your pom.xml
if you are using Maven.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.6.3</version>
</dependency>
Next, you need to configure Redis as your caching provider. Create a configuration class to set up Redis connection and cache manager.
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.cache.redis.RedisCacheManager;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.jedis.JedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
@Configuration
@EnableCaching
public class RedisConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
JedisConnectionFactory jedisConnectionFactory = new JedisConnectionFactory();
// Customize the connection details if necessary
// jedisConnectionFactory.setHostName("localhost");
// jedisConnectionFactory.setPort(6379);
return jedisConnectionFactory;
}
@Bean
public RedisTemplate<Object, Object> redisTemplate() {
RedisTemplate<Object, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory());
return template;
}
@Bean
public RedisCacheManager cacheManager(RedisConnectionFactory redisConnectionFactory) {
return RedisCacheManager.create(redisConnectionFactory);
}
}
Use the @EnableCaching
annotation in your Spring Boot application class to enable caching support.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cache.annotation.EnableCaching;
@SpringBootApplication
@EnableCaching
public class MySpringApplication {
public static void main(String[] args) {
SpringApplication.run(MySpringApplication.class, args);
}
}
Once caching is enabled, you can use the @Cacheable
, @CachePut
, and @CacheEvict
annotations to manage caching on methods. Here is an example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Cacheable(value = "users", key = "#userId")
public User getUserById(Long userId) {
// Method is cached.
return userRepository.findById(userId).orElse(null);
}
}
You may also want to customize cache properties such as expiration times. These configurations can be set in your application properties file:
spring.cache.redis.time-to-live=60000 # 1 minute in milliseconds
spring.cache.redis.cache-null-values=false # Do not cache null values
Distributed caching with Redis is particularly beneficial in scenarios where your application is deployed in a cluster or microservices architecture, allowing different instances to share the cached data. This reduces load on the primary data source and improves response times for repeated queries.
Benefits:
By integrating Redis for distributed caching, you can significantly enhance the performance and scalability of your Spring application.
With your Redis distributed cache now set up, you are ready to leverage the power of a centralized caching solution that scales with your application needs. In the next sections, we will explore advanced caching techniques and load testing strategies to ensure your cache implementation performs optimally under load.
Client-side caching is a crucial technique to optimize performance in web applications, particularly for reducing server load and improving response times. By instructing clients (browsers) to cache resources, we can significantly enhance the efficiency and responsiveness of our Spring HTTP Server. This section explores how to leverage HTTP headers like ETag, Cache-Control, and Last-Modified for effective client-side caching.
Cache-Control
is a versatile header that offers precise control over how, and for how long, resources are cached. It includes directives such as:
max-age
: Specifies the maximum amount of time a resource is considered fresh.no-cache
: Forces validation with the server before reuse.no-store
: Prevents caching entirely.Example
import org.springframework.http.CacheControl;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.concurrent.TimeUnit;
@RestController
public class ResourceController {
@GetMapping("/resource")
public ResponseEntity<String> getResource() {
return ResponseEntity.ok()
.cacheControl(CacheControl.maxAge(60, TimeUnit.SECONDS)) // Caches for 60 seconds
.body("Hello, World!");
}
}
ETags are unique identifiers assigned to each version of a resource. When the resource changes, the ETag changes. The client sends the ETag in a conditional request using the If-None-Match
header to check if the resource has been modified.
Example
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RestController;
import java.util.UUID;
@RestController
public class ETagController {
private String eTag = UUID.randomUUID().toString(); // Replace with actual ETag calculation
@GetMapping("/etag-resource")
public ResponseEntity<String> getETagResource(@RequestHeader(value = "If-None-Match", required = false) String ifNoneMatch) {
if (eTag.equals(ifNoneMatch)) {
return ResponseEntity.status(HttpStatus.NOT_MODIFIED).build(); // Resource not modified
}
return ResponseEntity.ok()
.eTag(eTag)
.body("Content with ETag.");
}
}
The Last-Modified
header indicates the date and time when the resource was last changed. Clients can use the If-Modified-Since
header to verify whether the resource has been updated since the last fetch.
Example
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RestController;
import java.time.Instant;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
@RestController
public class LastModifiedController {
private final ZonedDateTime lastModified = ZonedDateTime.now(); // Replace with actual last modified date
@GetMapping("/last-modified-resource")
public ResponseEntity<String> getLastModifiedResource(@RequestHeader(value = "If-Modified-Since", required = false) String ifModifiedSince) {
Instant sinceInstant = ifModifiedSince != null ? ZonedDateTime.parse(ifModifiedSince, DateTimeFormatter.RFC_1123_DATE_TIME).toInstant() : null;
if (sinceInstant != null && !lastModified.toInstant().isAfter(sinceInstant)) {
return ResponseEntity.status(HttpStatus.NOT_MODIFIED).build(); // Resource not modified
}
return ResponseEntity.ok()
.lastModified(lastModified.toInstant().toEpochMilli())
.body("Content with Last-Modified.");
}
}
For comprehensive client-side caching, you can combine these headers to leverage both validation and expiration mechanisms. Here’s an example of using Cache-Control
, ETag
, and Last-Modified
together:
Example
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.http.ResponseEntity;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
import java.util.UUID;
import java.util.concurrent.TimeUnit;
@RestController
public class CombinedCacheController {
private final String eTag = UUID.randomUUID().toString(); // Replace with actual ETag calculation
private final ZonedDateTime lastModified = ZonedDateTime.now(); // Replace with actual last modified date
@GetMapping("/combined-resource")
public ResponseEntity<String> getCombinedResource(@RequestHeader(value = "If-None-Match", required = false) String ifNoneMatch,
@RequestHeader(value = "If-Modified-Since", required = false) String ifModifiedSince) {
Instant sinceInstant = ifModifiedSince != null ? ZonedDateTime.parse(ifModifiedSince, DateTimeFormatter.RFC_1123_DATE_TIME).toInstant() : null;
if (eTag.equals(ifNoneMatch) || (sinceInstant != null && !lastModified.toInstant().isAfter(sinceInstant))) {
return ResponseEntity.status(HttpStatus.NOT_MODIFIED).build(); // Resource not modified
}
return ResponseEntity.ok()
.cacheControl(CacheControl.maxAge(60, TimeUnit.SECONDS).cachePrivate()) // Customize caching
.eTag(eTag)
.lastModified(lastModified.toInstant().toEpochMilli())
.body("Content with caching headers.");
}
}
Effectively utilizing client-side caching can dramatically reduce server load, decrease bandwidth usage, and improve load times for end-users. By leveraging headers such as Cache-Control
, ETag
, and Last-Modified
, you can finely tune how resources are cached and validated on the client side. Make sure to test your caching strategy thoroughly using tools like LoadForge to ensure optimal performance under various load conditions. Remember, the right caching strategy not only enhances performance but also provides a smoother experience for your users.
Effective caching isn't just about storing data; it's equally crucial to ensure that cached data remains fresh and relevant. This is where cache eviction and expiration strategies come into play. In this section, we will delve into different strategies for cache eviction and expiration, explaining how they contribute to efficient cache management in Spring HTTP Server applications.
Cache eviction and expiration strategies are mechanisms to remove stale or less frequently used data from the cache. These strategies help prevent the cache from becoming bloated with outdated or irrelevant information, ensuring optimal performance and data accuracy.
Time-to-Live (TTL): TTL specifies the duration for which a cache entry is valid. Once the TTL has elapsed, the cache entry is considered expired and is removed from the cache.
Example:
@Cacheable(value = "users", key = "#userId", cacheManager = "cacheManager")
public User getUserById(String userId) {
// Method implementation here
}
@Bean
public CacheManager cacheManager() {
CaffeineCacheManager cacheManager = new CaffeineCacheManager("users");
cacheManager.setCaffeine(Caffeine.newBuilder().expireAfterWrite(10, TimeUnit.MINUTES));
return cacheManager;
}
In this example, cached user data will expire 10 minutes after it is written to the cache.
Time-to-Idle (TTI): TTI defines the maximum time an entry can stay idle in the cache before it expires. This strategy is useful for ensuring only actively accessed data remains in the cache.
Example:
@Bean
public CacheManager cacheManager() {
CaffeineCacheManager cacheManager = new CaffeineCacheManager("users");
cacheManager.setCaffeine(Caffeine.newBuilder().expireAfterAccess(5, TimeUnit.MINUTES));
return cacheManager;
}
Here, the cached user data will expire if it has not been accessed for 5 minutes.
Least Recently Used (LRU): LRU is an eviction policy that removes the least recently accessed data when the cache reaches its maximum size. This is beneficial for retaining frequently accessed data.
Example:
@Bean
public CacheManager cacheManager() {
CaffeineCacheManager cacheManager = new CaffeineCacheManager("items");
cacheManager.setCaffeine(Caffeine.newBuilder().maximumSize(100).expireAfterWrite(10, TimeUnit.MINUTES));
return cacheManager;
}
This configuration sets a maximum cache size of 100 entries and uses LRU to evict the least recently accessed entries.
Custom Eviction Policies: Custom eviction policies can be implemented to handle specific use cases. These policies can be based on complex logic unique to your application's requirements.
Example:
public class CustomEvictionPolicy extends AbstractCacheManager {
@Override
protected Collection<? extends Cache> loadCaches() {
// Custom implementation here
}
@Override
protected Cache getMissingCache(String name) {
// Custom implementation here
}
}
Choosing the right eviction and expiration strategy depends on the nature of your application and data access patterns. Here are some guidelines:
Most caching providers integrated with Spring, like Caffeine and Ehcache, offer built-in support for TTL, TTI, and LRU policies. Here’s how you can configure these strategies in a Spring application:
@Bean
public CacheManager cacheManager() {
CaffeineCacheManager cacheManager = new CaffeineCacheManager("products");
cacheManager.setCaffeine(Caffeine.newBuilder()
.expireAfterWrite(5, TimeUnit.MINUTES) // TTL
.expireAfterAccess(2, TimeUnit.MINUTES) // TTI
.maximumSize(1000)); // LRU
return cacheManager;
}
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="http://www.ehcache.org/ehcache.xsd">
<cache name="usersCache"
maxEntriesLocalHeap="1000"
timeToLiveSeconds="300" <!-- TTL -->
timeToIdleSeconds="120"> <!-- TTI -->
</cache>
</ehcache>
@Bean
public CacheManager cacheManager() {
return new EhCacheCacheManager(ehCacheCacheManager().getObject());
}
@Bean
public EhCacheManagerFactoryBean ehCacheCacheManager() {
EhCacheManagerFactoryBean ehCacheManagerFactoryBean = new EhCacheManagerFactoryBean();
ehCacheManagerFactoryBean.setConfigLocation(new ClassPathResource("ehcache.xml"));
return ehCacheManagerFactoryBean;
}
Implementing the right cache eviction and expiration strategies is key to ensuring your cached data is both relevant and performant. By leveraging TTL, TTI, LRU, and custom policies in your Spring HTTP Server application, you can achieve a finely-tuned cache that enhances overall performance and reliability.
Up next, we will explore how to load test your caching strategy to validate its effectiveness using LoadForge.
Implementing a caching strategy is a crucial step in optimizing the performance of your Spring HTTP Server application, but ensuring that this strategy consistently meets performance goals under real-world conditions is equally important. Load testing allows you to simulate user traffic and measure how your caching strategy performs under various loads. LoadForge provides an efficient and user-friendly platform for this purpose. In this section, we will walk you through the process of using LoadForge to load test your caching strategy, from setting up tests to analyzing results and optimizing performance.
Sign Up and Set Up LoadForge Account:
Create a New Test:
Configure Load Profiles:
Add Scenario:
from loadforge import LoadForge
def scenario():
response = http.get("https://your-api-endpoint")
assert response.status_code == 200
LoadForge.run(scenario)
Run the Test:
Once the test is completed, LoadForge provides a comprehensive set of metrics and graphs to help you analyze the performance of your caching strategy.
Response Time and Throughput:
Error Rates:
Resource Utilization:
After gathering insights from your load test, consider the following optimization strategies:
Cache Configuration:
Code Optimization:
Scaling Infrastructure:
Suppose the test reveals that the cache hit ratio is lower than expected, causing unnecessary load on the database. You can optimize the situation by tuning cache settings:
# Adjusting cache settings in Spring configuration
spring.cache.caffeine.spec = maximumSize=1000,expireAfterAccess=5m
Then, re-run your load test to verify that the changes have improved performance.
Utilizing LoadForge for load testing helps ensure that your caching strategy is robust and effective under real-world traffic conditions. By identifying and addressing bottlenecks, you can optimize your Spring HTTP Server to deliver high performance and handle increased loads gracefully. Remember to regularly test and adjust your caching strategy as your application evolves.
Effectively monitoring and analyzing cache performance is crucial for ensuring that your Spring HTTP server maintains high performance and responsiveness. This section delves into techniques and tools for tracking cache metrics, diagnosing common issues, and optimizing your cache settings.
Monitoring the right metrics can provide insights into how well your caching strategy is performing. Here are some essential cache metrics to keep an eye on:
Several tools can help you monitor and analyze cache performance in a Spring application:
Spring Boot Actuator includes built-in support for cache metrics. Here's how you can configure it in your Spring Boot application:
Add the necessary dependencies in your pom.xml
or build.gradle
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Enable the cache metrics endpoint in your application.properties
:
management.endpoint.caches.enabled=true
management.endpoints.web.exposure.include=health,info,caches
Access the cache metrics via /actuator/caches
:
curl http://localhost:8080/actuator/caches
Micrometer integrates with many monitoring systems, such as Prometheus, Grafana, and more. Here's an example of how to configure Micrometer with Prometheus:
Add the Micrometer and Prometheus dependencies:
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-core</artifactId>
</dependency>
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
Configure Micrometer in your application.properties
:
management.metrics.export.prometheus.enabled=true
Expose the Prometheus endpoint and view metrics:
curl http://localhost:8080/actuator/prometheus
Here are some common cache-related issues and possible solutions:
Fine-tuning cache settings based on monitored metrics can significantly improve performance. Here are some optimization tips:
In conclusion, continuous monitoring and analysis are imperative to maintain an efficient caching strategy. Utilize the mentioned tools and techniques to gain insights, solve issues proactively, and optimize settings to keep your Spring HTTP server performant.
By thoroughly understanding and implementing these monitoring and analysis strategies, you can ensure that your caching mechanisms effectively reduce server load, decrease latency, and enhance user experience.
Caching is a critical component for optimizing performance in Spring HTTP Server applications. Implementing a well-designed caching strategy can significantly reduce response times, improve user experience, and decrease server load. Below are some best practices to follow when implementing caching in your Spring HTTP Server application:
Not all data is suitable for caching. Focus on identifying data that:
Ensuring you cache the right data can maximize the benefits and avoid unnecessary complexity.
Leverage different caching strategies based on your application needs:
Carefully analyze and align your caching strategy with your application's requirements.
Properly configuring your cache can significantly impact performance and behavior. For example, when using Spring Cache with a provider like Caffeine:
@Configuration
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
CaffeineCacheManager cacheManager = new CaffeineCacheManager();
cacheManager.setCaffeine(Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(1000));
return cacheManager;
}
}
Ensure that cached data remains fresh and relevant by implementing appropriate eviction and expiration strategies. Common policies include:
Consistent data is crucial in maintaining user trust and system integrity. Consider implementing the following practices:
Regularly monitor cache usage and performance to ensure optimal operation. Key metrics to track include:
Utilize tools like Spring Actuator, JMX, and custom logging to gather and analyze these metrics.
Avoid common pitfalls by following these optimization tips:
It’s essential to load test your caching strategy to identify bottlenecks and areas for improvement. Using LoadForge, you can simulate heavy load scenarios and analyze the performance of your caching implementation. This helps in making data-driven decisions to fine-tune your cache configuration.
Implement caching in a clean and modular manner. Use annotations like @Cacheable
, @CachePut
, and @CacheEvict
to keep your codebase maintainable and understandable:
@Service
public class ProductService {
@Cacheable("products")
public Product findProductById(Long id) {
// Method implementation
}
@CacheEvict(value = "products", key = "#id")
public void updateProduct(Long id, Product product) {
// Method implementation
}
}
By following these best practices, you can leverage caching optimally in your Spring HTTP Server application to enhance performance, ensure data consistency, and avoid common pitfalls. Remember that caching is not a one-size-fits-all solution, and continuous monitoring, testing, and tuning are essential for achieving the best results.
In this guide, we have comprehensively explored the various caching strategies available for Spring HTTP servers and provided detailed instructions on how to implement them. Caching is a vital optimization technique that can significantly improve the performance and scalability of your web application by reducing load times and minimizing redundant server requests. Let’s recap the key points discussed:
Introduction to Caching in Spring HTTP Server:
Understanding Different Caching Strategies:
Setting Up In-Memory Caching with Spring Cache:
@Cacheable("items")
public Item getItemById(Long id) {
// Database call
}
Implementing Distributed Caching with Redis:
spring:
cache:
type: redis
redis:
host: localhost
Client-Side Caching with HTTP Headers:
@GetMapping("/resource")
public ResponseEntity<Resource> getResource() {
HttpHeaders headers = new HttpHeaders();
headers.add("Cache-Control", "max-age=3600");
return ResponseEntity.ok()
.headers(headers)
.body(resource);
}
Cache Eviction and Expiration Strategies:
Load Testing Your Caching Strategy with LoadForge:
Monitoring and Analyzing Cache Performance:
Best Practices for Caching in Spring HTTP Server:
While the strategies and techniques covered in this guide provide a solid foundation, the key to achieving optimal performance lies in continuous experimentation and testing. Here are some steps you can take to further fine-tune your caching strategy:
By following these steps and continuously refining your approach, you can ensure that your Spring HTTP server performs optimally, providing a smooth and efficient user experience. Happy caching!