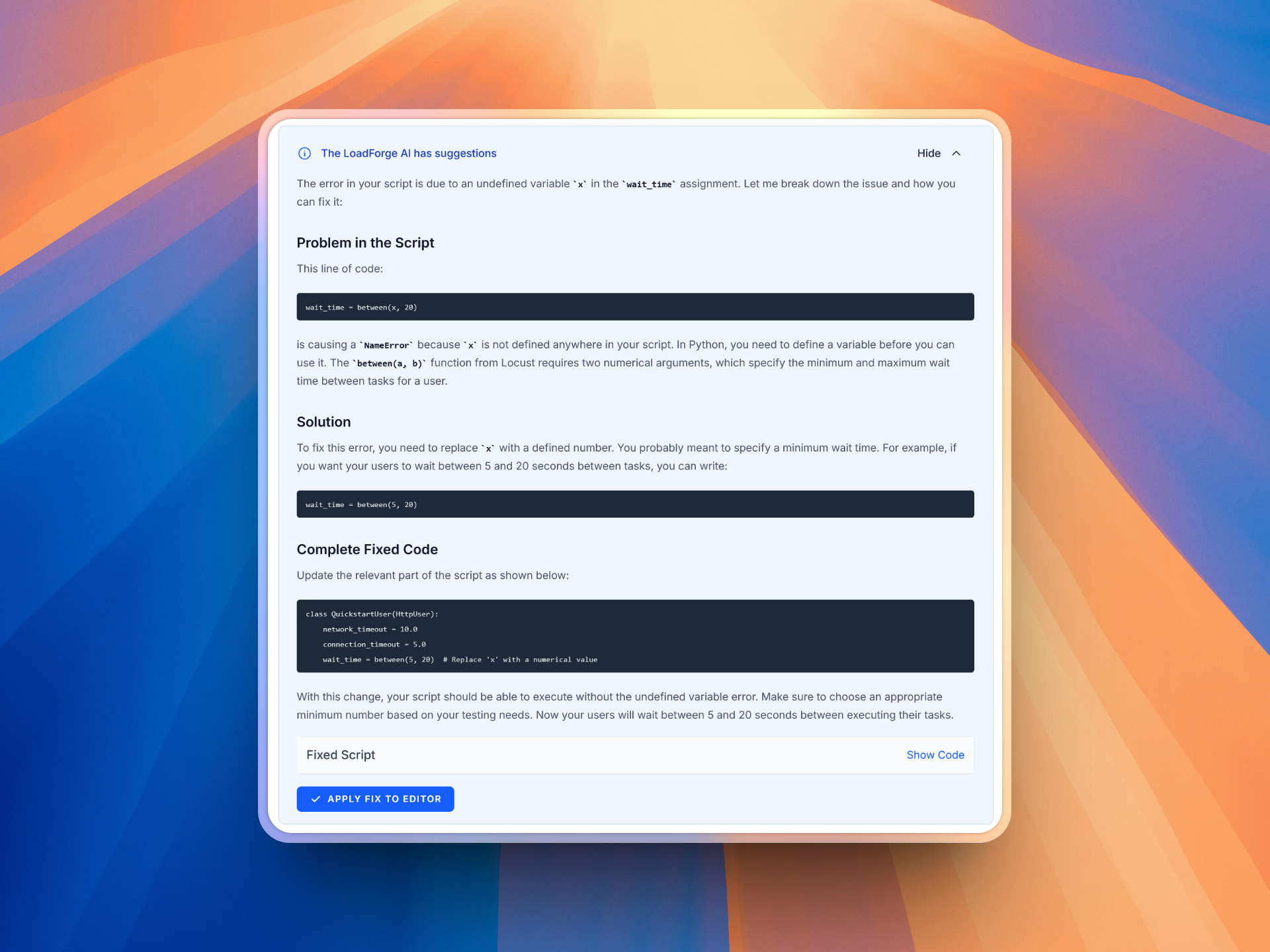
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
Ruby on Rails, commonly referred to as Rails, is a powerful web application framework written in the Ruby programming language. It provides developers with tools and conventions for building modern web applications efficiently. As Rails applications scale, especially those serving...
Ruby on Rails, commonly referred to as Rails, is a powerful web application framework written in the Ruby programming language. It provides developers with tools and conventions for building modern web applications efficiently. As Rails applications scale, especially those serving APIs, performance becomes a critical aspect to ensure a seamless user experience and maintain high levels of efficiency. In this guide, we'll delve into various strategies to enhance the performance of API endpoints in Ruby on Rails applications.
API performance is crucial for any web application, particularly as user loads increase and applications grow in complexity. The responsiveness of an API directly impacts user satisfaction, as slow response times can lead to poor user experiences and potential loss of traffic. Furthermore, efficient APIs contribute to better utilization of server resources, ensuring the application can handle more requests with less infrastructure.
Here are a few reasons why enhancing API performance in a Ruby on Rails application is essential:
User Experience: Fast APIs ensure that users receive responses quickly, improving the overall user experience. In the age of instant gratification, users expect quick interactions, and slow APIs can be detrimental to retention.
Scalability: As traffic to your application grows, the ability to handle more requests without a proportional increase in resource usage is vital. Optimized APIs help in scaling horizontally and vertically, accommodating rising user bases and expanding functionality.
Server Efficiency: Efficient APIs reduce the computational load on servers, which can lower operational costs and improve the application's reliability and uptime.
Resilience: A high-performance API helps in managing and mitigating risks associated with traffic spikes, ensuring the application remains responsive and available during peak loads.
Before diving into optimization techniques, it’s important to understand the key metrics that define API performance:
Response Time: The time it takes for the server to respond to a request. Lower response times are indicative of a more responsive API.
Throughput: The number of requests that can be processed per unit of time. High throughput indicates a capacity to handle more concurrent requests.
Error Rate: The percentage of requests that result in errors. A low error rate reflects a stable and reliable API.
Throughout this guide, we'll cover a comprehensive set of strategies to enhance your Rails application's API performance. You’ll learn about optimizing database interactions, implementing effective caching strategies, processing tasks in the background, designing efficient APIs, and much more. Each section is designed to provide practical insights and actionable steps, complete with code examples and best practices.
By the end of this guide, you'll have a robust understanding of how to identify performance bottlenecks, optimize your Ruby on Rails application, and ensure your API remains scalable and efficient as your user base grows.
Embark on this journey with us as we explore the depths of Ruby on Rails performance enhancement, setting your application on the path to excellence.
In order to build scalable and efficient web applications with Ruby on Rails, it is essential to grasp the fundamentals of API performance. This section delves into the key metrics that measure the performance of an API, including response time, throughput, and error rate. Understanding these metrics will provide a strong foundation for identifying and addressing performance bottlenecks.
Response time is the duration from when a request is sent by a client until the server sends back the corresponding response. Lower response times are crucial for a smooth user experience and better search engine rankings. Response time can be broken down into:
Monitoring response time will help identify slow endpoints and guide optimization efforts.
Throughput refers to the number of requests processed by the application per unit of time, typically measured in requests per second (RPS). It indicates the capacity of your API to handle workload and is a critical metric when considering the scalability of your application.
# Example: Checking throughput in a real-time scenario
# Here we simulate a simple endpoint hit and monitor throughput using Rails performance tools.
def perform_request
res = Net::HTTP.get_response(URI.parse('http://localhost:3000/api/v1/example'))
puts "#{res.code} - #{res.message}"
end
200.times { Thread.new { perform_request } }
Understanding throughput helps developers and administrators ensure the API can handle concurrent users and sustained traffic without degradation in performance.
Error rate is the percentage of requests that result in errors. Monitoring the error rate is essential to maintain the reliability and robustness of your API. A high error rate can indicate issues such as:
Error rates can be categorized by HTTP status codes:
Keeping a vigilant eye on error rates allows for quick identification and resolution of failures in your application.
Metric | Description | Importance |
---|---|---|
Response Time | Time taken to process and return a request | User experience and SEO |
Throughput | Number of requests handled per second | Scalability |
Error Rate | Percentage of requests that result in errors | Reliability and robustness |
Utilize various tools to monitor these key metrics:
Understanding API performance in terms of response time, throughput, and error rate provides a comprehensive view of how your application behaves under different conditions. This knowledge is critical for making informed decisions about optimizations and ensuring that your Ruby on Rails application delivers a seamless and reliable user experience. In the following sections, we will explore specific techniques to enhance these key metrics.
Optimizing database interactions is critical for enhancing the performance of your Ruby on Rails applications. Efficient database operations reduce latency, improve resource utilization, and ensure a smoother user experience. In this section, we’ll cover essential tips and best practices for optimizing your database interactions, including indexing strategies, query optimization, and leveraging ActiveRecord effectively.
Indexes are crucial for speeding up query operations. Without proper indexing, your database can become a major bottleneck. Here are some key practices for indexing:
add_index :posts, :user_id
When adding an index, ensure that it reflects the query patterns of your application. Use Rails migrations to add indexes:
class AddIndexToPosts < ActiveRecord::Migration[6.0]
def change
add_index :posts, :created_at
add_index :users, [:last_name, :first_name]
end
end
Poorly optimized queries can significantly degrade performance. Consider the following tips for query optimization:
SELECT *
. Instead, specify only the columns you need:
Post.select(:id, :title, :created_at)
pluck
for Simple Queries: pluck
is faster for retrieving specific columns:
User.pluck(:email)
includes
:
# Avoid N+1 queries
posts = Post.all
posts.each do |post|
puts post.user.name
end
# Use eager loading
posts = Post.includes(:user).all
posts.each do |post|
puts post.user.name
Post.find_each(batch_size: 1000) do |post|
# Process each post
end
ActiveRecord, Rails' ORM, offers powerful abstractions for database operations. Here are techniques to make the most of ActiveRecord:
class Post < ApplicationRecord
scope :published, -> { where(published: true) }
scope :recent, -> { order(created_at: :desc) }
end
Post.published.recent
find
, find_by
, and find_by!
for primary key lookups as they are optimized:
User.find(1)
User.find_by(email: '[email protected]')
ApplicationRecord.transaction do
user.update!(balance: user.balance - purchase.amount)
purchase.update!(status: 'completed')
end
By adhering to these best practices for database optimization, you can significantly enhance the performance of your Ruby on Rails application. Proper indexing, optimizing queries, and leveraging ActiveRecord effectively will lead to faster, more efficient data interactions, paving the way for scalable and robust web applications.
Caching is one of the most effective techniques to enhance the performance of Ruby on Rails applications by reducing database load and minimizing response times. Strategic implementation of caching can significantly improve your API's responsiveness and scalability. In this section, we will delve into various caching strategies, including fragment caching, page caching, and low-level caching.
Fragment caching allows you to cache parts of a view. This is particularly useful for pages where only a small portion of the content changes frequently. By caching static fragments, you can avoid redundant database queries and computation for the unchanged portions.
<% cache do %>
<%= render @comments %>
<% end %>
In this example, the comments section is cached. When comments remain unchanged, this cache helps in serving the content without re-rendering or querying the database.
Action caching is a more granular form of caching that caches the entire content generated by a controller action. This is suitable when you want to cache the entire response of an action while still allowing for changes due to before filters.
class ArticlesController < ApplicationController
caches_action :show
def show
@article = Article.find(params[:id])
end
end
Here, the show
action's output is cached, reducing the need to repeat the database fetch for the same article, which greatly improves response time.
Page caching is the simplest form of caching that involves caching the entire page, bypassing the Rails stack entirely. This is suitable for static content where database reads are not required for each request.
class ProductsController < ApplicationController
caches_page :index, :show
def index
@products = Product.all
end
def show
@product = Product.find(params[:id])
end
end
Here, the index
and show
actions are cached, meaning the rendered HTML is saved and directly served for subsequent requests without hitting the Rails stack.
Note: Page caching has been removed from Rails 4.0, and you may need to use rack-cache or other middleware for similar functionality.
Low-level caching allows you to cache any arbitrary data, not just view fragments or full pages. This is particularly useful for caching objects, query results, or computations that are expensive to fetch or calculate.
# Caching expensive query results
products = Rails.cache.fetch('all_products', expires_in: 12.hours) do
Product.all.to_a # Computationally expensive operation
end
In this example, the result of fetching all products is cached for 12 hours. Subsequent requests within this duration will read from the cache rather than hitting the database.
Rails supports various cache stores, such as:
Selecting the appropriate cache store depends on your application requirements and deployment environment.
# Configuring Redis as the cache store
config.cache_store = :redis_store, { url: 'redis://localhost:6379/0', namespace: 'cache' }
Proper cache expiration is crucial for ensuring data consistency. Rails provides helpers like expire_fragment
, expire_action
, and more to control cache expiry.
# Manually expiring a fragment cache
expire_fragment('comments_section')
# Automatically expire based on condition
<% cache @article do %>
<%= render @comments %>
<% end %>
In conclusion, caching is a robust strategy to enhance API performance by minimizing unnecessary database hits and decreasing response times. Strategic use of fragment caching, action caching, page caching, and low-level caching can make your Ruby on Rails application far more efficient and scalable.
Handling long-running tasks in the main thread of your Ruby on Rails application can lead to slow response times, dissatisfied users, and an overall sluggish experience. Offloading these tasks to a background job processing framework like Sidekiq can significantly boost your application's performance by freeing up the main thread to handle HTTP requests efficiently.
Background jobs are indispensable for tasks that are too time-consuming to be processed in the request-response cycle. These tasks include:
By processing these tasks in the background, you improve response times and ensure a smooth user experience.
Sidekiq is a popular and highly efficient background job processing library for Ruby that uses threads to handle many jobs at the same time in the same process. Let's go through the steps to set it up in your Rails application.
Add Sidekiq to your Gemfile:
gem 'sidekiq'
Install Redis: Sidekiq uses Redis for job management. You can install Redis through package managers or use a hosted service.
Create a Sidekiq Configuration File:
Create a configuration file at config/sidekiq.yml
:
:concurrency: 5
:queues:
- default
Create a Background Worker:
Generate a new worker:
rails generate sidekiq:worker Hard
This creates app/workers/hard_worker.rb
, where you define your long-running task:
class HardWorker
include Sidekiq::Worker
def perform(*args)
# long-running task here
sleep(10)
puts "Job done!"
end
end
Enqueue Jobs:
To enqueue jobs, you simply call the perform_async
method on your worker class:
HardWorker.perform_async('some_arg')
Update your config/application.rb
to set Sidekiq as the queue adapter:
module YourApp
class Application < Rails::Application
# Other configuration...
config.active_job.queue_adapter = :sidekiq
end
end
Sidekiq offers a Web UI to monitor job statistics. You can mount it in your Rails routes for easy access:
# config/routes.rb
require 'sidekiq/web'
mount Sidekiq::Web => '/sidekiq'
Accessing /sidekiq
in your browser will bring up the Sidekiq dashboard, where you can monitor job queues, retries, and failures.
Sending emails is a typical use case for background jobs. Here’s an example using ActionMailer
with Sidekiq:
Create a Mailer:
class UserMailer < ApplicationMailer
def welcome_email(user)
@user = user
mail(to: @user.email, subject: 'Welcome to My Awesome Site')
end
end
Create a Worker:
class EmailWorker
include Sidekiq::Worker
def perform(user_id)
user = User.find(user_id)
UserMailer.welcome_email(user).deliver_now
end
end
Enqueue the Job:
EmailWorker.perform_async(user.id)
By leveraging Sidekiq for background job processing, you can significantly enhance the performance of your Rails API. This setup allows your main application thread to remain responsive, providing a seamless experience to your users while handling intensive tasks in the background.
In the next section, we will delve into Efficient API Design, covering best practices for structuring endpoints and minimizing payloads for optimal performance.
## Efficient API Design
Designing an efficient API is paramount for ensuring that your Ruby on Rails application performs optimally. This section will delve into best practices for API design, focusing on RESTful conventions, endpoint structuring, and techniques to minimize payloads through serialization.
### Adhering to RESTful Conventions
REST (Representational State Transfer) is a widely adopted architectural style for designing networked applications. Designing your API following RESTful conventions ensures consistency, scalability, and ease of use. Here are some key principles:
1. **Use Meaningful Resource Names**: Your API endpoints should be named after the resource they represent and should be pluralized.
<pre><code>GET /articles</code></pre>
2. **Standard HTTP Methods**: Utilize the appropriate HTTP methods to perform CRUD (Create, Read, Update, Delete) operations.
- `GET` for retrieval
- `POST` for creation
- `PUT/PATCH` for updates
- `DELETE` for deletions
3. **Statelessness**: Each API request should contain all the information needed for the server to understand and process the request. This improves scalability and makes the API easier to debug.
4. **Error Handling**: Use standard HTTP status codes to indicate the result of an API request.
- `200 OK` for successful GET requests
- `201 Created` for successful resource creation
- `400 Bad Request` for invalid client input
- `500 Internal Server Error` for server issues
### Structuring Endpoints
Well-structured endpoints make your API more intuitive and efficient to use. Here are some tips for structuring your endpoints efficiently:
1. **Hierarchical Structure**: Use a hierarchical structure to represent relationships.
<pre><code>GET /users/:user_id/articles</code></pre>
2. **Versioning**: Version your API to manage changes without breaking existing clients.
<pre><code>GET /v1/articles</code></pre>
3. **Query Parameters**: Use query parameters for filtering, sorting, and pagination.
<pre><code>GET /articles?sort=created_at&order=desc&limit=10&page=2</code></pre>
4. **Singular Endpoints for Specific Actions**: For actions that do not map well to CRUD operations, use descriptive names.
<pre><code>POST /articles/:id/publish</code></pre>
### Minimizing Payloads Through Serialization
Reducing the amount of data transferred between the server and clients can significantly enhance performance. Here are some strategies:
1. **Serialization**: Use serializers to control the JSON output.
```ruby
class ArticleSerializer < ActiveModel::Serializer
attributes :id, :title, :summary, :author_name
def author_name
object.author.name
end
end
Selective Fields: Allow clients to specify the fields they need.
GET /articles?fields=id,title,summary
class ApplicationController < ActionController::API
def render_with_selected_fields(resource, fields)
if fields
fields = fields.split(',')
render json: resource, only: fields
else
render json: resource
end
end
end
Pagination: Implement pagination to limit the amount of data returned in a single response.
class ArticlesController < ApplicationController
def index
articles = Article.page(params[:page]).per(params[:per_page])
render json: articles
end
end
Compression: Enable gzip compression in your Rails application to reduce the payload size.
# config/environments/production.rb
config.middleware.use Rack::Deflater
Efficient API design is integral to achieving optimal performance in Ruby on Rails applications. By adhering to RESTful conventions, structuring endpoints thoughtfully, and minimizing payload sizes through appropriate serialization techniques, you can create an API that is both performant and scalable.
In the world of API development, ensuring fair use of resources and protecting your application from abuse is crucial. Implementing rate limiting and throttling mechanisms can safeguard your API from malicious attacks, prevent server overload, and guarantee an equitable distribution of resources to all clients. This section delves into the concepts of rate limiting and throttling, and demonstrates how to implement them effectively in a Ruby on Rails application.
Rate Limiting: This technique restricts the number of requests an API client can make within a specified time frame. For example, an API could be configured to allow no more than 100 requests per minute per user.
Throttling: While similar to rate limiting, throttling tends to be more dynamic, adjusting the allowed request rate based on the load on the server. It ensures the system doesn't get overwhelmed by gradually reducing the allocated rate as the load increases.
Rails provides a robust ecosystem through which we can enforce rate limiting and throttling. One popular gem for rate limiting is rack-attack
.
Add the rack-attack
gem to your Gemfile:
gem 'rack-attack'
Run the bundle command to install it:
bundle install
Create an initializer for rack-attack
in config/initializers/rack_attack.rb
:
class Rack::Attack
# Throttle requests to 5 requests per second per IP
throttle('req/ip', limit: 5, period: 1.second) do |req|
req.ip
end
# Throttle login attempts for a given email parameter to 6 reqs/minute
# Key: "rack::attack:#{Time.now.to_i/:period}:logins:#{req.ip}"
throttle('logins/email', limit: 6, period: 60.seconds) do |req|
if req.path == '/login' && req.post?
req.params['email'].presence
end
end
# Block any IP that has tried to login more than 20 times in 1 hour
blocklist('login/ip') do |req|
Rack::Attack::Allow2Ban.filter(req.ip, maxretry: 20, findtime: 1.hour, bantime: 1.hour) do
req.path == '/login' && req.post?
end
end
end
This configuration does the following:
While rack-attack
offers a basic form of throttling, Rails can leverage more advanced techniques by incorporating middleware or external services like Redis.
Here's an example of how you might use Redis to implement dynamic throttling:
Add the redis
gem to your Gemfile:
gem 'redis'
Install it by running:
bundle install
Create a middleware throttle_middleware.rb
:
class ThrottleMiddleware
def initialize(app)
@app = app
@redis = Redis.new
end
def call(env)
request = Rack::Request.new(env)
client_ip = request.ip
# Get request count for the IP
request_count = @redis.get(client_ip).to_i
if request_count >= 10
[429, { 'Content-Type' => 'application/json' }, [{ error: 'Rate limit exceeded' }.to_json]]
else
@redis.incr(client_ip)
@redis.expire(client_ip, 60)
@app.call(env)
end
end
end
In your config/application.rb
, insert the middleware:
config.middleware.use "ThrottleMiddleware"
In this setup, requests from each IP are stored in Redis, and if the number of requests exceeds the permitted limit (in this case, 10 requests per minute), the middleware responds with a 429 Too Many Requests
status code.
Rate limiting and throttling are essential strategies for maintaining the health and efficiency of your Ruby on Rails API. By incorporating gems like rack-attack
for simple rate limiting and Redis for more dynamic throttling, you can protect your application from abuse and ensure fair resource allocation. Always tailor these mechanisms to your application's specific requirements and continuously monitor their effectiveness in a production environment.
Monitoring and profiling are crucial steps in maintaining and enhancing the performance of your Ruby on Rails API. By continuously monitoring your application, you can identify performance bottlenecks and optimize them effectively. This section will delve into various tools and methods such as New Relic, Skylight, and native Rails profiling to help you keep your application running smoothly.
Before diving into the tools and methods, it's important to understand why monitoring and profiling are essential:
New Relic is a comprehensive monitoring tool that offers in-depth insights into your application's performance.
Getting Started with New Relic:
Add the New Relic Gem: Include the New Relic agent in your Gemfile.
gem 'newrelic_rpm'
Configure New Relic: Use the New Relic configuration file (newrelic.yml
) to set up your environment. You need to add your New Relic license key here.
Deploy and Monitor: Once configured, deploy your application. New Relic will start collecting data that you can view on the New Relic dashboard.
Key Metrics in New Relic:
Skylight is another powerful tool tailored for Ruby on Rails applications. It provides actionable insights into your application's performance.
Setting Up Skylight:
Add the Skylight Gem: Include Skylight in your Gemfile.
gem 'skylight'
Configuration: Run the Skylight setup to configure the gem, which involves setting up an API token.
bundle exec skylight setup <YOUR_SKYLIGHT_API_TOKEN>
Deploy and Analyze: Once set up, deploy your application. Skylight will provide visualizations of your application's performance metrics on the Skylight dashboard.
Features of Skylight:
Rails provides built-in tools to help you profile your code without additional gems.
Using rails performance
:
Generate a New Performance Test:
rails generate performance_test my_model
Edit the Generated Test: Modify the generated test file in test/performance
. For example:
require 'test_helper'
require 'rails/performance_test_help'
class MyModelTest < ActionDispatch::PerformanceTest
def test_my_method
get my_model_path
end
end
Run the Test:
rake test:benchmark
Using the Benchmark Module:
For simple profiling, the Benchmark
module in Ruby is quite handy:
require 'benchmark'
Benchmark.bm do |x|
x.report("My Method") { MyModel.my_method }
end
This will output the time taken by my_method
.
While tools like New Relic and Skylight provide extensive production insights, using lightweight profiling during development can be beneficial:
Rack Mini Profiler:
Add the Gem:
gem 'rack-mini-profiler', group: :development
Mount the Middleware:
if Rails.env.development?
use Rack::MiniProfiler
end
Bullet:
Add the Gem:
gem 'bullet', group: :development
Configure Bullet: Configure Bullet in config/environments/development.rb
.
Bullet.enable = true
Bullet.alert = true
Monitoring and profiling your Ruby on Rails API performance is not a one-time task but a continuous process. Tools like New Relic, Skylight, and native Rails profiling can give you the insights needed to optimize and maintain the performance of your application effectively. Employ these tools to gain a deeper understanding of your application's behavior, identify bottlenecks, and keep your API running smoothly.
Load testing is a crucial step in ensuring that your Ruby on Rails application can handle high traffic and perform optimally under stress. In this section, we will explore how to perform load testing using LoadForge. This detailed guide will help you simulate traffic, measure performance under stress, and identify potential weaknesses in your application.
To get started with LoadForge, you need to create an account and set up a new test. Here are the steps:
LoadForge allows you to write complex test scenarios using a user-friendly interface. Here’s an example of how you can define a test scenario to assess the performance of a Rails API endpoint:
// Sample LoadForge test scenario
{
"scenarios": [
{
"name": "Basic API Test",
"requests": [
{
"method": "GET",
"url": "/api/v1/resources",
"headers": {
"Authorization": "Bearer YOUR_API_KEY"
}
},
{
"method": "POST",
"url": "/api/v1/resources",
"headers": {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_API_KEY"
},
"body": {
"data": {
"type": "resource",
"attributes": {
"name": "Sample Resource"
}
}
}
}
]
}
]
}
Once your test scenarios are ready, it's time to run the test:
With the results from LoadForge, you can identify performance bottlenecks. Here are some of the common indicators to look for:
Load testing should not be a one-time activity. Integrate LoadForge into your continuous integration (CI) pipeline to ensure consistent performance monitoring and optimization. By regularly running load tests, you can proactively identify and address performance issues before they impact your users.
Here's how you can integrate LoadForge into a CI pipeline, using a shell script as an example:
#!/bin/bash
# Example CI integration script for LoadForge
# Run LoadForge test
response=$(curl -s -X POST "https://api.loadforge.com/v1/tests/run" \
-H "Authorization: Bearer YOUR_API_KEY" \
-H "Content-Type: application/json" \
-d '{"test_id": "YOUR_TEST_ID"}')
# Check test result
test_result=$(echo $response | jq .result)
if [ "$test_result" != "success" ]; then
echo "LoadForge test failed: $response"
exit 1
fi
echo "LoadForge test passed successfully"
By following these guidelines and leveraging the capabilities of LoadForge, you can ensure that your Ruby on Rails application performs reliably under load, delivering a positive user experience even during peak traffic times.
Scaling a Ruby on Rails application involves increasing its capacity to handle a growing number of requests. Depending on your application's needs, you can achieve this through horizontal scaling, vertical scaling, or a combination of both. This section will cover the techniques for scaling Rails applications, including the use of load balancers, optimizing server configurations, and employing distributed systems.
Horizontal scaling, or scaling out, involves adding more servers to handle additional load. This method distributes incoming traffic across multiple servers, ensuring no single server becomes a bottleneck.
Load balancers play a crucial role in horizontal scaling by directing incoming requests to different servers based on various algorithms (e.g., round-robin, least connections). Here's a basic example of setting up an NGINX load balancer:
http {
upstream my_rails_app {
server 192.168.1.10;
server 192.168.1.11;
server 192.168.1.12;
}
server {
listen 80;
location / {
proxy_pass http://my_rails_app;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
}
This simple configuration distributes the traffic among three backend servers.
Vertical scaling, or scaling up, involves increasing the resources (CPU, memory, etc.) of your existing server. This can be a quick way to handle additional load, but it has its limits due to hardware constraints.
Optimizing your server configurations can improve performance significantly. Here are a few tips:
Unicorn/Passenger Configuration: Adjust the number of worker processes according to your CPU cores.
# config/unicorn.rb
worker_processes 4
preload_app true
timeout 30
Database Connections: Ensure your database connection pool is adequately sized.
# config/database.yml
production:
adapter: postgresql
encoding: unicode
pool: 15
timeout: 5000
Distributed systems help in offloading certain tasks from the main application servers, minimizing load, and enhancing reliability and performance.
A distributed caching system like Memcached or Redis can be used to cache frequently accessed data, reducing database load.
# Gemfile
gem 'redis'
gem 'redis-rails'
# config/environments/production.rb
config.cache_store = :redis_cache_store, { url: ENV['REDIS_URL'] }
Using a distributed database system (like Amazon RDS, Google Cloud SQL) ensures high availability and scalability. You can also employ read replicas to distribute read operations.
Using Docker for containerization and Kubernetes for orchestration allows you to effortlessly manage multiple containerized instances of your Rails application. This setup ensures seamless horizontal scaling.
# Dockerfile
FROM ruby:2.7
RUN mkdir /app
WORKDIR /app
COPY . /app
RUN bundle install
CMD ["rails", "server", "-b", "0.0.0.0"]
# kubernetes-deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-rails-app
spec:
replicas: 3
template:
metadata:
labels:
app: my-rails-app
spec:
containers:
- name: rails
image: my-rails-app:latest
---
apiVersion: v1
kind: Service
metadata:
name: my-rails-app
spec:
selector:
app: my-rails-app
ports:
- protocol: TCP
port: 80
targetPort: 3000
type: LoadBalancer
Scaling your Ruby on Rails application involves a combination of strategies that fit your specific needs, whether through horizontal scaling, vertical scaling, or employing distributed systems. Implementing load balancers, optimizing server configurations, and utilizing modern tools like Docker and Kubernetes can significantly enhance your application's performance and reliability. As your application grows, continuously monitor and adjust your scaling strategies to maintain optimal performance.
In this guide, we explored various techniques and best practices to enhance API performance in Ruby on Rails applications. By focusing on key areas such as database optimization, caching strategies, background job processing, efficient API design, rate limiting and throttling, monitoring and profiling, load testing with LoadForge, and scaling, we can ensure that our applications remain scalable, responsive, and efficient.
Understanding API Performance:
Database Optimization:
Caching Strategies:
Background Job Processing:
Efficient API Design:
ActiveModel::Serializers
.Rate Limiting and Throttling:
Monitoring and Profiling:
Load Testing with LoadForge:
Scaling Your Rails Application:
Enhancing API performance is not a one-time effort; it requires continuous monitoring, testing, and optimization. By regularly analyzing performance metrics, running load tests, and employing profiling tools, you can identify bottlenecks and optimize your application accordingly. Remember, a well-optimized API not only provides a better user experience but also reduces infrastructure costs and enhances the scalability of your application.
To stay ahead, adopt a proactive approach to performance management. Regularly revisit your code, database queries, and caching strategies. Implement automated tests and monitor their results frequently. The goal is to create a culture of performance awareness within your development team, ensuring that every code change is made with efficiency in mind.
By applying the insights and practices outlined in this guide, you can build high-performance Ruby on Rails applications that are capable of handling the demands of modern web traffic. Keep learning, keep optimizing, and your Rails applications will continue to deliver outstanding performance.