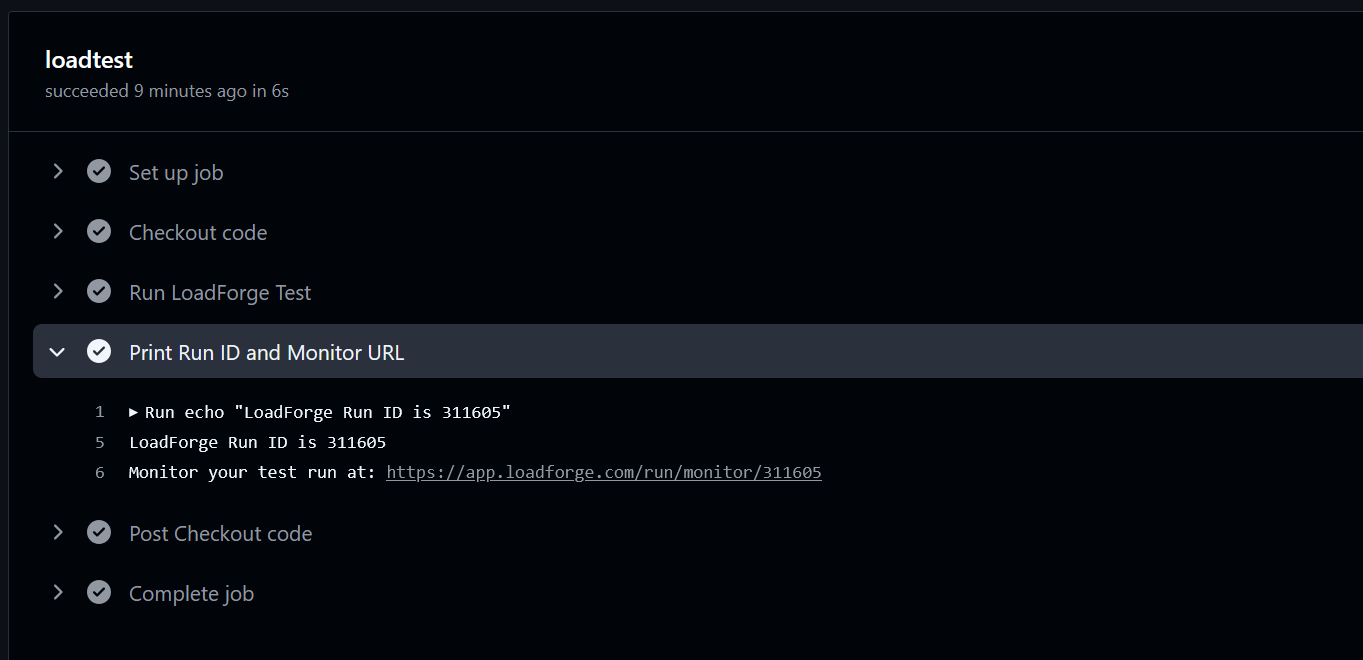
LoadForge GitHub Integration
Performance testing just got a major upgrade. LoadForge is thrilled to announce a seamless GitHub integration that lets you launch...
Efficient database operations are at the heart of any high-performance web application. In a NestJS application, which relies heavily on non-blocking I/O and robust architecture to deliver scalable and maintainable services, the efficiency of your database interactions can make or...
Efficient database operations are at the heart of any high-performance web application. In a NestJS application, which relies heavily on non-blocking I/O and robust architecture to deliver scalable and maintainable services, the efficiency of your database interactions can make or break the overall performance of your system. This guide aims to equip you with the best practices and techniques to optimize database operations within your NestJS applications.
Handling data efficiently is crucial for ensuring low latency, high throughput, and scalability of your application. Whether you are developing a small application or a large-scale enterprise solution, here are some key reasons why focusing on efficient database operations is critical:
In this guide, we'll take a deep dive into several key areas to help you master efficient database operations in NestJS:
async/await
for better performance.By the end of this guide, you will have a thorough understanding of how to optimize your database operations, ensuring your NestJS applications are performant, scalable, and responsive. Let's embark on this journey towards mastering efficient database operations in NestJS.
Selecting the appropriate database and ORM (Object-Relational Mapping) is crucial for ensuring seamless integration and efficient data handling in NestJS applications. The choice largely depends on your project's specific requirements, including scalability, complexity, and performance needs. In this section, we'll explore the various options available and provide guidance on how to make an informed decision.
Before diving into specific databases and ORMs, it's important to understand your application's requirements:
Here are some popular databases you might consider:
NestJS integrates seamlessly with several ORMs, providing various options depending on your chosen database. Here are a few notable ones:
TypeORM: A highly popular ORM for TypeScript and JavaScript, TypeORM supports MySQL, PostgreSQL, SQLite, and more. It offers a rich set of features, including active-record and data-mapper patterns.
Example: Integrating TypeORM with NestJS
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm'; import { User } from './user.entity'; import { UsersService } from './users.service'; import { UsersController } from './users.controller';
@Module({ imports: [ TypeOrmModule.forRoot({ type: 'postgres', host: 'localhost', port: 5432, username: 'test', password: 'test', database: 'test', entities: [User], synchronize: true, }), TypeOrmModule.forFeature([User]), ], providers: [UsersService], controllers: [UsersController], }) export class UsersModule {}
Sequelize: A promise-based Node.js ORM for relational databases like PostgreSQL, MySQL, and SQLite. Sequelize provides a strong TypeScript support and a flexible, low-level API.
Example: Integrating Sequelize with NestJS
import { Module } from '@nestjs/common';
import { SequelizeModule } from '@nestjs/sequelize'; import { User } from './user.model'; import { UsersService } from './users.service'; import { UsersController } from './users.controller';
@Module({ imports: [ SequelizeModule.forRoot({ dialect: 'mysql', host: 'localhost', port: 3306, username: 'root', password: 'root', database: 'test', models: [User], }), SequelizeModule.forFeature([User]), ], providers: [UsersService], controllers: [UsersController], }) export class UsersModule {}
Mongoose: For those choosing MongoDB, Mongoose is a popular ODM that allows you to define schemas and interact with MongoDB using models.
Example: Integrating Mongoose with NestJS
import { Module } from '@nestjs/common';
import { MongooseModule } from '@nestjs/mongoose'; import { UserSchema } from './schemas/user.schema'; import { UsersService } from './users.service'; import { UsersController } from './users.controller';
@Module({ imports: [ MongooseModule.forRoot('mongodb://localhost/nest'), MongooseModule.forFeature([{ name: 'User', schema: UserSchema }]), ], providers: [UsersService], controllers: [UsersController], }) export class UsersModule {}
Feature | TypeORM | Sequelize | Mongoose |
---|---|---|---|
Languages | TypeScript/JavaScript | TypeScript/JavaScript | JavaScript (strong TypeScript module) |
Databases | MySQL, PostgreSQL, SQLite, more | PostgreSQL, MySQL, SQLite, more | MongoDB |
Patterns | Active-Record, Data-Mapper | Active-Record | Schema-based |
Learning Curve | Moderate | Moderate | Easy to Moderate |
Community Support | High | High | High |
Selecting the right database and ORM is foundational to achieving efficient database operations in your NestJS application. By considering your project's specific needs and evaluating the pros and cons of various databases and ORMs, you can make an informed decision that balances performance, scalability, and ease of use. In the subsequent sections, we’ll delve deeper into optimizing these choices to further enhance your application's performance.
Efficient database connectivity is paramount in any database-driven application. One of the key techniques to optimize database operations in NestJS is through connection pooling. This section explains how to implement and configure connection pooling to reduce the overhead associated with frequently creating and closing database connections.
Connection pooling is a method used to minimize the cost of establishing connections to the database. Instead of creating and closing a new connection for each individual database operation, connection pooling maintains a pool of open connections ready to be reused. This results in faster execution times and better resource management, essentially allowing the database and the application to handle more requests seamlessly.
To implement connection pooling in NestJS, you need to appropriately configure your ORM (e.g., TypeORM or Sequelize). Here’s a step-by-step guide to setting up connection pooling:
TypeORM is one of the most popular ORMs in the NestJS ecosystem. Below is an example configuration in ormconfig.json
that includes connection pooling settings.
{
"type": "postgres",
"host": "localhost",
"port": 5432,
"username": "test",
"password": "test",
"database": "test",
"synchronize": true,
"logging": false,
"entities": [
"dist/**/*.entity.js"
],
"cli": {
"entitiesDir": "src/entity"
},
"extra": {
"max": 10, // maximum number of clients in the pool
"min": 2, // minimum number of clients in the pool
"idleTimeoutMillis": 30000 // close idle clients after 30 seconds
}
}
In the example, extra
is used to specify connection pooling settings:
max
: The maximum number of clients in the pool.min
: The minimum number of clients in the pool.idleTimeoutMillis
: Defines how long a client must sit idle in the pool before being closed.Setting up TypeORM in NestJS
You will also need to integrate this configuration within your NestJS application module:
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
@Module({
imports: [
TypeOrmModule.forRoot({
type: 'postgres',
host: 'localhost',
port: 5432,
username: 'test',
password: 'test',
database: 'test',
entities: [__dirname + '/**/*.entity{.ts,.js}'],
synchronize: true,
extra: {
max: 10,
min: 2,
idleTimeoutMillis: 30000,
},
}),
],
})
export class AppModule {}
If you prefer Sequelize, here’s how you can set up connection pooling:
import { Sequelize } from 'sequelize-typescript';
const sequelize = new Sequelize({
dialect: 'postgres',
host: 'localhost',
port: 5432,
username: 'test',
password: 'test',
database: 'test',
models: [__dirname + '/models'],
pool: {
max: 10,
min: 2,
idle: 30000,
acquire: 60000,
}
});
In this example, the pool
object within the Sequelize configuration specifies:
max
: Maximum number of connections in the pool.min
: Minimum number of connections in the pool.idle
: Maximum time, in milliseconds, that a connection can be idle before being released.acquire
: The maximum time, in milliseconds, that pool will try to get a connection before throwing an error.Optimal Pool Sizes: The optimal pool sizes (max
and min
values) depend on your application's concurrency levels and the database's capacity. Analyze your workload and test with different configurations to find the sweet spot.
Time-Out Settings: Configuring appropriate idle
and acquire
timeout values helps in efficiently managing the pool, ensuring idle connections are freed without waiting too long, and avoiding too many clients waiting for a connection.
Monitoring: Regularly monitor the connection pool statistics and database server performance. Adjust the pool settings based on the observed metrics and usage patterns.
Error Handling: Implement robust error handling to catch and manage exceptions that might occur due to connection pool exhaustion or other related issues.
By implementing and tuning connection pooling, you can significantly enhance the performance and scalability of your NestJS applications, ensuring efficient and reliable database operations.
Efficient database queries are crucial for the overall performance of your NestJS application. Poorly optimized queries can lead to slow response times, increased server load, and a subpar user experience. In this section, we will cover techniques for writing efficient database queries, including the use of indexes, avoiding SELECT *
, and implementing pagination for large data sets.
Indexes are essential for improving the speed of data retrieval operations. They allow the database to find rows quickly without scanning the entire table. Here are some key points on using indexes effectively:
WHERE
, JOIN
, ORDER BY
, and GROUP BY
clauses, and create indexes on those columns.CREATE INDEX idx_user_email ON users(email);
SELECT *
Using SELECT *
retrieves all columns from a table, which might include unnecessary data and increase the amount of data transferred between the database and your NestJS application. Instead, specify only the columns you need.
-- Inefficient query
SELECT * FROM users WHERE email = '[email protected]';
-- Optimized query
SELECT id, email, first_name, last_name FROM users WHERE email = '[email protected]';
When dealing with large datasets, returning the entire dataset in a single query can lead to performance issues. Pagination breaks the data into smaller, more manageable chunks.
import { getRepository } from 'typeorm';
import { User } from './user.entity';
async function getUsers(page: number, limit: number) {
const userRepository = getRepository(User);
const [users, total] = await userRepository.findAndCount({
skip: (page - 1) * limit,
take: limit,
});
return {
data: users,
total,
page,
pages: Math.ceil(total / limit),
};
}
EXPLAIN
in MySQL/PostgreSQL) to understand query performance and identify bottlenecks.Effective query optimization can lead to significant performance improvements in your NestJS application. Keep the discussed techniques in mind to ensure your database operations are as efficient as possible.
Efficient caching strategies are crucial for reducing database load and improving response times in your NestJS application. By temporarily storing frequently accessed data in a high-speed storage layer, you can alleviate the pressure on your database and provide faster data access to your users. Let's explore the different caching techniques you can implement in your NestJS applications with a focus on in-memory caching using Redis.
Caching involves storing copies of data in a storage medium that's faster to access than the primary data store. Here are two primary types of caching mechanisms you can leverage in a NestJS application:
We'll primarily focus on Redis, a powerful tool for implementing both in-memory and distributed caching strategies in NestJS.
To get started with Redis in NestJS, you'll need to install the necessary dependencies and configure the Redis client in your application.
Install Dependencies:
npm install --save @nestjs-modules/cache cache-manager cache-manager-redis-store redis
Configure the Cache Module:
In your app.module.ts
, import and configure the CacheModule
:
import { CacheModule, Module } from '@nestjs/common';
import * as redisStore from 'cache-manager-redis-store';
@Module({
imports: [
CacheModule.register({
store: redisStore,
host: 'localhost', // Redis server address
port: 6379, // Redis port
ttl: 600, // Time to live in seconds
}),
],
// other imports and providers
})
export class AppModule {}
To leverage the cache in your NestJS services, inject the CACHE_MANAGER
and use it to get and set cache entries.
Injecting Cache Manager:
import { Injectable, Inject, CACHE_MANAGER } from '@nestjs/common';
import { Cache } from 'cache-manager';
@Injectable()
export class DataService {
constructor(@Inject(CACHE_MANAGER) private cacheManager: Cache) {}
async getData(key: string): Promise<any> {
const cachedData = await this.cacheManager.get(key);
if (cachedData) {
return cachedData;
}
// Fetch data from the database if not found in cache
const data = await this.fetchDataFromDatabase(key);
// Store the fetched data in cache for future use
await this.cacheManager.set(key, data, { ttl: 600 });
return data;
}
private fetchDataFromDatabase(key: string): Promise<any> {
// Database fetch operation
}
}
Cache-Aside (Lazy Loading):
In this strategy, the application first checks the cache. If the data is not found, it fetches the data from the database and then stores it in the cache for future requests.
Write-Through:
Every time data is written to the database, it is also written to the cache, ensuring that the cache is always in sync with the database.
Write-Behind (Write-Back):
Data is written to the cache and is asynchronously written to the database afterward. This strategy can reduce write latency but requires careful handling to avoid data loss.
Cache Eviction Policies:
Implementing appropriate cache eviction policies such as Least Recently Used (LRU) or Time-To-Live (TTL) ensures that your cache doesn't grow indefinitely and remains efficient.
To cache user profiles that are frequently accessed, you can use the Cache-Aside strategy:
@Injectable()
export class UserProfileService {
constructor(@Inject(CACHE_MANAGER) private cacheManager: Cache) {}
async getUserProfile(userId: string): Promise<UserProfile> {
const cacheKey = `user_profile_${userId}`;
let userProfile = await this.cacheManager.get<UserProfile>(cacheKey);
if (!userProfile) {
userProfile = await this.getUserProfileFromDb(userId);
await this.cacheManager.set(cacheKey, userProfile, { ttl: 3600 });
}
return userProfile;
}
private async getUserProfileFromDb(userId: string): Promise<UserProfile> {
// Your database logic to fetch user profile
}
}
Implementing efficient caching strategies in your NestJS application has a substantial impact on performance by reducing database load and improving response times. In-memory caching with Redis is a powerful way to achieve this, ensuring that cached data is easily accessible. By adopting appropriate caching strategies such as Cache-Aside, Write-Through, and selecting suitable eviction policies, you can ensure your NestJS application performs optimally under varying load conditions.
Database transactions are critical for maintaining data integrity and ensuring that a series of operations either complete successfully or fail as a single unit, thus avoiding partial updates that can lead to data inconsistencies. NestJS, being a progressive Node.js framework, offers robust ways to handle database transactions efficiently. In this section, we will explore how to implement and manage database transactions in a NestJS environment using popular ORMs.
Before diving into the implementation, it's important to understand why transactions are necessary:
TypeORM is one of the most popular Object-Relational Mapping (ORM) tools used with NestJS. It provides a straightforward way to manage transactions. Let’s see how to implement a basic transaction in NestJS using TypeORM.
Install Dependencies: Make sure you have TypeORM and the database driver installed:
npm install typeorm mysql2
Create a Service: Add transaction logic in your service where the actual database operations occur.
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Connection, Repository } from 'typeorm';
import { User } from './user.entity';
import { Order } from './order.entity';
@Injectable()
export class UserService {
constructor(
@InjectRepository(User)
private readonly userRepository: Repository<User>,
@InjectRepository(Order)
private readonly orderRepository: Repository<Order>,
private readonly connection: Connection,
) {}
async createUserWithOrder(user: User, order: Order): Promise<void> {
await this.connection.transaction(async manager => {
await manager.save(User, user);
await manager.save(Order, order);
});
}
}
Handle Transactions Properly:
Use the transaction
method provided by TypeORM to ensure that either both user and order are saved, or neither is, maintaining database integrity.
async createUserWithOrder(user: User, order: Order): Promise<void> {
await this.connection.transaction(async manager => {
const createdUser = await manager.save(User, user);
order.userId = createdUser.id; // Linking the order to the user
await manager.save(Order, order);
});
}
Managing transactions efficiently is key to maintaining performance. Consider the following:
Minimize Transaction Scope: Only include the necessary operations in the transaction block to reduce lock contention.
Error Handling: Always catch errors within your transaction to ensure that proper rollbacks happen.
async createUserWithOrder(user: User, order: Order): Promise<void> {
await this.connection.transaction(async manager => {
try {
const createdUser = await manager.save(User, user);
order.userId = createdUser.id; // Linking the order to the user
await manager.save(Order, order);
} catch (err) {
// Handle error, e.g., logging
throw new Error('Transaction failed');
}
});
}
Isolation Levels: Adjust the isolation level according to the need of your application to balance between data consistency and system performance.
If you prefer Sequelize, another popular ORM, the approach is slightly different but follows similar principles.
Install Dependencies:
npm install sequelize sequelize-typescript mysql2
Implement Transaction in Service:
import { Injectable } from '@nestjs/common';
import { InjectModel } from '@nestjs/sequelize';
import { User } from './user.model';
import { Order } from './order.model';
import { Sequelize } from 'sequelize-typescript';
@Injectable()
export class UserService {
constructor(
@InjectModel(User)
private readonly userModel: typeof User,
@InjectModel(Order)
private readonly orderModel: typeof Order,
private readonly sequelize: Sequelize,
) {}
async createUserWithOrder(user: User, order: Order): Promise<void> {
const transaction = await this.sequelize.transaction();
try {
const createdUser = await this.userModel.create(user, { transaction });
order.userId = createdUser.id; // Linking the order to the user
await this.orderModel.create(order, { transaction });
await transaction.commit();
} catch (err) {
await transaction.rollback();
throw new Error('Transaction failed');
}
}
}
By properly implementing and managing database transactions in your NestJS application, you can ensure data integrity and consistency while also optimizing performance. Whether you choose TypeORM, Sequelize, or any other ORM, the principles remain the same—atomicity, consistency, isolation, and durability (ACID). Through these practices, you can maintain robust and efficient database operations in your NestJS applications.
The importance of asynchronous operations for database interactions in NestJS cannot be overstated. They play a crucial role in ensuring that your application remains non-blocking and responsive, especially when dealing with I/O-bound operations like database interactions. In this section, we will discuss why asynchronous operations are vital for performance in NestJS, and how to effectively implement async/await for database calls in your application.
Non-Blocking Operations: Asynchronous operations prevent your application from blocking the execution thread while waiting for I/O-bound tasks like database queries. This allows other operations to continue running concurrently, improving overall performance and scalability.
Simplicity and Readability: Using async/await
makes your code look synchronous, which simplifies error handling and increases code readability compared to traditional callback-based approaches or even Promises.
Enhanced Performance: By handling multiple database calls concurrently without blocking the event loop, your application can perform more efficiently under load, which is crucial for scaling.
NestJS, built on top of Node.js, naturally supports asynchronous programming paradigms. Here’s a guide to implementing async/await
for database interactions in a NestJS application:
First, ensure that you have a database module set up with a configured ORM (e.g., TypeORM, Sequelize). For instance, with TypeORM:
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { User } from './entities/user.entity';
import { UserService } from './user.service';
import { UserController } from './user.controller';
@Module({
imports: [TypeOrmModule.forFeature([User])],
providers: [UserService],
controllers: [UserController],
})
export class UserModule {}
Create service methods that utilize async/await
for database operations. Here's an example service with TypeORM:
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { User } from './entities/user.entity';
@Injectable()
export class UserService {
constructor(
@InjectRepository(User)
private userRepository: Repository<User>,
) {}
async findAll(): Promise<User[]> {
return await this.userRepository.find();
}
async create(user: User): Promise<User> {
return await this.userRepository.save(user);
}
}
Use the await
keyword to handle asynchronous calls in your controllers, ensuring that each endpoint can efficiently manage database interactions:
import { Controller, Get, Post, Body } from '@nestjs/common';
import { UserService } from './user.service';
import { User } from './entities/user.entity';
@Controller('users')
export class UserController {
constructor(private readonly userService: UserService) {}
@Get()
async findAll(): Promise<User[]> {
return await this.userService.findAll();
}
@Post()
async create(@Body() user: User): Promise<User> {
return await this.userService.create(user);
}
}
Keep in mind that async/await
can throw errors that need to be properly handled. You can use try/catch
blocks to manage errors gracefully:
async create(@Body() user: User): Promise<User> {
try {
return await this.userService.create(user);
} catch (error) {
throw new HttpException('Failed to create user', HttpStatus.BAD_REQUEST);
}
}
Using async/await
for database calls is not just a modern JavaScript best practice but a necessity for building performant and scalable NestJS applications. By ensuring non-blocking database operations, your application can handle larger loads more efficiently. As you delve further into optimizing your NestJS application, remember that combining these asynchronous practices with other performance-tuning strategies discussed in this guide will yield the best results.
Load testing is a critical step in ensuring that your NestJS application can handle high volumes of traffic and database interactions without compromising performance. By simulating real-world usage scenarios, load testing helps identify bottlenecks and areas for optimization, particularly in database operations. In this section, we will focus on using LoadForge to effectively perform load testing on your database interactions within a NestJS application.
Before diving into the specifics, it’s essential to understand why load testing is pivotal:
LoadForge is a powerful tool designed to test the performance of your web applications, including complex database interactions. Below are the steps to conduct load testing with LoadForge:
Sign Up: Sign up on the LoadForge website and set up a new test project.
Create a Load Test: Define the type of load test you want to run. You can use pre-defined templates or create custom scenarios to match your application's usage.
Configure a test scenario that reflects real-user interactions with the database. This can include:
LoadForge provides a user-friendly interface to set these parameters.
Using LoadForge, you can write test scripts that mimics the interactions your NestJS application has with the database. This can be done using LoadForge's scripting language or via its integrations. Below is an example script:
import { http } from 'loadforge';
export default async function () {
// Simulate a login request
let response = await http.get('https://your-nestjs-app/api/login?username=test&password=1234');
console.log(response.status); // Check for successful login
// Simulate fetching data from the database
response = await http.get('https://your-nestjs-app/api/posts');
console.log(response.json()); // Check for expected data
// Simulate posting new data
response = await http.post('https://your-nestjs-app/api/posts', {
title: 'New Post',
body: 'This is the content of the new post.'
});
console.log(response.status); // Check for successful post creation
}
Run the load test and monitor the results through the LoadForge dashboard.
Start the Test: Execute the load test by selecting the created scenario and specifying the parameters such as the number of virtual users, duration, and ramp-up time.
Monitor Real-Time Metrics: LoadForge provides real-time metrics including response times, error rates, and throughput. These metrics help in identifying how well your database is handling the load.
Analyze Results: Post-test, LoadForge generates detailed reports. Key metrics to look for include:
Use the insights gained from LoadForge tests to optimize your database operations:
Load testing with LoadForge provides valuable insights that allow you to debug, analyze, and enhance the efficiency of your database operations in a NestJS environment. By simulating real-world data loads, you can preemptively address performance issues, ensuring your application remains responsive and reliable. Remember, the key is not just running the tests but also iteratively refining your database interactions based on the data presented by LoadForge.
Effective monitoring and performance tuning are crucial for maintaining optimal database operations in your NestJS applications. This section delves into various tools and techniques that can help you analyze database performance, as well as offers practical tips for tuning both database settings and queries.
NestJS comes with a built-in logger that you can extend for monitoring purpose. Custom logging can help track slow queries and performance bottlenecks.
Example of custom logging:
import { Logger } from '@nestjs/common';
import { DataSource } from 'typeorm';
const logger = new Logger('DatabaseLogger');
const dataSource = new DataSource({
// other datasource options
logging: true,
logger: 'advanced-console',
maxQueryExecutionTime: 1000, // milliseconds
});
dataSource.initialize()
.then(() => logger.log('Data Source has been initialized!'))
.catch(err => logger.error('Error during Data Source initialization:', err));
For PostgreSQL databases, tools like pgAdmin offer a user-friendly interface to monitor query performance, track slow queries, and view system performance metrics.
PM2 is a powerful process manager for Node.js applications. It offers built-in monitoring capabilities:
$ pm2 start dist/main.js
$ pm2 monit
Application Performance Management (APM) tools such as New Relic, Datadog, or AppDynamics provide comprehensive performance monitoring, including detailed insights into database query performance.
SELECT *
: Specify only the required columns in your SELECT statements.Example:
import { Repository } from 'typeorm';
import { InjectRepository } from '@nestjs/typeorm';
import { User } from './user.entity';
export class UserService {
constructor(
@InjectRepository(User)
private userRepository: Repository<User>,
) {}
async findPaginated(page: number, limit: number): Promise<User[]> {
return this.userRepository.find({
take: limit,
skip: (page - 1) * limit,
});
}
}
Example configuration for TypeORM:
import { DataSource } from 'typeorm';
const dataSource = new DataSource({
// other datasource options
extra: {
max: 10, // maximum number of connections
min: 2, // minimum number of connections
},
});
Example for PostgreSQL:
ALTER SYSTEM SET work_mem = '16MB';
ALTER SYSTEM SET maintenance_work_mem = '64MB';
SELECT pg_reload_conf();
Identify Performance Bottlenecks:
Iterate and Tune:
Automated Testing:
By continuously monitoring and tuning your database operations, you will maintain efficient and high-performing NestJS applications.
## Handling Database Failover and Replication
Ensuring high availability and reliability in database operations is crucial for any production environment, especially for maintaining seamless user experiences and data integrity. Failover and replication strategies are vital components in achieving these goals. This section delves into the techniques for setting up database failover and replication in a NestJS application, ensuring that your application remains resilient and performs optimally even during database downtimes or failures.
### Understanding Failover and Replication
**Failover** refers to the automatic switching to a standby database server when the primary server fails, ensuring continuous availability.
**Replication** is the process of copying data from one database server to one or more standby servers, enhancing data availability, redundancy, and reliability.
### Setting up Failover
Failover techniques can be implemented to ensure your NestJS application seamlessly switches to a standby database in the event of a primary database failure. Here's a simplified example of setting up a failover configuration using PostgreSQL.
#### Steps to Implement Failover:
1. **Primary and Standby Configuration**:
Configure your primary and standby PostgreSQL servers.
2. **Monitoring and Promotion**:
Use an automatic monitoring tool like `repmgr` or `Patroni` to monitor the primary server and promote the standby to primary if the original primary fails.
3. **Database Connection Settings**:
Configure your NestJS application to connect to the cluster using a load balancer or a specialized connection string that handles failover.
Example configuration with a connection string:
<pre><code>DB_URL=postgresql://primary_host:5432,standby_host:5432/mydatabase</code></pre>
### Setting up Replication
Replication can be implemented to keep standby databases up-to-date with the primary database's state, ensuring data consistency and availability. Below is an example of setting up asynchronous replication for a PostgreSQL database.
#### Steps to Implement Replication:
1. **Primary Server Configuration**:
Modify the PostgreSQL configuration (`postgresql.conf`) to enable replication and specify necessary settings:
<pre><code># Enable replication
wal_level = replica
max_wal_senders = 3
wal_keep_segments = 64</code></pre>
2. **Standby Server Configuration**:
On the standby server, configure the PostgreSQL to act as a hot standby by editing `recovery.conf`:
<pre><code>standby_mode = 'on'
primary_conninfo = 'host=primary_host port=5432 user=replication password=yourpassword'</code></pre>
3. **Starting Replication**:
Restart both servers and verify the replication status using `pg_stat_replication`.
### Implementing Failover and Replication in NestJS
Integrating these configurations within a NestJS application requires some setup in your database connection settings.
#### Example:
```typescript
import { TypeOrmModule } from '@nestjs/typeorm';
@Module({
imports: [
TypeOrmModule.forRoot({
type: 'postgres',
url: process.env.DB_URL,
entities: [__dirname + '/**/*.entity{.ts,.js}'],
synchronize: true,
logging: true,
}),
],
})
export class AppModule {}
To ensure the failover and replication mechanisms work correctly, conduct thorough testing. Simulate primary server failures and observe if the connections switch seamlessly to the standby database. Validate that the data remains consistent across the primary and standby databases.
By implementing robust failover and replication strategies, you can significantly enhance the resilience and reliability of your NestJS application's database operations, ensuring seamless performance and data integrity even in the face of unexpected failures.
Efficient database operations are crucial for ensuring the performance and scalability of your NestJS applications. By focusing on optimizing various aspects of database interactions, you can significantly enhance the overall responsiveness and reliability of your system. Let's summarize the key points discussed in this guide and provide a checklist of best practices to maintain efficient database operations in your NestJS applications.
SELECT *
, and implementing pagination for handling large data sets.const connectionOptions = {
type: 'postgres',
host: 'localhost',
port: 5432,
username: 'test',
password: 'test',
database: 'test',
synchronize: true,
logging: false,
entities: [User],
extra: {
max: 10, // Maximum number of connections in the pool
min: 2, // Minimum number of connections in the pool
},
};
const connection = await createConnection(connectionOptions);
const users = await this.userRepository.find({
skip: (page - 1) * pageSize,
take: pageSize,
});
import * as redis from 'redis';
const client = redis.createClient();
client.set('key', 'value');
client.get('key', (err, value) => {
console.log(value);
});
await connection.transaction(async manager => {
await manager.save(user1);
await manager.save(user2);
});
const user = await this.userRepository.findOne(userId);
By following these best practices, you can significantly improve the efficiency and performance of your database operations within a NestJS application, leading to a more robust, responsive, and scalable system.