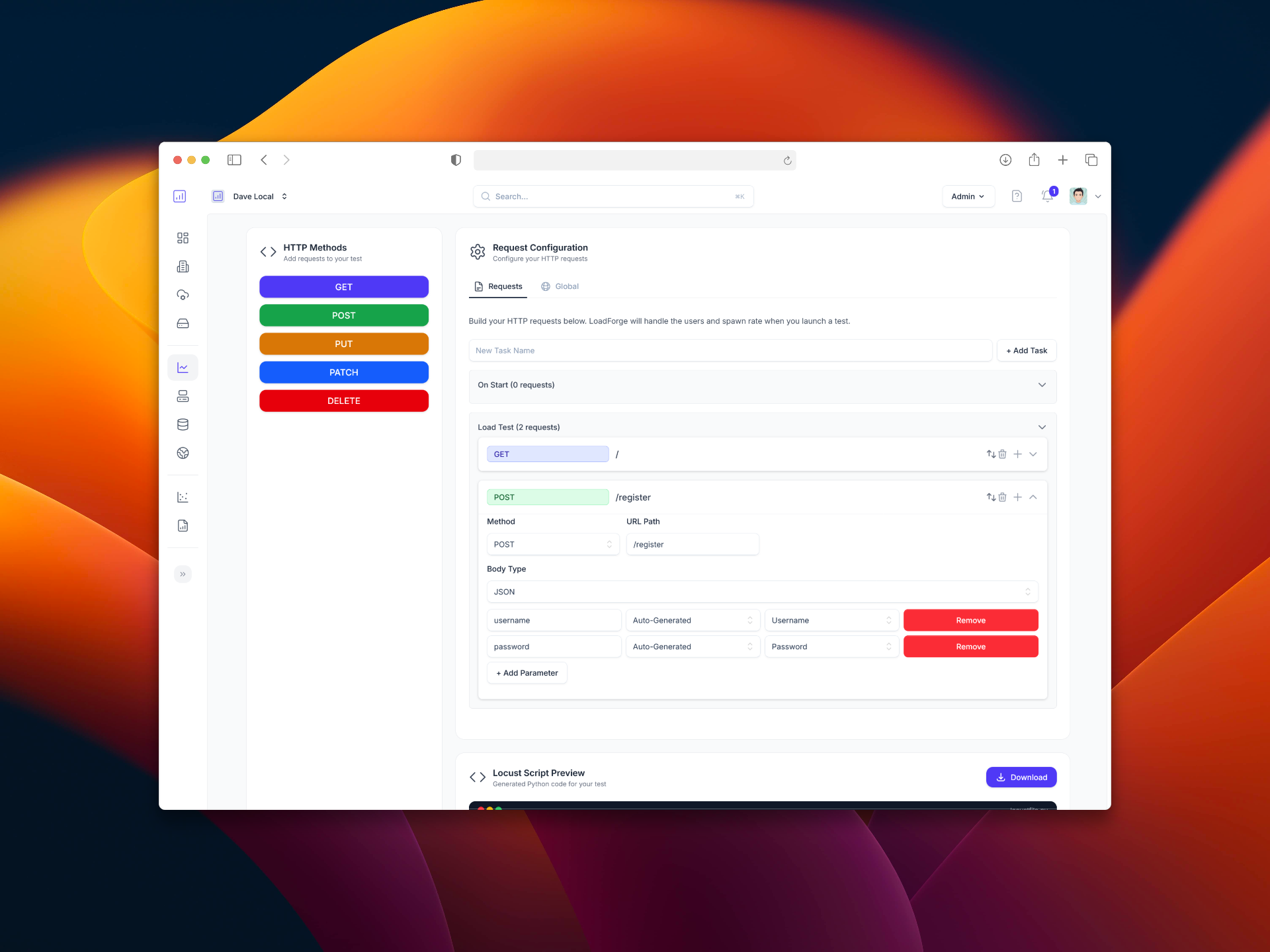
Jira Integration & Graphical Test Builder
We are proud to announce the release of two new features, available immediately. 🚀 Jira Integration: Automate Issue Tracking for...
In the digital age where instantaneous access to information has become the norm, web performance plays a critical role in user satisfaction and retention. For developers building Flask applications, one of the most effective methods to enhance performance is through...
In the digital age where instantaneous access to information has become the norm, web performance plays a critical role in user satisfaction and retention. For developers building Flask applications, one of the most effective methods to enhance performance is through caching. Caching can significantly reduce server load, decrease latency, and enable a seamless user experience.
But what exactly is caching and why is it so integral to web performance? In its simplest form, caching is the storage of copies of data in a temporary storage location (cache), so future requests for that data can be served faster. Instead of executing complex computations or fetching data from a slower database source every time a request is made, a cache serves the requested content directly from its quick-retrieval storage.
The importance of caching can be summarized in the following key points:
Flask, being a micro web framework written in Python, is known for its simplicity and flexibility. However, as your application grows, so does the need for efficient data retrieval methods to maintain performance. This is where caching comes into play. Effective caching strategies in Flask can lead to:
In this comprehensive guide, we will explore various caching strategies that can be employed to supercharge the performance of your Flask applications. From setting up Flask-Caching, implementing in-memory and HTTP caches, to advanced techniques like fragment caching and caching API responses, we will cover it all. Additionally, we'll delve into cache invalidation strategies, monitoring and analyzing cache performance using tools like LoadForge, and common pitfalls to avoid.
Together, we will unlock the full potential of caching to transform your Flask application into a high-performance, scalable web service. Let’s get started!
Caching is a crucial concept in web performance optimization, helping reduce latency, lighten server loads, and deliver faster user experiences. In the context of Flask applications, caching can be implemented at various layers, each serving distinct roles. This section delves into client-side, server-side, and reverse proxy caching—defining them and explaining their importance.
Client-side caching leverages the user's browser to store static resources such as HTML, CSS, JavaScript, and images. When users revisit a site, the browser can fetch these resources from its local cache instead of making a network request to the server.
Key Components:
Example of Setting Cache-Control Headers in Flask:
from flask import Flask, make_response
app = Flask(__name__)
@app.route('/')
def index():
response = make_response("Caching demonstration with Flask")
response.headers['Cache-Control'] = 'public, max-age=3600'
response.headers['ETag'] = 'unique-resource-identifier'
return response
Server-side caching involves storing responses on the server so that identical requests can be served more rapidly. This could include caching at the application level, using tools like Redis or Memcached, to store frequently accessed data and reduce the load on databases and other backend systems.
Key Components:
Example of Setting Up Flask-Caching:
from flask import Flask
from flask_caching import Cache
app = Flask(__name__)
cache = Cache(app, config={'CACHE_TYPE': 'simple'})
@app.route('/')
@cache.cached(timeout=50)
def cached_view():
return "This is a cached response"
Reverse proxy caching involves using a reverse proxy server to cache responses from backend servers. Tools such as Nginx, Varnish, or AWS CloudFront can be employed to cache responses, reducing latency and server load by serving cached responses for repeated requests.
Key Components:
Example Configuration for Nginx:
server {
listen 80;
server_name example.com;
location / {
proxy_pass http://127.0.0.1:5000;
proxy_cache my_cache;
proxy_cache_valid 200 1h;
}
}
proxy_cache_path /var/cache/nginx levels=1:2 keys_zone=my_cache:10m max_size=1g;
By understanding and properly implementing these caching strategies, you can significantly enhance the performance of your Flask applications, ensuring they are both robust and responsive. In the following sections, we'll delve into the practicalities of implementing these strategies within your Flask applications.
To begin optimizing your Flask application's performance with caching, you first need to set up Flask-Caching. This section covers installing the necessary packages and configuring Flask-Caching for your application.
The first step is to install Flask-Caching. This can be done using pip, the Python package installer. Open your terminal and run the following command:
pip install Flask-Caching
This will install Flask-Caching and its dependencies.
Once Flask-Caching is installed, you need to configure it in your Flask application. Here are the steps to set up Flask-Caching with basic configuration:
Cache
class and configure it with your Flask app.Here is an example of how to set this up:
from flask import Flask
from flask_caching import Cache
# Initialize Flask app
app = Flask(__name__)
# Define cache configuration settings
cache_config = {
'CACHE_TYPE': 'simple', # Simple in-memory cache
'CACHE_DEFAULT_TIMEOUT': 300 # Cache timeout in seconds
}
# Initialize the Cache with the configuration
cache = Cache(app, config=cache_config)
@app.route('/')
@cache.cached(timeout=50) # Cache this view for 50 seconds
def home():
return "Hello, World!"
if __name__ == '__main__':
app.run(debug=True)
First, import the Cache
class from the flask_caching
module:
from flask_caching import Cache
Initialize your Flask application as usual:
app = Flask(__name__)
Set up a configuration dictionary to define your cache settings. In this case, we're using a simple in-memory cache which is good for development and testing:
cache_config = {
'CACHE_TYPE': 'simple',
'CACHE_DEFAULT_TIMEOUT': 300
}
CACHE_TYPE
: The type of caching backend to use. Options include 'simple', 'redis', 'memcached', etc. Here, we use 'simple' for an in-memory cache.CACHE_DEFAULT_TIMEOUT
: Sets the default timeout for cached items in seconds.Create an instance of the Cache
class with the Flask app and the cache configuration:
cache = Cache(app, config=cache_config)
Use the @cache.cached(timeout=50)
decorator to cache the output of views. The timeout
parameter specifies how long the cache is valid (in seconds):
@app.route('/')
@cache.cached(timeout=50)
def home():
return "Hello, World!"
By following these steps, you have set up Flask-Caching in your application. This basic configuration uses an in-memory cache, suitable for development environments. As you move forward, you can use more powerful caching backends like Redis or Memcached to further enhance performance. Stay tuned for the next sections where we will cover in-memory caching with Redis and Memcached, among other caching strategies.
In-memory caching is a powerful technique to speed up your Flask application by temporarily storing frequently accessed data in a system's memory. This allows for faster data retrieval compared to querying a database or generating content dynamically. Tools like Memcached and Redis are popular choices for in-memory caching due to their performance and ease of use. In this section, we'll guide you through setting up and using in-memory caching with Flask-Caching.
Before getting started with in-memory caching, ensure you have Flask and Flask-Caching installed. If not, you can install them using pip:
pip install Flask Flask-Caching
Additionally, you will need either Redis or Memcached installed and running on your system. For Redis, you can install it via package managers like apt
, brew
, or choco
, or using Docker. Similarly, Memcached can be installed using package managers or Docker.
First, let's configure Flask-Caching to use Redis as the backend for our cache. Follow these steps to set it up:
Install Redis and Flask-Caching Redis Client:
pip install redis
Configure Flask-Caching with Redis:
Below is an example configuration for setting up Flask-Caching with Redis.
from flask import Flask
from flask_caching import Cache
app = Flask(__name__)
# Configure the cache
app.config['CACHE_TYPE'] = 'redis'
app.config['CACHE_REDIS_HOST'] = 'localhost'
app.config['CACHE_REDIS_PORT'] = 6379
app.config['CACHE_REDIS_DB'] = 0
app.config['CACHE_REDIS_URL'] = 'redis://localhost:6379/0'
cache = Cache(app)
@app.route('/')
@cache.cached(timeout=60)
def home():
return "Welcome to the cached Home Page!"
if __name__ == '__main__':
app.run(debug=True)
In the example above:
CACHE_TYPE
specifies the use of Redis.CACHE_REDIS_HOST
and CACHE_REDIS_PORT
set the hostname and port for the Redis server.CACHE_REDIS_DB
specifies the Redis database number.CACHE_REDIS_URL
provides a complete URL to the Redis server.Run your application:
python app.py
Now, let's configure Flask-Caching to use Memcached as the backend.
Install pymemcache:
pip install pymemcache
Configure Flask-Caching with Memcached:
Here's an example configuration for setting up Flask-Caching with Memcached.
from flask import Flask
from flask_caching import Cache
app = Flask(__name__)
# Configure the cache
app.config['CACHE_TYPE'] = 'memcached'
app.config['CACHE_MEMCACHED_SERVERS'] = ['127.0.0.1:11211']
cache = Cache(app)
@app.route('/')
@cache.cached(timeout=60)
def home():
return "Welcome to the Memcached Home Page!"
if __name__ == '__main__':
app.run(debug=True)
In the example above:
CACHE_TYPE
specifies the use of Memcached.CACHE_MEMCACHED_SERVERS
sets the list of Memcached servers.Run your application:
python app.py
timeout
parameter of the @cache.cached
decorator to define how long each cached result should be stored before expiration.In-memory caching can significantly enhance the performance of your Flask application by reducing the time needed to retrieve data. By properly configuring and managing your cache, you can ensure quick and efficient access to frequently requested information.
HTTP caching is a powerful technique to speed up your Flask application by reducing the load on the server and decreasing the latency experienced by end-users. By leveraging HTTP caching, you instruct browsers and intermediate proxies on how to store and reuse responses. This section will guide you through implementing HTTP caching in Flask using Cache-Control
headers and ETag
, and explain how these headers work to enhance web performance.
The Cache-Control
header is fundamental in managing HTTP caching. It provides directives to control how, and for how long, the response is cached. Here are some common directives:
You can set the Cache-Control
header in Flask by modifying the response object. Here’s an example of how to set this header:
from flask import Flask, make_response
app = Flask(__name__)
@app.route('/')
def index():
response = make_response("Hello, world!")
response.headers['Cache-Control'] = 'public, max-age=3600'
return response
if __name__ == '__main__':
app.run()
In this example, the Cache-Control
header instructs the browser to cache the response for 3600 seconds (1 hour).
ETag (Entity Tag) is another HTTP header used for caching. It provides a way to validate the cache, allowing browsers to check if the content has changed without downloading it again.
To implement ETags in Flask, you can use the etag
decorator:
from flask import Flask, make_response
app = Flask(__name__)
@app.route('/')
def index():
response = make_response("Hello, world!")
response.set_etag('unique-etag-value')
return response
if __name__ == '__main__':
app.run()
In a real-world scenario, you can generate an ETag based on the content's hash or a timestamp. Flask automatically handles the If-None-Match
header sent by the client and responds with a 304 Not Modified
status if the ETag matches, meaning the cached version is still valid.
Cache-Control
and ETag
headers.Cache-Control
header’s directives to determine if it can use the cached response.If-None-Match
header with the ETag value. The server compares it with the current ETag and, if it matches, responds with 304 Not Modified
, indicating that the cached version can be used.Here’s an example combining both Cache-Control
and ETag
for robust HTTP caching:
from flask import Flask, make_response
import hashlib
app = Flask(__name__)
def get_resource():
# Simulated resource content
return "Hello, world!"
@app.route('/')
def index():
resource = get_resource()
etag = hashlib.md5(resource.encode('utf-8')).hexdigest()
response = make_response(resource)
response.headers['Cache-Control'] = 'public, max-age=3600'
response.set_etag(etag)
if response.make_conditional(request):
response.status_code = 304
return response
if __name__ == '__main__':
app.run()
In this example, the etag
is calculated using the MD5 hash of the resource content, ensuring that any change to the content will generate a new ETag. The make_conditional
method helps handle the If-None-Match
header by setting the response status to 304 Not Modified
when appropriate.
Implementing these HTTP caching strategies in your Flask application will not only improve performance by reducing server load and latency but will also enhance the user experience by making your application more responsive.
Database query caching is a crucial strategy to reduce the load on your database and significantly improve the response times of your Flask application. By storing the results of expensive queries and reusing them for subsequent requests, you can avoid repeated database hits and enhance overall performance. In this section, we will explore how to implement database query caching with Flask-SQLAlchemy and Redis.
Before diving into caching, make sure you have Flask-SQLAlchemy and Redis installed in your Flask application. You can install these packages using pip:
pip install Flask-SQLAlchemy redis
First, configure Flask-SQLAlchemy and Redis in your Flask application:
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
from redis import Redis
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db'
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
db = SQLAlchemy(app)
redis_client = Redis(host='localhost', port=6379, db=0)
To cache the results of a database query, you need to fetch the cached data from Redis if it exists, otherwise, run the query and store the result in Redis. Here’s how you can achieve this:
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
def __repr__(self):
return f'<User {self.username}>'
@app.route('/users')
def get_users():
cache_key = 'all_users'
cached_users = redis_client.get(cache_key)
if cached_users:
users = json.loads(cached_users)
app.logger.info('Serving from cache')
else:
users = User.query.all()
users_json = [{'id': user.id, 'username': user.username, 'email': user.email} for user in users]
redis_client.setex(cache_key, 300, json.dumps(users_json)) # Cache for 5 minutes
app.logger.info('Serving from database')
return jsonify(users)
Properly invalidating cache is critical to ensure data consistency. Common strategies include:
setex
.Here's an example of how you could invalidate the cache manually when adding a new user:
@app.route('/add_user', methods=['POST'])
def add_user():
data = request.get_json()
new_user = User(username=data['username'], email=data['email'])
db.session.add(new_user)
db.session.commit()
# Invalidate cache
redis_client.delete('all_users')
return jsonify({'message': 'User added successfully!'})
Database query caching can lead to substantial performance gains for your Flask application by reducing the load on your database and improving response times. By leveraging Flask-SQLAlchemy and Redis, you can implement effective caching strategies to optimize your application. Remember to handle cache invalidation carefully to ensure data consistency and accuracy.
When building Flask applications, not all parts of a webpage change at the same frequency. For instance, a user profile page might have a frequently updated activity feed but a rarely changing user bio. By caching only certain parts of a view or template, known as fragment caching, you can strike an optimal balance between dynamic content and performance. This section explores how to implement fragment caching in Flask.
Fragment caching involves storing a portion of a webpage or template in the cache. This method is particularly useful for complex pages where only certain sections need to be updated regularly. By caching those immutable or infrequently changing sections, you can significantly reduce rendering times and server load.
To implement fragment caching in Flask, we'll use Flask-Caching, which is a powerful caching extension for Flask. We'll walk through a basic example where parts of a template are cached separately.
First, ensure you have Flask-Caching installed in your Flask project:
pip install Flask-Caching
Next, configure Flask-Caching in your Flask application:
from flask import Flask
from flask_caching import Cache
app = Flask(__name__)
# Use Redis as the cache backend
app.config['CACHE_TYPE'] = 'redis'
app.config['CACHE_REDIS_URL'] = 'redis://localhost:6379/0'
cache = Cache(app)
You can use the cache.cached
decorator to wrap specific functions or parts of your view logic to enable fragment caching. Here’s an example illustrating how to cache fragments:
from flask import render_template
@app.route('/user/<username>')
def user_profile(username):
user_info = get_user_info(username) # Assume this is a slow operation
activity_feed = get_activity_feed(username) # This is dynamic, don't cache
# Cache only the rendering of user info
user_info_html = cache.get_or_set(f'user_info_{username}', lambda: render_template('user_info.html', user=user_info), timeout=300)
return render_template('profile.html',
user_info=user_info_html,
activity_feed=activity_feed)
In the above example:
get_user_info()
fetches user data which changes infrequently. We cache its rendered HTML for 300 seconds.get_activity_feed()
fetches dynamic data which changes often, hence, we do not cache it.Sometimes, you may want to cache only parts of HTML templates. For this, you may create a custom template tag:
{% raw %}
{% cache key timeout %}
<!-- Rendered HTML goes here -->
{% endcache %}
{% endraw %}
<!-- Use it in a template -->
{% with cache_key='user_info_' + user.username %}
{% cache cache_key 300 %}
<div>
User bio: {{ user.bio }}
</div>
{% endcache %}
{% endwith %}
Fragment caching is a powerful tool for optimizing Flask applications. By judiciously applying caching to sections of your application, you can achieve responsive, high-performance web experiences without compromising on the freshness of dynamic content.
In this section, we'll delve into strategies for caching API responses in Flask applications to ease server load and enhance API response times. Given the frequent and dynamic nature of API requests, caching can significantly improve the scalability and performance of your Flask app. We'll explore using Flask-Caching
and CacheControl
for this purpose.
API endpoints are often the backbone of modern web applications, responsible for serving data to both front-end interfaces and other services. By caching API responses:
To start caching your API responses in Flask, you'll need to install the Flask-Caching
extension.
pip install Flask-Caching
Next, configure Flask-Caching
in your application:
from flask import Flask, jsonify
from flask_caching import Cache
app = Flask(__name__)
# Define cache configuration
cache = Cache(config={
'CACHE_TYPE': 'SimpleCache', # Options include 'MemcachedCache', 'RedisCache', etc.
'CACHE_DEFAULT_TIMEOUT': 300 # Default timeout in seconds
})
cache.init_app(app)
@app.route('/api/data')
@cache.cached(timeout=60)
def get_data():
# Assume this function retrieves data from a slow source
data = {
'message': 'Hello, World!',
'data': 'This is an example response'
}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
In this example:
Flask-Caching
with a simple in-memory cache.@cache.cached(timeout=60)
decorator caches the response of the get_data
function for 60 seconds.While Flask-Caching
handles in-app caching, CacheControl
is a great way to leverage HTTP caching by adding caching headers to your API responses. This directs clients (like browsers and services) to cache responses.
Install CacheControl
:
pip install CacheControl
Configure CacheControl
to manage caching behavior:
from flask import Flask, jsonify, make_response
from cachecontrol import CacheControl
app = Flask(__name__)
app = CacheControl(app)
@app.route('/api/data')
def get_data():
response = make_response(jsonify({
'message': 'Hello, World!',
'data': 'This is an example response'
}))
response.headers['Cache-Control'] = 'public, max-age=60'
return response
if __name__ == '__main__':
app.run(debug=True)
Here:
CacheControl
-wrapped Flask app.Cache-Control
header is set to public with a max-age
of 60 seconds, telling clients to cache the response for 60 seconds.For optimal performance, you may combine in-memory caching with HTTP caching:
from flask import Flask, jsonify, make_response
from flask_caching import Cache
from cachecontrol import CacheControl
app = Flask(__name__)
cache = Cache(config={'CACHE_TYPE': 'SimpleCache'})
cache.init_app(app)
app = CacheControl(app)
@app.route('/api/data')
@cache.cached(timeout=60)
def get_data():
response = make_response(jsonify({
'message': 'Hello, World!',
'data': 'This is an example response'
}))
response.headers['Cache-Control'] = 'public, max-age=60'
return response
if __name__ == '__main__':
app.run(debug=True)
In this setup:
Flask-Caching
caches the response within the application.CacheControl
instructs clients to cache the response, further reducing load on your server.Caching API responses effectively balances server load and response times, enhancing the user experience and making your Flask application more robust. By using Flask-Caching
for server-side caching and CacheControl
for client-side HTTP caching, you can ensure your API responds swiftly even under heavy load.
## Cache Invalidation Strategies
Effective caching can vastly improve the performance of your Flask application; however, it’s equally important to handle cache invalidation properly to ensure data freshness. Cache invalidation essentially means removing or updating cached data when it's no longer valid, becoming essential when dealing with frequently changing data. Let’s explore common cache invalidation strategies and how you can implement them in your Flask application.
### Time-Based Expiration
Time-based expiration, also known as time-to-live (TTL), involves setting an expiry time for cached data. Once this time is reached, the cache entry is invalidated and removed from the cache.
In Flask-Caching, you can easily set a TTL for your cached data:
```python
from flask import Flask
from flask_caching import Cache
app = Flask(__name__)
cache = Cache(app, config={'CACHE_TYPE': 'simple'})
@app.route('/data')
@cache.cached(timeout=60) # Cache this route for 60 seconds
def get_data():
data = fetch_expensive_data()
return data
def fetch_expensive_data():
# Simulated data-fetching function
return "Expensive Data"
In this example, the /data
endpoint's result is cached for 60 seconds. After the TTL, the cache entry expires, and a fresh value is fetched and cached again.
Sometimes, you need immediate invalidation of cached data, which can be achieved manually. This is useful when underlying data changes and you need to reflect those changes immediately.
Flask-Caching provides functions to manually delete specific cache entries:
@app.route('/update-data')
def update_data():
# Code to update your data
cache.delete('/data') # Manually invalidate the cache for the '/data' route
return 'Data Updated and Cache Cleared'
Above, the cache for the /data
route is cleared as soon as the data is updated.
Cache busting involves changing the cache key whenever the underlying data changes. This ensures that cached data is invalidated automatically when new data is available.
You can append a version number or timestamp to the key:
@app.route('/data')
@cache.cached(key_prefix='data', timeout=300)
def get_data():
version = get_data_version()
cache_key = f'data_{version}'
data = cache.get(cache_key)
if data is None:
data = fetch_expensive_data()
cache.set(cache_key, data, timeout=300)
return data
def get_data_version():
# Logic to get a version number or timestamp
# Example: return the last modified timestamp of your data source
return 'v1.0'
In this example, the cache key includes a version number that changes whenever the data changes, thus invalidating old cache entries automatically.
Flask has a signals framework that lets you trigger actions based on certain events. You can use signals to invalidate cache automatically when specific events occur:
from flask.signals import Namespace
my_signals = Namespace()
data_updated = my_signals.signal('data-updated')
def invalidate_cache_on_update(sender):
cache.delete('/data')
print('Cache invalidated due to data update')
data_updated.connect(invalidate_cache_on_update)
@app.route('/update-data')
def update_data():
# Code to update your data
data_updated.send()
return 'Data Updated and Cache Invalidated via Signal'
Implementing these cache invalidation strategies—time-based expiration, manual invalidation, cache busting, and using signals—ensures that your caching mechanism remains effective and your Flask application serves fresh data. Each strategy comes with its own use cases and can be combined to best fit your application's requirements.
## Monitoring and Analyzing Cache Performance
Effective caching strategies are essential for optimizing your Flask application's performance, but their success depends heavily on proper monitoring and analysis. Using a robust load testing and monitoring tool like LoadForge, you can comprehensively evaluate your caching strategy’s efficacy. Here’s how you can leverage LoadForge to ensure your caching mechanisms are making your application faster and more resilient under load.
### Why Monitor Cache Performance?
Monitoring your caching performance is critical because it helps in:
- **Identifying Bottlenecks:** Even with caching in place, there may still be bottlenecks hindering performance.
- **Ensuring Cache Hits:** Ensuring that your cache hit ratio is high and your cache misses are minimized.
- **Optimizing Resource Utilization:** Making sure that your resources are being used efficiently.
- **Detecting Expirable Caches:** Ensuring that the cache invalidation strategies work correctly and do not serve stale data.
### Using LoadForge for Performance Monitoring
LoadForge offers powerful tools to simulate traffic, stress-test your application, and monitor various performance metrics. Here’s a step-by-step guide to using LoadForge for monitoring and analyzing your Flask application's cache performance.
#### Step 1: Setting Up LoadForge
First, you need to set up an account on LoadForge and integrate it with your Flask application. Follow these basic steps:
1. **Create a LoadForge Account:** Sign up for an account at [LoadForge](https://www.loadforge.com).
2. **Generate an API Token:** Navigate to your account settings and generate an API token.
3. **Install LoadForge SDK:** Install the LoadForge SDK in your Flask application.
```bash
pip install loadforge
LoadForge allows you to define custom load scenarios to mimic real-world usage patterns. Create a scenario where the application utilizes its caching layers.
from loadforge import LoadTest, Scenario
scenario = Scenario("Basic Caching Test")
scenario.step("Home Page", "/home", method="GET")
test = LoadTest("Cache Performance Test")
test.add_scenario(scenario)
test.execute(api_key="YOUR_API_KEY")
During the test, pay close attention to the following metrics to analyze the effectiveness of your caching strategy:
After running your load tests, LoadForge provides detailed reports with actionable insights:
Here's a sample analysis snippet in code:
# code to analyze results
import loadforge
results = loadforge.get_results(test_id="YOUR_TEST_ID", api_key="YOUR_API_KEY")
print("Avg Response Time:", results.avg_response_time)
print("Cache Hit Ratio:", results.cache_hit_ratio)
print("Errors:", results.errors)
Caching strategies are not a one-time setup; they require continuous monitoring and tweaking. Schedule regular load tests with LoadForge to ensure your caching layers adapt to changing traffic patterns and data loads.
Utilizing LoadForge for monitoring and analyzing your caching performance helps ensure that your Flask application remains fast and scalable. By paying attention to key performance metrics, you can identify weaknesses, optimize resources, and maintain a robust and responsive application.
In the next section, we'll discuss Common Pitfalls and Best Practices to help you avoid common mistakes and follow best practices when implementing caching solutions.
When implementing caching solutions in your Flask applications, there are several common mistakes that developers often encounter. Understanding these pitfalls and following best practices can help ensure your caching strategy is both effective and efficient.
Over-Caching
@cache.cached(timeout=0) # Infinite timeout
def get_heavy_data():
# fetch heavy data processing
return data
Under-Caching
Improper Cache Invalidation
@cache.cached(timeout=300) # 5 minutes
def get_user_info(user_id):
# fetch user info
...
If user information updates frequently, such a timeout might be too long.Ignoring Cache Key Management
Excluding Cache in Development
Optimize Cache Expiration
@cache.cached(timeout=60) # 1 minute
def get_stock_prices():
# fetch the latest stock prices
return stock_prices
Use Versioned Cache Keys
cache_key = f"user_info_v2:{user_id}"
user_info = cache.get(cache_key)
if not user_info:
user_info = get_user_info_from_db(user_id)
cache.set(cache_key, user_info, timeout=300)
Leverage Cache Hierarchy
Monitor Cache Performance
# Use LoadForge to simulate and analyze your caching strategy
Ensure Data Freshness
# Example of manual cache invalidation
cache.delete(f"user_info:{user_id}")
Test in Production-like Environments
# Configure caching for both development and production
By understanding these common pitfalls and adhering to best practices, you can significantly improve the performance of your Flask applications. Caching, when implemented correctly, can help reduce server load, improve response times, and enhance the overall user experience.
Implementing an effective caching strategy is paramount for optimizing the performance of Flask applications. Throughout this guide, we explored various caching techniques, from fundamental concepts to detailed implementation strategies, and discussed how they can significantly enhance your application's responsiveness and efficiency.
Introduction to Caching:
Understanding Caching:
Setting Up Flask Cache:
In-Memory Caching with Flask-Caching:
HTTP Caching with Flask:
Cache-Control
headers and ETag
.Database Query Caching:
Fragment Caching:
Caching API Responses:
Cache Invalidation Strategies:
Monitoring and Analyzing Cache Performance:
Common Pitfalls and Best Practices:
Effective caching is not merely an optional optimization—it's a necessity for any scalable and high-performing Flask application. By carefully implementing and configuring caching mechanisms, you can:
Remember, a well-thought-out caching strategy is a continuous process of monitoring, adjusting, and refining to adapt to changing data patterns and user loads. Utilizing tools like LoadForge for load testing and performance analysis can provide valuable insights and help fine-tune your caching strategy for maximum impact.
Taking the time to implement these strategies will undoubtedly pay off, making your Flask applications faster, more reliable, and capable of handling increased demand effortlessly.