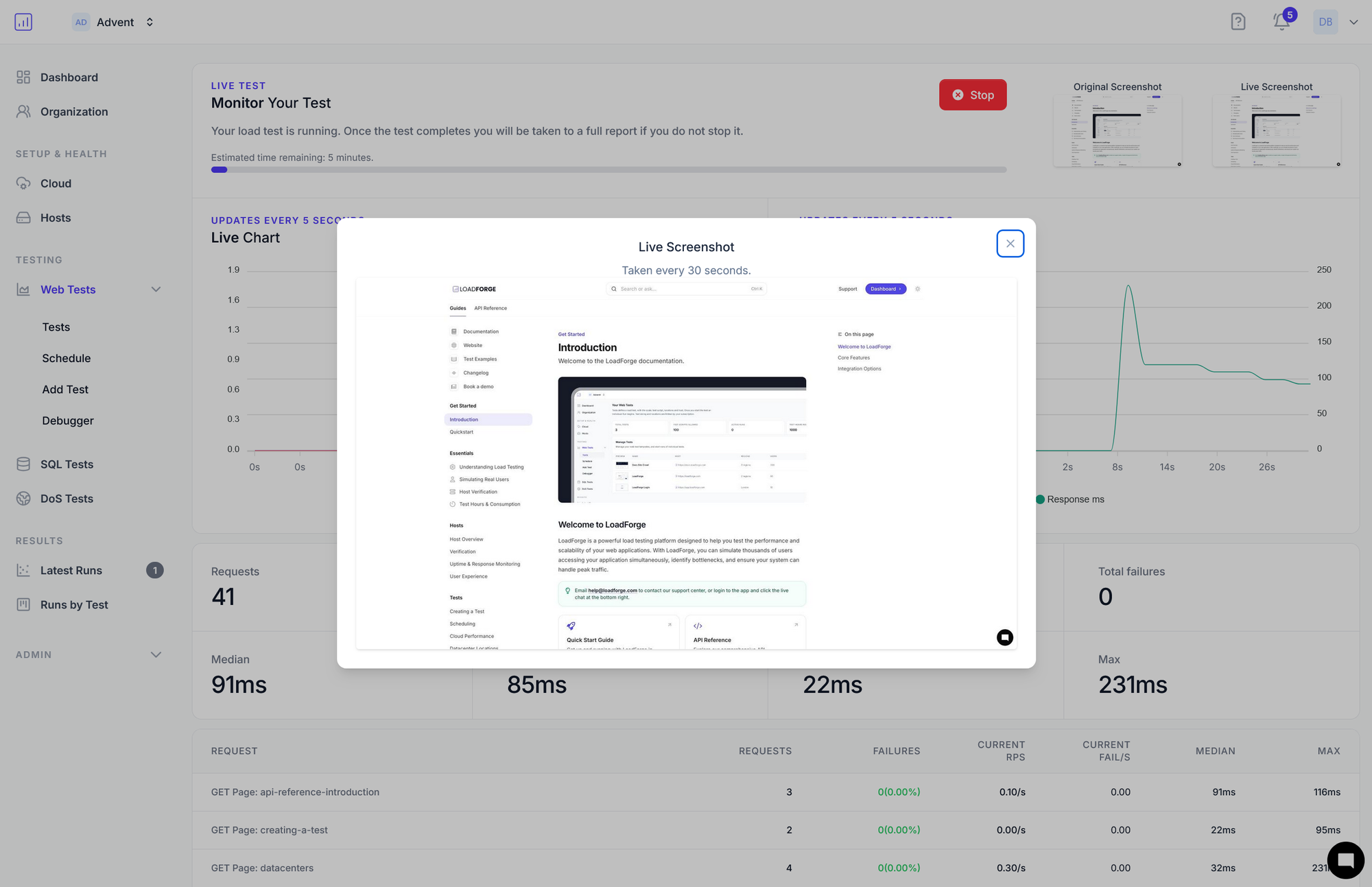
Faster Launches, Better Screenshots
Sometimes it's the small things that make the load testing experience better! We've rolled out an update that lets you...
Wait times, iterations per second, and other ways to control request rates in LoadForge.
You are now browsing the LoadForge locust test directory. You can use these tests as a starting point for your own tests, or use our AI wizard to generate one automatically.
There are various ways to control the requests per second, iterations, and user load in LoadForge.
Naturally, the primary way to control the request rate is with the number of Virtual Users you specify. This guide will focus on additional control beyond that.
Typical LoadForge scripts include a wait_time
parameter. Examples of this are below:
from locust import HttpUser, task, between, constant
class QuickstartUser(HttpUser):
wait_time = between(5, 9)
# or
wait_time = constant(0.5)
@task(3)
def index_page(self):
self.client.get("/")
wait_time
is designed to simulate a real browser, where users wait a random amount of time between requests due
to them consuming the content on the page.
For the maximum possible request rate (RPS) do not specify a wait time and the script will loop as fast as possible.
You should also consider FastHttpUser if doing that, for a significant improvement to the request rate while sacrificing some of the complex test abilities.
The constant_total_ips
helper will ensure that the total number of requests per second is constant, regardless of the number of users.
This is useful when you want to ensure a constant load on your server, regardless of the number of users.
This will not work with the wait_time
attribute, as it will override it. Also note, IPS is iterations per second, and
means your full test script.
from locust import HttpUser, task
from locust_plugins import constant_total_ips
class MyUser(HttpUser):
wait_time = constant_total_ips(5)
# the below task has two requests, meaning
# our rps will be around 10 per second (due to 5 IPS above)
@task
def t(self):
self.client.get("/")
self.client.get("/contact-us")