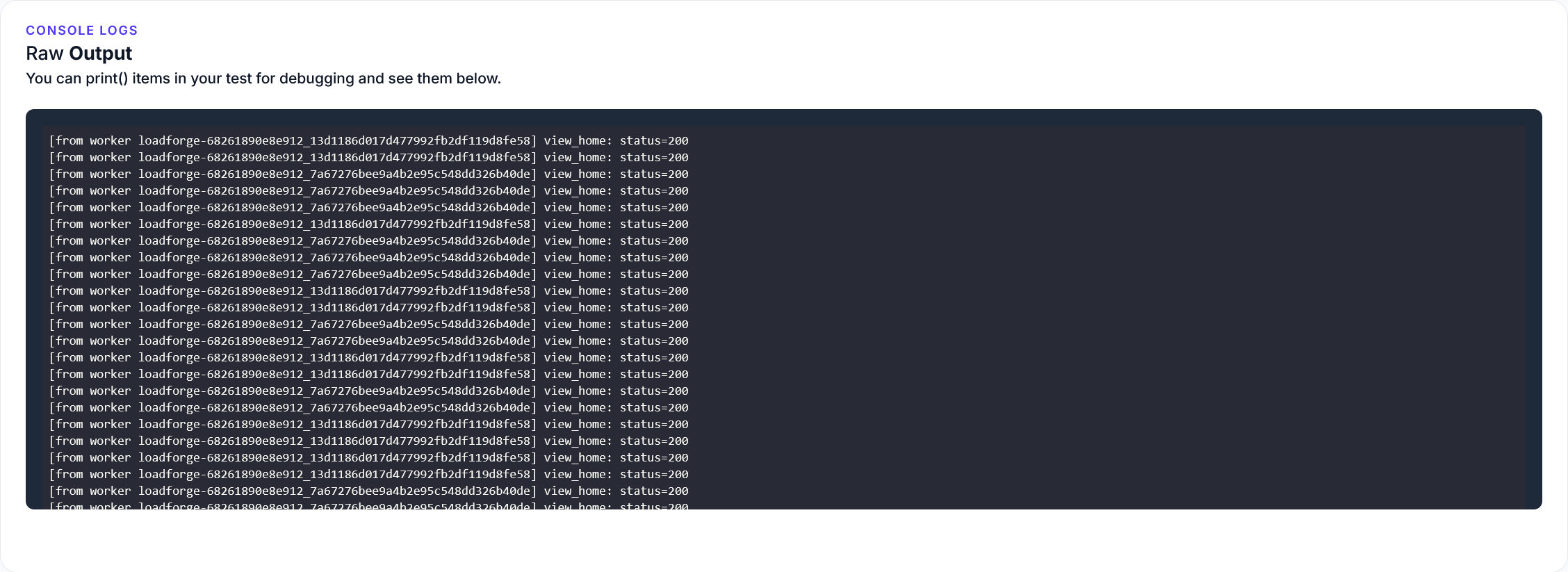
Easier Debug Logging
We're excited to announce a powerful new feature for LoadForge users: comprehensive debug logging for test runs. This highly requested...
## Introduction When it comes to building robust, high-performance web applications with Symfony, optimizing for efficiency is paramount. This is where Symfony's built-in profiler comes into play. The Symfony Profiler is an indispensable tool for developers aiming to gain deep...
When it comes to building robust, high-performance web applications with Symfony, optimizing for efficiency is paramount. This is where Symfony's built-in profiler comes into play. The Symfony Profiler is an indispensable tool for developers aiming to gain deep insights into the performance characteristics of their applications. It provides a detailed examination of various aspects, from HTTP requests and response times to database queries and Twig template rendering.
The Symfony Profiler is a powerful diagnostic tool integrated into the Symfony framework. It collects a wealth of data about each request made to your application, presenting it in an easy-to-navigate web interface. This tool is designed to help developers identify performance bottlenecks, debug errors, and optimize application behavior with precision. In essence, it serves as a real-time X-ray for your Symfony application, illuminating areas of improvement that might otherwise go unnoticed.
Here are some compelling reasons why leveraging the Symfony Profiler is crucial for performance optimization:
By utilizing the Symfony Profiler, you'll be able to systematically approach performance tuning and debugging, leading to a faster and more reliable application.
This guide takes you through the essential steps and best practices for using Symfony's built-in profiler to optimize your application's performance. Specifically, you will learn:
With these skills, you will be well-equipped to transform the performance and reliability of your Symfony-based applications, ensuring they can handle increased load and provide a seamless user experience.
In the following sections, we will dive deep into each of these topics, offering practical advice, code snippets, and actionable tips to make the most out of Symfony's built-in profiler.
## Setting Up Symfony Profiler
The Symfony Profiler is an essential tool for developers aiming to enhance their application's performance. Initially designed to track and debug Symfony applications, it provides an intuitive interface and detailed insights that are crucial for performance tuning. In this section, we will guide you through the steps to enable and configure the Symfony Profiler in your development environment, ensuring you have access to the necessary diagnostic tools.
### Prerequisites
Before diving into the setup, make sure your environment meets the following prerequisites:
- Symfony 4.4 or higher
- Composer
### Enabling the Symfony Profiler
Symfony Profiler is already included as part of the Symfony Debug Bundle, which comes pre-installed in the standard Symfony distribution. Typically, it is enabled by default in the development environment. However, if it's not present or if you need to verify the configuration, follow these steps:
1. **Add the Debug Bundle**: Ensure that the Symfony Debug Bundle is added to your `composer.json` file. You can do this by running the following Composer command:
```sh
composer require --dev symfony/profiler-pack
```
2. **Enable the Profiler**: Verify that the profiler is enabled in your development environment. Open your `config/packages/dev/web_profiler.yaml` and `config/packages/prod/web_profiler.yaml` files and ensure they contain the following configuration:
<pre><code>
web_profiler:
toolbar: true
intercept_redirects: false
# Uncomment these lines if you wish to enable the profiler in production (not recommended)
# web_profiler:
# toolbar: false
# intercept_redirects: false
</code></pre>
### Configuring the Web Debug Toolbar
The Web Debug Toolbar is a small panel that appears at the bottom of your browser when accessing your Symfony application in the development environment. It provides quick access to key performance metrics.
1. **Toolbar Configuration**: Open the same `web_profiler.yaml` file, and ensure the toolbar is enabled:
<pre><code>
web_profiler:
toolbar: true
</code></pre>
2. **Interception of Redirects**: For debugging purposes, you may want the profiler to intercept redirects:
<pre><code>
web_profiler:
intercept_redirects: true
</code></pre>
Enabling this allows you to inspect requests before they are redirected, which can be particularly useful for debugging complex flows and performance issues.
### Accessing the Profiler Interface
Once you have set up and configured the Symfony Profiler, access it by following these steps:
1. Start your Symfony server:
```sh
symfony server:start
Navigate to your application in the browser. You should see the Web Debug Toolbar at the bottom of the page. Click on it to access the full Symfony Profiler interface:
http://localhost:8000
Alternatively, you can access the Profiler dashboard directly via:
http://localhost:8000/_profiler/
By following these steps, you will have successfully enabled and configured the Symfony Profiler. You are now ready to dive into the next stages of performance analysis and optimization using this powerful tool.
Next, we will explore how to navigate the Profiler interface to make the most of the insights it offers.
This concludes the setup section, making sure your development environment is primed for detailed performance analysis using Symfony's built-in profiler.
## Navigating the Profiler Interface
The Symfony Profiler is an indispensable tool for debugging and optimizing your Symfony applications. Once you have the Profiler set up, the next step is understanding how to navigate its interface effectively to extract valuable insights. This section provides a detailed walkthrough of the key sections you’ll encounter in the Symfony Profiler, making it easier to pinpoint performance bottlenecks and other issues.
### Request Overview
When you open the Symfony Profiler for a specific request, you are greeted with a comprehensive overview of that request. This page is designed to give you a bird's-eye view of various metrics and data points:
- **HTTP Method and Status Code:** Displays the HTTP method (GET, POST, etc.) and the HTTP status code returned.
- **Controller and Route:** Shows the controller and route responsible for handling the request.
- **Execution Time:** Provides the total time taken to process the request.
- **Memory Usage:** Indicates the peak memory used to handle the request.
Here’s an example of what this summary might look like:
<pre><code>
Method: GET
Status Code: 200 OK
Controller: App\Controller\DefaultController::index
Route: index
Execution Time: 120ms
Memory Usage: 5MB
</code></pre>
### Timeline
The timeline section is particularly useful for understanding how time is distributed across various operations during request handling. This includes Symfony’s core processes as well as your application-specific tasks. The timeline is visualized as a Gantt chart, showing start and end times for different operations, allowing you to identify time-consuming steps at a glance.
Key blocks in the timeline might include:
- **Kernel events**
- **Listeners**
- **Controller execution**
- **Twig rendering**
By examining the timeline, you can identify stages that might need optimization, such as a controller action taking longer than expected or Twig's template rendering being slower than usual.
### Performance Metrics
Performance metrics give you a deeper understanding of your application’s behavior during request handling. Key metrics include:
- **Execution Time:** The total time taken to process a request.
- **Memory Usage:** The peak memory usage during the request.
- **Cache Hits/Misses:** Provides insight into your application’s caching effectiveness.
- **Doctrine Queries:** Number of database queries run during the request.
These metrics are crucial for spotting inefficiencies, such as abnormally high memory usage or an excessive number of database queries.
### Database Queries
The database queries section lists all the SQL queries executed during the handling of a request. Each query is detailed with:
- **SQL Statement:** The exact SQL query executed.
- **Execution Time:** Time taken to execute each query.
- **Parameters:** Parameters bound to the query.
This allows you to identify slow-performing queries and provides a starting point for optimization. For example:
<pre><code>
SELECT * FROM users WHERE id = ?
Parameters: [1]
Execution Time: 10ms
</code></pre>
### Viewing Timeline and Queries
Pay special attention to the timeline and query count. A typical optimization strategy involves aligning heavy SQL operations and intense PHP computations to a minimum. If your timeline shows prolonged database interaction, it may serve as a clear indicator that your SQL queries need optimization or you should implement additional caching strategies.
### Putting It All Together
As you become more familiar with the Symfony Profiler interface:
1. **Inspect the Request Overview:** Start by checking basic metrics to catch obvious red flags.
2. **Analyze the Timeline:** Use the Gantt chart to pinpoint operations consuming the most time.
3. **Review Performance Metrics:** Look for any unusual patterns or metrics that deviate from the norm.
4. **Examine Database Queries:** Dive deep into each query’s execution time and look for ways to optimize.
Understanding these key sections and how to navigate them will empower you to optimize your Symfony application effectively, leading to faster response times and a more efficient system overall.
## Analyzing HTTP Requests
In this section, we'll delve into how Symfony's built-in Profiler allows you to thoroughly inspect and analyze HTTP requests. This process is vital for identifying performance bottlenecks, understanding request headers, analyzing response times, and ultimately enhancing the efficiency of your Symfony application.
### Understanding the Request Overview
When you navigate to the Symfony Profiler, you'll immediately notice the **Request** panel. This panel provides a comprehensive overview of every HTTP request made to your application. Key elements you'll find here include:
- **Request and Response Information**: Detailed data about the request URL, method (GET, POST, etc.), status code, and response content type.
- **Headers**: All request and response headers, which can be crucial for debugging and performance insights.
- **Session Attributes**: Information about the session state, which can be important for stateful applications.
- **Cookies**: Both incoming and outgoing cookies are listed here, helping you to troubleshoot cookie-related issues.
### Inspecting Headers
Headers carry essential metadata about each HTTP request and response. To access headers in the Symfony Profiler:
1. **Open the Request Panel**: Click on an individual request in the profiler to view its details.
2. **Headers Tab**: Navigate to the Headers tab, where you will see both **Request Headers** and **Response Headers**.
Inspect these headers to ensure that no unnecessary data is being sent and to verify that security headers, such as `Content-Security-Policy` and `Strict-Transport-Security`, are correctly configured.
### Analyzing Response Times
Response times are a critical aspect of web performance. The Symfony Profiler provides detailed timing information for each request:
- **Total Time**: The total time taken to process the request from start to finish.
- **Timeline View**: A graphical representation of the request lifecycle, broken down into segments such as **controller execution**, **template rendering**, **database queries**, and more.
#### Example: Finding Bottlenecks
In the Timeline view, you can identify which part of the request lifecycle is consuming the most time. For instance, if you notice that controller execution is taking an unusually long time, you may need to optimize the logic within that controller.
### Identifying Potential Bottlenecks
To identify potential bottlenecks, focus on the following:
- **Long Running Queries**: Quickly identify if database queries are taking too long.
- **Expensive Controller Actions**: Pinpoint controller actions that are more time-consuming to process.
- **Template Rendering**: Determine if any templates are particularly slow to render.
#### Example Bottleneck Analysis
Imagine a situation where a request takes 800ms, but the **controller execution** alone takes 600ms. By drilling down into the controller, you might find that a specific service call or data processing operation is the bottleneck.
```php
// Example of inefficient data processing
public function indexAction()
{
$data = $this->getDataFromService();
foreach ($data as &$item) {
$item = $this->expensiveFunction($item);
}
return $this->render('index.html.twig', ['data' => $data]);
}
In this example, expensiveFunction
might be the source of inefficiency. Profiling can help identify and target such issues.
By leveraging the Symfony Profiler's robust capabilities to inspect HTTP requests, you can gain clear, actionable insights that drive targeted performance enhancements throughout your application.
In the next section, we'll explore how to further optimize performance by identifying and speeding up slow database queries using the Symfony Profiler.
Effective database query optimization is crucial for enhancing the performance of your Symfony application. The Symfony Profiler provides detailed insights that can help you identify slow queries and resolve performance bottlenecks. In this section, we'll explore how to leverage the profiler for optimizing your database queries, along with practical tips for indexing and caching.
The Symfony Profiler displays a thorough breakdown of the database queries executed during a request. You can access this information by navigating to the "Doctrine" section in the profiler interface. Here, you'll find:
To identify slow queries:
Once you've identified slow queries, you can start optimizing them using the following methods:
Indexes significantly speed up query execution by allowing the database to quickly locate the required rows without scanning the entire table.
Adding an Index in Doctrine To add an index to a field in your Doctrine entity, modify the entity mapping as follows:
/**
* @ORM\Entity
* @ORM\Table(name="example_table", indexes={@ORM\Index(name="search_idx", columns={"search_field"})})
*/
class ExampleEntity
{
// ...
}
Run the following command to update your database schema:
php bin/console doctrine:schema:update --force
Query caching can drastically reduce the time needed to retrieve data by storing the results of expensive queries.
Enabling Query Caching
To enable caching in Doctrine, you can configure your cache provider in the config/packages/doctrine.yaml
file:
doctrine:
orm:
metadata_cache_driver:
type: pool
pool: doctrine.system_cache_pool
query_cache_driver:
type: pool
pool: doctrine.result_cache_pool
second_level_cache:
enabled: true
region_lifetime: 3600
JOIN FETCH
) instead of lazy loading to reduce the number of database calls.Instead of:
$articles = $entityManager->getRepository(Article::class)->findAll();
foreach ($articles as $article) {
echo $article->getAuthor()->getName();
}
Use eager loading:
$articles = $entityManager->createQuery('SELECT a FROM App\Entity\Article a JOIN FETCH a.author')->getResult();
foreach ($articles as $article) {
echo $article->getAuthor()->getName();
}
By effectively utilizing the database query insights provided by the Symfony Profiler, you can significantly improve your application's performance. Focus on identifying slow queries, applying indexing appropriately, and leveraging query caching. Remember, optimization is an iterative process; continually monitor and refine your database interactions for sustained performance gains.
Maintaining an efficient Symfony application goes beyond just fast database queries and optimized templates. Monitoring the overall execution time of your application is crucial to understanding its performance. The Symfony Profiler offers a wealth of information on PHP execution, service container performance, and the utilization of auto-wired services, which can help you pinpoint bottlenecks and improve the responsiveness of your application.
The PHP execution timeline is a critical metric captured by the Symfony Profiler. This timeline offers a granular view of how long each part of the application takes to process. Here's how you can monitor and interpret PHP execution times using Symfony Profiler:
Access the Timeline Panel: Navigate to the Timeline panel in the profiler. This panel provides a breakdown of execution times for different components.
Analyze Execution Phases: Look at the segments of the timeline, such as controller execution, middleware processing, and event listeners. This breakdown helps you identify the areas causing delays.
Example Code Inspection: When you spot a performance issue, drill down into the corresponding parts of your code. For instance, if a controller action is slow, inspect it closely:
public function myAction(Request $request)
{
// Initial processing
sleep(2); // Intentional delay for example
// Further Code
}
Optimize Code: Rewrite inefficient code segments, ensuring that you're making the most of PHP's capabilities and minimizing unnecessary computations.
Symfony's service container is pivotal in managing dependencies. However, misconfigurations and overuse of services can impair performance. The Symfony Profiler provides insights into the service container's behavior:
View Service Load Times: In the profiler, check the "Service Container" panel to see how long it takes to load each service.
Identify Heavy Services: Pay attention to services with unusually high load times. These might be services performing resource-intensive tasks or poorly configured Singleton services.
Optimize Service Definitions: Reduce the load time by refining how services are defined:
services:
App\Service\HeavyService:
arguments:
- '%some_parameter%'
lazy: true # Lazy load to improve performance
Symfony's auto-wiring feature simplifies dependency injection but can sometimes obscure performance costs. Monitoring auto-wired services helps ensure they don’t become performance liabilities. Follow these steps:
Inspect Auto-Wiring: Use the profiler to examine the auto-wired services. Look into the "Service Container" section to identify which services have been auto-wired.
Evaluate Creation Time: Assess the instantiation times for these services and identify any that take a significant amount of time to instantiate.
Decide on Manual Wiring: For complex services that slow down application performance, consider switching from auto-wiring to manual wiring for finer control.
services:
App\Service\ComplexService:
class: App\Service\ComplexService
arguments:
- '@App\Repository\SomeRepository'
- '@App\Service\AnotherService'
Monitoring the overall execution time of your Symfony application with the built-in profiler is indispensable for maintaining high performance. By examining PHP execution, service container performance, and auto-wired services, you can identify inefficiencies and optimize your application effectively. In the subsequent sections, we'll delve into more specific strategies for performance optimization, but with a thorough understanding of your application's execution, you'll have a strong foundation to build on.
Effective caching is a cornerstone of web application performance optimization. It reduces the load on the database, decreases page load times, and generally improves the user experience on your website. In this section, we'll delve into the importance of caching, and explore how to analyze cache hit ratios and strategies using the Symfony Profiler.
Caching is crucial because it allows frequently accessed data to be stored in a readily retrievable format, reducing the need to fetch or compute the data repeatedly. Here's why caching matters:
Symfony Profiler provides a detailed view of cache operations, helping you fine-tune your caching strategy. Here's how you can leverage it:
To analyze cache usage, you need to navigate to the "Cache" panel within the Symfony Profiler. This panel provides insights into cache operations during the lifecycle of a request.
Cache Calls:
Efficiency Ratios:
In the Symfony Profiler, the "Cache" panel displays these metrics in an accessible format. Here’s how you can interpret them:
+--------------------+--------------------+ | Metric | Description | +--------------------+--------------------+ | Cache Hits | 120 | | Cache Misses | 30 | | Cache Writes | 50 | | Cache Deletes | 10 | | Hit Ratio | 80% (120/150) | | Write to Hit Ratio | 41.67% (50/120) | +--------------------+--------------------+
- **High Hit Ratio**: Indicates that most requested data is found in the cache, which is optimal.
- **Low Hit Ratio**: Suggests either infrequent access patterns or insufficient cache size/lifetime.
- **High Write to Hit Ratio**: Might indicate that unnecessary data is being cached or that the cache is being invalidated too frequently.
### Strategies for Improving Cache Performance
1. **Optimize Cache Keys**: Use efficient keys to ensure data can be quickly retrieved.
2. **Tune Cache Expiry**: Balance between data freshness and cache hit rates. Too frequent invalidation leads to cache misses.
3. **Indexing**: Make sure that frequently accessed data is easily identifiable through appropriate indexing.
4. **Layered Caching**: Implement different layers of caching (e.g. in-memory cache like Redis, file-based cache) to optimize performance further.
### Example: Configuring Cache in Symfony
To set up and fine-tune caching in Symfony, modify the `cache.yaml` configuration file:
<pre><code>
# config/packages/cache.yaml
framework:
cache:
default_redis_provider: redis://localhost
pools:
my_cache_pool:
adapter: cache.adapter.redis
default_lifetime: 3600
</code></pre>
This configuration sets up a Redis cache pool with a default lifetime of 3600 seconds. You can adjust these values according to your application’s needs.
### Conclusion
Understanding and optimizing cache usage is pivotal for enhancing your application's performance. Using the Symfony Profiler, you can gain valuable insights into cache operations and fine-tune your caching strategies for optimal results. By monitoring key metrics and applying best practices for cache management, you’ll be able to significantly improve the speed and efficiency of your Symfony-based applications.
## Profiling Twig Templates
Twig, the flexible, fast, and secure template engine for PHP, is at the heart of Symfony's view layer. While Twig is designed for high performance, it’s crucial to ensure your templates are optimized, especially as your application grows. In this section, we'll dive into using Symfony's built-in profiler to identify rendering bottlenecks in your Twig templates and suggest optimizations to boost your template performance.
### Understanding the Twig Profile Panel
Symfony Profiler comes with a dedicated Twig panel that provides detailed insights into your template rendering processes. Here’s a breakdown of what you can find in the Twig panel:
- **Template Calls**: Lists all the templates that were called during the request.
- **Block Calls**: Shows the rendered blocks within templates.
- **Macro Calls**: Displays macros that were invoked.
- **Rendering Time**: Highlights the time each template took to render, which is crucial for spotting bottlenecks.
### Accessing the Twig Panel
To navigate to the Twig panel:
1. Open your Symfony Profiler by clicking on the Symfony icon in the web debug toolbar.
2. Select the "Twig" section from the toolbar at the top.
### Identifying Rendering Bottlenecks
The primary goal of profiling Twig templates is to identify the parts of your templates that are slow to render. Here's how you can use the profiler to spot these bottlenecks:
1. **Examine Rendering Times**:
Look for templates with high rendering times. These are the candidates for optimization.
<pre><code>
{% if render_time > 100 %}
<div class="alert alert-warning">
Alert: Rendering of this template took longer than expected.
</div>
{% endif %}
</code></pre>
2. **Nested Template Calls**:
Check for deeply nested template includes, which can significantly increase rendering time.
<pre><code>
{# Multiple nested includes can slow down performance #}
{% include 'header.html.twig' %}
{% include 'sidebar.html.twig' %}
{% include 'footer.html.twig' %}
</code></pre>
3. **Review Block Usage**:
Ensure that you're not over-using blocks, which can add overhead.
### Optimizing Twig Templates
Once you’ve identified the slow parts of your Twig templates, the next step is to optimize them. Here are some practical tips:
1. **Optimize Template Inheritance**:
Use template inheritance efficiently to minimize the rendering time of nested templates.
<pre><code>
{# base.html.twig #}
<html>
<head>{# head content #}</head>
<body>
{% block body %}{% endblock %}
</body>
</html>
{# child.html.twig #}
{% extends 'base.html.twig' %}
{% block body %}
{# child-specific content #}
{% endblock %}
</code></pre>
2. **Leverage TWIG Cache**:
Enable caching for frequently reused fragments to reduce repetitive rendering.
<pre><code>
{% cache 'my_cache_key' %}
{# Cached content #}
{% endcache %}
</code></pre>
3. **Minimize Function Calls**:
Avoid extensive use of functions within your templates as they can degrade performance.
<pre><code>
{# Avoid complex operations within templates #}
{# Use a controller or service to perform logic and pass the result to your view #}
</code></pre>
4. **Reduce Include Calls**:
Where possible, avoid multiple `include` calls by consolidating template snippets.
<pre><code>
{# Consolidate small template parts into a single template where appropriate #}
</code></pre>
### Example: Profiling and Optimizing a Slow Template
Let’s consider a practical example of profiling and optimizing a slow-rendering template.
1. **Profiling the Template**:
The profiler shows that `slow_template.html.twig` takes a notable amount of time to render due to multiple nested includes and heavy logic within the template itself.
2. **Optimization Steps**:
- **Consolidate Includes**: Combine smaller components into fewer template files.
- **Move Logic to Controller**: Ensure that any data processing is handled in the controller or a service, rather than directly in the template.
<pre><code>
{# Before Optimization: slow_template.html.twig #}
{% include 'header.html.twig' %}
{% include 'data_processing.html.twig' %}
{% include 'footer.html.twig' %}
{# After Optimization: optimized_template.html.twig #}
{# Data passed from the controller #}
{% include 'layout.html.twig' %}
</code></pre>
By efficiently utilizing Symfony’s Twig profiler panel, you can pinpoint and resolve performance bottlenecks in your templates, ensuring swift and smooth rendering for your users. In the next section, we will delve into adding custom metrics and logs to Symfony Profiler to further fine-tune your application's performance.
## Custom Metrics and Logging
As you delve deeper into optimizing your Symfony application, you might find that the built-in metrics provided by the Symfony Profiler don't fully cover all the performance aspects specific to your application. In such cases, adding custom metrics and logs becomes essential for a more granular performance analysis tailored to your needs. This section will guide you through the process of creating custom metrics and logging them within the Symfony Profiler.
### Adding Custom Metrics
Symfony allows you to add custom data collectors to gather the specific metrics you want to monitor. Follow these steps to create and integrate a custom data collector:
1. **Create a Data Collector Class**:
First, create a new class that extends `Symfony\Component\HttpKernel\DataCollector\DataCollector`. Override the necessary methods to collect and present your custom metric.
```php
namespace App\DataCollector;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\HttpKernel\DataCollector\DataCollector;
class CustomMetricCollector extends DataCollector
{
private $metricData;
public function __construct()
{
$this->metricData = [];
}
public function collect(Request $request, Response $response, \Throwable $exception = null)
{
// Collect the necessary metric data
$this->metricData = [
'custom_metric' => rand(1, 100), // Example metric
];
}
public function getMetricData()
{
return $this->metricData;
}
public function reset()
{
$this->metricData = [];
}
public function getName(): string
{
return 'app.custom_metric_collector';
}
}
Register the Data Collector as a Service:
Define your data collector as a service in your services.yaml
file and tag it with data_collector
.
services:
App\DataCollector\CustomMetricCollector:
tags:
- { name: data_collector, template: '@App/Collector/custom_metric.html.twig', id: 'app.custom_metric_collector' }
Create a Twig Template: Create a Twig template to render your custom metric data in the Profiler.
{# templates/Collector/custom_metric.html.twig #}
{% extends '@WebProfiler/Profiler/layout.html.twig' %}
{% block toolbar %}
{% set custom_metric = collector.metricData['custom_metric'] %}
<div class="sf-toolbar-info-piece">
<b>Custom Metric</b>
<span>{{ custom_metric }}</span>
</div>
{% endblock %}
{% block panel %}
<h2>Custom Metrics</h2>
<div class="metrics">
<div class="metric">
<span class="value">{{ custom_metric }}</span>
<span class="label">Custom Metric</span>
</div>
</div>
{% endblock %}
Inspect Your Custom Metrics: With everything set up, you can now inspect your new custom metrics in the Symfony Profiler under the "Custom Metrics" section.
Logging custom messages or specific data points can provide deeper insights and context for your application’s performance issues. Symfony uses the Monolog library for logging, which can be easily extended for your custom needs.
Configure Monolog:
Add a configuration for your custom log channel in config/packages/monolog.yaml
.
monolog:
channels: ['custom']
handlers:
custom:
type: stream
path: '%kernel.logs_dir%/%kernel.environment%.custom.log'
level: debug
channels: ['custom']
Log Custom Data: Inject the Monolog logger service into your services or controllers and log custom data points.
namespace App\Controller;
use Psr\Log\LoggerInterface;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\Routing\Annotation\Route;
class CustomLoggingController extends AbstractController
{
private $logger;
public function __construct(LoggerInterface $customLogger)
{
$this->logger = $customLogger;
}
/**
* @Route("/custom-log", name="custom_log")
*/
public function logCustomData(): Response
{
$this->logger->info('Custom metric value', ['metric' => rand(1, 100)]);
return new Response('Custom log entry created');
}
}
By integrating custom metrics and logging into your Symfony application, you can tailor the performance data collection to your exact needs, enabling more precise tuning and optimization. Utilize these tools to gain deeper insights into application-specific performance issues and to create targeted strategies for resolving them.
In combination with built-in metrics such as those provided by Symfony Profiler, custom metrics give you a full spectrum view of your application's performance, allowing you to keep it running efficiently even under load.
This section provides a clear and comprehensive guide on how to add custom metrics and logs to Symfony Profiler, enhancing the ability to perform granular performance analysis tailored to your application's needs. By following the provided steps and code examples, developers can gain deeper insights and optimize their applications more effectively.
## Using LoadForge for Load Testing
Performance profiling and optimization go hand in hand with load testing, as the latter reveals how your application behaves under various levels of stress. While Symfony's built-in profiler provides in-depth insights into how your application performs at an individual request level, LoadForge enables you to simulate realistic traffic, helping to identify performance limits and potential bottlenecks in your application.
In this section, we'll demonstrate how to use LoadForge in conjunction with Symfony Profiler to achieve comprehensive performance analysis and optimization.
### Why Load Testing is Crucial
Load testing goes beyond regular functional testing by simulating load scenarios - such as peak traffic times, concurrent user access, and extended usage periods - to ensure your application remains robust under stress. Without load testing, you might overlook critical performance issues that only emerge under significant traffic.
### Step-by-Step Guide to Using LoadForge
Follow these steps to set up and execute load testing on your Symfony application using LoadForge:
### Step 1: Register for LoadForge
If you haven't already, sign up for a LoadForge account at [LoadForge's website](https://loadforge.com). Choose a plan that suits your needs and access your dashboard.
### Step 2: Create a New Load Test
1. **Initiate Load Test**: From your LoadForge dashboard, click "New Load Test" to start configuring your test.
2. **Name Your Test**: Provide a descriptive name for your load test for easy identification.
3. **Set Environment Variables**: Add any environment variables your Symfony application might need during the testing phase.
### Step 3: Define Your Load Test Parameters
1. **Set the Target URL**: Input the base URL of your Symfony application that you want to test.
2. **Define Requests**: Specify the HTTP requests, including endpoints, methods, headers, and payloads you wish to simulate. This can mimic user interactions with your application.
<pre><code>
GET /homepage
POST /login {username: "testuser", password: "password"}
GET /dashboard
</code></pre>
3. **Concurrency and Duration**: Configure the number of concurrent virtual users and the duration of the test. For example:
- **Concurrency**: 100 users
- **Duration**: 5 minutes
This setup simulates 100 users using your application simultaneously for a sustained period.
### Step 4: Execute the Load Test
1. **Run the Test**: Click on the "Run Test" button to start the load test. LoadForge will gradually ramp up the traffic to reach the specified concurrency levels.
2. **Monitor Progress**: Observe real-time metrics that LoadForge provides during the test execution, such as response times, error rates, and server resource utilization.
### Step 5: Analyze Load Test Results
Once the test is completed, LoadForge will generate detailed reports highlighting key performance indicators:
- **Response Times**: Evaluate the average, median, and 95th percentile response times. This helps you understand how your application performs under load.
- **Error Rates**: Identify the proportion of failed requests, crucial for detecting and addressing reliability issues.
- **Resource Utilization**: Look into CPU, memory, and disk I/O usage to ensure your server infrastructure is not a limiting factor.
### Step 6: Correlate with Symfony Profiler Data
With the load test report in hand, revisit the Symfony Profiler to drill down into specific performance issues:
- **Database Queries**: Check for slow queries that appear frequently during load testing.
- **HTTP Requests**: Analyze which requests are taking the longest and might be causing bottlenecks.
- **Service Performance**: Review slow services or auto-wired components that may need optimization.
By correlating load test data with the granular insights from Symfony Profiler, you can target specific areas for performance improvements.
### Conclusion
Integrating load testing with LoadForge into your performance optimization strategy ensures a holistic understanding of how your Symfony application performs under real-world conditions. Regular load testing, complemented by Symfony Profiler's detailed insights, equips you to proactively address performance bottlenecks, ultimately leading to a more robust and scalable application.
Next, we’ll discuss best practices and additional tips to further refine your performance optimization efforts.
## Best Practices and Tips
Performance optimization with Symfony Profiler can significantly enhance your application's responsiveness and scalability. Here, we compile best practices, additional tips, and common pitfalls to avoid:
### Best Practices
1. **Regular Monitoring**: Regularly use the profiler to monitor your application's performance, especially after major changes or deployments. This helps catch regressions early.
2. **Prioritize Bottlenecks**: Focus on high-priority bottlenecks first—identify critical paths that users frequently traverse. Reduce TTFB (Time to First Byte) for a faster overall experience.
3. **Keep It Clean**: Clean up unused services, parameters, and routes. An uncluttered service container results in faster dependency resolution.
4. **Database Indexing**: Use the profiler to spot slow queries and ensure that relevant fields are indexed. Proper indexing can dramatically reduce query times.
5. **Cache Wisely**: Implement efficient caching strategies. Ensure key components like templates and API responses are cached appropriately. Analyze cache hit ratios to refine the strategy.
6. **Optimize Twig Templates**: Use Twig's built-in extensions to pass only the necessary data to views. Simplify loops and conditionals where possible to reduce rendering time.
7. **Keep Dependencies Up-to-date**: Regularly update Symfony and its dependencies. Each release brings performance improvements and bug fixes that you can leverage.
### Additional Tips
- **Use Debug Mode Strategically**: While developing, run your Symfony application in debug mode to access the profiler. However, disable it in production as it can significantly slow down your application.
- **Custom Data Collection**: Use the `DataCollector` component to add custom metrics that are specific to your application. This offers granular insights tailored to your business logic.
```php
namespace App\DataCollector;
use Symfony\Component\HttpKernel\DataCollector\DataCollector;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\Response;
class MyCustomCollector extends DataCollector
{
public function collect(Request $request, Response $response, \Exception $exception = null)
{
$this->data = ['custom_metric' => 'value'];
}
public function getCustomMetric()
{
return $this->data['custom_metric'];
}
public function getName()
{
return 'my_custom_collector';
}
}
Profile in Staging: Before going live, always profile your application in a staging environment that mirrors your production setup. This helps in identifying environment-specific issues.
Automated Load Testing: Integrate automated load testing using LoadForge as part of your deployment pipeline. This helps in understanding how your application behaves under stress and scale.
# Example LoadForge configuration
url: https://yourapp.test
duration: 5m
users: 100
Neglecting Smaller Issues: Large performance gains are often achieved by addressing multiple smaller issues. Do not ignore minor slowdowns; they add up.
Overlooking Error Handling: Proper error handling is crucial for performance. Non-handled errors can significantly slow down your application especially under load.
Excessive Logging: Be mindful of logging levels in production; excessive logging can degrade performance. Use appropriate log levels and filter out non-critical logs.
Ineffective Caching: Over-caching or incorrect caching configurations can result in stale data or even poor performance. Ensure your caching strategy is balanced and aligns with your application’s usage patterns.
By adhering to these best practices and tips, leveraging the Symfony Profiler effectively, and avoiding common pitfalls, you can ensure that your Symfony application remains performant and responsive under varying loads. This is crucial for providing a seamless user experience and maintaining operational efficiency.
Throughout this guide, we've delved into the rich functionalities of Symfony's built-in profiler and how it can be leveraged to gain deep performance insights into your application. Here's a brief recap of the essential points covered:
Introduction to Symfony Profiler: We started with an overview of what the Symfony Profiler is, its significance in performance optimization, and the valuable insights you can derive from using it.
Setting Up Symfony Profiler: We provided step-by-step guidance on enabling and configuring the Symfony Profiler in your development environment, ensuring that you have the necessary dependencies and a proper setup to begin performance analysis.
Navigating the Profiler Interface: We walked through the main sections of the Symfony Profiler interface, explaining how to use tools such as request overview, timeline, performance metrics, and database queries to gather performance data.
Analyzing HTTP Requests: This section focused on inspecting and analyzing HTTP requests, shedding light on headers, response times, and identifying potential bottlenecks.
Database Query Optimization: Leveraging the profiler to identify and optimize slow database queries was the crux of this segment, where we discussed indexing, query caching, and other optimization techniques.
Monitoring Application Execution: We explored how to monitor overall application execution time, including PHP execution, service container performance, and auto-wired services.
Evaluating Cache Usage: The importance of caching in performance optimization was highlighted, along with methods to analyze cache hit ratios and strategies using Symfony Profiler.
Profiling Twig Templates: This section provided insights into profiling Twig templates, identifying rendering bottlenecks, and presenting optimizations for template performance.
Custom Metrics and Logging: We discussed adding custom metrics and logs to Symfony Profiler for more granular performance analysis tailored to specific application needs.
Using LoadForge for Load Testing: Emphasizing the importance of load testing, we offered a step-by-step guide on using LoadForge to simulate traffic and identify performance limits, making it clear how load testing complements profiling.
Best Practices and Tips: Finally, we compiled a set of best practices, additional tips, and common pitfalls to avoid when using Symfony Profiler for performance optimization.
Remember, performance tuning is not a one-time activity; it's a continuous process that evolves with your application. Regularly profiling your Symfony application helps catch performance regressions early, ensures that optimizations remain effective, and adapts to changes in user behavior and data patterns.
By integrating Symfony Profiler into your routine development and maintenance workflows, and coupling it with robust load testing using tools like LoadForge, you can enhance the performance, reliability, and scalability of your application. Keep iterating on the metrics, stay vigilant for bottlenecks, and be proactive in applying optimizations.
With these tools and practices in your toolkit, you'll be well-equipped to maintain a high-performing Symfony application that delights users and meets the demands of modern web applications.