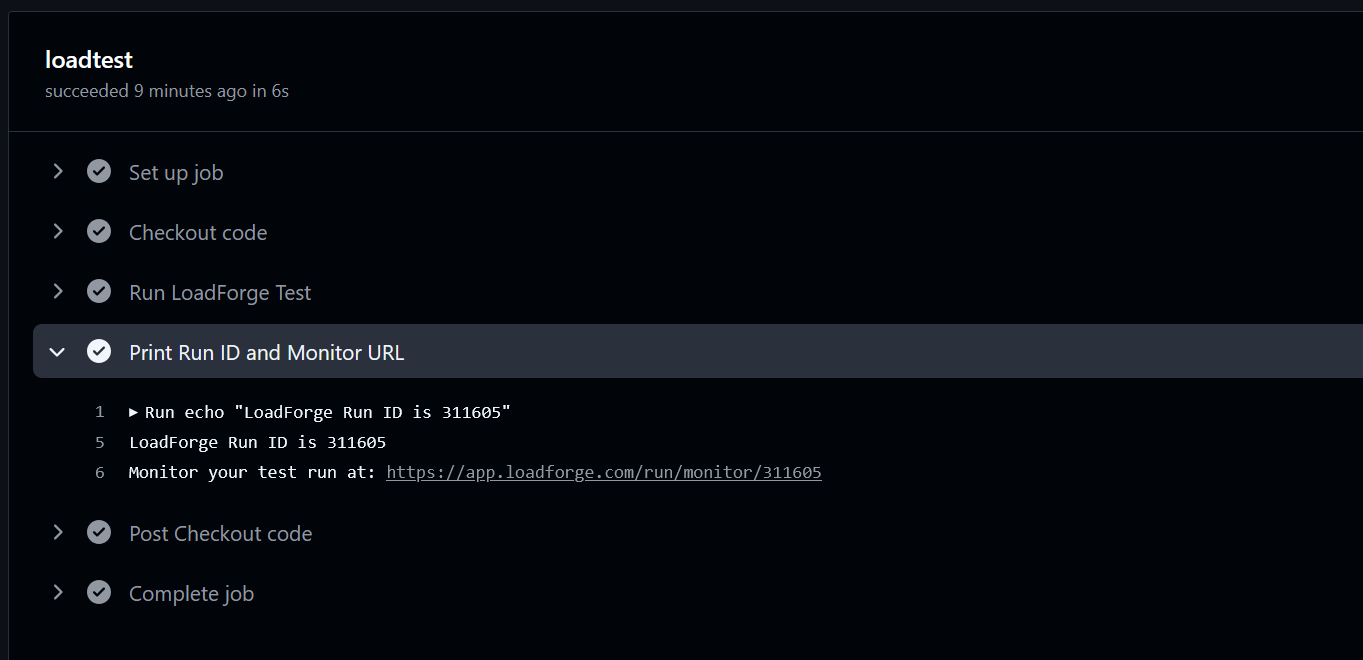
LoadForge GitHub Integration
Performance testing just got a major upgrade. LoadForge is thrilled to announce a seamless GitHub integration that lets you launch...
NestJS is a progressive Node.js framework that's designed to build scalable and efficient server-side applications. It leverages TypeScript to provide a strongly-typed and modular architecture, making it ideal for creating robust, maintainable applications by following best practices like Dependency Injection...
NestJS is a progressive Node.js framework that's designed to build scalable and efficient server-side applications. It leverages TypeScript to provide a strongly-typed and modular architecture, making it ideal for creating robust, maintainable applications by following best practices like Dependency Injection (DI) and decorators.
Server performance is critical for delivering a smooth and responsive experience to end-users. Slow or unreliable servers can lead to high bounce rates, user dissatisfaction, and ultimately, a loss of revenue. Some key benefits of optimizing server performance include:
LoadForge is a powerful load testing platform that helps developers identify and address performance bottlenecks in their applications. By simulating real-world traffic and conditions, LoadForge allows you to:
Integrating LoadForge with your NestJS applications enables you to effectively gauge performance and scalability. With a few simple steps, you can set up a load testing scenario tailored to your application’s needs. Here's a brief overview of how LoadForge can be utilized:
Here's a simple example to demonstrate a basic NestJS controller that could be a target for load testing with LoadForge.
import { Controller, Get } from '@nestjs/common';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
getHello(): string {
return this.appService.getHello();
}
}
The getHello
endpoint can be load tested to measure how it performs under various conditions. By using LoadForge, you can simulate multiple concurrent requests to this endpoint and analyze its behavior under stress.
Understanding the basics of NestJS and the importance of server performance sets the stage for effectively using LoadForge to load test your applications. In the following sections, we will delve deeper into setting up your NestJS project, identifying performance bottlenecks, and integrating LoadForge for comprehensive load testing. By the end of this guide, you'll be equipped with the knowledge and tools to optimize your NestJS server for peak performance and scalability.
Before diving into optimizing and load testing, it is crucial to start with a solid foundation by setting up your NestJS application properly. This section will guide you through the process of installing the NestJS CLI, creating a new project, and setting up the initial project structure.
The NestJS Command Line Interface (CLI) is a powerful tool for quickly generating NestJS projects and various artifacts such as modules, controllers, and services. You can install the CLI globally using npm by running the following command:
npm install -g @nestjs/cli
To verify the installation, you can check the version of the NestJS CLI:
nest --version
Once you have the NestJS CLI installed, you can create a new project using the nest new
command followed by your project's name. For example, to create a project named nestjs-app
, you would run:
nest new nestjs-app
The CLI will prompt you to choose a package manager (npm or yarn). Select your preferred package manager, and the CLI will scaffold the new project and install the necessary dependencies.
After creating your project, navigate into the newly created project directory:
cd nestjs-app
The initial project structure includes several key directories and files:
nestjs-app/
├── src/
│ ├── app.controller.ts
│ ├── app.controller.spec.ts
│ ├── app.module.ts
│ ├── app.service.ts
│ └── main.ts
├── test/
├── .eslintrc.js
├── .prettierrc
├── nest-cli.json
├── package.json
├── README.md
├── tsconfig.build.json
└── tsconfig.json
To verify that everything is set up correctly, you can run your NestJS application using:
npm run start
By default, the application will run on http://localhost:3000. You can open your web browser and navigate to this URL to see the basic "Hello World!" response served by your application.
With your NestJS application set up, you are now ready to move on to understanding performance bottlenecks and preparing your server for load testing. In the next sections, we will dive deeper into these topics to ensure your application is robust and scalable.
To ensure your NestJS application performs optimally under load, it's crucial to understand the common performance bottlenecks that can negatively impact your server's efficiency. This section will help you identify and address these bottlenecks, focusing on synchronous operations, improper database queries, and blocking event loop issues.
Synchronous code execution in an otherwise asynchronous environment can significantly degrade your server's performance. When the Node.js event loop encounters synchronous code, it pauses all other operations until the synchronous task completes, leading to potential delays and reduced throughput.
Common Synchronous Operations:
Example:
import { Controller, Get } from '@nestjs/common';
import * as fs from 'fs';
@Controller('synchronous')
export class SynchronousController {
@Get()
handleRequest(): string {
// Synchronous file read operation
const data = fs.readFileSync('largefile.txt', 'utf8');
return data;
}
}
Solution: Replace synchronous code with its asynchronous counterparts wherever possible.
Optimized Example:
import { Controller, Get } from '@nestjs/common';
import { promises as fs } from 'fs';
@Controller('synchronous')
export class SynchronousController {
@Get()
async handleRequest(): Promise<string> {
// Asynchronous file read operation
const data = await fs.readFile('largefile.txt', 'utf8');
return data;
}
}
Inefficient database queries can be a major source of performance problems. Issues such as N+1 query problems, unindexed table scans, and large data set retrievals can significantly slow down your server.
Common Database Query Issues:
Example (N+1 Query Problem):
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { User } from './user.entity';
import { Post } from './post.entity';
@Injectable()
export class UserService {
constructor(
@InjectRepository(User)
private userRepository: Repository<User>,
) {}
async getUsersWithPosts(): Promise<User[]> {
const users = await this.userRepository.find();
for (const user of users) {
user.posts = await this.userRepository
.createQueryBuilder('user')
.leftJoinAndSelect('user.posts', 'post')
.where('user.id = :id', { id: user.id })
.getMany();
}
return users;
}
}
Solution: Use eager loading or join queries to reduce the number of database calls.
Optimized Example:
@Injectable()
export class UserService {
constructor(
@InjectRepository(User)
private userRepository: Repository<User>,
) {}
async getUsersWithPosts(): Promise<User[]> {
return await this.userRepository.find({ relations: ['posts'] });
}
}
The Node.js event loop can handle many requests concurrently, but its non-blocking nature can be compromised by long-running or blocking operations, leading to performance degradation.
Common Blocking Operations:
Example:
import { Controller, Get } from '@nestjs/common';
@Controller('blocking')
export class BlockingController {
@Get()
handleRequest(): string {
// Blocking the event loop with a long-running computation
const start = Date.now();
while (Date.now() - start < 5000) {
// Simulate heavy computation
}
return 'Done';
}
}
Solution: Offload heavy computations to worker threads or use libraries that support non-blocking operations.
Optimized Example:
import { Controller, Get } from '@nestjs/common';
import { Worker, isMainThread, parentPort } from 'worker_threads';
@Controller('blocking')
export class BlockingController {
@Get()
handleRequest(): Promise<string> {
return new Promise((resolve, reject) => {
const worker = new Worker(__filename);
worker.on('message', resolve);
worker.on('error', reject);
worker.postMessage('start');
});
}
}
if (!isMainThread) {
parentPort?.on('message', () => {
setTimeout(() => {
parentPort?.postMessage('Done');
}, 5000);
});
}
Identifying and mitigating these common bottlenecks will set a strong foundation for your NestJS server's performance. In the following sections, we'll dive deeper into load testing scenarios to ensure your application scales efficiently.
## Load Testing Basics
In this section, we'll explore the fundamental concepts of load testing, why it's a critical component of developing scalable and high-performing applications, and the key metrics to monitor during load testing.
### What is Load Testing?
Load testing is a type of performance testing that simulates real-user traffic to your application in a controlled environment. The primary goal of load testing is to understand an application's behavior under specific load conditions, such as varying user counts, request rates, and workloads. It helps identify performance bottlenecks, system capacity, and whether the current infrastructure can handle expected traffic without degrading the user experience.
### Why Load Testing is Important
Load testing is essential for several reasons:
1. **Identifying Bottlenecks:** Helps in identifying the parts of your application that slow down under load, such as inefficient queries, slow API endpoints, or resource contention issues.
2. **Ensuring Scalability:** Validates if your application can handle increased traffic and grow as your user base expands.
3. **Preventing Downtime:** Helps in catching issues before they become critical, thus minimizing the risk of downtime during peak usage.
4. **Optimizing Performance:** Provides insights into what elements you need to optimize to improve speed and efficiency, such as middleware, databases, or your server configuration.
5. **User Satisfaction:** Ensures that end-users have a seamless experience even during high-traffic periods, which is crucial for user satisfaction and retention.
### Key Metrics in Load Testing
- **Response Time:** The time taken to receive a response from the server after sending a request. Lower response times usually indicate better performance.
- **Throughput:** The number of requests processed per unit of time, often measured in requests per second (RPS). Higher throughput is generally better but also depends on the application's capacity.
- **Error Rate:** The percentage of requests resulting in errors. A high error rate under load typically indicates your server can't handle the traffic well.
- **CPU Utilization:** The percentage of CPU being used. High CPU usage can be a sign that your server is under heavy load and may need optimization or scaling.
- **Memory Usage:** Tracks the amount of memory being used. High memory usage can lead to slower performance and potential crashes if the server runs out of memory.
- **Latency:** The delay before the transfer of data begins following a request. This is different from response time and is crucial for understanding network performance.
### Example Code: Measuring Key Metrics in NestJS
NestJS provides integrated tools for logging and monitoring metrics. Here’s how you can measure some basic performance indicators using middleware:
```typescript
import { Injectable, NestMiddleware } from '@nestjs/common';
import { Request, Response, NextFunction } from 'express';
@Injectable()
export class MetricsMiddleware implements NestMiddleware {
use(req: Request, res: Response, next: NextFunction) {
const startHrTime = process.hrtime();
res.on('finish', () => {
const elapsedHrTime = process.hrtime(startHrTime);
const elapsedTimeInMs = elapsedHrTime[0] * 1000 + elapsedHrTime[1] / 1e6;
const statusCode = res.statusCode;
console.log(`Method: ${req.method}, URL: ${req.url}, Status: ${statusCode}, Time: ${elapsedTimeInMs.toFixed(3)} ms`);
});
next();
}
}
To apply this middleware, attach it in your main module or a specific module:
import { Module, MiddlewareConsumer, RequestMethod } from '@nestjs/common';
import { MetricsMiddleware } from './metrics.middleware';
@Module({
imports: [],
controllers: [],
providers: [],
})
export class AppModule {
configure(consumer: MiddlewareConsumer) {
consumer
.apply(MetricsMiddleware)
.forRoutes({ path: '*', method: RequestMethod.ALL });
}
}
Understanding the KPIs for load testing can help you make informed decisions. Here is a summary of the key indicators you should be focusing on:
Load testing is a vital practice to ensure your NestJS server is robust, scalable, and performs well under load. By focusing on key metrics and understanding performance indicators, you can make data-driven decisions to optimize and scale your application effectively. Next, we'll cover how to integrate LoadForge to monitor and improve your NestJS application comprehensively.
In this section, we'll walk through the process of integrating LoadForge to load test your NestJS application. By the end of this section, you will be able to set up LoadForge, configure various load test scenarios, and execute your first load test successfully.
Before we begin, make sure you have the following set up:
Sign Up and Log In:
Create a New Project:
Create a Test Plan:
Define Test Scenarios:
Example of a simple GET request scenario:
{
"name": "GET /api/health",
"method": "GET",
"url": "https://your-nestjs-app.com/api/health",
"headers": {
"Content-Type": "application/json"
}
}
Set Load Parameters:
{
"virtual_users": 50,
"ramp_up_time": 60, // in seconds
"duration": 300 // in seconds
}
Start the Test:
Monitor Metrics:
Here is a more detailed example of a complete test configuration in JSON format:
{
"project_name": "NestJS Performance Testing",
"test_plan": {
"name": "NestJS Load Test",
"scenarios": [
{
"name": "GET /api/users",
"method": "GET",
"url": "https://your-nestjs-app.com/api/users",
"headers": {
"Content-Type": "application/json"
},
"virtual_users": 100,
"ramp_up_time": 120, // 2 minutes
"duration": 600 // 10 minutes
},
{
"name": "POST /api/users",
"method": "POST",
"url": "https://your-nestjs-app.com/api/users",
"headers": {
"Content-Type": "application/json"
},
"payload": {
"name": "Test User",
"email": "[email protected]"
},
"virtual_users": 50,
"ramp_up_time": 60, // 1 minute
"duration": 300 // 5 minutes
}
]
}
}
By following these steps, you have successfully integrated LoadForge to load test your NestJS application. Regular load testing using LoadForge will help you identify performance bottlenecks and ensure your NestJS server scales effectively under increased load. In the next section, we will learn how to analyze the results from these load tests and derive meaningful insights for optimization.
Once you’ve executed your load tests using LoadForge, it's crucial to understand the results to optimize your NestJS server effectively. This section will guide you through the key metrics provided by LoadForge and how to interpret them to identify potential performance bottlenecks and areas for improvement.
Response time is the duration your server takes to process a request and send back a response. High response times can indicate performance issues and bottlenecks. Look out for the following types of response times:
Throughput measures the number of requests processed by your server per second. It indicates your application's ability to handle high traffic loads. Higher throughput values are generally better, but ensure they correlate with acceptable response times.
Throughput = Number of Requests / Duration of Test
An error rate is the percentage of failed requests during the load test. High error rates suggest instability in your application under load. Common errors include HTTP 4xx and 5xx status codes, which might point to issues such as rate limiting, insufficient resources, or unhandled exceptions.
Error Rate = (Number of Failed Requests / Total Number of Requests) * 100%
Monitoring server resource utilization helps you understand how your application impacts server resources such as CPU, memory, and disk I/O during load. This can reveal inefficiencies in your code or configuration. Key resource metrics include:
Visualizing metrics through graphs and trends during and after load tests provides insights into how your NestJS application behaves over time under varying loads. LoadForge typically provides:
Let's say you run a load test and observe the following results:
From these results, you have a few insights:
Based on your load test results, you can take action such as:
Regular load testing with LoadForge and thorough analysis of the results will help you ensure that your NestJS server remains performant and scalable. By continuously monitoring and optimizing, you can provide a reliable, fast user experience even under high load conditions.
Based on your LoadForge load testing results, you'll need to implement various strategies to optimize your NestJS server. This section provides practical tips and techniques to enhance performance at different levels, including middleware optimization, route handling, and database interactions.
Middleware functions act as intermediaries that process requests before reaching the actual route handlers. Optimizing middleware can dramatically reduce response times and enhance throughput.
Avoid Synchronous Code: Synchronous operations in middleware can block the request-response cycle, negatively impacting performance.
// Avoid synchronous code like this
app.use((req, res, next) => {
for (let i = 0; i < 1e8; i++) {}
next();
});
Use asynchronous, non-blocking functions instead:
app.use(async (req, res, next) => {
await someAsyncFunction();
next();
});
Minimize Execution Time: Ensure middleware does only what's necessary and offload heavy computation.
// Cache results when possible to avoid repeated computations
const cache = new Map();
app.use((req, res, next) => {
if (cache.has(req.url)) {
res.send(cache.get(req.url));
} else {
next();
}
});
Route handlers are core to your NestJS application's functionality. Efficient route handling is vital for performance.
Implement Caching: Use caching mechanisms like Redis to store frequently accessed data.
@Get('data')
async getData(@Res() res: Response) {
const cacheKey = 'dataKey';
const cachedData = await this.cacheService.get(cacheKey);
if (cachedData) {
return res.send(cachedData);
}
const data = await this.dataService.getData(); // Fetch data from database
await this.cacheService.set(cacheKey, data);
res.send(data);
}
Use Pagination: For routes that return large data sets, implement pagination to reduce the load.
@Get('items')
async getItems(@Query('page') page: number = 1, @Query('limit') limit: number = 10) {
return this.itemService.getPaginatedItems(page, limit);
}
Optimize DTOs: Data Transfer Objects (DTOs) can help in validating and transforming the payload.
@Post('create')
async createItem(@Body() createItemDto: CreateItemDto) {
return this.itemService.createItem(createItemDto);
}
// as opposed to unstructured JSON payloads
Database interactions often pose significant performance bottlenecks. Optimizing these can lead to substantial performance gains.
Efficient Queries: Avoid N+1 query issues by using appropriate ORM methods or native SQL queries.
// Avoid this
const users = await this.userRepository.find();
for (const user of users) {
user.posts = await this.postRepository.find({ userId: user.id });
}
// Prefer this
const usersWithPosts = await this.userRepository.find({
relations: ['posts'],
});
Indexes: Ensure appropriate indexes are set on frequently queried fields.
CREATE INDEX idx_user_email ON users(email);
Connection Pooling: Use connection pooling to manage database connections efficiently, reducing overhead.
// Example configuration for TypeORM
TypeOrmModule.forRoot({
type: 'postgres',
host: 'localhost',
port: 5432,
username: 'test',
password: 'test',
database: 'test',
synchronize: true,
extra: {
max: 10, // Pool size
},
});
Static File Serving: Serve static files using a CDN or reverse proxy like Nginx.
Compression: Use Gzip compression to reduce response sizes.
import * as compression from 'compression';
app.use(compression());
HTTP/2: Enable HTTP/2 for better performance with multiplexing and server push.
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
import * as fs from 'fs';
async function bootstrap() {
const httpsOptions = {
key: fs.readFileSync('path/to/private-key.pem'),
cert: fs.readFileSync('path/to/public-certificate.pem'),
allowHTTP1: true,
};
const app = await NestFactory.create(AppModule, { httpsOptions });
await app.listen(3000);
}
bootstrap();
By optimizing middleware, route handling, and database interactions, you can substantially enhance the performance of your NestJS server. Always base your optimizations on thorough load testing results to ensure targeted and effective improvements.
As your NestJS application grows in popularity, it will encounter increased demands and higher traffic. Ensuring that your application can scale to handle this load is essential for maintaining performance and user satisfaction. Here, we'll explore several strategies for handling increased load and scaling your application, focusing on horizontal scaling, using cluster modes in Node.js, and leveraging containerization tools like Docker and Kubernetes.
Horizontal scaling involves adding more instances of your application to distribute the load across multiple servers. This approach is often more cost-effective and flexible compared to vertical scaling (upgrading hardware).
Load Balancers: Use a load balancer to distribute traffic evenly across your application instances. Popular choices include NGINX, HAProxy, and cloud-based services like AWS Elastic Load Balancing.
Statelessness: Ensure your application is stateless, meaning that each request can be handled by any instance without dependency on previous requests. Store session data in shared storage systems like Redis or databases.
Clustering Mode: Utilize Node.js's built-in clustering to create multiple processes within a single server:
const cluster = require('cluster');
const http = require('http'); const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
console.log(Master ${process.pid} is running
);
// Fork workers. for (let i = 0; i < numCPUs; i++) { cluster.fork(); }
cluster.on('exit', (worker, code, signal) => {
console.log(Worker ${worker.process.pid} died
);
});
} else {
const app = require('./app'); // Your NestJS application entry
app.listen(3000, () => console.log(Worker ${process.pid} started
));
}
Containerization provides an efficient way to manage and deploy multiple instances of your application. Docker and Kubernetes are popular tools for containerization and orchestration.
Dockerize Your Application: Create a Dockerfile for your NestJS application to define the container's environment:
FROM node:14
WORKDIR /usr/src/app
COPY package*.json ./ RUN npm install
COPY . .
EXPOSE 3000 CMD ["npm", "run", "start:prod"]
docker build -t nestjs-app .
docker run -p 3000:3000 nestjs-app
Kubernetes (K8s) allows for automating deployment, scaling, and management of containerized applications.
Create Deployment and Service YAML:
apiVersion: apps/v1
kind: Deployment metadata: name: nestjs-app spec: replicas: 3 selector: matchLabels: app: nestjs-app template: metadata: labels: app: nestjs-app spec: containers: - name: nestjs-app image: nestjs-app:latest ports: - containerPort: 3000
apiVersion: v1 kind: Service metadata: name: nestjs-app-service spec: selector: app: nestjs-app ports: - protocol: TCP port: 80 targetPort: 3000 type: LoadBalancer
kubectl apply -f deployment.yaml
kubectl apply -f service.yaml
By implementing horizontal scaling with load balancers, leveraging Node.js cluster mode, and utilizing containerization tools like Docker and Kubernetes, you can efficiently handle increased load and ensure your NestJS application scales seamlessly. These strategies help distribute the workload, enhance reliability, and maintain optimal performance as your user base grows. In the next section, we will explore setting up automated tests using LoadForge and integrating them into your CI/CD pipelines to continuously monitor and improve your server's performance.
Establishing a routine of continuous performance testing is vital for maintaining and improving the performance of your NestJS server. In this section, we will walk you through how to set up automated tests using LoadForge, integrate them with your CI/CD pipelines, and monitor performance over time.
Automating Load Tests can save you time and ensure that your server consistently meets performance benchmarks. Here’s how you can do it with LoadForge:
Create Load Tests in LoadForge:
Generate API Keys:
Store API Keys Securely:
Here's a sample configuration file in YAML for integrating LoadForge with a CI/CD pipeline:
version: 2.1
jobs:
load-test:
docker:
- image: circleci/node:14
steps:
- checkout
- run:
name: Run LoadForge Test
command: |
curl -X POST \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $LOADFORGE_API_KEY" \
-d '{
"scenarios": [ "stress-test-scenario" ],
"users": 100,
"duration": 300
}' \
https://api.loadforge.io/v1/load-tests
workflows:
version: 2
test-deploy:
jobs:
- load-test
Continuous Integration and Continuous Deployment (CI/CD) pipelines ensure that your application is automatically tested and deployed. Here is how you can integrate LoadForge into your CI/CD pipelines:
Choose a CI/CD Tool: Popular tools include Jenkins, CircleCI, GitHub Actions, and GitLab CI.
Set Up Pipeline Stages: Create stages for building, testing, and deploying your application.
Trigger Load Tests Automatically: Configure your pipeline to run LoadForge tests after the deployment stage. This ensures that any code changes are tested under load before they go live.
Here’s an example using GitHub Actions:
name: CI/CD Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run Unit Tests
run: npm test
deploy:
needs: build
runs-on: ubuntu-latest
steps:
- name: Deploy Application
# Deployment steps here...
load-test:
needs: deploy
runs-on: ubuntu-latest
steps:
- name: Run LoadForge Load Test
run: |
curl -X POST \
-H "Content-Type: application/json" \
-H "Authorization: Bearer ${{ secrets.LOADFORGE_API_KEY }}" \
-d '{
"scenarios": [ "performance-test-scenario" ],
"users": 50,
"duration": 180
}' \
https://api.loadforge.io/v1/load-tests
Consistent Monitoring: Performance should be monitored continuously to identify trends and address issues promptly.
Set Baseline Metrics: Establish baseline performance metrics against which future test results will be compared.
Analyze Trends: Use LoadForge's reporting tools to track key metrics like response time, throughput, and error rates over time.
Alerting: Configure alerts within LoadForge or using external monitoring tools to notify your team about significant performance degradation.
By following these best practices, you can effectively establish a routine of continuous performance testing, ensuring your NestJS server remains performant and scalable under varying loads, thanks to LoadForge.
We've journeyed through the essentials of load testing your NestJS server using LoadForge. Here's a concise summary of the critical points covered in this guide:
Introduction to NestJS and LoadForge: We explored the importance of server performance and how LoadForge can assist in effectively load testing NestJS applications for enhanced performance and scalability.
Setting Up Your NestJS Application: A step-by-step guide to setting up a basic NestJS project, including installing the CLI, creating a new project, and establishing the initial structure.
Understanding Performance Bottlenecks: Detailed insights into common performance bottlenecks such as synchronous operations, improper database queries, and event loop blocking issues, enabling you to pinpoint problem areas swiftly.
Load Testing Basics: The fundamentals of load testing, understanding its importance, and recognizing key performance metrics such as response time, throughput, and error rates.
Integrating LoadForge with NestJS: Configuration steps to integrate LoadForge seamlessly with your NestJS application, including setting up LoadForge, defining load test scenarios, and running your first load test.
Analyzing Load Test Results: How to interpret load test results using key metrics and indicators, such as response times, throughput, error rates, and server resource utilization, to understand your server's performance profile.
Optimizing Your NestJS Server: Practical tips and techniques to tune your server's performance based on load test results, addressing middleware, route handling, and database interaction optimizations.
Handling Increased Load and Scaling: Strategies for scaling your application, including horizontal scaling, cluster modes in Node.js, and utilizing Docker and Kubernetes for containerization.
Best Practices for Continuous Testing: Establishing a routine for continuous performance testing using LoadForge, integrating with CI/CD pipelines, and monitoring server performance over time.
The journey to optimal server performance is continuous. Here are some actionable steps to maintain and improve your NestJS server's performance:
Automate Testing: Implement automated load tests in your CI/CD pipeline to catch performance issues early.
steps:
- name: Run Load Tests
run: |
loadforge-cli test --config loadforge-config.yml
Monitor Regularly: Regularly monitor performance metrics and logs to promptly detect any anomalies.
Stay Updated: Keep your dependencies up to date, including NestJS, to leverage the latest performance improvements and security patches.
Regular load testing is vital to ensure your server can handle varying loads and scales efficiently. Here are some guidelines:
Ensuring the performance and scalability of your NestJS server is crucial for delivering a smooth and responsive user experience. LoadForge provides a powerful and straightforward way to load test your applications, identify bottlenecks, and optimize your server.
By integrating regular load testing into your development workflow and following the best practices outlined in this guide, you can maintain a high-performing and scalable NestJS server. Remember, server performance optimization is an ongoing process, and staying vigilant through consistent testing and monitoring is key to long-term success.
Happy testing! 🚀