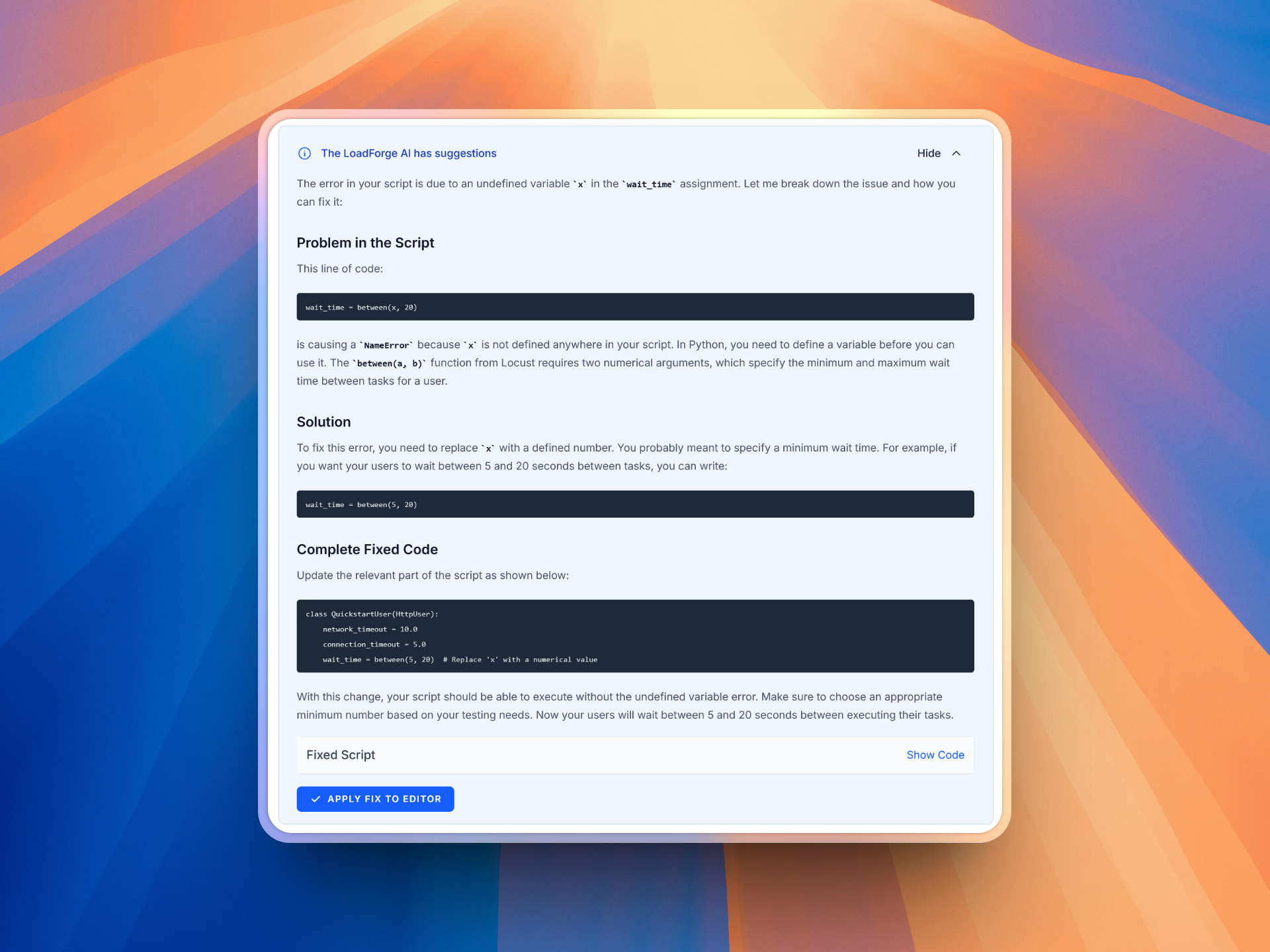
One-Click Scheduling & AI Test Fixes
We're excited to announce two powerful new features designed to make your load testing faster, smarter, and more automated than...
In the development of web applications, managing the flow of requests and responses effectively is crucial for ensuring high performance and maintainability. In the context of NestJS—a progressive Node.js framework for building efficient, reliable, and scalable server-side applications—two fundamental tools...
In the development of web applications, managing the flow of requests and responses effectively is crucial for ensuring high performance and maintainability. In the context of NestJS—a progressive Node.js framework for building efficient, reliable, and scalable server-side applications—two fundamental tools help achieve this: middleware and interceptors.
Middleware functions are a familiar concept to many web developers, especially those with experience in frameworks like Express.js. In NestJS, middleware is used to process requests before they reach the route handler. They are an essential part of the request-response cycle, providing a way to execute code, make changes to the request and response objects, end the request-response cycle, and call the next middleware function in the stack.
Here's a basic example of a middleware function in NestJS:
typescript
import { Injectable, NestMiddleware } from '@nestjs/common';
@Injectable()
export class LoggerMiddleware implements NestMiddleware {
use(req: Request, res: Response, next: Function) {
console.log(`Request...`);
next();
}
}
While middleware functions act before the route handler processes a request, interceptors are more versatile and operate both before and after the route handler is invoked. Interceptors in NestJS can transform data coming into and leaving the application, making them powerful tools for manipulating and controlling request-response data flow in a more granular way.
Here's a basic example of an interceptor in NestJS:
typescript
import { Injectable, NestInterceptor, ExecutionContext, CallHandler } from '@nestjs/common';
import { Observable } from 'rxjs';
import { map } from 'rxjs/operators';
@Injectable()
export class TransformInterceptor implements NestInterceptor> {
intercept(context: ExecutionContext, next: CallHandler): Observable> {
return next
.handle()
.pipe(
map(data => ({ data }))
);
}
}
Aspect | Middleware | Interceptors |
---|---|---|
Position in Cycle | Before the route handler | Before and after the route handler |
Main Use Cases | Request/response modification, authentication | Data transformation, performance monitoring |
Flexibility | Focused on request processing | Can process both requests and responses |
Implementation Focus | Simple request processing tasks | Complex data handling and transformation tasks |
Understanding the distinct roles of middleware and interceptors is crucial for optimizing your NestJS application. Middleware is best suited for straightforward pre-processing tasks, while interceptors provide a more flexible and powerful toolset for managing complex data transformations and cross-cutting concerns. As we proceed with this guide, we will delve deeper into identifying performance bottlenecks and implementing best practices for both middleware and interceptors.
Performance bottlenecks can greatly affect the scalability and responsiveness of your NestJS application. Identifying these bottlenecks is the first crucial step towards optimization. This section will guide you through the process of detecting and analyzing performance issues using tools like LoadForge and other monitoring instruments.
Load testing is essential to evaluate how your server performs under different conditions. LoadForge is a powerful tool you can use to simulate various loads on your NestJS application. Here’s how you can set up and interpret load tests with LoadForge:
Define Test Scenarios: Establish different scenarios that represent real-world usage patterns. This might include varying the number of concurrent users, the types of requests being made, and the duration of the tests.
Setup LoadForge: Use LoadForge’s intuitive interface to configure your test parameters. For instance:
{
"testName": "Middleware Stress Test",
"targetURL": "https://your-nestjs-app.com/api",
"duration": 300, // in seconds
"concurrentUsers": 100,
"rampUpTime": 60 // in seconds
}
Run the Test: Execute the load test and monitor the performance metrics provided by LoadForge. Look for key indicators such as response time, throughput, and error rates.
Analyze Results: Identify endpoints with high latency or error rates, often a sign of bottlenecks in your middleware or interceptors. LoadForge provides detailed reports that can help pinpoint the problematic areas.
In addition to load testing, real-time monitoring tools can provide continuous insights into your application’s performance. Here are some popular tools:
Profiling your code is another effective way to uncover performance bottlenecks. Tools like clinic.js
can be used for detailed analysis:
Install Clinic.js:
npm install -g clinic
Run Clinic.js with Your NestJS Application:
clinic doctor -- node dist/main.js
Analyze the Output: After running your application under load, clinic.js
will generate a report highlighting slow or blocking operations within your middleware and interceptors.
Consider a simple logging middleware that adds significant delay to your requests:
import { Injectable, NestMiddleware } from '@nestjs/common';
@Injectable()
export class LoggingMiddleware implements NestMiddleware {
use(req: Request, res: Response, next: Function) {
console.time('Request-Response Time');
res.on('finish', () => {
console.timeEnd('Request-Response Time');
});
// Simulate slow operation
setTimeout(() => next(), 100);
}
}
Identified Issue: The setTimeout
function introduces a 100ms delay, which can become a performance bottleneck under high load.
Solution: Remove unnecessary delays and optimize the logging process. Alternatively, implement asynchronous logging mechanisms.
The above example highlights a common issue that profiling can identify. By using tools like LoadForge for load testing and other monitoring solutions, you can effectively pinpoint and address performance bottlenecks, ensuring your NestJS application runs smoothly and efficiently.
Middleware in NestJS plays a critical role in processing requests before they reach your routes. As such, optimizing middleware execution is vital for enhancing the overall performance of your NestJS application. In this section, we'll cover best practices for optimizing middleware, including minimizing synchronous operations and using efficient algorithmic approaches.
Synchronous operations, particularly those involving I/O, can be a significant bottleneck in middleware execution. When middleware operations are synchronous, they block the event loop, leading to decreased throughput and increased response times.
Long-running tasks should be avoided within middleware. For example, instead of performing a synchronous, blocking database query, use an asynchronous approach:
// Avoid
const fetchUserDataSync = (req, res, next) => {
const userData = database.fetchUserSync(req.userId); // Blocking operation
req.user = userData;
next();
};
// Use
const fetchUserDataAsync = async (req, res, next) => {
try {
const userData = await database.fetchUser(req.userId); // Non-blocking operation
req.user = userData;
next();
} catch (error) {
next(error);
}
};
Selecting efficient algorithms for processing within middleware can make a significant difference in performance. Avoiding operations with high time complexity (e.g., O(n²)) in favor of more efficient ones (e.g., O(n) or O(log n)) is crucial.
Consider a middleware that searches for a user in a list. Instead of using a linear search, which has O(n) complexity, a hash map can be used to bring this down to O(1) complexity for lookups.
// Linear search (O(n))
const findUser = (req, res, next) => {
const user = users.find(u => u.id === req.userId); // O(n) complexity
req.user = user;
next();
};
// Using a hash map for constant time lookup (O(1))
const userMap = new Map(users.map(u => [u.id, u])); // Preprocessing users
const findUserEfficiently = (req, res, next) => {
const user = userMap.get(req.userId); // O(1) complexity
req.user = user;
next();
};
In scenarios where middleware is used for data transformation, efficient techniques and libraries optimized for performance should be leveraged.
Using libraries like fast-json-stringify
for JSON operations can improve performance as they are designed to be more efficient:
// Standard JSON stringify
const transformData = (req, res, next) => {
req.body.transformed = JSON.stringify(req.body.data); // Standard transformation
next();
};
// Using fast-json-stringify for better performance
const fastJson = require('fast-json-stringify');
const stringify = fastJson({
type: 'object',
properties: {
data: { type: 'string' }
}
});
const transformDataEfficiently = (req, res, next) => {
req.body.transformed = stringify({ data: req.body.data });
next();
};
Ensure that only necessary middleware is used and that they are applied to specific routes rather than globally. This minimizes the overhead and improves the performance.
Instead of applying middleware globally, apply it only where needed:
// Apply globally
app.use(logRequests);
// Apply to specific route
app.use('/api/user', logRequests);
app.get('/api/user', userController.getUser);
By applying these practices, you can significantly improve the performance of your NestJS middleware, reducing the load on your server and speeding up the request-response cycle. In the next sections, we'll delve into more advanced techniques such as using asynchronous middleware and leveraging built-in and third-party middleware effectively.
Middleware is a powerful feature in NestJS, allowing you to modify the incoming request object, process the response object, and execute code during the request-response cycle. One of the key performance improvements you can make in your NestJS application involves converting synchronous middleware functions to asynchronous ones. This can significantly enhance performance, especially under load, by improving concurrency and reducing blocking operations.
Asynchronous middleware leverages non-blocking I/O operations, allowing your application to handle more requests concurrently. When middleware is synchronous, each request may block the event loop, leading to performance degradation under high traffic. By using asynchronous operations, you ensure that the event loop remains unblocked, leading to improved throughput and responsiveness.
Converting synchronous middleware to asynchronous in NestJS is straightforward, thanks to JavaScript's async/await syntax and Promise-based patterns. Below are practical examples and tips for converting your middleware functions.
Here's an example of a simple synchronous middleware function:
typescript
import { Injectable, NestMiddleware } from '@nestjs/common';
@Injectable()
export class SyncMiddleware implements NestMiddleware {
use(req: Request, res: Response, next: Function) {
// Synchronous blocking operation
for (let i = 0; i < 1000000000; i++) { /* some CPU intensive task */ }
next();
}
}
This middleware performs a CPU-intensive task synchronously, which can block the event loop and degrade performance.
To convert this middleware to asynchronous, you can perform the CPU-intensive task in a non-blocking way, such as using asynchronous APIs or moving heavy computation to worker threads.
import { Injectable, NestMiddleware } from '@nestjs/common';
import { readFile } from 'fs/promises'; // Example of an asynchronous operation
@Injectable()
export class AsyncMiddleware implements NestMiddleware {
async use(req: Request, res: Response, next: Function) {
try {
// Asynchronous non-blocking I/O operation
const data = await readFile('/path/to/large/file.txt', 'utf-8');
console.log(data);
} catch (error) {
console.error('Error reading file:', error);
}
next();
}
}
Use Async/Await and Promises: Make use of JavaScript's async/await syntax or Promise-based APIs to write non-blocking code. This ensures that I/O operations do not block the event loop.
Avoid Blocking Operations: Move CPU-intensive tasks or long-running computations to worker threads or external services. This allows your main application thread to handle more requests concurrently.
Parallelize Workloads: For operations that can be performed in parallel (e.g., multiple API calls), use Promise.all or other concurrency control mechanisms to optimize performance.
Leverage Built-in Asynchronous Functions: Utilize Node.js built-in asynchronous functions instead of their synchronous counterparts (e.g., readFile
from fs/promises
instead of fs.readFileSync
).
Handle Errors Gracefully: Ensure proper error handling in asynchronous middleware to avoid unhandled promise rejections and maintain application stability.
import { Injectable, NestMiddleware } from '@nestjs/common';
import { readFile } from 'fs/promises'; // Example of an asynchronous operation
@Injectable()
export class AsyncMiddleware implements NestMiddleware {
async use(req: Request, res: Response, next: Function) {
try {
// Asynchronous non-blocking I/O operation
const data = await readFile('/path/to/large/file.txt', 'utf-8');
console.log(data);
} catch (error) {
console.error('Error reading file:', error);
}
next();
}
}
By incorporating asynchronous middleware into your NestJS application, you can greatly improve its performance and ensure that it scales efficiently under varying loads. Remember to test and monitor your modifications using tools like LoadForge to validate performance gains and identify any further optimizations needed.
Continue optimizing your application by exploring other sections of this guide, including efficient use of built-in and third-party middleware, creating efficient interceptors, and leveraging caching strategies.
Middleware in NestJS plays a crucial role in managing the request-response cycle, especially when it comes to adding functionalities such as logging, authentication, and data transformation. Efficient use of both built-in and third-party middleware is essential for optimizing performance in your NestJS applications. This section will provide insights into how to leverage these middleware types efficiently.
NestJS comes with several built-in middleware options that are designed to handle common tasks efficiently. Here’s how you can make the most out of these built-in middleware:
Body Parsing Middleware:
import { Module, NestModule, MiddlewareConsumer } from '@nestjs/common';
import { json, urlencoded } from 'express';
@Module({
// Module metadata
})
export class AppModule implements NestModule {
configure(consumer: MiddlewareConsumer) {
consumer
.apply(json(), urlencoded({ extended: true }))
.forRoutes('*');
}
}
Compression Middleware:
import * as compression from 'compression';
import { Module, NestModule, MiddlewareConsumer } from '@nestjs/common';
@Module({
// Module metadata
})
export class AppModule implements NestModule {
configure(consumer: MiddlewareConsumer) {
consumer
.apply(compression())
.forRoutes('*');
}
}
Third-party middleware can add significant value beyond the built-in capabilities. However, it’s essential to carefully select and configure them for optimal performance:
Helmet for Security:
import * as helmet from 'helmet';
import { Module, NestModule, MiddlewareConsumer } from '@nestjs/common';
@Module({
// Module metadata
})
export class AppModule implements NestModule {
configure(consumer: MiddlewareConsumer) {
consumer
.apply(helmet())
.forRoutes('*');
}
}
Rate Limiting Middleware:
import * as rateLimit from 'express-rate-limit';
import { Module, NestModule, MiddlewareConsumer } from '@nestjs/common';
@Module({
// Module metadata
})
export class AppModule implements NestModule {
configure(consumer: MiddlewareConsumer) {
consumer
.apply(rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per windowMs
}))
.forRoutes('*');
}
}
It's important to strike a balance between functionality and performance. Here are a few best practices:
Below is an example demonstrating efficient use of both built-in and third-party middleware:
import { Module, NestModule, MiddlewareConsumer } from '@nestjs/common';
import { json, urlencoded } from 'express';
import * as helmet from 'helmet';
import * as compression from 'compression';
import * as rateLimit from 'express-rate-limit';
@Module({
// Module metadata
})
export class AppModule implements NestModule {
configure(consumer: MiddlewareConsumer) {
consumer
.apply(
json(),
urlencoded({ extended: true }),
helmet(),
compression(),
rateLimit({
windowMs: 15 * 60 * 1000,
max: 100,
}),
)
.forRoutes('*');
}
}
This example demonstrates the application of various middleware to handle body parsing, security, compression, and rate limiting efficiently. By carefully selecting and configuring middleware, you can ensure that your NestJS application remains both functional and performant.
In NestJS, interceptors are a powerful feature that allow you to shape the behavior of your application’s request-response cycle in a highly flexible manner. While middleware processes requests before they reach your route handlers, interceptors wrap around your method calls, offering additional points of control both before and after method execution. This section delves into what interceptors are, how they differ from middleware, and their pivotal role in boosting the performance of your NestJS application.
Interceptors in NestJS are methods that can intercept method calls and modify their input/output. Their primary purposes include:
Consider interceptors as middleware that can operate at the more granular service or controller level and can work both synchronously and asynchronously.
While middleware and interceptors might appear similar at first glance, they serve different purposes and are used at different stages of the request-response cycle.
Execution Context: Middleware operates globally or at the router level before the request reaches the route handler, often used for tasks such as authentication or body parsing. Interceptors, on the other hand, wrap around route handlers and service methods, providing more focused control.
Behavior Scope: Middleware primarily influences input data coming into the application, whereas interceptors can manipulate both input and output data.
Flexibility: Interceptors offer finer control as they allow execution before and after method execution. Middleware generally does not have this dual-phase capability.
Interceptors can play a critical role in enhancing the performance of your NestJS application. Here are several scenarios where they can significantly boost efficiency:
The following example demonstrates a basic interceptor that logs the execution time of a route handler:
import {
CallHandler,
ExecutionContext,
Injectable,
NestInterceptor,
} from '@nestjs/common';
import { Observable } from 'rxjs';
import { tap } from 'rxjs/operators';
@Injectable()
export class LoggingInterceptor implements NestInterceptor {
intercept(context: ExecutionContext, next: CallHandler): Observable<any> {
const now = Date.now();
return next
.handle()
.pipe(
tap(() => console.log(`Execution time: ${Date.now() - now}ms`))
);
}
}
Usage:
To use the interceptor, apply it to a controller or method:
import { Controller, Get, UseInterceptors } from '@nestjs/common';
import { LoggingInterceptor } from './logging.interceptor';
@Controller('items')
@UseInterceptors(LoggingInterceptor)
export class ItemsController {
@Get()
findAll(): string {
return 'This action returns all items';
}
}
This interceptor logs the time taken to execute the findAll
method. By implementing such interceptors, you can monitor and optimize performance-critical sections of your application.
Interceptors in NestJS provide the ability to enhance the performance of your application by wrapping around method calls and manipulating data at strategic points. Understanding their distinct role from middleware and leveraging their capabilities for tasks like caching, logging, and response transformation can significantly enhance the efficiency and responsiveness of your application. In the subsequent sections, we will explore how to create and optimize these interceptors further for peak performance.
Interceptors in NestJS offer a powerful way to manipulate request and response data, handle errors, manage caching, and implement cross-cutting concerns such as logging and authentication in a modular and reusable manner. However, creating efficient interceptors is crucial to avoid introducing performance bottlenecks. In this section, we will explore best practices for crafting efficient interceptors in NestJS, focusing on reducing unnecessary operations and optimizing data transformation processes.
Synchronous operations can significantly impact the performance of your application, especially when dealing with high concurrency. Instead, leverage asynchronous operations where applicable to ensure non-blocking behavior.
Example of an inefficient synchronous interceptor:
import { CallHandler, ExecutionContext, Injectable, NestInterceptor } from '@nestjs/common';
import { Observable } from 'rxjs';
@Injectable()
export class SyncInterceptor implements NestInterceptor {
intercept(context: ExecutionContext, next: CallHandler): Observable {
const start = Date.now();
// Example of synchronous blocking code
while (Date.now() - start < 1000) {
// Blocking for 1 second
}
return next.handle();
}
}
Example of an efficient asynchronous interceptor:
import { CallHandler, ExecutionContext, Injectable, NestInterceptor } from '@nestjs/common';
import { Observable, of } from 'rxjs';
@Injectable()
export class AsyncInterceptor implements NestInterceptor {
intercept(context: ExecutionContext, next: CallHandler): Observable {
return new Promise((resolve) => setTimeout(resolve, 1000)).then(() => next.handle());
}
}
Eliminate operations within interceptors that do not directly contribute to the specific goals of the interceptor. Avoid redundant computations or unnecessary tasks that can be deferred or omitted.
Map
instead of Array
for lookups).Example of unnecessary operations:
import { CallHandler, ExecutionContext, Injectable, NestInterceptor } from '@nestjs/common';
import { Observable } from 'rxjs';
@Injectable()
export class InefficientInterceptor implements NestInterceptor {
intercept(context: ExecutionContext, next: CallHandler): Observable {
const request = context.switchToHttp().getRequest();
// Simulate redundant logging
console.log(`Processing request for ${request.url}`);
console.log(`Received headers: ${JSON.stringify(request.headers)}`);
return next.handle();
}
}
Data transformation is a common task in interceptors, involving serialization, deserialization, or modification of request/response data. Aim to optimize these processes to minimize the overhead.
JSON.parse
and JSON.stringify
are generally fast).Example of optimized data transformation:
import { CallHandler, ExecutionContext, Injectable, NestInterceptor } from '@nestjs/common';
import { Observable } from 'rxjs';
import { map } from 'rxjs/operators';
@Injectable()
export class TransformInterceptor implements NestInterceptor {
intercept(context: ExecutionContext, next: CallHandler): Observable {
return next.handle().pipe(
map(data => {
// Example of optimized transformation
data.modifiedAt = new Date();
return data;
})
);
}
}
If your interceptor performs expensive operations, consider implementing caching strategies to avoid redundant processing. This is discussed in-depth in another section, but consider how it applies to your transformations and repetitive calculations.
Creating efficient interceptors in NestJS hinges on minimizing synchronous operations, reducing redundant tasks, and optimizing data transformations. These practices help ensure that your interceptors enhance functionality without compromising the performance of your NestJS application. In the following sections, we'll delve deeper into advanced tips, including practical caching strategies and leveraging LoadForge for testing your optimizations under real-world load conditions.
Effective caching strategies play a pivotal role in improving the performance of your NestJS applications. By reducing the need for repeated processing of identical requests, caching helps minimize response times and server load. In this section, we will explore various caching techniques and strategies that can be implemented within interceptors to enhance the overall performance of your NestJS application.
Interceptors in NestJS are capable of transforming or manipulating requests and responses. By integrating caching mechanisms within interceptors, you can:
To implement caching in interceptors, you can use various in-memory caches like Redis or in-memory storage available within the application. Here’s a step-by-step guide on how to integrate caching within a custom interceptor:
Install Dependencies:
First, you need to install the necessary caching library. For Redis, you can use the ioredis
library:
npm install ioredis
Create a Cache Service: Create a dedicated cache service to handle cache logic:
import { Injectable } from '@nestjs/common';
import * as Redis from 'ioredis';
@Injectable()
export class CacheService {
private client: Redis.Redis;
constructor() {
this.client = new Redis();
}
async get(key: string): Promise<string | null> {
return this.client.get(key);
}
async set(key: string, value: string, ttl: number): Promise<void> {
await this.client.set(key, value, 'EX', ttl);
}
}
Create the Interceptor:
Create an interceptor that leverages the CacheService
to handle caching:
import {
CallHandler,
ExecutionContext,
Injectable,
NestInterceptor,
} from '@nestjs/common';
import { Observable, of } from 'rxjs';
import { tap } from 'rxjs/operators';
import { CacheService } from './cache.service';
@Injectable()
export class CachingInterceptor implements NestInterceptor {
constructor(private readonly cacheService: CacheService) {}
async intercept(
context: ExecutionContext,
next: CallHandler,
): Promise<Observable<any>> {
const request = context.switchToHttp().getRequest();
const cacheKey = `${request.method}_${request.url}`;
const cachedResponse = await this.cacheService.get(cacheKey);
if (cachedResponse) {
return of(JSON.parse(cachedResponse));
}
return next.handle().pipe(
tap(response => {
this.cacheService.set(cacheKey, JSON.stringify(response), 300);
}),
);
}
}
Apply the Interceptor:
Finally, apply the CachingInterceptor
to your routes or globally:
import { Controller, Get, UseInterceptors } from '@nestjs/common';
import { CachingInterceptor } from './caching.interceptor';
@Controller('example')
@UseInterceptors(CachingInterceptor)
export class ExampleController {
@Get()
findAll() {
// Your logic here
}
}
When using caching strategies in interceptors, keep the following best practices in mind:
By thoughtfully implementing caching strategies in interceptors, you can significantly enhance the efficiency and responsiveness of your NestJS applications, ensuring a seamless experience for your users.
## Error Handling and Logging in Interceptors
Efficient error handling and logging are crucial components in building robust NestJS applications. Interceptors provide an ideal mechanism for managing these concerns globally. In this section, we will discuss the importance of implementing non-blocking operations and maintaining minimal performance overhead when handling errors and logging information within interceptors.
### Importance of Efficient Error Handling
Efficient error handling ensures that your application can gracefully recover from unexpected conditions without significantly degrading performance. In a high-performance NestJS application, it is essential to:
1. **Catch and Handle Errors Proactively**: Prevent unhandled exceptions from propagating through the system.
2. **Provide Informative and Consistent Responses**: Return meaningful error messages to clients while avoiding information leakage.
3. **Minimize Performance Overhead**: Implement error handling that does not introduce significant latency or resource consumption.
### Efficient Logging Techniques
Logging is critical for monitoring application health, debugging issues, and maintaining an audit trail. However, logging operations can become a performance bottleneck if not handled properly. To ensure efficient logging in interceptors, consider the following practices:
1. **Use Asynchronous Logging**: Write logs asynchronously to avoid blocking the main request processing thread.
2. **Selective Logging**: Log only essential information to reduce the volume of log data and improve performance.
3. **Batch Logging**: Accumulate log entries and write them in batches to reduce I/O operations.
### Implementing Non-blocking Operations
To maintain performance, it is critical to implement non-blocking operations within interceptors. This means leveraging asynchronous patterns and avoiding synchronous and blocking calls where possible. Here’s an example of an interceptor implementing asynchronous error handling and logging:
<pre><code>
import { CallHandler, ExecutionContext, Injectable, NestInterceptor } from '@nestjs/common';
import { Observable, throwError } from 'rxjs';
import { catchError, tap } from 'rxjs/operators';
import { LoggerService } from '../services/logger.service';
@Injectable()
export class LoggingInterceptor implements NestInterceptor {
constructor(private readonly logger: LoggerService) {}
intercept(context: ExecutionContext, next: CallHandler): Observable<any> {
const now = Date.now();
return next
.handle()
.pipe(
tap(() => this.logSuccess(context, now)),
catchError((err) => this.handleAndLogError(context, err, now)),
);
}
private logSuccess(context: ExecutionContext, startTime: number) {
const { method, url } = context.switchToHttp().getRequest();
const responseTime = Date.now() - startTime;
this.logger.log(`Request to ${method} ${url} succeeded in ${responseTime}ms`);
}
private handleAndLogError(context: ExecutionContext, err: any, startTime: number): Observable<never> {
const { method, url } = context.switchToHttp().getRequest();
const responseTime = Date.now() - startTime;
// Asynchronous logging
this.logger.error(`Request to ${method} ${url} failed in ${responseTime}ms`, err.stack);
// Transform error response if needed
return throwError(() => new Error('Internal server error'));
}
}
</code></pre>
### Practical Tips
- **Use Efficient Logging Libraries**: Use libraries like `winston` or `pino` that support asynchronous logging and highly performant logging operations.
- **Avoid Blocking Calls**: In error handling and logging, avoid synchronous operations such as file I/O or database writes. Instead, use asynchronous APIs.
- **Rate Limiting**: Implement rate-limiting mechanisms to prevent log flooding during high error rates.
- **Monitoring and Alerts**: Set up monitoring and alerts to track and notify significant error patterns and performance degradation.
### Conclusion
By applying these strategies, you can ensure that error handling and logging in your NestJS interceptors are both efficient and effective, thus maintaining high performance while providing robust error management. In the next section, we will explore how to test the performance of your middleware and interceptors using LoadForge, ensuring they meet your application's requirements under different load conditions.
## Testing Middleware and Interceptors with LoadForge
When it comes to ensuring the robustness and performance of your NestJS application, load testing is an indispensable practice. LoadForge is a powerful tool designed to help you simulate various load conditions and measure the performance of your middleware and interceptors. Here, we'll walk you through the steps to effectively test the performance of your middleware and interceptors using LoadForge.
### Setting Up LoadForge for Your NestJS Application
Before diving into load testing, ensure you have LoadForge set up. If you haven't already, create an account with LoadForge and configure your project. Next, follow these steps:
1. **Install the LoadForge CLI:**
Download and install the LoadForge CLI from the official LoadForge website.
2. **Configure Your NestJS Application:**
Ensure your NestJS application is running and accessible. You may want to set up a test environment that mirrors your production environment as closely as possible.
3. **Create Your LoadForge Test Plan:**
Develop a test plan that includes scenarios reflecting real-world usage patterns. Consider different endpoints that involve various middleware and interceptor chains.
### Writing LoadForge Test Scripts
To test specific middleware and interceptors, you'll need to write scripts that exercise the parts of your application where they are applied. Below is an example of a simple LoadForge test script:
<pre><code>
import { test } from 'loadforge';
test('GET /api/users', async ({ request }) => {
const response = await request.get('/api/users');
console.log(`Status: ${response.status}`);
assert(response.status).toBe(200);
});
test('POST /api/users', async ({ request }) => {
const response = await request.post('/api/users', {
json: {
name: 'Test User',
email: '[email protected]'
},
});
console.log(`Status: ${response.status}`);
assert(response.status).toBe(201);
});
</code></pre>
This example targets two endpoints, one with a GET request and another with a POST request. Both endpoints should ideally invoke various middleware and interceptors you've set up.
### Running Load Tests
With your scripts in place, you're ready to run load tests and monitor performance metrics:
1. **Execute LoadForge Test Plan:**
Using the LoadForge CLI, execute your test plan. Monitor real-time feedback provided by LoadForge to ensure the tests are running as expected.
```sh
loadforge run testPlan.json
LoadForge offers several key metrics that are crucial for performance analysis:
Once you have identified bottlenecks, you can fine-tune your middleware and interceptors:
Performance optimization is an iterative process. After making adjustments, re-run your LoadForge tests to verify improvements. Continue this cycle until you meet your performance goals.
Effective load testing with LoadForge will help you ensure that your NestJS middleware and interceptors are optimized for performance. This rigorous testing process allows you to confidently handle real-world traffic, providing a smooth and responsive experience for your users.
By integrating LoadForge into your development workflow, you gain invaluable insights into your application's performance characteristics, leading to more efficient and reliable NestJS applications.
In this guide, we have delved into various strategies to optimize middleware and interceptors in NestJS, enhancing the overall performance of your applications. Here, we'll summarize the key points and provide some best practices for effectively managing middleware and interceptors in NestJS.
Introduction to Middleware and Interceptors:
Identifying Performance Bottlenecks:
Optimizing Middleware Execution:
Asynchronous Middleware:
async/await
to leverage non-blocking operations.Efficient Use of Built-in and Third-party Middleware:
Creating Efficient Interceptors:
Caching Strategies in Interceptors:
Error Handling and Logging in Interceptors:
Optimize for Asynchronicity:
async function loggerMiddleware(req, res, next) {
await performAsyncOperation();
next();
}
Avoid Redundant Middleware:
Order Matters:
Leverage NestJS Built-in Middleware:
Use Cache Wisely:
@UseInterceptors(CacheInterceptor)
Efficient Data Transformation:
Asynchronous and Non-blocking Operations:
Effective Error Handling:
Monitoring and Load Testing:
Optimizing middleware and interceptors in NestJS is crucial for maintaining efficient, responsive, and scalable applications. By following the best practices outlined in this guide, you can significantly enhance the performance of your NestJS applications. Remember, continuous monitoring, testing with tools like LoadForge, and iterative optimization are key to achieving consistently high performance.