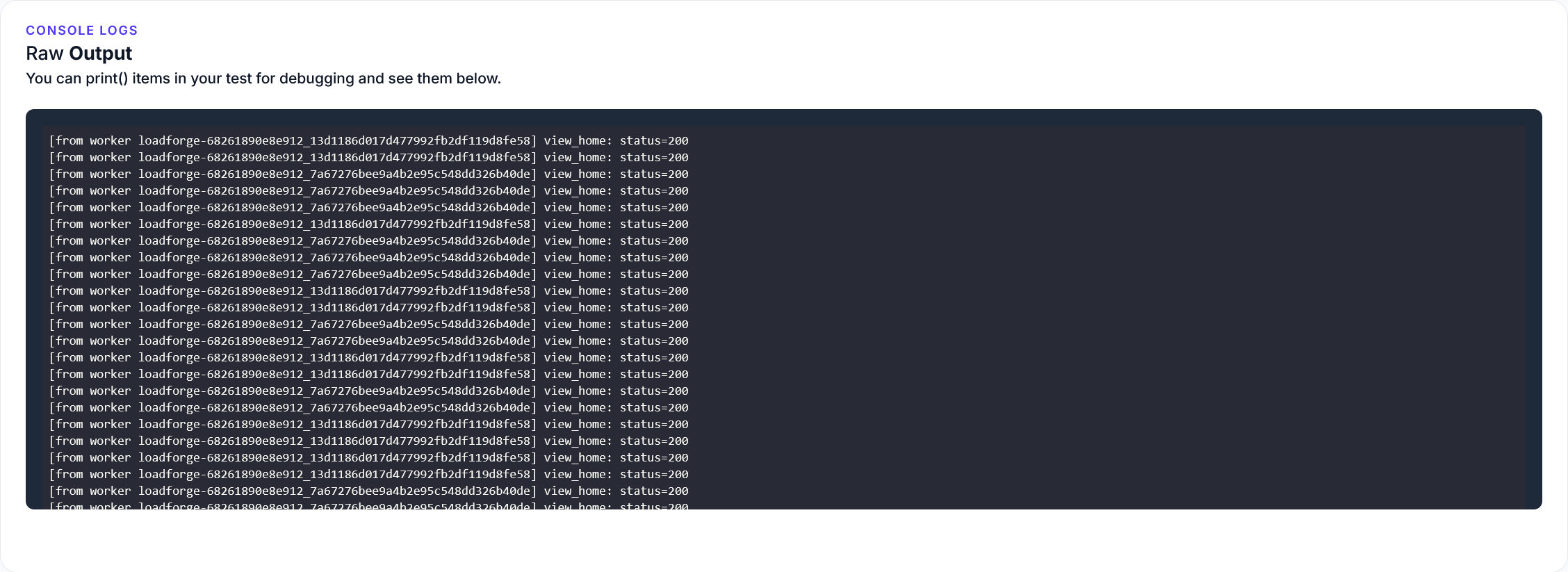
Easier Debug Logging
We're excited to announce a powerful new feature for LoadForge users: comprehensive debug logging for test runs. This highly requested...
## Introduction In today's digital age, the performance of a web application is paramount. Users demand swift interactions and rapid content load times, making it crucial for developers to prioritize optimization strategies. One effective method to enhance your application's performance...
In today's digital age, the performance of a web application is paramount. Users demand swift interactions and rapid content load times, making it crucial for developers to prioritize optimization strategies. One effective method to enhance your application's performance is through caching. This guide focuses on leveraging caching mechanisms within Strapi, an open-source headless CMS, to accelerate response times and improve overall user experience.
The primary objective of this guide is to provide you with a comprehensive understanding of various caching techniques and how they can be implemented in Strapi. We'll start with the basics of caching and progressively dive into more advanced concepts, ensuring you have the knowledge to make informed decisions about your caching strategy.
Caching plays a pivotal role in the performance optimization of web applications. By storing frequently accessed data in a readily available cache, you reduce the need to fetch this data repeatedly from the original source, be it a database or an external API. This not only speeds up data retrieval but also decreases the load on your servers, resulting in enhanced scalability and user experience. For CMS platforms like Strapi, where content delivery speed is essential, efficient caching mechanisms are even more critical.
This guide will cover the following key areas:
Introduction to Caching: We'll begin by explaining what caching is, how it works, and why it's vital for web applications, especially content management systems like Strapi.
Types of Caching: There are various caching methods available, each with its unique benefits. We'll explore in-memory caching, database caching, and CDN caching to give you a well-rounded perspective.
Configuring In-memory Caching in Strapi: Learn how to set up in-memory caching using tools like Redis and configure appropriate cache policies.
Database Query Caching: Discover how to cache database queries in Strapi to minimize database load and improve response times.
Using Content Delivery Networks (CDNs): Understand how integrating CDNs with Strapi can cache static assets and optimize traffic routing for faster content delivery.
Implementing Cache-Control Headers: Learn how to set up and manage cache-control headers to guide the caching behaviors of HTTP responses, attenuating content delivery efficiency.
Cache Invalidation Strategies: Explore different strategies for cache invalidation to ensure users receive the most current content without redundant data fetching.
Monitoring and Analyzing Cache Performance: Introduction to tools and techniques for monitoring and analyzing the effectiveness of your caching strategies, focusing on hit-miss ratios and overall performance.
Advanced Caching Techniques: Delve into advanced concepts like edge-side includes (ESI) and partial content caching for more granular control over content delivery.
Leveraging LoadForge for Load Testing: Understand how to use LoadForge to perform load testing on your Strapi application, ensuring it efficiently handles traffic with implemented caching strategies.
By the end of this guide, you will be equipped with the knowledge to implement and fine-tune caching strategies in Strapi, significantly enhancing its performance and scalability. Let's get started on optimizing your Strapi application for blazing-fast speed and outstanding user experience.
## What is Caching?
Caching is a critical optimization technique that enhances the performance of web applications by storing copies of frequently accessed data in temporary storage, or "caches," for quicker retrieval. Instead of fetching or computing data from the original source each time it's requested, the application serves the stored data from the cache, resulting in significantly faster response times. This is particularly vital for CMS platforms like Strapi, which handle a large volume of content queries and data transactions.
### How Caching Works
Understanding how caching works involves recognizing its core components and operations:
1. **Cache Storage**: This is where the cached data resides. It can be memory (RAM), disk storage, or a distributed cache system like Redis or Memcached.
2. **Cache Keys**: Each piece of data stored in the cache is identified by a unique cache key. This key is used to retrieve the data quickly.
3. **Cache Lifetimes (TTL)**: Cached data often has a time-to-live (TTL), which specifies the duration the data should be retained in the cache before it is considered stale.
4. **Cache Eviction Policies**: Determines how old or less frequently accessed data is removed from the cache when new data needs to be stored, ensuring optimal use of the cache storage.
Here’s a basic example of caching a result in a JavaScript object, which can be a crude form of in-memory caching:
```javascript
const cache = {};
function fetchData(key) {
if (cache[key]) {
return cache[key]; // Return from cache
}
const data = databaseQuery(key); // Assume databaseQuery fetches data from DB
cache[key] = data;
return data;
}
// Usage
let result = fetchData('some-key');
console.log(result);
Caching is crucial in web applications for several reasons:
Strapi, being a headless CMS, often handles dynamic content that needs to be efficiently retrieved and served. Here’s why caching is particularly relevant:
By implementing strategic caching at various levels—such as in-memory caching, database query caching, and CDN caching—Strapi developers can achieve optimal performance, reduce server loads, and ensure a seamless user experience. The following sections will delve deeper into specific caching strategies and their configurations tailored for Strapi.
To effectively enhance the performance of your Strapi application, it's crucial to understand the various types of caching methods available and how they can be leveraged. In this section, we’ll cover three primary types of caching techniques relevant to Strapi: in-memory caching, database caching, and CDN caching. Each of these methods has its own strengths and specific use cases, enabling you to significantly improve response times and reduce server load.
In-memory caching involves storing frequently accessed data in the system memory (RAM) instead of a disk-based storage system. This type of caching is extremely fast because it eliminates the need for time-consuming disk I/O operations. Redis is a commonly used tool for implementing in-memory caching in Strapi.
Benefits:
Example Configuration:
To set up in-memory caching using Redis in Strapi, you can use the following configuration in your Strapi middleware settings:
// /config/middleware.js
module.exports = {
settings: {
cache: {
enabled: true,
type: 'redis',
host: '127.0.0.1',
port: 6379,
},
},
};
Database caching is aimed at reducing the load on your database by caching the results of expensive or frequently executed queries. By doing so, you can speed up read operations and improve overall database performance. Database caching can be implemented within Strapi using a caching layer that intercepts query results and stores them for future use.
Benefits:
Example Implementation:
// Using a custom service for query caching in Strapi
module.exports = {
async findCached(ctx) {
const cacheKey = 'your_unique_cache_key';
const cachedData = await strapi.services.cache.get(cacheKey);
if (cachedData) {
return cachedData;
}
const data = await strapi.services.yourService.find(ctx);
await strapi.services.cache.set(cacheKey, data, { ttl: 3600 }); // TTL in seconds
return data;
},
};
CDN caching involves deploying a network of geographically distributed servers that store copies of your static assets and serve them to users from the closest possible location. This reduces latency and speeds up the delivery of static content such as images, CSS files, and JavaScript files.
Benefits:
Example Configuration:
To include a CDN in your Strapi app, you might configure your static file serving middleware to point to your CDN.
// /config/middleware.js
module.exports = {
settings: {
'static': {
enabled: true,
config: {
publicPath: 'https://cdn.yourdomain.com/', // Your CDN URL
},
},
},
};
By understanding and effectively implementing these types of caching, you can ensure that your Strapi application is highly performant, can handle larger volumes of traffic, and delivers a superior user experience. Each caching type serves different needs and can be used in conjunction for optimal results.
## Configuring In-memory Caching in Strapi
In-memory caching can dramatically improve the performance of your Strapi application by temporarily storing frequently accessed data in fast, volatile memory. Using in-memory caches like Redis, you can reduce database load and speed up data retrieval. This section provides step-by-step instructions on setting up in-memory caching in Strapi using Redis and configuring cache policies.
### Step 1: Install Redis and Required Packages
First, you need to have Redis installed on your server. If Redis is not already installed, you can install it using the package manager of your choice:
For Ubuntu:
```sh
sudo apt update
sudo apt install redis-server
For macOS:
brew install redis
Next, install the necessary npm packages in your Strapi project. You will need the ioredis
package for interacting with Redis.
npm install ioredis
Create a configuration file for Redis inside the config
folder of your Strapi project. If the config
folder doesn’t exist, create it first.
Create a file config/redis.js
:
const Redis = require('ioredis');
module.exports = ({ env }) => ({
defaultConnection: 'default',
connections: {
default: {
connector: 'redis',
settings: {
host: env('REDIS_HOST', '127.0.0.1'),
port: env('REDIS_PORT', 6379),
db: env('REDIS_DB', 0),
password: env('REDIS_PASSWORD', null),
},
options: {},
},
},
});
Next, integrate Redis caching into your Strapi services. For instance, to cache API responses, you can modify a service like article.js
located in api/article/services
.
First, include the Redis client at the top of your service file:
const Redis = require('ioredis');
const redisClient = new Redis();
Then, implement caching within the service operations. For example, to cache the result of a 'find' operation:
async find(params) {
const cacheKey = `articles::${JSON.stringify(params)}`;
const cached = await redisClient.get(cacheKey);
if (cached) {
return JSON.parse(cached);
}
const articles = await strapi.query('article').find(params);
await redisClient.set(cacheKey, JSON.stringify(articles), 'EX', 3600); // Cache for 1 hour
return articles;
}
To fine-tune your caching strategy, you can implement cache policies according to your application’s needs. This includes specifying cache duration, setting up namespaced keys, and handling cache invalidation.
In the example above, we set the cache expiration to 1 hour using the 'EX'
option in Redis. You can adjust this value based on how frequently your data changes.
Ensure your Redis instance is secured, especially if it's exposed to the internet. Use strong passwords and restrict access by binding Redis to your localhost or specific IP addresses:
In redis.conf
:
bind 127.0.0.1 ::1
requirepass your-secure-password
By setting up in-memory caching with Redis in Strapi, you can significantly improve data retrieval speeds and reduce database load. This step-by-step guide helps you get started with basic configurations. Always remember to monitor your cache performance and adjust policies as needed for optimal results.
When it comes to enhancing the performance of your Strapi application, caching database queries plays a crucial role. By storing the results of frequent or expensive database queries in a cache, we can significantly reduce the load on our database and accelerate response times for end-users. In this section, we'll cover guidelines on how to efficiently implement database query caching in Strapi.
Caching database queries provides several benefits:
Follow these steps to implement database query caching in Strapi:
A popular choice for caching in Node.js applications is Redis due to its speed and simplicity. Ensure you have Redis installed and running. For more detailed information on setting up Redis, refer to the official Redis documentation.
To interact with Redis from your Strapi application, you need a Redis client. We recommend ioredis
for its comprehensive functionality.
Run the following command to install ioredis
:
npm install ioredis
Create a Redis client instance in Strapi. For simplicity, you can configure it within a middleware or a service.
const Redis = require("ioredis");
const redis = new Redis();
module.exports = redis;
Here's how you can cache database queries in Strapi:
// services/cache.js
const redis = require('../config/redis');
const CACHE_TTL = 60 * 5; // Cache Time-To-Live: 5 minutes
module.exports = {
async get(key) {
const data = await redis.get(key);
return data ? JSON.parse(data) : null;
},
async set(key, value) {
await redis.set(key, JSON.stringify(value), 'EX', CACHE_TTL);
},
};
In your controllers or services, wrap the database fetching logic with the cache:
// services/article.js
const cacheService = require('./cache');
async function getArticles() {
const cacheKey = 'articles_all';
// Check if data is in cache
const cachedData = await cacheService.get(cacheKey);
if (cachedData) {
return cachedData;
}
// If not in cache, fetch from database
const articles = await strapi.query('article').find();
// Store the database result in cache
await cacheService.set(cacheKey, articles);
return articles;
}
module.exports = {
getArticles,
};
By following this pattern, subsequent requests for articles will fetch the data from Redis instead of querying the database, significantly improving response times.
It's crucial to ensure cached data is invalidated when the underlying data changes. Implement cache invalidation logic within your Strapi lifecycle hooks:
// config/functions/lifecycle.js
const cacheService = require('../../services/cache');
module.exports = {
lifecycles: {
async afterCreate(result, data) {
await cacheService.set('articles_all', null);
},
async afterUpdate(result, params, data) {
await cacheService.set('articles_all', null);
},
async afterDelete(result, params) {
await cacheService.set('articles_all', null);
},
},
};
While implementing database query caching involves some setup and maintenance tasks like handling cache invalidation, the performance gains are well worth the effort. Caching database queries in Strapi with Redis can drastically reduce your database load and provide your users with faster responses. Remember to monitor the cache effectiveness regularly to ensure your strategy remains optimal.
Content Delivery Networks (CDNs) play a pivotal role in enhancing the performance of web applications by caching static assets and routing traffic through a distributed network of servers. Integrating a CDN with your Strapi application can drastically reduce load times and improve the user experience by delivering content more efficiently. In this section, we'll explore how to integrate CDNs with Strapi, focusing on caching static assets and effective traffic routing.
A CDN is a network of servers strategically distributed across various geographical locations. Its primary goal is to deliver content to users with minimal latency and high availability by caching static assets such as images, CSS files, JavaScript files, and other media. When a user requests a resource, the CDN serves the content from the server closest to the user's location, significantly reducing load times.
Choose a CDN Provider: Popular CDN providers include Cloudflare, Amazon CloudFront, Akamai, and Fastly. For this guide, we'll use Cloudflare as an example, but the principles apply to other providers as well.
Set up a CDN Account: Create an account with your chosen CDN provider and follow their instructions to set up a new distribution.
Configure Your CDN:
Configure your CDN to cache specific paths and file types. For example, in Cloudflare, you can set up rules to cache assets like *.css
, *.js
, *.jpg
, etc.
Update Your DNS Settings: Point your domain's DNS settings to the CDN's nameservers as provided by your CDN provider. This step routes all traffic through the CDN.
Integrate CDN URLs in Strapi: Update your Strapi application to serve static assets through the CDN. Typically, this involves setting environment variables or configuring your asset URLs in Strapi to point to the CDN.
Here is an example configuration in your Strapi project:
config/plugins.js
module.exports = ({ env }) => ({
upload: {
provider: 'local',
providerOptions: {
sizeLimit: 1000000, // Maximum file size in bytes
},
actionOptions: {
upload: {
// Add your CDN URL here
baseUrl: env('CDN_BASE_URL', 'https://your-cdn-url.com'),
// Add other configurations if needed
},
},
},
});
config/env/production/server.js
module.exports = ({ env }) => ({
host: env('HOST', '0.0.0.0'),
port: env.int('PORT', 1337),
url: env('CDN_BASE_URL', 'https://your-cdn-url.com'),
});
Sign Up for Cloudflare:
DNS Configuration:
Page Rules for Caching:
URL: *your-domain.com/assets/*
Setting: Cache Level: Cache Everything, Edge Cache TTL: a month
Purge Cache on Deploy:
Here is a sample script using the Cloudflare API:
curl -X POST "https://api.cloudflare.com/client/v4/zones/YOUR_ZONE_ID/purge_cache" \
-H "X-Auth-Email: YOUR_CLOUDFLARE_EMAIL" \
-H "X-Auth-Key: YOUR_CLOUDFLARE_API_KEY" \
-H "Content-Type: application/json" \
--data '{"purge_everything":true}'
By integrating a CDN with your Strapi application, you can significantly improve load times and overall site performance. The distributed nature of CDNs ensures that static assets are served quickly from the nearest available server, enhancing the user experience across different geographical locations. Remember to continuously monitor and optimize your CDN configuration to achieve the best possible performance outcomes.
Cache-Control headers play a crucial role in managing the caching behavior of HTTP responses. By carefully setting these headers, you can optimize how your content is cached and served to users, reducing load times and server stress. This section will guide you through the process of setting up and managing Cache-Control headers in a Strapi application.
Before diving into implementation, it's important to understand what Cache-Control headers do. They instruct the browser and intermediate caches (like CDNs and proxy servers) on how to handle the cached content. Some common directives include:
public
: Indicates that the response can be cached by any cache.private
: Indicates that the response is intended for a single user and should not be stored by shared caches.no-cache
: Forces caches to submit a request to the origin server for validation before releasing a cached copy.no-store
: Prevents the response from being cached anywhere.max-age=<seconds>
: Specifies the maximum amount of time the resource is considered fresh.must-revalidate
: Forces caches to obey any freshness information you give them.Strapi provides flexibility to manage HTTP headers, including Cache-Control. Follow these steps to configure Cache-Control headers in your Strapi application:
You will need to create or modify a middleware to set these headers. Navigate to the ./config/middleware.js
file in your Strapi project.
module.exports = ({ env }) => [
// Other middleware configurations
{
name: 'cache-control',
configure: {
enabled: true,
after: 'parser', // Make sure this comes after the parser middleware
load: {
initialize: (middlewares, { strapi }) => {
return async (ctx, next) => {
if (ctx.method === 'GET') {
// Customize the Cache-Control header as needed
ctx.set('Cache-Control', 'public, max-age=3600, must-revalidate');
}
await next();
};
},
},
},
},
];
Make sure that the middleware is applied in your Strapi configuration. This should be done in your ./config/middleware.js
.
module.exports = [
// Other middleware
'cache-control',
];
In some cases, you may want different caching policies for different routes. You can achieve this by adding custom logic within the middleware to differentiate based on the route path.
module.exports = ({ env }) => [
// Other middleware configurations
{
name: 'cache-control',
configure: {
enabled: true,
after: 'parser',
load: {
initialize: (middlewares, { strapi }) => {
return async (ctx, next) => {
if (ctx.method === 'GET') {
// Customize per route
if (ctx.path.startsWith('/api/public-content')) {
ctx.set('Cache-Control', 'public, max-age=3600');
} else if (ctx.path.startsWith('/api/private-content')) {
ctx.set('Cache-Control', 'private, no-cache');
} else {
ctx.set('Cache-Control', 'no-store');
}
}
await next();
};
},
},
},
},
];
must-revalidate
and other directives helps ensure that users always get the most up-to-date content.Setting up Cache-Control headers in Strapi requires thoughtful configuration to balance performance and freshness of the content. By implementing these headers and fine-tuning them based on your application's needs, you can achieve substantial performance gains, making the user experience smoother and more responsive.
In the next sections, we will delve into various cache invalidation strategies and monitoring techniques to ensure your caching setup remains optimal as your application scales and evolves.
Ensuring that users receive the most up-to-date content while effectively utilizing caching mechanisms can be tricky. Cache invalidation is the process of clearing out old or outdated data from the cache when updates occur. If not managed correctly, stale data can be served, which undermines the purpose of having a dynamic, content-rich CMS like Strapi. In this section, we'll discuss various cache invalidation strategies to help maintain the balance between cache efficiency and data freshness.
Time-To-Live (TTL) is one of the simplest and most common cache invalidation strategies. By assigning an expiration time to cached data, you can ensure that the data is automatically invalidated after a specific duration.
To set a TTL in Redis, you can use the EXPIRE
command when caching data:
const redis = require('redis');
const client = redis.createClient();
// Store a value with a TTL of 60 seconds
client.set('key', 'value', 'EX', 60);
Manual invalidation involves explicitly removing or updating the cache at specific points in your application. This is particularly useful for operations that modify the underlying data, such as create, update, and delete operations.
Strapi’s lifecycle hooks can be utilized to invalidate cache manually upon content updates:
module.exports = {
lifecycles: {
async afterCreate(result, data) {
// Invalidate cache after creating a new entry
await redisClient.del('cachedDataKey');
},
async afterUpdate(result, params, data) {
// Invalidate cache after updating an entry
await redisClient.del('cachedDataKey');
},
async afterDelete(result, params) {
// Invalidate cache after deleting an entry
await redisClient.del('cachedDataKey');
},
},
};
Batch invalidation aggregates multiple invalidation requests and executes them in one go. This can be useful for paginated data or bulk updates where individual invalidations would be too costly.
const keysToInvalidate = ['key1', 'key2', 'key3'];
// Use Redis's pipeline to batch invalidate multiple keys
const pipeline = redisClient.pipeline();
keysToInvalidate.forEach(key => pipeline.del(key));
pipeline.exec();
This strategy involves invalidating the cache based on specific conditions or triggers, such as changes in related content or metadata. It is used to maintain cache coherence in interconnected datasets.
Suppose you have a blog post and comments, and any update in comments should invalidate the blog post cache:
module.exports = {
lifecycles: {
async afterUpdate(result, params, data) {
if (params.model == 'comment') {
// Invalidate the related blog post cache
const blogPostId = result.blogPostId;
await redisClient.del(`blogPost_${blogPostId}`);
}
},
},
};
This strategy serves a slightly stale version of the data while asynchronously updating the cache. Users get quick responses without waiting for revalidation processes to complete.
ctx.set('Cache-Control', 'stale-while-revalidate=30');
Mechanisms like cache stampede prevention should also be considered. Using tools such as Memcached or Redis, implementing locks, and throttling can prevent the more common pitfalls in cache invalidation.
By implementing these cache invalidation strategies, you can better manage the latency-vs-freshness conundrum and ensure your Strapi application delivers up-to-date content efficiently:
Proactively managing cache invalidation can dramatically improve your application's performance and reliability, ensuring that users have access to the latest content without compromising on speed.
Once you've implemented various caching strategies in Strapi, the next critical step is to monitor and analyze their effectiveness. Proper monitoring helps you understand the impact of caching on your application's performance and provides insights to fine-tune your strategies for optimal results. This section delves into the tools and practices you can use to monitor and analyze cache performance, with a focus on hit-miss ratios, response times, and overall efficiency.
If you're using Redis for in-memory caching, the Redis Insights tool offers a comprehensive dashboard for monitoring your Redis instance. You can view metrics like:
You can install Redis Insights via Docker with the following command:
docker run -d --name redisinsight -p 8001:8001 redislabs/redisinsight
For those who use MongoDB as their database provider, MongoDB Atlas comes with built-in monitoring tools that help you keep an eye on database query performance. These insights can guide your database query caching strategies.
Key metrics to track include:
Strapi allows for extending its functionalities, and custom monitoring is no exception. You can create custom middleware to log cache performance metrics. Here’s a simple example:
const monitorCache = (req, res, next) => {
const start = process.hrtime.bigint();
res.on('finish', () => {
const end = process.hrtime.bigint();
const duration = (end - start) / BigInt(1000000); // Convert to milliseconds
const cacheStatus = res.get('X-Cache-Status'); // Assuming 'X-Cache-Status' is set in headers
console.log(\`[Monitor] \${req.method} \${req.url} - Cache Status: \${cacheStatus} - Duration: \${duration} ms\`);
});
next();
};
module.exports = monitorCache;
You can then add this middleware to your Strapi application:
// In your middlewares setup
module.exports = {
load: {
before: [
'yourCustomMiddleware',
],
after: [],
},
settings: {
yourCustomMiddleware: {
enabled: true,
path: './middlewares/monitorCache',
},
},
};
A high hit ratio indicates efficient caching, where most of the data requests are served from the cache. On the other hand, a low hit ratio suggests that the cache strategies might need revisiting.
Monitor the average response times for your API endpoints and static assets. Faster response times generally indicate effective caching strategies. Tools like New Relic and Datadog can offer deeper insights into response times and other performance metrics.
Regularly check the eviction and invalidation rates. High rates may indicate that your cache size is too small or that your invalidation strategy needs adjustments. Ensure that your cache retains frequently accessed data and evicts the least used items effectively.
Grafana, in combination with Prometheus, can be exceptionally useful for visualizing caching metrics. Set up dashboards to track:
By integrating these monitoring tools and practices, you can gain a comprehensive understanding of how caching is impacting your Strapi application. This will enable you to make data-driven decisions and continuously optimize your caching strategies.
This section lays the groundwork for effective monitoring and analysis of caching in Strapi, helping you ensure that your caching strategies are both efficient and effective.
To further enhance the performance of your Strapi application, employing advanced caching techniques can make a significant difference. This section delves into two such techniques: Edge-side Includes (ESI) and partial content caching. These methods provide more granular control over what gets cached and when, leading to better usability and resource management.
Edge-Side Includes (ESI) is a powerful technique for dynamic content assembly. ESI allows portions of web pages to be cached and served from a content delivery network (CDN) while enabling dynamic updates for specific page components. This method provides a way to mix cached and fresh content in a single HTTP request, significantly reducing the server's load and improving response times.
<esi:include>
tags.To implement ESI in your Strapi application, follow these steps:
Identify Dynamic and Static Parts: Determine which parts of your content can be cached and which need to be dynamically loaded.
Add ESI Tags: Update your Strapi templates to include ESI tags for dynamic components.
<!-- Example ESI tag usage -->
<html>
<body>
<!-- Static Content -->
<header>
<esi:include src="/header.html" />
</header>
<!-- Dynamic Content -->
<main>
<esi:include src="/api/dynamic-content" />
</main>
<!-- Static Content -->
<footer>
<esi:include src="/footer.html" />
</footer>
</body>
</html>
Configure CDN: Ensure your CDN supports ESI and configure it accordingly to process these tags.
Partial content caching allows you to cache specific parts of a response rather than the entire response. This is particularly useful for Strapi applications where some elements of a page can be cached for longer durations compared to others.
Here's an example using Node.js with memory caching for specific parts of a response:
const cache = require('memory-cache');
const CACHE_DURATION = 60000; // 60 seconds
module.exports = {
async find(ctx) {
const cacheKey = ctx.request.url;
const cachedResponse = cache.get(cacheKey);
if (cachedResponse) {
ctx.body = cachedResponse;
return;
}
const response = await strapi.services.article.find(ctx.query);
cache.put(cacheKey, response, CACHE_DURATION);
ctx.body = response;
}
};
By incorporating ESI and partial content caching into your Strapi application, you can significantly enhance performance and provide a seamless user experience. As always, make sure to test these strategies using LoadForge to ensure they are effectively reducing load times and improving scalability.
Once you've implemented various caching strategies in your Strapi application, it is paramount to ensure that your optimizations effectively handle the anticipated load. LoadForge is an invaluable tool for load testing, allowing you to simulate high-traffic scenarios and validate the performance improvements achieved through caching. Below, we outline guidelines for leveraging LoadForge to perform load testing on your Strapi application.
Create a LoadForge Account:
Set Up a Load Testing Project:
Define Test Scenarios:
Configure Your Test Plan:
Example load test plan:
{
"test_duration": "10 minutes",
"concurrent_users": 100,
"ramp_up_period": "5 minutes"
}
Setup Testing Endpoints:
Example setup:
[
{
"method": "GET",
"url": "https://your-strapi-app.com/api/posts",
"weight": 70
},
{
"method": "POST",
"url": "https://your-strapi-app.com/api/comments",
"payload": {
"comment": "This is a test comment"
},
"weight": 30
}
]
Run the Load Test:
If the load test exposes any performance bottlenecks, consider the following steps for optimization:
Always re-test your Strapi application after making any changes to validate improvements. Continuous load testing ensures that your application remains robust and performant as you evolve your caching strategies.
By using LoadForge effectively, you're equipped to measure and optimize the performance of your Strapi application, ensuring a responsive experience for your users even under heavy load.
Next Steps: Continue to the next section on "Conclusion" to encapsulate key takeaways and best practices.
By integrating LoadForge in your load testing workflow, you can confidently validate your Strapi application's performance, ensuring it remains efficient and reliable under varying traffic conditions.
In this guide, we've comprehensively explored how caching can be leveraged to enhance the performance of your Strapi CMS application. By implementing various caching techniques and strategies, you can significantly reduce response times, decrease load on your server, and improve overall user experience.
Importance of Caching:
Types of Caching:
Configuring In-memory Caching:
{
"host": "127.0.0.1",
"port": 6379,
"password": "your_redis_password"
}
Database Query Caching:
Using CDNs:
Implementing Cache-Control Headers:
{
"Cache-Control": "public, max-age=3600"
}
Cache Invalidation Strategies:
Monitoring and Analyzing Cache Performance:
Advanced Caching Techniques:
Leveraging LoadForge for Load Testing:
Caching is not a one-time setup; it requires ongoing attention. Regularly analyze your caching logs, monitor performance metrics, and use load testing tools like LoadForge to validate your caching strategies under different conditions. Continuous optimization ensures that your Strapi application remains performant and responsive as your data and user base grow.
By adhering to the best practices and strategies outlined in this guide, you can maximize the performance benefits that caching offers in Strapi, providing a faster and more efficient experience for your users.