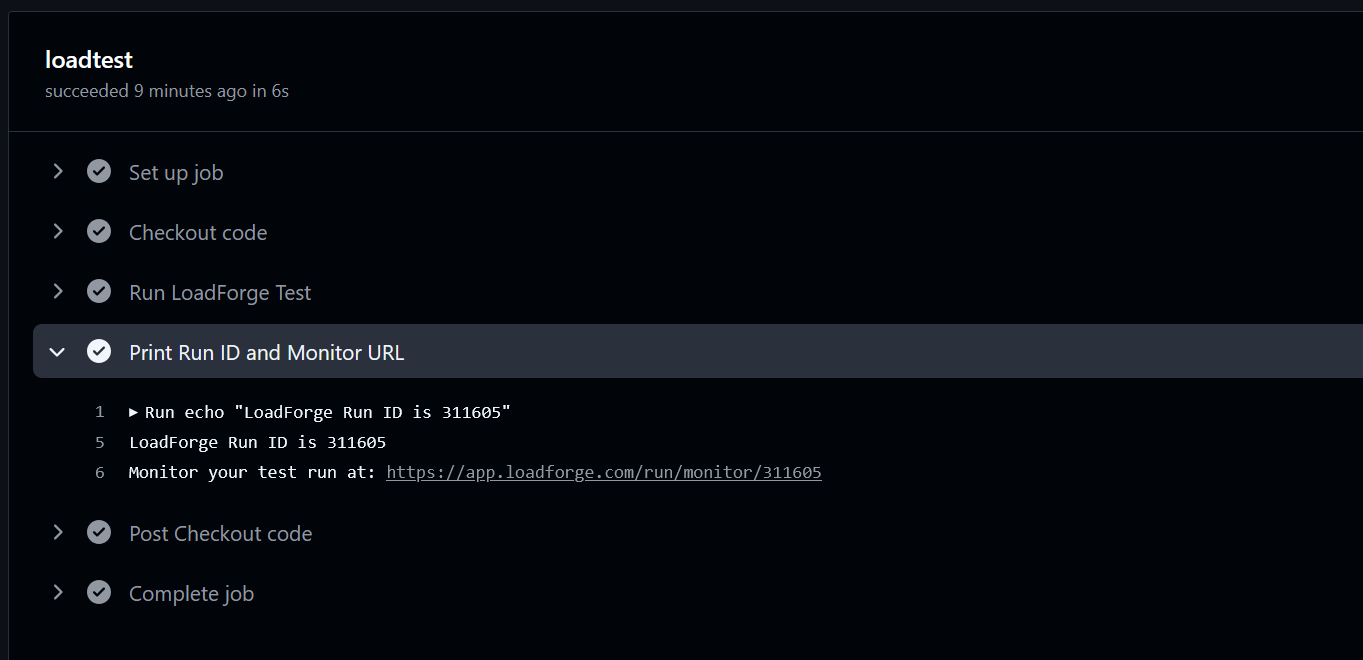
LoadForge GitHub Integration
Performance testing just got a major upgrade. LoadForge is thrilled to announce a seamless GitHub integration that lets you launch...
Learn how to send an HTTP POST request with a JSON data payload using LoadForge.
You are now browsing the LoadForge locust test directory. You can use these tests as a starting point for your own tests, or use our AI wizard to generate one automatically.
Using LoadForge, sending HTTP POST requests with JSON data is straightforward. Before making the request, you need to prepare and parse your JSON data. This documentation will guide you on how to send a POST request with JSON data using LoadForge.
To send JSON data via an HTTP POST request, the data needs to be serialized before transmission. In Python, you can utilize the json.dumps()
method to serialize your data.
In addition to preparing your data, you should also set the content type of your request to application/json
in the headers to inform the receiving server that you're sending JSON data.
In the provided example, a payload consisting of two key-value pairs will be posted to the /post/endpoint
. Follow the steps and the code sample below:
Setup and Imports
Start by importing necessary modules like time
, json
, and relevant locust
classes.
Define User Behavior
Create a class (QuickstartUser
) that extends the HttpUser
class from Locust. This will define the behavior of a simulated user.
Set Waiting Time
Use wait_time
to simulate the wait period between tasks. This example uses a randomized wait time of 3 to 5 seconds.
Define the Task
Within the user class, define a task named api_page
. This task sends a POST request with the payload.
import time, json
from locust import HttpUser, task, between
class QuickstartUser(HttpUser):
wait_time = between(3, 5)
@task(1)
def api_page(self):
payload = {
'key': 'value',
'question': 'answer123'
}
headers = {'content-type': 'application/json'}
response = self.client.post(
"/post/endpoint",
data=json.dumps(payload),
headers=headers
)
Note: There was a missing comma in the
payload
dictionary which has been corrected in the example above.
LoadForge is built on top of locust.io, allowing you to leverage the full capabilities of the open-source Locust framework. If you're already using Locust, consider importing your scripts to LoadForge to enhance and simplify your load testing process.
By following the above instructions, you can effectively send POST requests with JSON data using LoadForge. Remember always to prepare your data and set the appropriate headers to ensure successful data transmission.