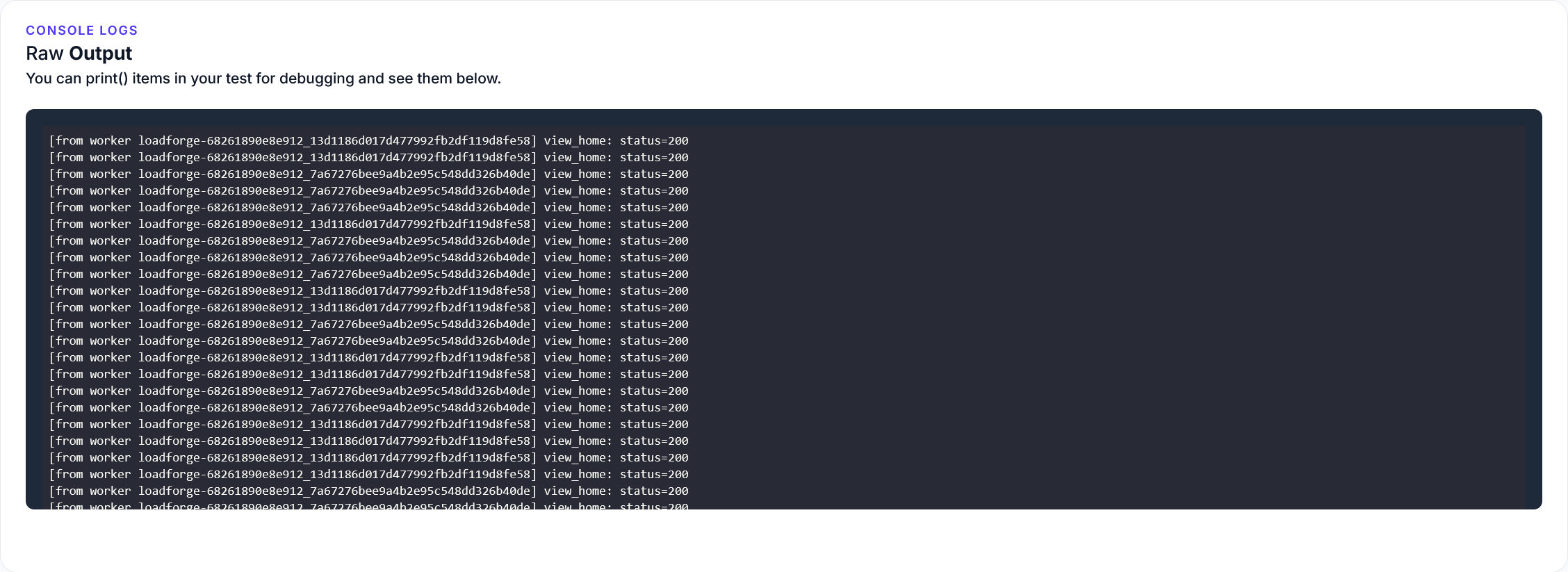
Easier Debug Logging
We're excited to announce a powerful new feature for LoadForge users: comprehensive debug logging for test runs. This highly requested...
In the context of web development, particularly with applications built using Laravel, understanding and optimizing database interactions is crucial for maintaining fast response times and efficient data handling. One common challenge that developers encounter is the N+1 query problem. This...
In the context of web development, particularly with applications built using Laravel, understanding and optimizing database interactions is crucial for maintaining fast response times and efficient data handling. One common challenge that developers encounter is the N+1 query problem. This issue can severely degrade the performance of your application, affecting user experience and increasing server load unexpectedly.
The N+1 query problem occurs when an application makes one initial query to the database followed by an additional query for each result obtained from the first query. This typically happens in object-relational mapping (ORM) frameworks when dealing with relationships between models.
For instance, imagine a scenario in a Laravel application where you need to display all posts and their associated comments on a webpage. An intuitive approach might involve loading all posts first and then iterating through each post to load its related comments:
$posts = Post::all();
foreach ($posts as $post) {
$comments = $post->comments; // Additional query for each post
}
In this example, if there are N
posts, the first query retrieves all the posts, and then N
additional queries are executed to fetch the comments for each post. Hence, for N
posts, there will be N+1
queries to the database: 1 for retrieving all the posts and N for fetching the comments for each post.
The N+1 query problem can lead to several performance issues:
Such performance degradation not only affects the backend efficiency but also impairs the front-end user experience. Users may experience slow page renders and sluggish interaction with the application, which could dissuade them from using the platform.
The following sections of this guide will dive into the methods for detecting and resolving N+1 query issues to optimize both developer and end-user experiences in Laravel-powered applications. By addressing these issues, you can improve your application's responsiveness, lower its resource demands, and ensure a smoother, more scalable solution.
In Laravel applications, N+1 query problems can subtly degrade performance, often going unnoticed during early stages of development. Understanding common scenarios where these issues arise and learning how to detect them are crucial for maintaining efficient database interactions and application responsiveness.
N+1 issues typically occur when an application retrieves a list of objects and then, for each object, makes additional queries to fetch related data. This is common in scenarios involving relational database relationships such as one-to-many or many-to-many associations.
For example, consider a blog application where each Post
has many Comments
. Fetching all posts along with their comments without proper query optimization can lead to an N+1 problem:
$posts = Post::all();
foreach ($posts as $post) {
$comments = $post->comments; // Additional query for each post
}
In this scenario, if there are N posts, there will be 1 query to fetch all posts plus N additional queries to fetch comments for each post, resulting in N+1 queries.
One of the most popular tools for identifying N+1 queries in a Laravel application is the Laravel Debugbar. It provides a visual representation of all database queries executed during a request, including the time each query takes. Here's how to utilize Laravel Debugbar to spot N+1 queries:
Installation: You can install Debugbar via Composer:
composer require barryvdh/laravel-debugbar --dev
This package is only registered in debug mode to avoid impact on production performance.
Configuration: After installation, Debugbar is automatically enabled in development environments. You can configure it further by publishing its config file:
php artisan vendor:publish --provider="Barryvdh\Debugbar\ServiceProvider"
Usage: With Debugbar enabled, perform operations on your application that load data relationships. Debugbar will show all executed queries under the "Queries" tab, allowing you to identify repeated queries that may be optimized.
Laravel Telescope is an elegant debug assistant for Laravel that provides insight into queries, jobs, exceptions, and more. It has built-in support for detecting N+1 queries:
Installation: Similarly to Debugbar, install Telescope via Composer:
composer require laravel/telescope --dev
Configuration and Usage: After installation, publish its assets and configure it:
php artisan telescope:install
php artisan migrate
Navigate to /telescope
on your application's URL to access Telescope's dashboard. Use the "Queries" panel to watch for repeated queries indicative of N+1 issues.
Identifying and resolving N+1 query issues early in the development lifecycle is essential. By leveraging tools like Laravel Debugbar and Telescope, developers can gain deep insights into application performance pitfalls and optimize data fetching strategies. Regularly checking your database queries during development and in code review stages will help avoid performance degradation and improve the scalability of your Laravel applications.
In this section, we examine practical scenarios to illustrate how N+1 query problems manifest in Laravel applications. By exploring real-world examples, we can better understand the process of identifying these issues and the impact they have on database and overall application performance.
Imagine a common feature in a blog application where each post can have multiple comments. Using Eloquent's lazy loading to retrieve comments for a batch of posts can inadvertently trigger an N+1 query issue.
Let's say you have the following code:
$posts = Post::all();
foreach ($posts as $post) {
echo $post->comments->count();
}
In the above scenario, first, all posts are retrieved from the database. However, for each post, another query is executed to count the comments, which results in one initial query to fetch the posts and then one query per post to fetch the comments. If there are 10 posts, this results in 1 (to fetch the posts) + 10 (to fetch comments for each post) = 11 queries.
Using tools like Laravel Debugbar or the N+1 query alert feature in Laravel Telescope, you can easily identify when multiple queries are executed in loops like the above example. These tools will highlight the repeated queries during a single request, signaling a potential N+1 problem.
The performance implications of this scenario include increased latency in page response times and higher database server load, which is less efficient and scalable, especially with increasing load and data volume.
Consider a social media platform where you need to load user profiles along with their friends, posts, and likes. Here’s how a naive approach may look:
$users = User::all();
foreach ($users as $user) {
echo $user->friends->count();
echo $user->posts->count();
echo $user->likes->count();
}
In this example, retrieving each user’s related data (friends, posts, likes) separately within a loop causes an N+1 query for each relationship.
With Laravel Telescope’s N+1 query detection, such instances are flagged when you load pages containing these loops. The query log will show repeated queries originating from the loop, which clearly illustrates how the N+1 issue compounds with multiple relationships.
The additional queries not only increase the network traffic between your application and the database but also complicate the database's work, slowing down response times and increasing CPU usage. This can hinder the scalability of the application, affecting the user experience negatively.
The prevention of N+1 queries in such cases involves modifying the approach to data retrieval, primarily utilizing Eloquent's eager loading feature:
$users = User::with('friends', 'posts', 'likes')->get();
foreach ($users as $user) {
echo $user->friends->count();
echo $user->posts->count();
echo $user->likes->count();
}
By using with()
, all related data is loaded in a few initial queries rather than multiple queries within the loop.
These examples highlight the practical implications of N+1 queries and underscore the importance of recognizing and addressing them in Laravel applications. As demonstrated, the impact on application performance can be significant, making it essential to employ appropriate query optimization strategies to maintain an efficient, scalable, and performant application.
In Laravel, an N+1 query problem typically arises when an Eloquent ORM model is used to load data from database relationships in a loop. Each iteration of the loop executes a separate query to retrieve related data, resulting in poor performance as the number of iterations grows. Fortunately, Laravel provides several methods to efficiently load related data and solve the N+1 query problem, primarily through eager loading.
with()
MethodEager loading is the process of retrieving related data along with the main model data in a single query, rather than executing additional queries later on. In Laravel, this can be accomplished using the with()
method on an Eloquent query. The with()
method accepts an array of relationships that you want to load alongside the primary model.
Example:
Consider a scenario where you need to display all posts and their associated comments. Without eager loading, this would typically result in an N+1 problem:
$posts = Post::all();
foreach ($posts as $post) {
echo $post->comments; // Additional query for each post
}
To solve this using eager loading, you can modify the query as follows:
$posts = Post::with('comments')->get();
foreach ($posts as $post) {
echo $post->comments; // Comments are already loaded and no extra query is executed
}
load()
to Eager Load on Existing ModelsWhile with()
is used for eager loading relations at query build time, the load()
method can be applied to an existing model instance that was retrieved without certain relationships.
Example:
If you have a post instance and later decide to load comments, you can do this without causing N+1 queries:
$post = Post::find(1);
// Later in the code, if you need comments
$post->load('comments');
with()
and load()
with()
when you know in advance which relationships you need to retrieve along with the main model. This is usually the most efficient approach and is well-suited for scenarios where the related models are required from the start.load()
when the requirement for related data is conditional or you don't initially need the data when retrieving the main model. This provides flexibility to load additional data as needed without initially loading it.By implementing these techniques judiciously, you can significantly enhance the performance and scalability of your Laravel applications, effectively managing database load and improving overall user experience.
In this section, we delve into more sophisticated techniques to help you tackle N+1 query problems in Laravel applications effectively. By smartly utilizing Laravel's query builder, implementing caching strategies, and optimizing batch operations, you can significantly boost your application's performance. We'll also cover best practices that ensure your application remains efficient and scalable.
Laravel's query builder is powerful, but when used improperly, it can lead to N+1 query issues. To prevent these, follow these advanced tips:
Select Only Required Columns: Reduce overhead by fetching only the columns you need from the database. This can be done elegantly using the select
method.
$users = User::select('id', 'name')->get();
Conditional Relationships: If you only need a relationship under certain conditions, use the when
method to conditionally load relationships.
$users = User::with(['posts' => function ($query) {
$query->where('status', 'published');
}])->get();
Caching is a vital strategy to minimize the repetition of database queries, including N+1 queries. Here are some effective ways to implement caching in a Laravel environment:
Cache Query Results: Store the results of queries or loaded relationships in the cache. Use Laravel's cache tools to remember these results for repeated use.
$users = Cache::remember('users', $minutes, function () {
return User::with('posts')->get();
});
Tagged Cache for Complex Applications: For larger applications, use tagged caching to invalidate related caches collectively, preventing stale data.
Batch operations can reduce the number of queries by handling multiple tasks in a single query. Use these techniques:
Chunking Results: Process large datasets in chunks rather than all at once to keep memory usage low and avoid performance hits.
User::chunk(100, function ($users) {
foreach ($users as $user) {
// Process each user
}
});
Mass Insertions and Updates: Use insert
and update
methods to handle multiple rows in one operation, significantly reducing database trips.
User::insert([
['name' => 'John', 'email' => '[email protected]'],
['name' => 'Jane', 'email' => '[email protected]']
]);
Maintaining optimal performance isn't just about writing efficient code; it's about adopting practices that keep your application running smoothly:
Regularly Review Queries: Use Laravel Telescope or Debugbar to continually review and optimize your queries. Look for new N+1 issues as your application evolves.
Implement Performance Testing: Regularly test your application's performance using tools like LoadForge to simulate real-world load scenarios and identify bottlenecks early in the development cycle.
Continuous Integration: Integrate performance checks into your CI/CD pipelines to ensure that performance regressions are caught before deployment.
Stay Updated: Keep your Laravel application and its dependencies up to date. Newer versions often include optimizations that can improve performance.
By implementing these advanced solutions and adhering to best practices, you can ensure that your Laravel applications remain robust, responsive, and scalable. It's not only about fixing N+1 queries but also about fostering a culture of performance optimization within your development workflow.
Ensuring that your Laravel application operates efficiently and remains performant under various loads is an essential part of the development and maintenance process. Without thorough testing and continuous monitoring, N+1 query problems might only surface under real-world usage conditions, leading to poor user experiences and potentially heavy server loads. This section explores robust strategies for testing and monitoring the performance of Laravel applications, focusing on leveraging LoadForge for performance testing, using monitoring tools effectively, and integrating these processes into your CI/CD pipelines.
LoadForge is a powerful tool designed to simulate high traffic and analyze the performance of web applications under load. Implementing LoadForge tests allows you to understand how your Laravel application behaves when facing numerous simultaneous requests, specifically focusing on how efficiently your database handles these situations – an essential check against N+1 query issues.
Here’s a basic guide on how to setup LoadForge for testing a Laravel application:
Create Your Load Test Script: Define your user scenarios by scripting typical user interactions with your application. Here is a simple example where users perform queries that could expose N+1 issues:
from loadforge.https import User
class QuickTest(User):
def run(self):
# A GET request to a page that could have N+1 query problems
self.client.get("/products")
self.client.get("/products/details")
Configure and Run Your Test: Upload the script to LoadForge, set the number of users and the test duration, then start the test. LoadForge will generate a detailed report showing response times, failure rates, and other critical metrics.
Analyze Results: Look specifically for increases in response times as user load increases, which might suggest inefficient database queries or other performance bottlenecks.
Constant monitoring is critical in proactively detecting performance issues before they affect users. Integration of monitoring tools like Laravel Telescope provides real-time insights into query performance and system health. Here’s how to set it up:
Install Telescope:
composer require laravel/telescope
php artisan telescope:install
php artisan migrate
Use Telescope to Monitor Queries: Access the Telescope dashboard at your laravel app’s /telescope
URL. Keep an eye on the "Queries" tab to spot any sudden increase in query load or slow queries indicative of N+1 issues.
Embedding performance checks into your continuous integration (CI) workflow ensures that N+1 queries and other inefficiencies are caught before code reaches production. Implement these steps:
Automate Load Testing: Use CI tools like Jenkins, GitLab CI, or GitHub Actions to automate LoadForge tests as part of your CI pipeline.
# Sample GitHub Action setup
jobs:
performance-test:
runs-on: ubuntu-latest
steps:
- name: Run LoadForge Test
run: curl -X POST https://api.loadforge.com/runs -d "token=YOUR_TOKEN&id=LF_TEST_ID"
Use Service Hooks for Alerts: Configure your CI tools and monitoring solutions to send alerts if performance metrics degrade beyond acceptable thresholds.
Combining LoadForge for detailed stress tests, continuous monitoring via Laravel Telescope, and integrating these tools into your CI/CD pipelines sets a comprehensive backdrop for maintaining the performance integrity of your Laravel application. This proactive approach allows for timely remediation of any issues, ensuring optimal performance and a high-quality user experience.